Using the Shopify API to Create or Update Product Variants (with Python examples)
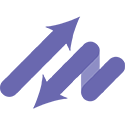
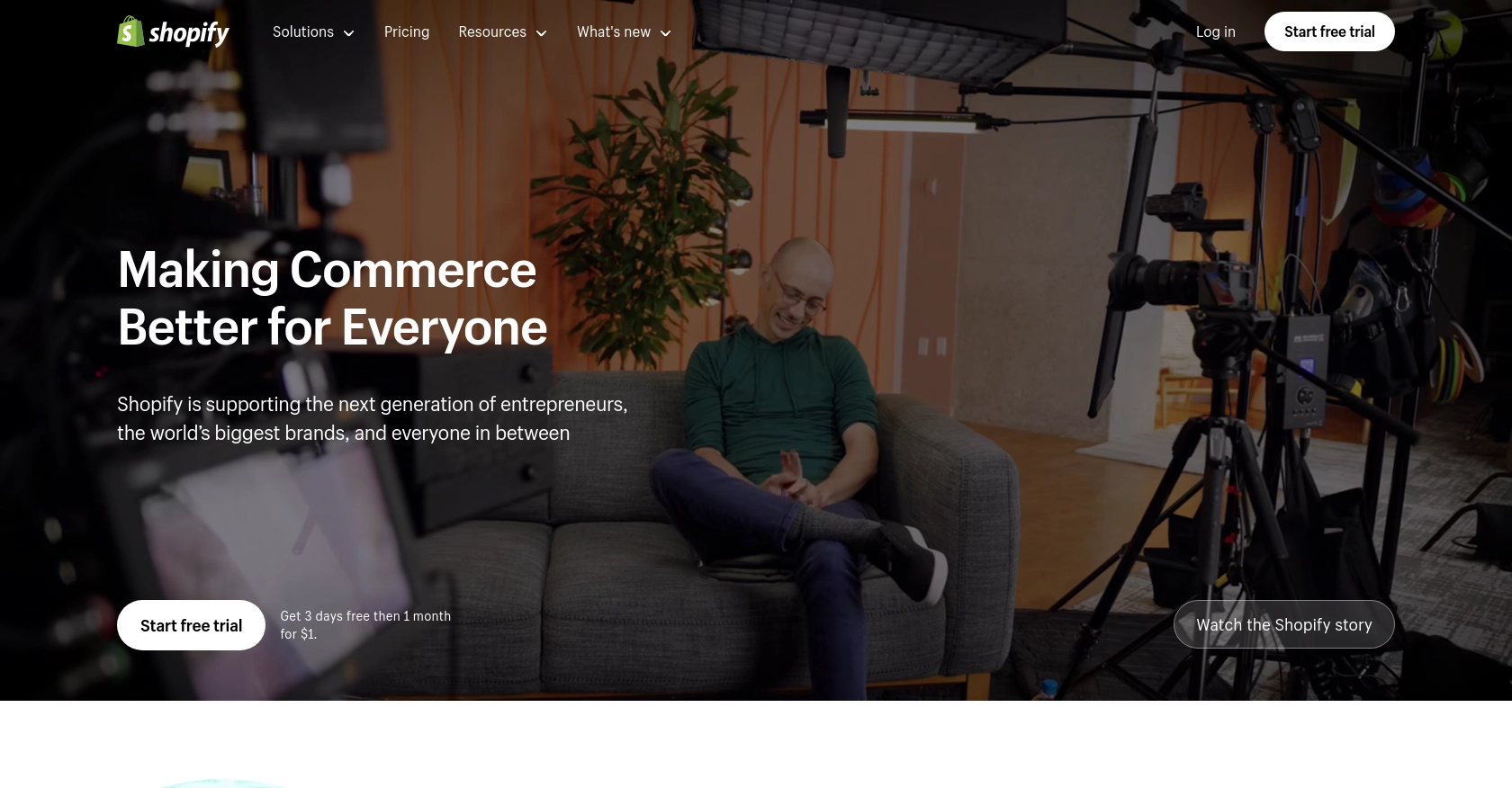
Introduction to Shopify API for Product Variants
Shopify is a leading e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a robust set of tools and features that cater to businesses of all sizes, enabling them to sell products, manage inventory, and process payments efficiently.
Integrating with Shopify's API allows developers to automate and enhance various aspects of store management. One common use case is managing product variants, which are different versions of a product, such as size or color. By using the Shopify API, developers can programmatically create or update product variants, streamlining inventory management and ensuring that product listings are always up-to-date.
For example, a developer might use the Shopify API to automatically update product variant prices based on current market trends or inventory levels, ensuring competitive pricing and optimal stock levels.
