Using the Sage Accounting API to Create or Update Vendors (with Python examples)
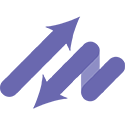
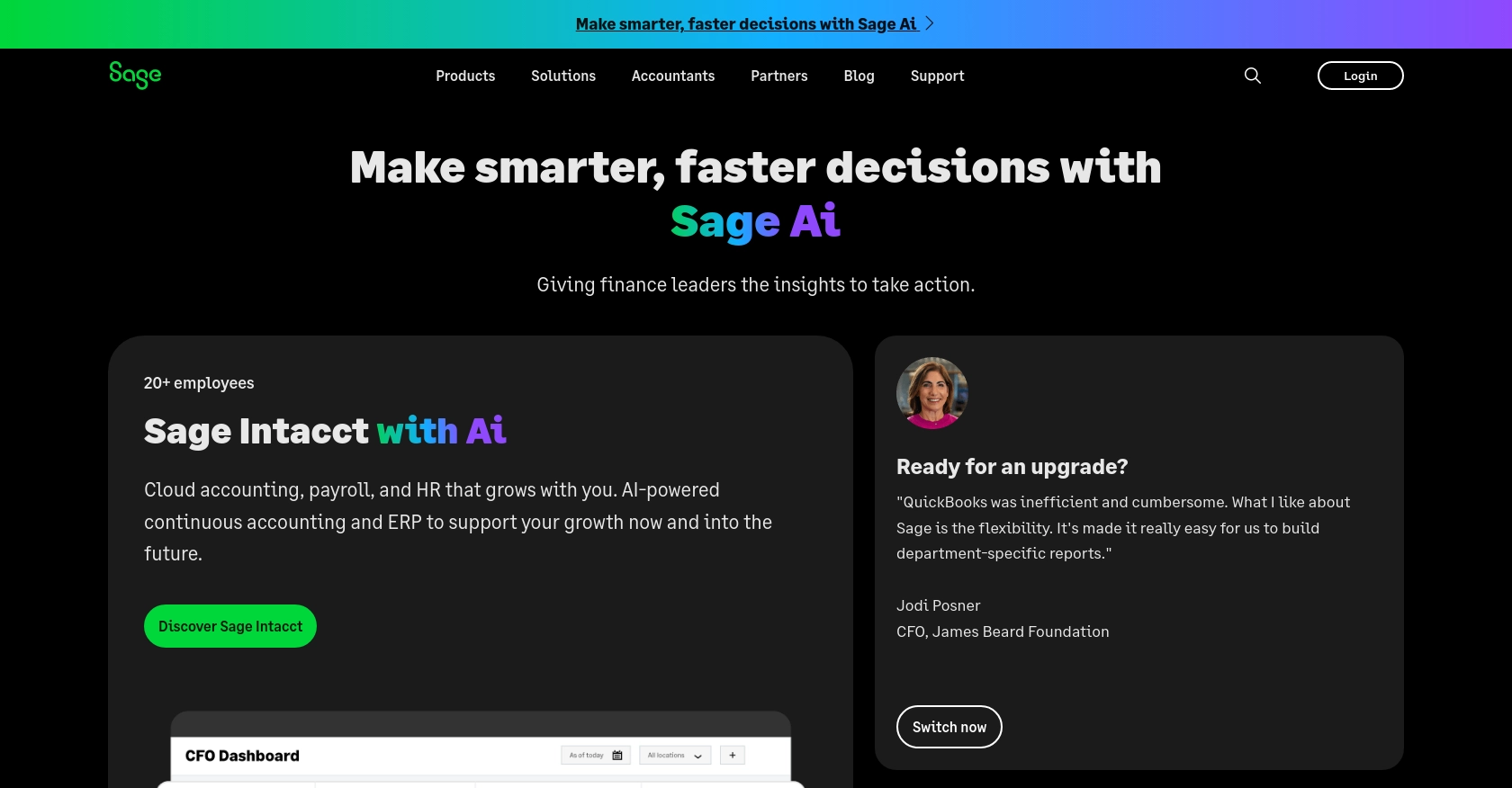
Introduction to Sage Accounting API for Vendor Management
Sage Accounting is a comprehensive cloud-based accounting solution tailored for small to medium-sized businesses. It offers a suite of tools to manage finances, including invoicing, cash flow, and tax management. With its robust API, Sage Accounting allows developers to integrate seamlessly with its platform, enhancing business operations and efficiency.
