How to Create or Update Records with the MongoDB API in PHP
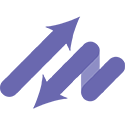
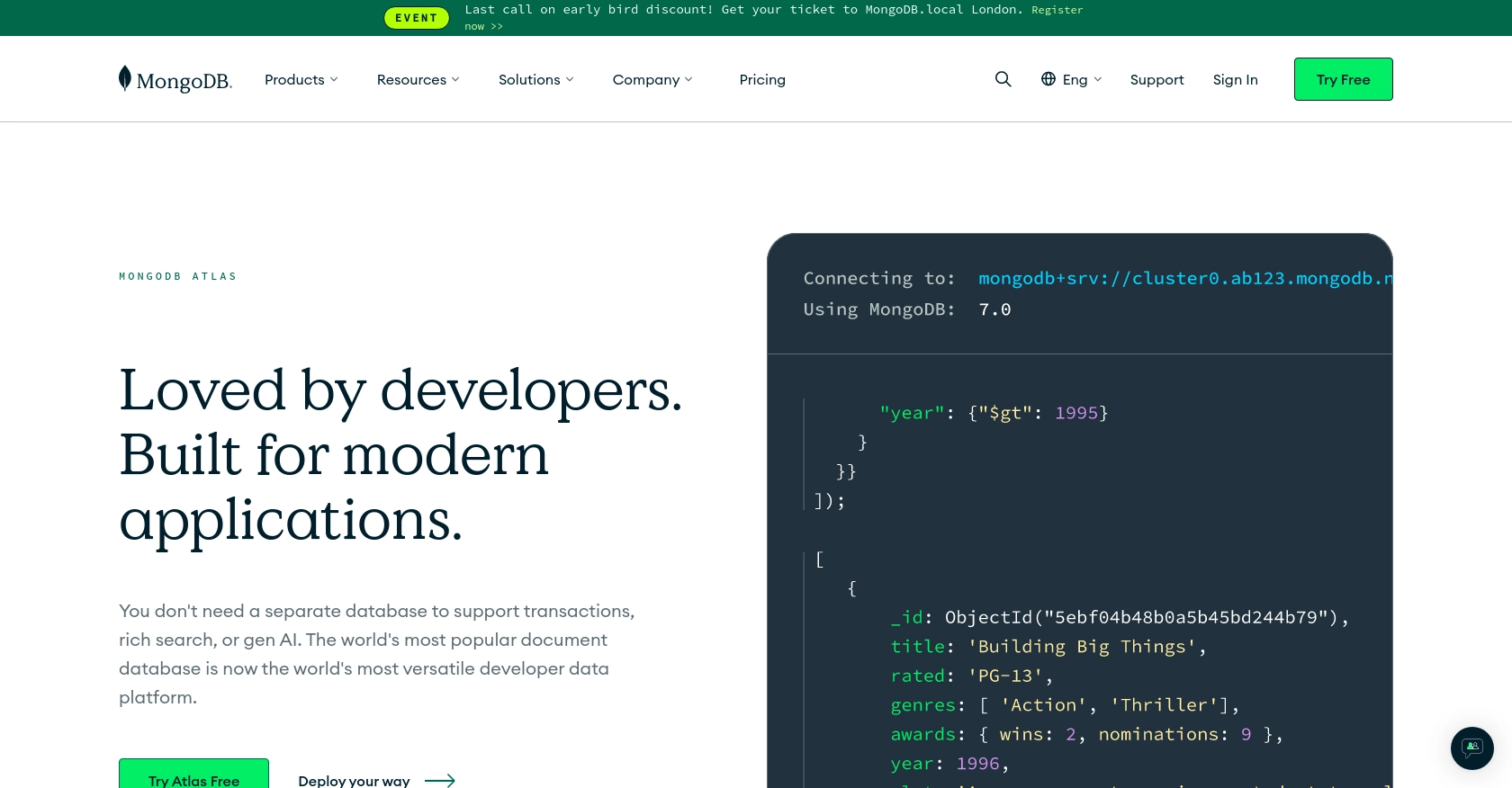
Introduction to MongoDB and Its API
MongoDB is a powerful, open-source, document-oriented database designed for scalability and flexibility. It is widely used by developers to build modern applications that require high performance and availability. MongoDB's document model allows for easy storage and retrieval of complex data structures, making it a popular choice for applications that handle large volumes of data.
Integrating with MongoDB's API enables developers to efficiently manage and manipulate data within their applications. For example, you might want to create or update records in a MongoDB database using PHP to keep your application data synchronized with user interactions or external data sources.