Setting Up Your Shopify Test or Sandbox Account for API Integration
Before you can start working with the Shopify API to create or update product variants, you'll need to set up a Shopify test or sandbox account. This environment allows you to safely develop and test your integrations without affecting a live store.
Creating a Shopify Partner Account
To access Shopify's development features, you first need to create a Shopify Partner account. This account will enable you to create development stores, which are ideal for testing and development purposes.
- Visit the Shopify Partners page and sign up for a free account.
- Fill in the required information and complete the registration process.
- Once registered, log in to your Shopify Partner dashboard.
Setting Up a Shopify Development Store
With your Partner account ready, you can now create a development store. This store will serve as your sandbox environment for testing API interactions.
- In your Shopify Partner dashboard, navigate to the "Stores" section.
- Click on "Add store" and select "Development store" as the store type.
- Fill in the necessary details, such as store name and password, and click "Save."
- Your development store is now ready for use.
Creating a Shopify App for OAuth Authentication
Shopify uses OAuth for authentication, which requires you to create an app to obtain the necessary credentials.
- In your Shopify Partner dashboard, go to the "Apps" section and click "Create app."
- Provide a name for your app and select the development store you created earlier.
- Under "App setup," configure the necessary scopes for product variants, such as
write_products
andread_products
. - Save your app to generate the client ID and client secret, which you'll use for OAuth authentication.
Obtaining Access Tokens for API Requests
With your app created, you can now obtain access tokens to authenticate API requests.
- Direct users to the Shopify authorization URL, including your app's client ID and requested scopes.
- Upon user approval, Shopify will redirect to your app's redirect URI with a temporary code.
- Exchange this code for a permanent access token by making a POST request to Shopify's token endpoint.
import requests
# Exchange temporary code for access token
url = "https://your-development-store.myshopify.com/admin/oauth/access_token"
payload = {
"client_id": "your_client_id",
"client_secret": "your_client_secret",
"code": "temporary_code"
}
response = requests.post(url, data=payload)
access_token = response.json().get("access_token")
print(access_token)
With the access token, you're now ready to make authenticated API calls to Shopify.
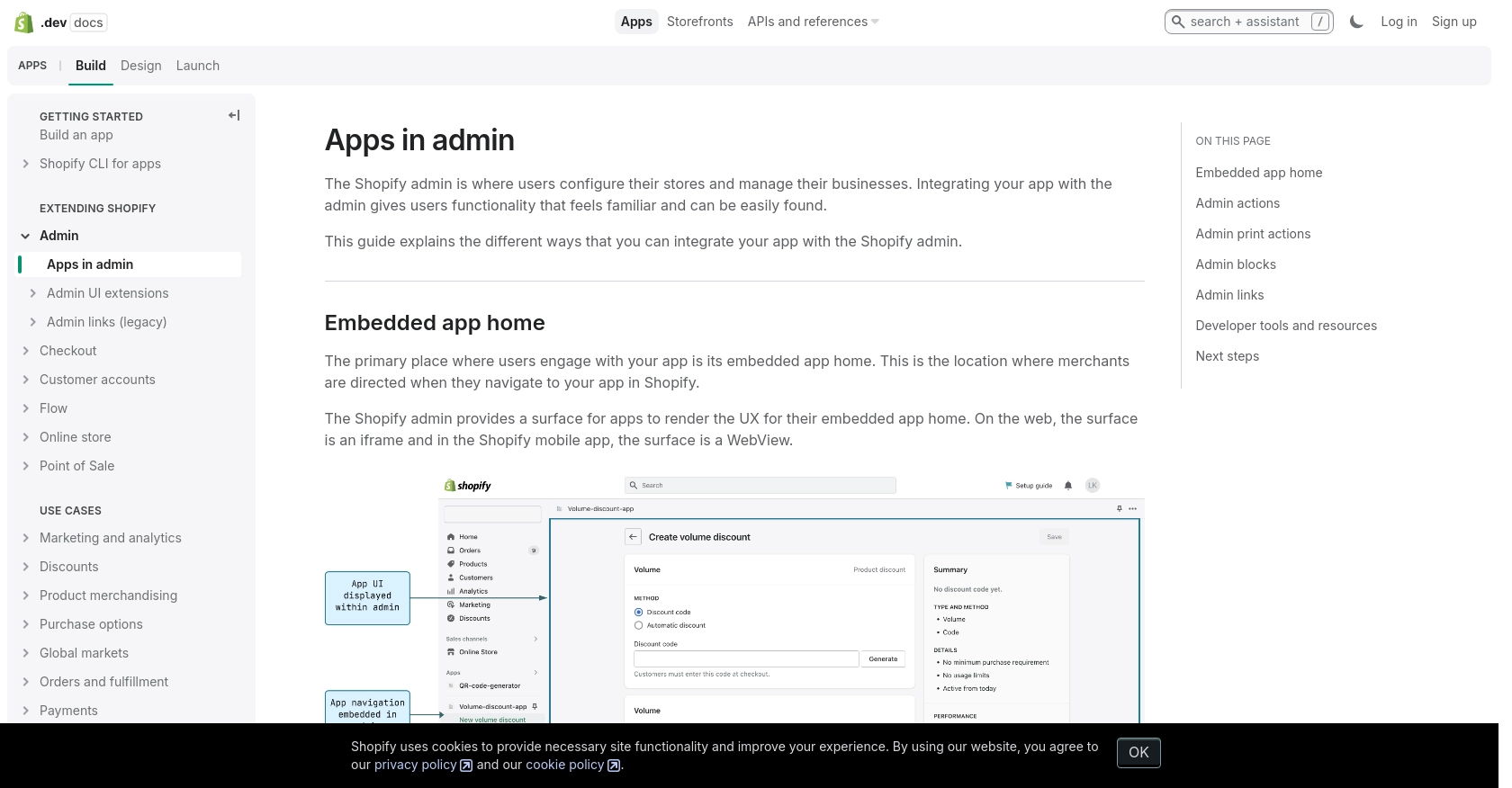
sbb-itb-96038d7
Making API Calls to Shopify for Product Variant Management Using Python
To interact with Shopify's API for creating or updating product variants, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for Shopify API Integration