Integrating with the Sage Accounting API can empower developers to automate vendor management tasks, such as creating or updating vendor information. For example, a developer might want to automate the process of adding new vendors from a procurement system into Sage Accounting, ensuring that vendor records are always up-to-date and accurate.
This article will guide you through using Python to interact with the Sage Accounting API, focusing on creating or updating vendor details. By the end of this tutorial, you'll be equipped to streamline vendor management processes within your application.
Setting Up Your Sage Accounting Test/Sandbox Account for API Integration
Before you begin integrating with the Sage Accounting API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Sage Developer Account
To access the Sage Accounting API, you need a Sage Developer account. This account will enable you to register and manage applications, obtain client credentials, and specify essential details such as callback URLs.
- Visit the Sage Developer Portal.
- Sign up using your GitHub account or an email address.
- Follow the guide on creating an app to complete your registration.
Set Up a Trial Business for Development
Sage allows you to create trial businesses for development purposes. This setup is crucial for testing your integration without any risk to actual business data.
- Choose the appropriate subscription tier for your needs: Accounting Start, Accounting Standard, or Accounting Plus.
- Use email services that support aliasing to manage multiple environments efficiently.
- Sign up for a trial account in your region by visiting the relevant links provided on the Sage Developer Portal.
Register Your Application and Obtain OAuth Credentials
Once your developer account is set up, the next step is to register your application to obtain OAuth credentials, which are necessary for authenticating API requests.
- Log in to your Sage Developer account.
- Navigate to the "Create App" section.
- Enter a name and callback URL for your app. Optionally, provide an alternative email address and homepage URL.
- Click "Save" to generate your Client ID and Client Secret.
These credentials will be used to authenticate your API requests, ensuring secure access to the Sage Accounting platform.
Upgrade to a Developer Account
To fully utilize the Sage Accounting API, upgrade your trial account to a developer account. This upgrade provides 12 months of free access for testing your integration.
- Submit the necessary details, including your name, email address, app name, and Client ID, through the upgrade form on the Sage Developer Portal.
- Wait for confirmation from the Sage team, which typically takes 3-5 working days.
With your test environment and credentials ready, you can now proceed to interact with the Sage Accounting API using Python, as detailed in the following sections.
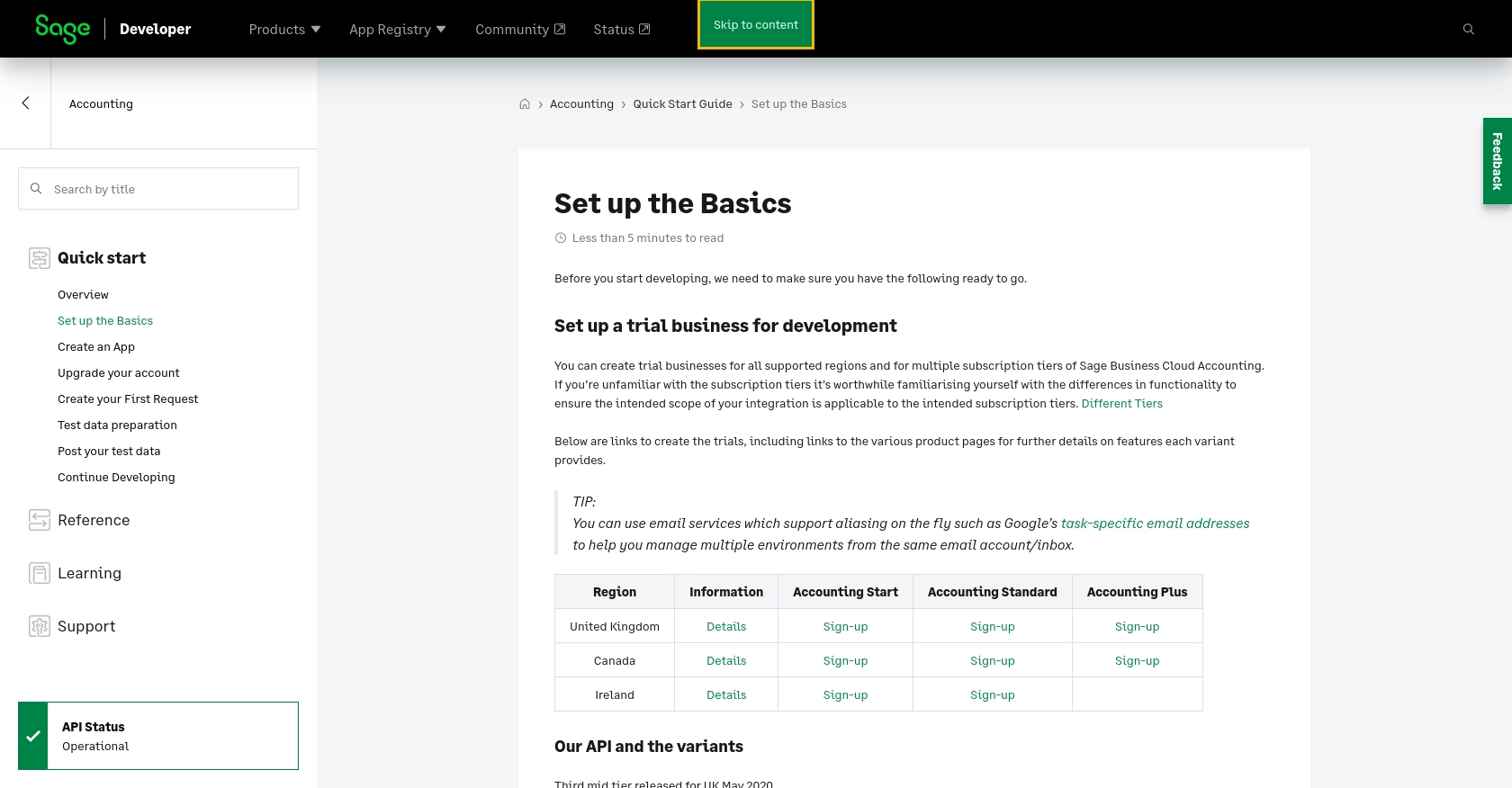
sbb-itb-96038d7
