How to Get Deals with the Pipedrive API in PHP
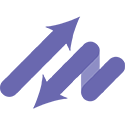
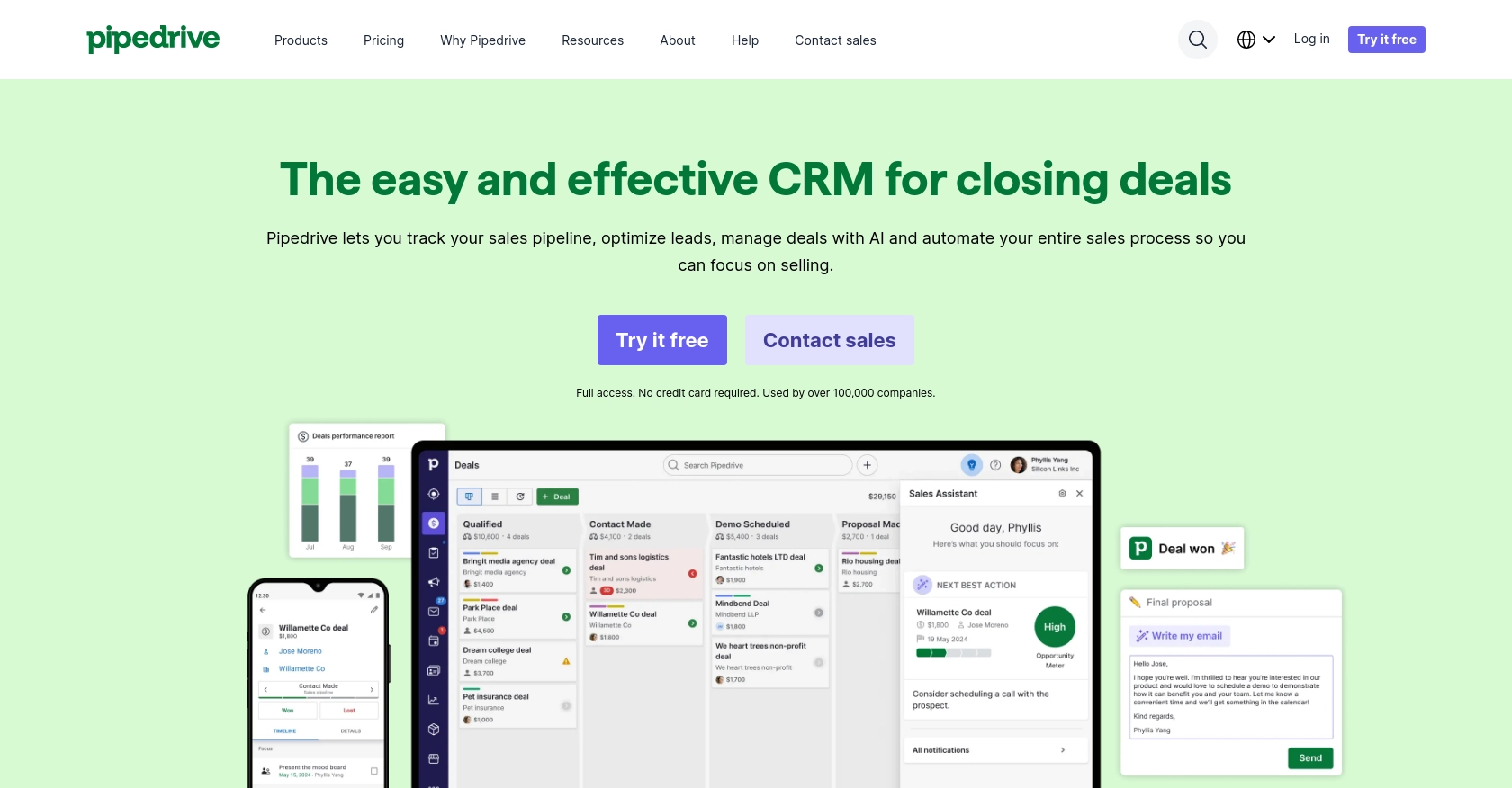
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track deals, manage contacts, and automate workflows, making it a popular choice for businesses looking to enhance their sales operations.
