Using the Stamped API to Create Customers in Python
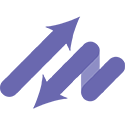
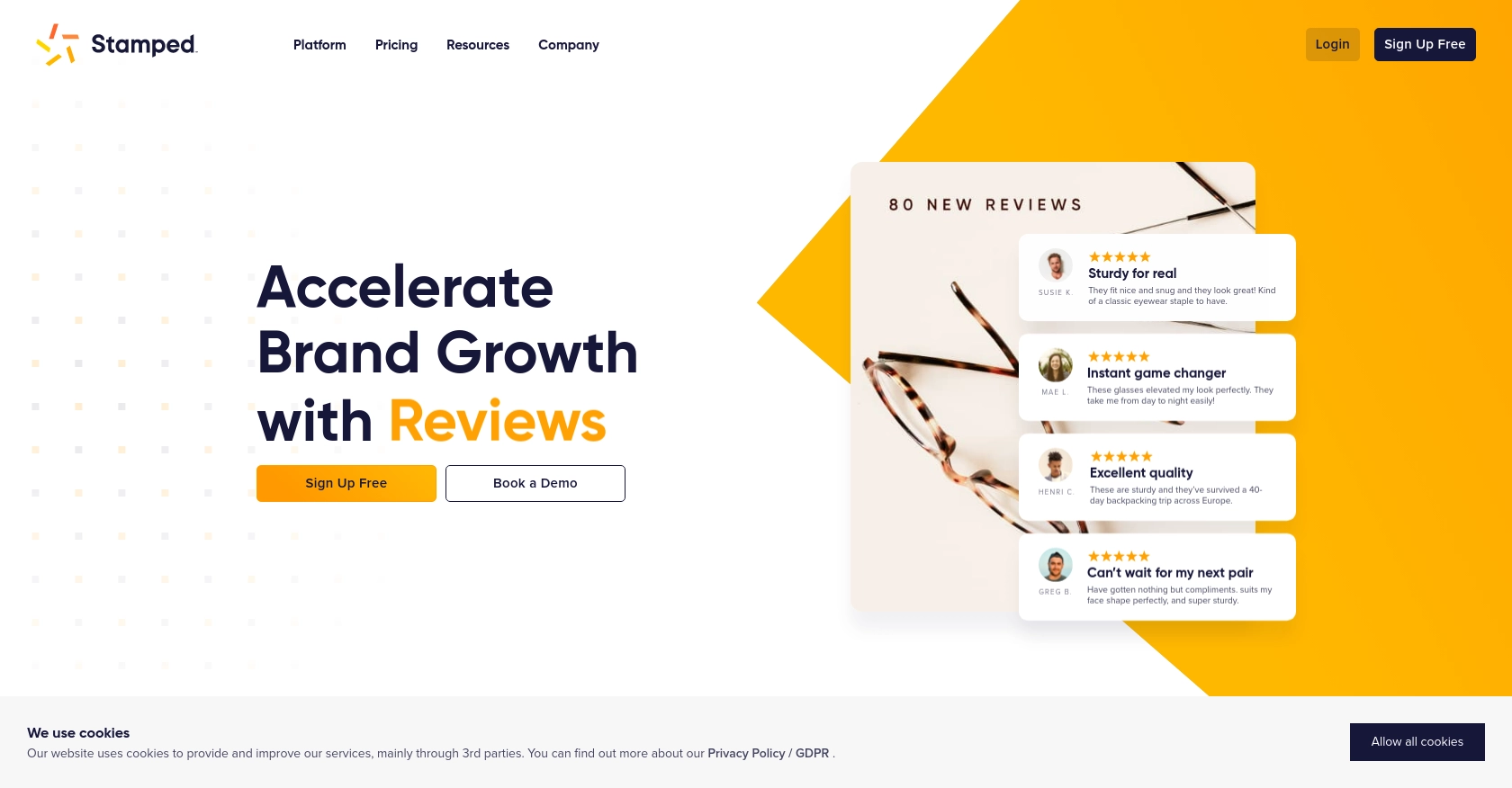
Introduction to Stamped API for Customer Management
Stamped is a powerful platform that empowers over 75,000 brands to enhance customer engagement through reviews, loyalty programs, and more. By offering a comprehensive suite of tools, Stamped helps businesses drive faster and more efficient growth.