Making API Calls to Sage Accounting for Vendor Management Using Python
To interact with the Sage Accounting API for creating or updating vendors, you'll need to set up your Python environment and make authenticated API requests. This section will guide you through the necessary steps, including setting up your Python environment, writing the code to make API calls, and handling responses.
Setting Up Your Python Environment for Sage Accounting API Integration
Before making API calls, ensure your Python environment is properly configured. You'll need Python 3.11.1 and the requests
library to handle HTTP requests.
- Ensure Python 3.11.1 is installed on your machine.
- Install the
requests
library using pip:
pip install requests
Creating or Updating Vendors with Sage Accounting API
Once your environment is set up, you can proceed to create or update vendor information using the Sage Accounting API. Below is a Python example demonstrating how to perform these actions.
Example Code to Create a Vendor
import requests
# Define the API endpoint and headers
url = "https://api.accounting.sage.com/v3.1/contacts"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the vendor data
vendor_data = {
"contact": {
"name": "New Vendor",
"contact_type_ids": ["supplier_id"],
"reference": "Vendor123",
"main_address": {
"address_line_1": "123 Vendor St",
"city": "Vendor City",
"postal_code": "12345",
"country_id": "country_id"
}
}
}
# Make the POST request to create a vendor
response = requests.post(url, json=vendor_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Vendor created successfully.")
else:
print("Failed to create vendor:", response.json())
Replace Your_Access_Token
with your actual OAuth token. The supplier_id
and country_id
should be replaced with valid IDs from your Sage Accounting setup.
Example Code to Update a Vendor
import requests
# Define the API endpoint and headers
vendor_id = "existing_vendor_id"
url = f"https://api.accounting.sage.com/v3.1/contacts/{vendor_id}"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the updated vendor data
updated_vendor_data = {
"contact": {
"name": "Updated Vendor Name",
"reference": "UpdatedVendor123"
}
}
# Make the PUT request to update the vendor
response = requests.put(url, json=updated_vendor_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Vendor updated successfully.")
else:
print("Failed to update vendor:", response.json())
Ensure you replace existing_vendor_id
with the ID of the vendor you wish to update.
Verifying API Request Success and Handling Errors
After making API calls, it's crucial to verify the success of your requests. Check the response status codes to determine if the operation was successful:
- 201 Created: Indicates successful creation of a vendor.
- 200 OK: Indicates successful update of a vendor.
- For error handling, inspect the response JSON for error messages and codes.
Refer to the Sage Accounting API documentation for detailed information on error codes and handling strategies.
