How to Get Departments with the FreshService API in Javascript
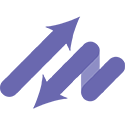
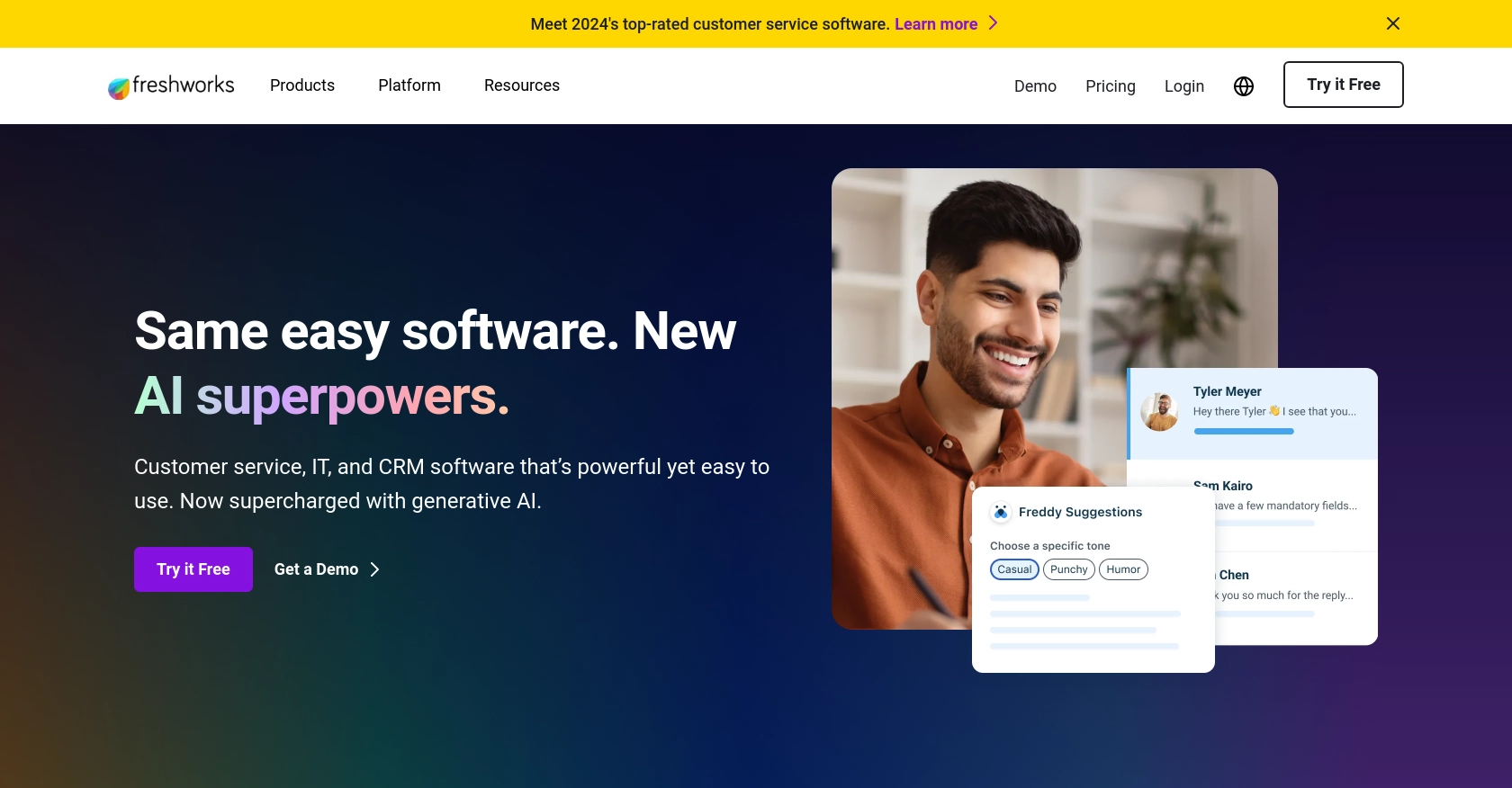
Introduction to FreshService API Integration
FreshService is a cloud-based IT service management solution designed to simplify IT operations for businesses of all sizes. It offers a comprehensive suite of tools for incident management, asset management, change management, and more, making it a popular choice for IT teams looking to streamline their processes.