Before making API calls, ensure you have the correct version of Python and necessary libraries installed. This tutorial uses Python 3.11.1 and the requests
library for HTTP requests.
- Ensure Python 3.11.1 is installed on your machine.
- Install the
requests
library using pip:
pip install requests
Creating a New Product Variant with Shopify API
To create a new product variant, you'll need to make a POST request to Shopify's API. Here's how you can do it using Python:
import requests
# Define the API endpoint and headers
url = "https://your-development-store.myshopify.com/admin/api/2024-07/products/{product_id}/variants.json"
headers = {
"Content-Type": "application/json",
"X-Shopify-Access-Token": "your_access_token"
}
# Define the product variant data
variant_data = {
"variant": {
"option1": "Yellow",
"price": "1.00"
}
}
# Make the POST request
response = requests.post(url, json=variant_data, headers=headers)
# Check the response
if response.status_code == 201:
print("Product Variant Created Successfully")
else:
print("Failed to Create Product Variant:", response.json())
Replace your_access_token
and {product_id}
with your actual access token and product ID. Upon successful creation, you should see a confirmation message.
Updating an Existing Product Variant with Shopify API
To update an existing product variant, use a PUT request. Here's an example:
import requests
# Define the API endpoint and headers
url = "https://your-development-store.myshopify.com/admin/api/2024-07/variants/{variant_id}.json"
headers = {
"Content-Type": "application/json",
"X-Shopify-Access-Token": "your_access_token"
}
# Define the updated variant data
updated_variant_data = {
"variant": {
"id": {variant_id},
"price": "2.00"
}
}
# Make the PUT request
response = requests.put(url, json=updated_variant_data, headers=headers)
# Check the response
if response.status_code == 200:
print("Product Variant Updated Successfully")
else:
print("Failed to Update Product Variant:", response.json())
Replace your_access_token
and {variant_id}
with your actual access token and variant ID. A successful update will return a confirmation message.
Handling Shopify API Responses and Errors
It's crucial to handle API responses and errors effectively. Shopify's API returns various status codes to indicate success or failure:
- 201 Created: The product variant was successfully created.
- 200 OK: The product variant was successfully updated.
- 401 Unauthorized: Incorrect authentication credentials.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
For more details on status codes, refer to the Shopify API documentation.
Verifying API Call Success in Shopify Sandbox
After making API calls, verify the changes in your Shopify development store. Check the product listings to ensure that the variants have been created or updated as expected.
By following these steps, you can efficiently manage product variants using Shopify's API and Python, streamlining your e-commerce operations.