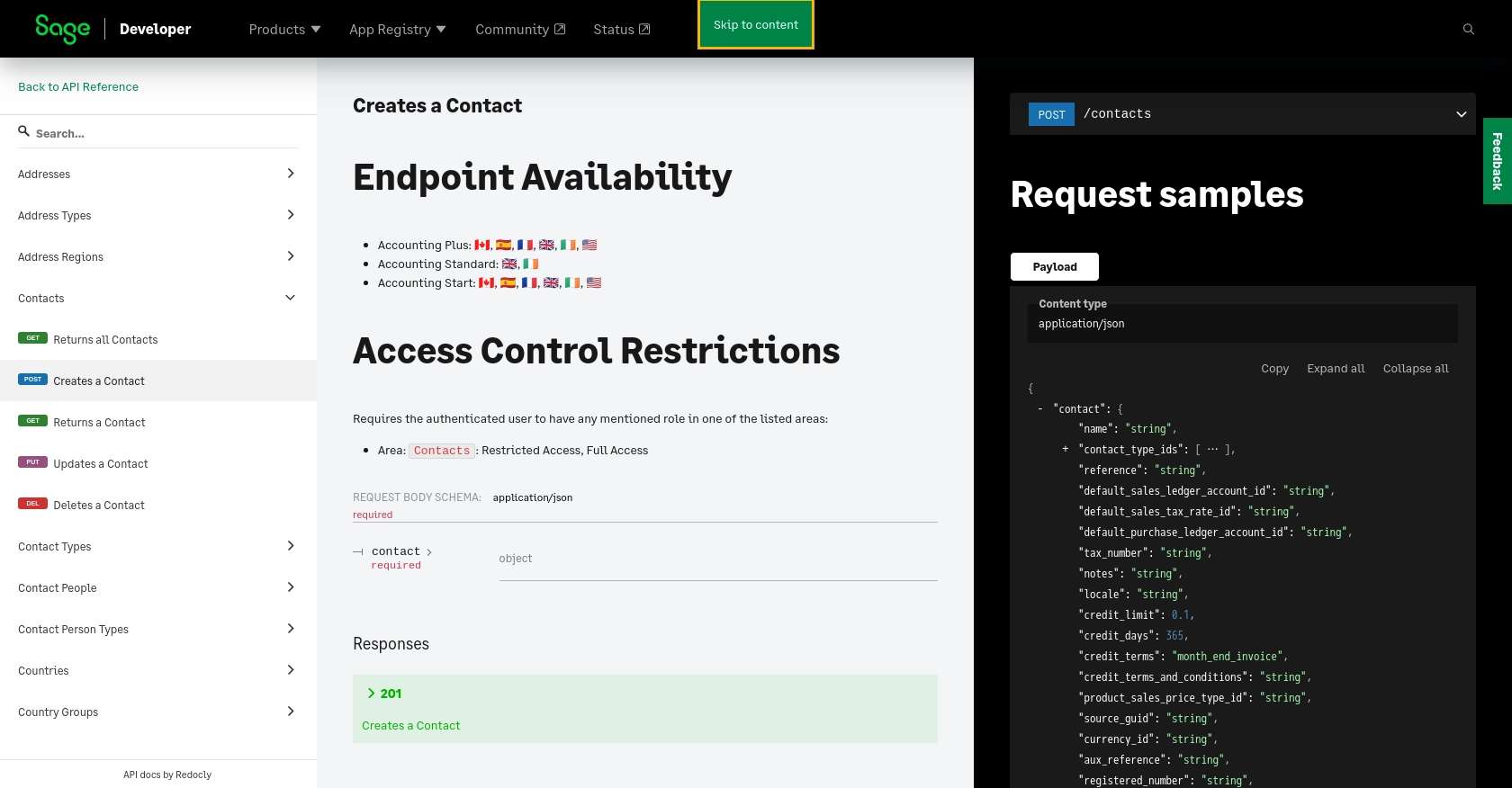
Conclusion and Best Practices for Using Sage Accounting API for Vendor Management
Integrating with the Sage Accounting API to manage vendors can significantly streamline your business operations. By automating tasks such as creating and updating vendor information, you can ensure data consistency and reduce manual errors.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be mindful of API rate limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized across your systems to maintain consistency and accuracy in vendor records.
- Error Handling: Implement robust error handling by checking response status codes and parsing error messages. Refer to the Sage Accounting API documentation for detailed error code information.
Streamline Your Integration Process with Endgrate
For developers looking to simplify the integration process, consider using Endgrate. By leveraging Endgrate's unified API, you can connect to multiple platforms, including Sage Accounting, with ease. This allows you to focus on your core product while outsourcing complex integrations.
Visit Endgrate to learn more about how you can save time and resources by building once for each use case instead of multiple times for different integrations.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/contacts/#tag/Contacts/operation/postContacts
Ready to get started?