This article will guide you through the process of using PHP to create or update records in MongoDB, providing you with the necessary steps and code examples to get started.
Setting Up Your MongoDB Test or Sandbox Account
Before you can start creating or updating records with the MongoDB API in PHP, you need to set up a MongoDB test or sandbox account. This will allow you to safely experiment with the API without affecting any production data.
Creating a MongoDB Atlas Account
MongoDB Atlas is a cloud-based database service that provides a free tier for developers to get started. Follow these steps to create your MongoDB Atlas account:
- Visit the MongoDB Atlas website and click on the "Try Free" button.
- Sign up using your email address or an existing Google account.
- Once registered, log in to your MongoDB Atlas account.
Setting Up a Free Tier Cluster
After creating your MongoDB Atlas account, you need to set up a free tier cluster to host your database:
- In the MongoDB Atlas dashboard, click on "Build a Cluster."
- Select the free tier option and choose your preferred cloud provider and region.
- Follow the prompts to configure your cluster and click "Create Cluster."
Generating MongoDB API Credentials
To interact with your MongoDB database programmatically, you need to generate API credentials:
- Navigate to the "Database Access" section in the MongoDB Atlas dashboard.
- Click on "Add New Database User" and create a user with the necessary permissions.
- Save the username and password, as you'll need these credentials to authenticate your API requests.
Configuring Network Access
Ensure that your application can connect to your MongoDB cluster by configuring network access:
- Go to the "Network Access" section in the MongoDB Atlas dashboard.
- Click on "Add IP Address" and enter your application's IP address or allow access from anywhere for testing purposes.
- Save the changes to update your network access settings.
With your MongoDB Atlas account and cluster set up, and API credentials generated, you're ready to start integrating with the MongoDB API using PHP.
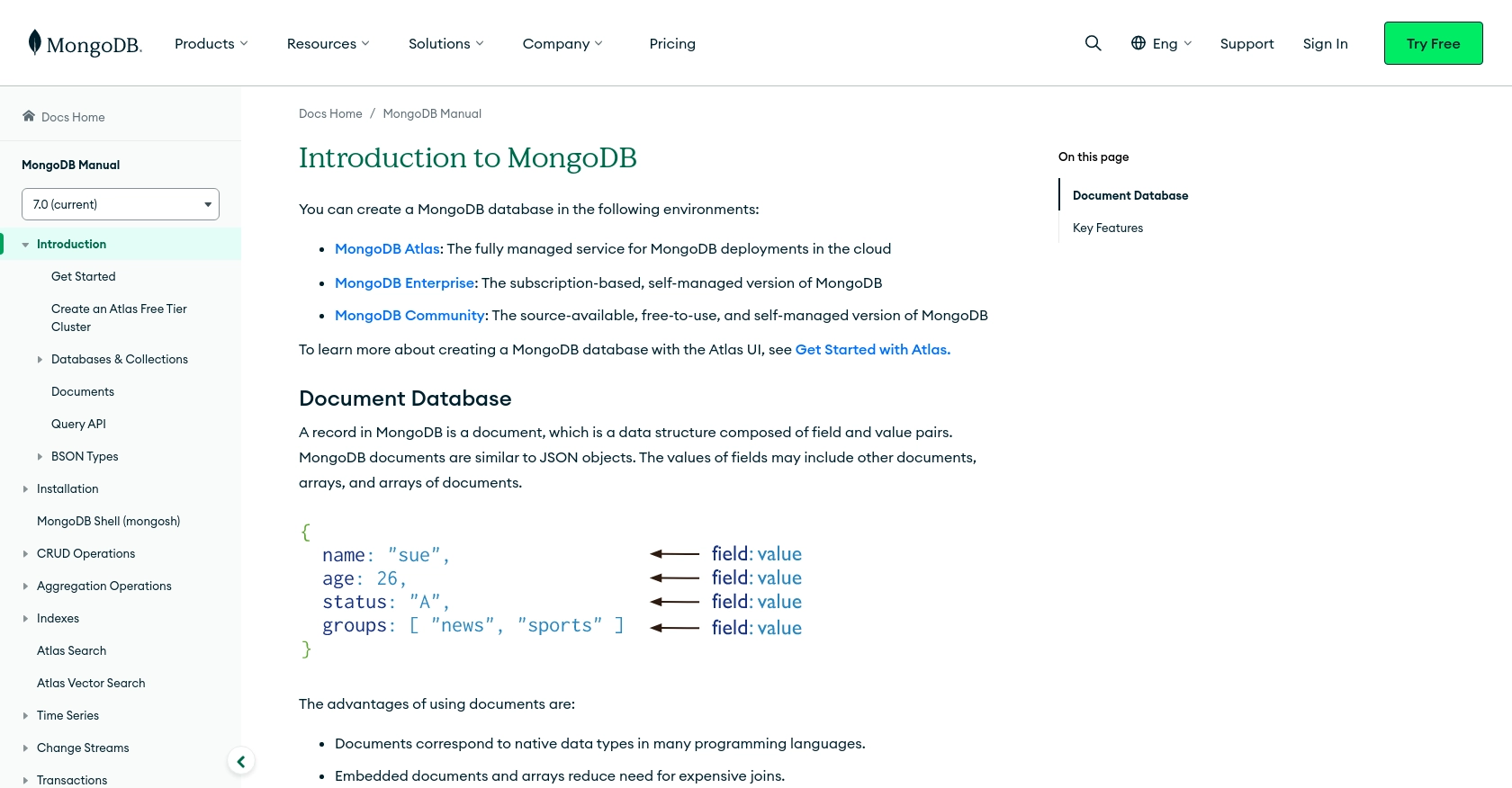
sbb-itb-96038d7
Making API Calls to Create or Update Records in MongoDB Using PHP
To interact with MongoDB using PHP, you'll need to set up your environment with the necessary tools and libraries. This section will guide you through the process of making API calls to create or update records in MongoDB.