Integrating with the FreshService API allows developers to automate and enhance various IT service management tasks. For example, you might want to retrieve department information to generate reports or integrate with other systems for a more cohesive IT management experience.
This article will guide you through the process of using JavaScript to interact with the FreshService API, specifically focusing on retrieving department data. By the end of this tutorial, you'll be equipped with the knowledge to efficiently access and manage department information within FreshService using JavaScript.
Setting Up Your FreshService Test Account
Before you can start interacting with the FreshService API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a FreshService Account
If you don't already have a FreshService account, you can sign up for a free trial on the FreshService website. This trial will give you access to all the features you need to test the API integration.
- Visit the FreshService signup page.
- Fill out the required information to create your account.
- Once your account is created, log in to access the FreshService dashboard.
Generating API Key for FreshService
FreshService uses a custom authentication method that involves generating an API key. This key will be used to authenticate your API requests.
- Log in to your FreshService account.
- Navigate to the profile icon in the top-right corner and select "Profile Settings."
- Under the "API Key" section, click on "Generate New API Key."
- Copy the generated API key and store it securely, as you'll need it for making API calls.
Configuring Access Permissions
Ensure that your API key has the necessary permissions to access department data. You may need to adjust settings in your FreshService account to allow this.
- Go to "Admin" settings in the FreshService dashboard.
- Select "API" from the menu and review the permissions associated with your API key.
- Ensure that the key has read access to department information.
With your FreshService account set up and your API key generated, you're ready to start making API calls to retrieve department data using JavaScript.
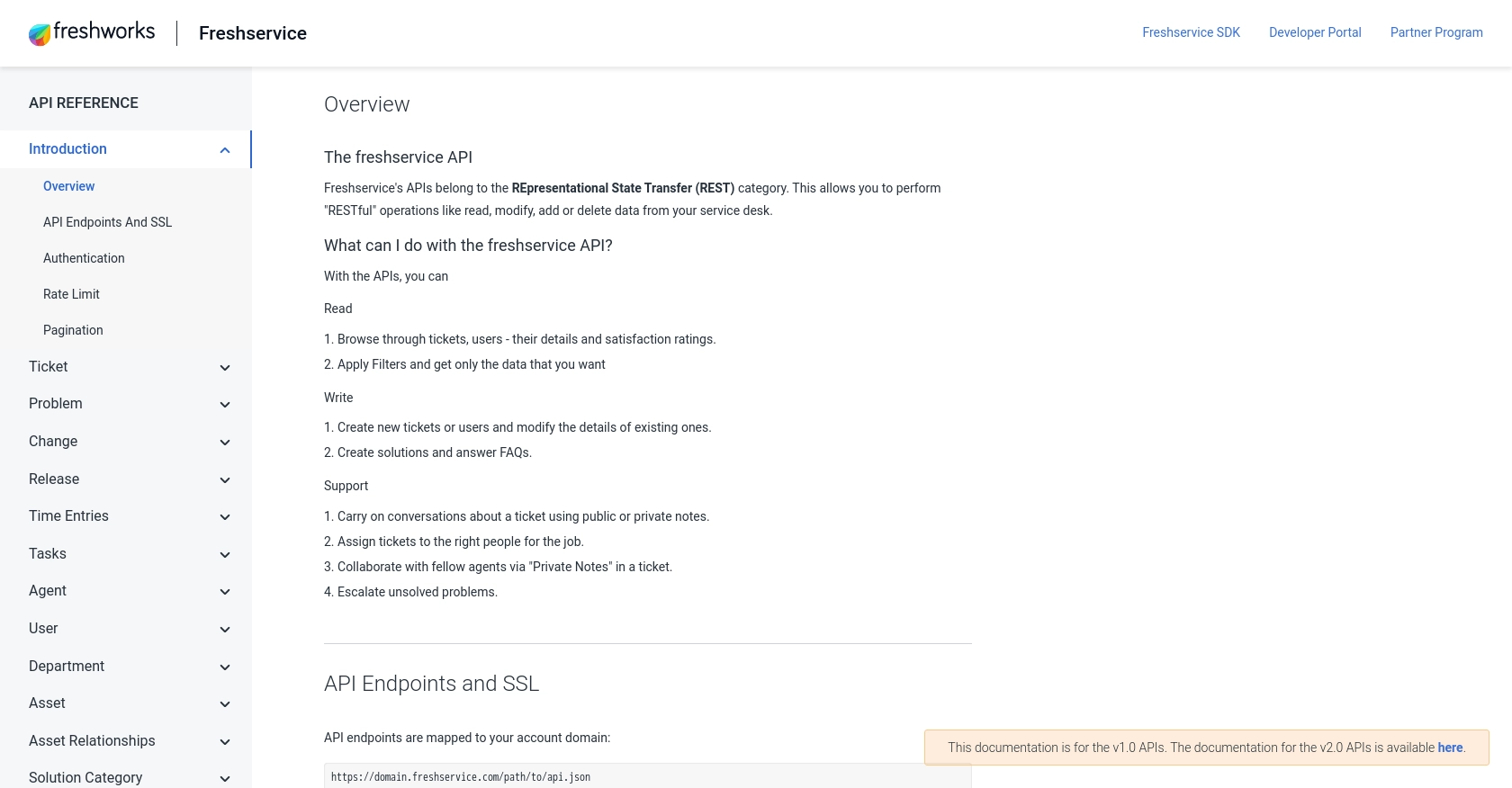
sbb-itb-96038d7
Making API Calls to Retrieve Department Data from FreshService Using JavaScript
Now that you have your FreshService account set up and your API key ready, it's time to dive into making API calls using JavaScript. This section will guide you through the process of retrieving department data from FreshService, ensuring you have all the necessary tools and knowledge to interact with the API effectively.
Setting Up Your JavaScript Environment for FreshService API
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js for server-side applications or a browser-based environment for client-side applications. For this tutorial, we'll focus on using Node.js.