For developers, integrating with the Stamped API provides the ability to manage customer data seamlessly. This can be particularly useful for automating customer interactions and enhancing user experience. For example, you might want to create new customer profiles in Stamped directly from your application, streamlining the process and ensuring data consistency across platforms.
Setting Up Your Stamped Test Account for API Integration
Before you can start creating customers using the Stamped API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Stamped requires a Professional or Enterprise plan to access the API, so ensure you have the appropriate account level.
Creating a Stamped Account
If you don't already have a Stamped account, you can sign up on the Stamped website. Follow the instructions to create your account and select the Professional or Enterprise plan to gain API access.
Generating API Keys for Stamped Integration
Once your account is set up, you'll need to generate API keys to authenticate your requests. Follow these steps to obtain your public and private API keys:
- Log in to your Stamped account and navigate to the API Keys section in the Control Panel.
- Here, you will find your public and private API keys. These keys are essential for authenticating your API requests.
- Copy both keys and store them securely, as you will need them for making API calls.
Understanding Stamped API Authentication
The Stamped API uses HTTP Basic Auth for authentication. When making requests to private endpoints, you will use your public API key as the username and your private API key as the password. For public endpoints, only the store hash or public key is required.
For more detailed information on authentication, you can refer to the Stamped REST API documentation.
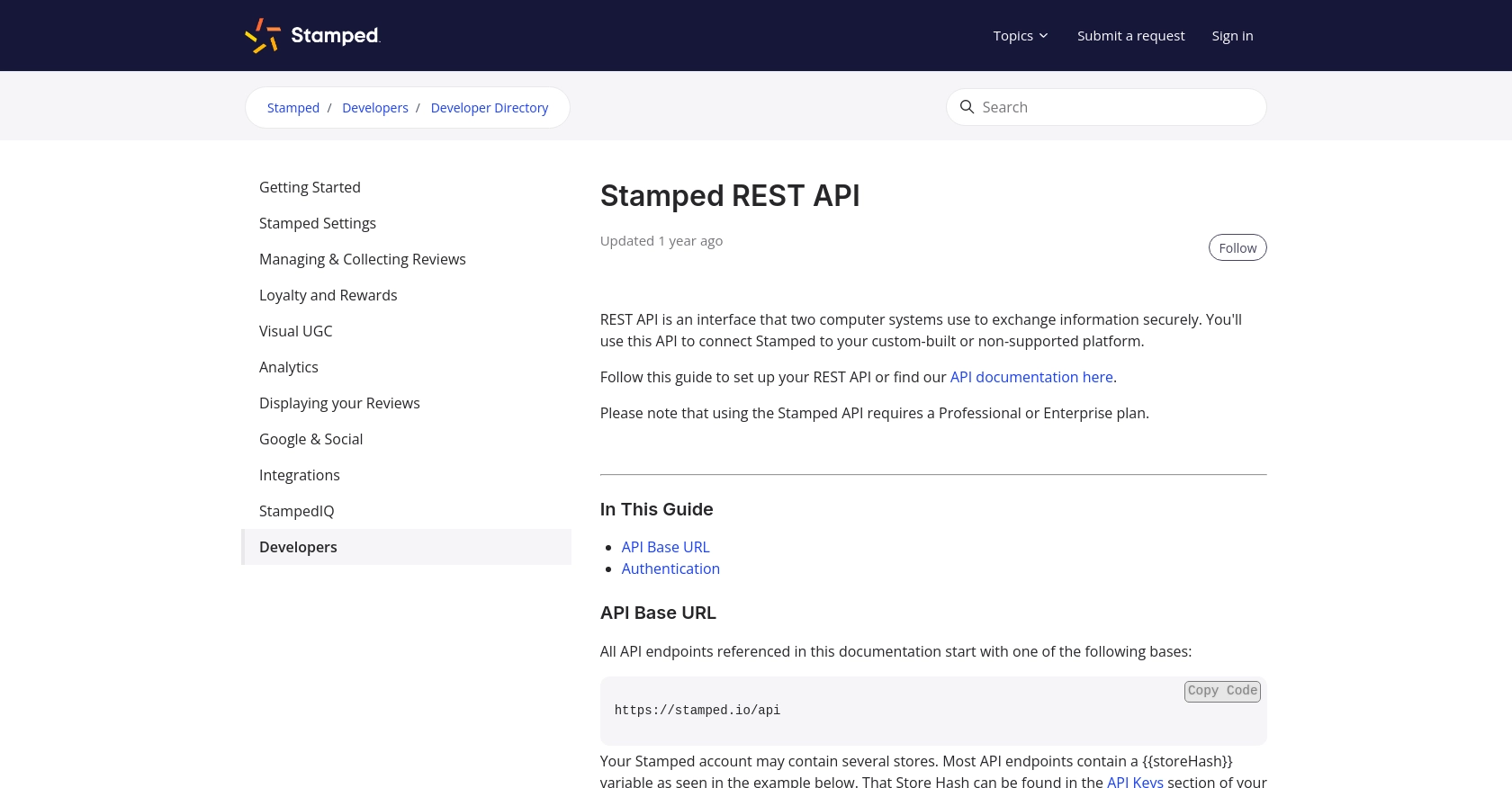
sbb-itb-96038d7
Making API Calls to Create Customers with Stamped API in Python
To create customers using the Stamped API, you'll need to make HTTP requests from your Python application. This section will guide you through the process of setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Stamped API Integration