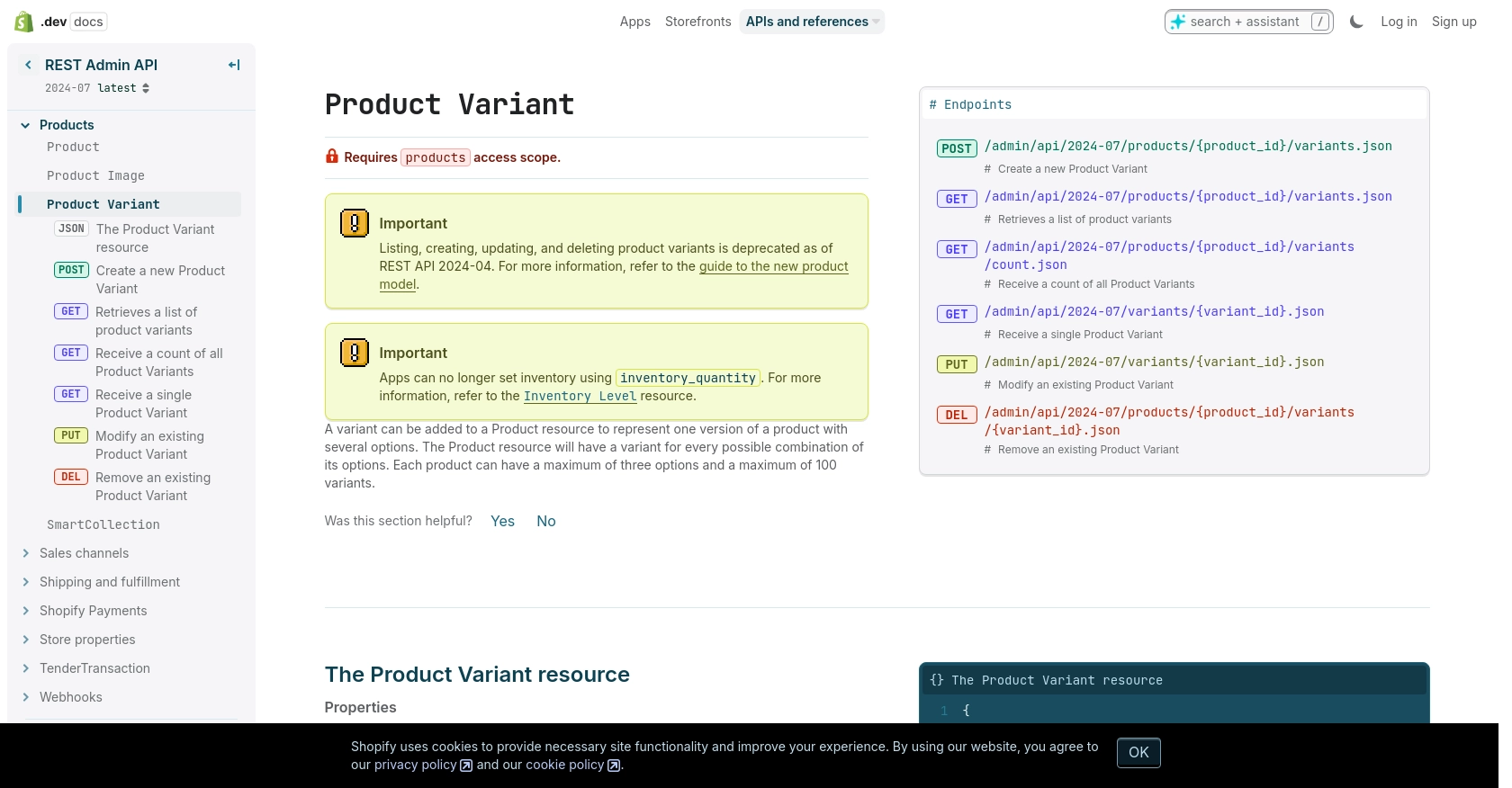
Conclusion and Best Practices for Shopify API Integration
Integrating with the Shopify API to manage product variants can significantly enhance your e-commerce operations by automating inventory management and ensuring accurate product listings. By leveraging Python, developers can efficiently create and update product variants, streamlining workflows and improving store management.
Best Practices for Secure and Efficient Shopify API Usage
- Securely Store Credentials: Always store your API credentials, such as access tokens, securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits: Shopify's API has a rate limit of 40 requests per app per store per minute. Implement retry logic and monitor the
X-Shopify-Shop-Api-Call-Limit
header to manage requests efficiently. For more details, refer to the Shopify API documentation. - Data Standardization: Ensure consistent data formats when creating or updating product variants. This helps maintain data integrity across your store.
- Error Handling: Implement robust error handling to manage API responses effectively. Check for status codes and handle errors such as 401 Unauthorized and 429 Too Many Requests appropriately.
Streamline Your Integrations with Endgrate
While integrating with Shopify's API can be powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API endpoint that simplifies the integration process across various platforms, including Shopify.
By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?