Integrating with Pipedrive's API allows developers to access and manipulate sales data programmatically. For example, you might want to retrieve deal information to generate sales reports or automate follow-up tasks based on deal status. This integration can streamline sales processes and improve data accuracy.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you need to set up a developer sandbox account. This account provides a risk-free environment where you can test and develop your integration without affecting live data.
Creating a Pipedrive Developer Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to a sandbox account. This account will give you access to Pipedrive's Developer Hub, where you can manage your apps and integrations.
- Once your account is set up, you can import sample data to familiarize yourself with the Pipedrive interface. Navigate to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app to import template spreadsheets with sample data.
Creating a Pipedrive App for OAuth Authentication
Pipedrive uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to create your app:
- Log in to your sandbox account and navigate to the Developer Hub.
- Register your app by providing the required details. This will generate a client ID and client secret, which are essential for the OAuth flow.
- Ensure your app has the necessary scopes and permissions for accessing deals. Choose only the scopes that are essential for your integration to maintain security.
With your sandbox account and app set up, you're ready to start making API calls to Pipedrive. In the next section, we'll explore how to retrieve deals using PHP.
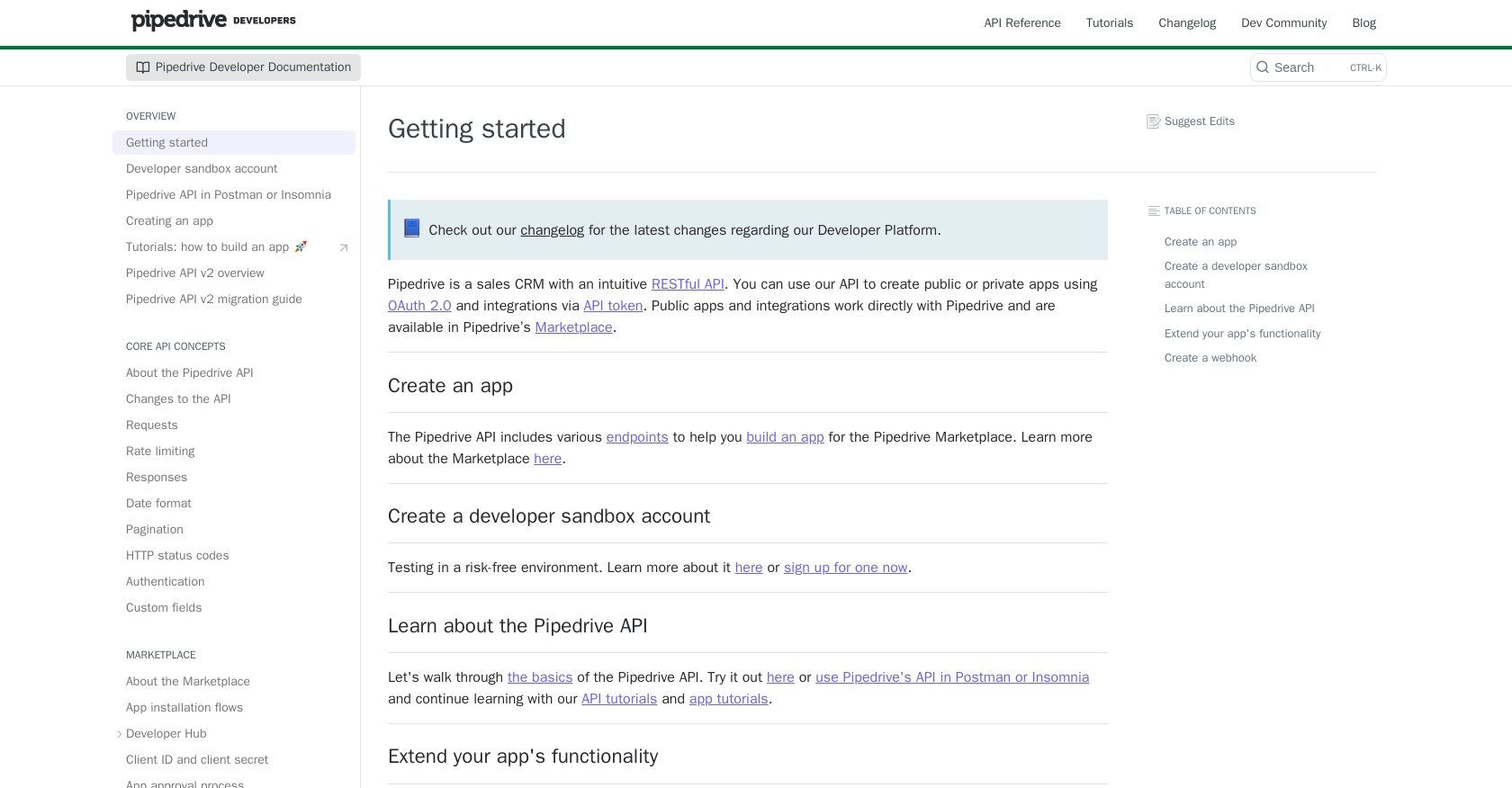
sbb-itb-96038d7
Making API Calls to Retrieve Deals from Pipedrive Using PHP
To interact with the Pipedrive API and retrieve deals, you'll need to use PHP, a popular server-side scripting language. This section will guide you through the process of setting up your PHP environment, making API calls, and handling responses effectively.
Setting Up Your PHP Environment for Pipedrive API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or later installed on your machine.