Before you start coding, ensure you have Python 3.x installed on your machine. You will also need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Writing Python Code to Create Customers in Stamped
Once your environment is ready, you can proceed to write the Python script to create customers in Stamped. Here's a step-by-step guide:
import requests
# Define the API endpoint and your store ID
store_id = "your_store_id"
url = f"https://stamped.io/api/v3/merchant/shops/{store_id}/customers"
# Set up your API keys
public_api_key = "your_public_api_key"
private_api_key = "your_private_api_key"
# Define the customer data
customer_data = {
"email": "customer@example.com",
"firstName": "John",
"lastName": "Doe"
}
# Make the POST request to create a customer
response = requests.post(
url,
json=customer_data,
auth=(public_api_key, private_api_key)
)
# Check the response status
if response.status_code == 201:
print("Customer created successfully.")
else:
print(f"Failed to create customer: {response.status_code} - {response.text}")
Replace your_store_id
, your_public_api_key
, and your_private_api_key
with your actual Stamped store ID and API keys.
Verifying Successful API Requests in Stamped
After running your script, you should verify that the customer has been created in your Stamped account. Log in to your Stamped dashboard and navigate to the Customers section to see the newly created customer.
Handling Errors and Troubleshooting Stamped API Requests
When making API calls, it's essential to handle potential errors gracefully. The Stamped API may return various status codes indicating different issues:
- 400 Bad Request: The request was invalid. Check your data format and required fields.
- 401 Unauthorized: Authentication failed. Verify your API keys.
- 404 Not Found: The endpoint or resource was not found. Check the URL and store ID.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error handling, refer to the Stamped API documentation.