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the Axios library to handle HTTP requests by running
npm install axios
.
Writing JavaScript Code to Fetch Department Data from FreshService
With your environment set up, you can now write the JavaScript code to interact with the FreshService API and retrieve department data.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://yourdomain.freshservice.com/api/v2/departments';
const apiKey = 'Your_API_Key';
const headers = {
'Content-Type': 'application/json',
'Authorization': `Basic ${Buffer.from(apiKey + ':X').toString('base64')}`
};
// Function to get departments
async function getDepartments() {
try {
const response = await axios.get(endpoint, { headers });
const departments = response.data.departments;
console.log('Departments:', departments);
} catch (error) {
console.error('Error fetching departments:', error.response ? error.response.data : error.message);
}
}
// Call the function
getDepartments();
Replace Your_API_Key
with the API key you generated earlier. This code uses Axios to send a GET request to the FreshService API endpoint for departments. The response is then logged to the console, displaying the department data.
Verifying Successful API Calls and Handling Errors
After running the code, you should see the department data printed in your console. If the request is successful, the data will match the departments available in your FreshService account.
In case of errors, the code is designed to catch and log them. Common errors might include authentication issues or incorrect endpoint URLs. Ensure your API key is correct and that you have the necessary permissions to access department data.
By following these steps, you can efficiently retrieve department data from FreshService using JavaScript, enabling you to integrate this information into your applications seamlessly.
Conclusion and Best Practices for FreshService API Integration
Integrating with the FreshService API using JavaScript provides a powerful way to automate and enhance IT service management tasks. By retrieving department data, developers can create seamless integrations that improve reporting and system cohesion.
Best Practices for Secure and Efficient FreshService API Usage
- Secure API Key Storage: Always store your API keys securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of FreshService's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from FreshService is standardized before integrating it with other systems. This helps maintain data consistency across platforms.
- Error Handling: Implement robust error handling to manage potential API call failures. Log errors for troubleshooting and provide meaningful feedback to users.
Streamline Your Integrations with Endgrate
While integrating with FreshService directly is a valuable skill, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including FreshService.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?