- Composer, the PHP package manager, to manage dependencies.
Install the necessary dependencies by running the following command in your terminal:
composer require guzzlehttp/guzzle
This command installs Guzzle, a PHP HTTP client that simplifies making HTTP requests.
Writing PHP Code to Retrieve Deals from Pipedrive
Now that your environment is set up, you can write the PHP code to interact with the Pipedrive API. Create a file named get_pipedrive_deals.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle client
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://api.pipedrive.com/v1/deals';
$accessToken = 'Your_Access_Token';
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
];
try {
// Make a GET request to the API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Parse the JSON data from the response
$data = json_decode($response->getBody(), true);
// Loop through the deals and print their information
foreach ($data['data'] as $deal) {
echo 'Deal ID: ' . $deal['id'] . ' - Title: ' . $deal['title'] . PHP_EOL;
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the access token obtained during the OAuth authentication process.
Understanding the API Response and Handling Errors
After running the script, you should see a list of deals printed in your terminal. This confirms that the API call was successful. If you encounter any errors, the script will catch exceptions and display an error message.
Common HTTP status codes you might encounter include:
- 200 OK: The request was successful.
- 401 Unauthorized: Invalid or missing access token.
- 429 Too Many Requests: Rate limit exceeded. Refer to the rate limiting documentation for more details.
For more information on error codes, visit the Pipedrive HTTP status codes documentation.
Verifying API Call Success in Pipedrive Sandbox
To verify that the API call retrieved the correct data, log in to your Pipedrive sandbox account and check the deals section. The deals listed in your PHP script output should match those in the sandbox environment.