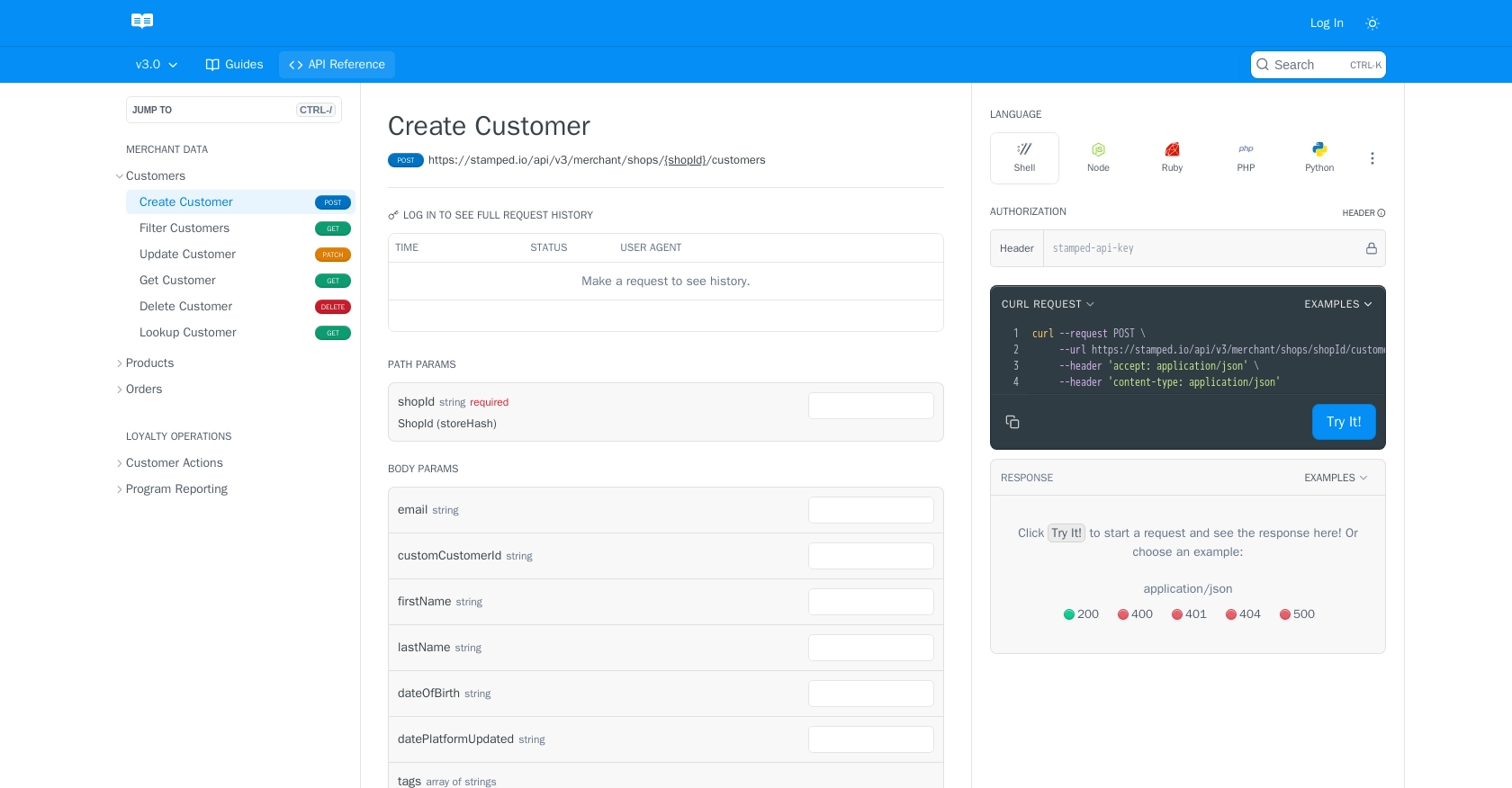
Best Practices for Using Stamped API in Python
When integrating with the Stamped API, it's crucial to follow best practices to ensure security, efficiency, and reliability. Here are some recommendations:
- Securely Store API Credentials: Always store your API keys securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Stamped API. Implement exponential backoff or retry logic to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that the data you send to Stamped is consistent and follows any required formats. This will help prevent errors and maintain data integrity.
Streamlining Integration with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Stamped. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?