Setting Up Your PHP Environment for MongoDB Integration
Before you start coding, ensure that your PHP environment is properly configured to interact with MongoDB:
- Ensure you have PHP 7.4 or later installed on your system.
- Install Composer, the PHP package manager, if you haven't already.
- Use Composer to install the MongoDB PHP library by running the following command in your terminal:
composer require mongodb/mongodb
Connecting to Your MongoDB Database
Once your environment is set up, you can connect to your MongoDB database using the MongoDB PHP library. Here's how you can establish a connection:
require 'vendor/autoload.php';
$client = new MongoDB\Client("mongodb+srv://<username>:<password>@cluster0.mongodb.net/test?retryWrites=true&w=majority");
$collection = $client->databaseName->collectionName;
Replace <username>
and <password>
with your MongoDB Atlas credentials, and databaseName
and collectionName
with your specific database and collection names.
Creating a Record in MongoDB Using PHP
To create a new record in your MongoDB collection, use the following code:
$document = [
'name' => 'John Doe',
'email' => 'john.doe@example.com',
'age' => 29
];
$result = $collection->insertOne($document);
echo "Inserted with Object ID '{$result->getInsertedId()}'";
This code snippet creates a new document with fields for name, email, and age, and inserts it into the specified collection.
Updating a Record in MongoDB Using PHP
To update an existing record, you can use the following code:
$filter = ['email' => 'john.doe@example.com'];
$update = ['$set' => ['age' => 30]];
$updateResult = $collection->updateOne($filter, $update);
echo "Matched {$updateResult->getMatchedCount()} document(s) and modified {$updateResult->getModifiedCount()} document(s)";
This example updates the age of the document where the email is 'john.doe@example.com'.
Verifying API Call Success and Handling Errors
After making API calls, it's crucial to verify their success and handle any potential errors:
- Check the returned result object to confirm the operation's success.
- Use try-catch blocks to handle exceptions and errors gracefully.
try {
// Your MongoDB operations here
} catch (Exception $e) {
echo 'Caught exception: ', $e->getMessage(), "\n";
}
By following these steps, you can effectively create and update records in MongoDB using PHP, ensuring your application data remains consistent and up-to-date.
Conclusion and Best Practices for MongoDB API Integration in PHP
Integrating with the MongoDB API using PHP allows developers to efficiently manage and manipulate data within their applications. By following the steps outlined in this guide, you can create and update records in your MongoDB database, ensuring your application data remains synchronized and up-to-date.
Best Practices for MongoDB API Integration
- Securely Store Credentials: Always store your MongoDB credentials securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by MongoDB and implement retry logic to handle rate-limited responses gracefully.
- Data Validation: Validate and sanitize data before inserting or updating records to ensure data integrity and prevent injection attacks.
- Error Handling: Implement robust error handling using try-catch blocks to manage exceptions and provide informative error messages.
- Optimize Queries: Use indexes and optimize queries to improve performance and reduce latency when accessing MongoDB data.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify the process by leveraging a unified API endpoint that connects to multiple platforms, including MongoDB. This allows you to focus on your core product while outsourcing integration complexities.
Explore how Endgrate can enhance your integration experience by visiting Endgrate. Save time and resources by building once for each use case and providing an intuitive integration experience for your customers.
Read More
Ready to get started?