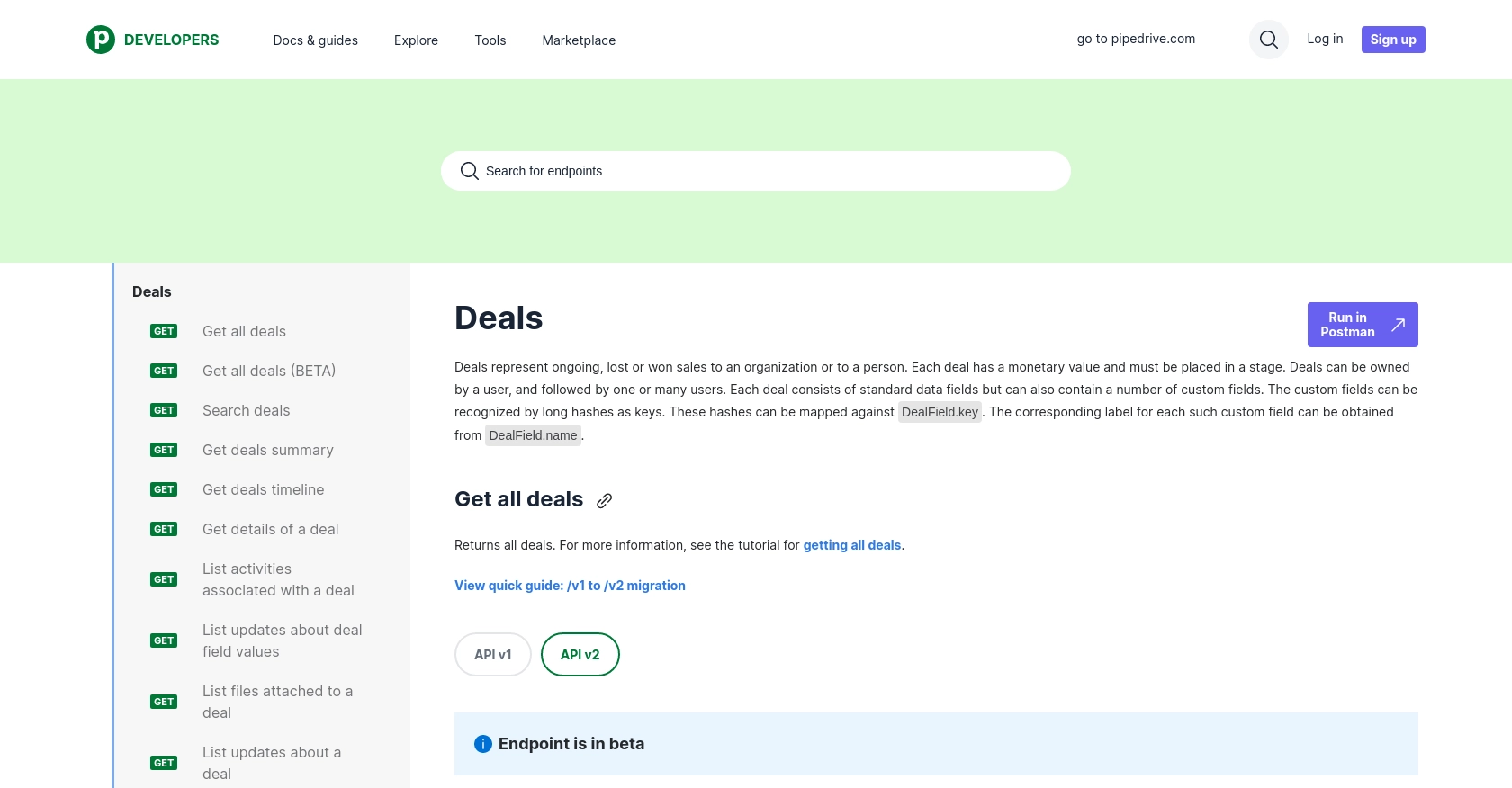
Best Practices for Using Pipedrive API in PHP
When working with the Pipedrive API, it's crucial to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Pipedrive's API has rate limits based on your plan. For OAuth apps, the limit is up to 480 requests per 2 seconds per access token for Enterprise plans. Implement logic to handle HTTP 429 errors gracefully by retrying requests after a delay. Refer to the rate limiting documentation for more details.
- Use Pagination: When retrieving large datasets, use pagination to manage data efficiently. Pipedrive supports cursor-based pagination for deal endpoints. This ensures you don't overwhelm your application with too much data at once.
- Standardize Data Fields: When dealing with custom fields, ensure consistent naming conventions and data formats. This will help maintain data integrity across different integrations.
Leveraging Endgrate for Seamless Pipedrive Integrations
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Pipedrive. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of integration.
- Build Once, Use Everywhere: Develop a single integration for multiple platforms, reducing redundancy and maintenance efforts.
- Enhance User Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Conclusion on Integrating Pipedrive API with PHP
Integrating with the Pipedrive API using PHP allows developers to automate and enhance sales processes efficiently. By following the steps outlined in this guide, you can successfully retrieve and manage deals, improving your sales operations. Remember to adhere to best practices for security and performance, and consider leveraging tools like Endgrate to simplify your integration efforts.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Deals
- https://developers.pipedrive.com/docs/api/v1/DealFields
Ready to get started?