How to Get Profiles with the Klaviyo API in Javascript
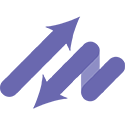
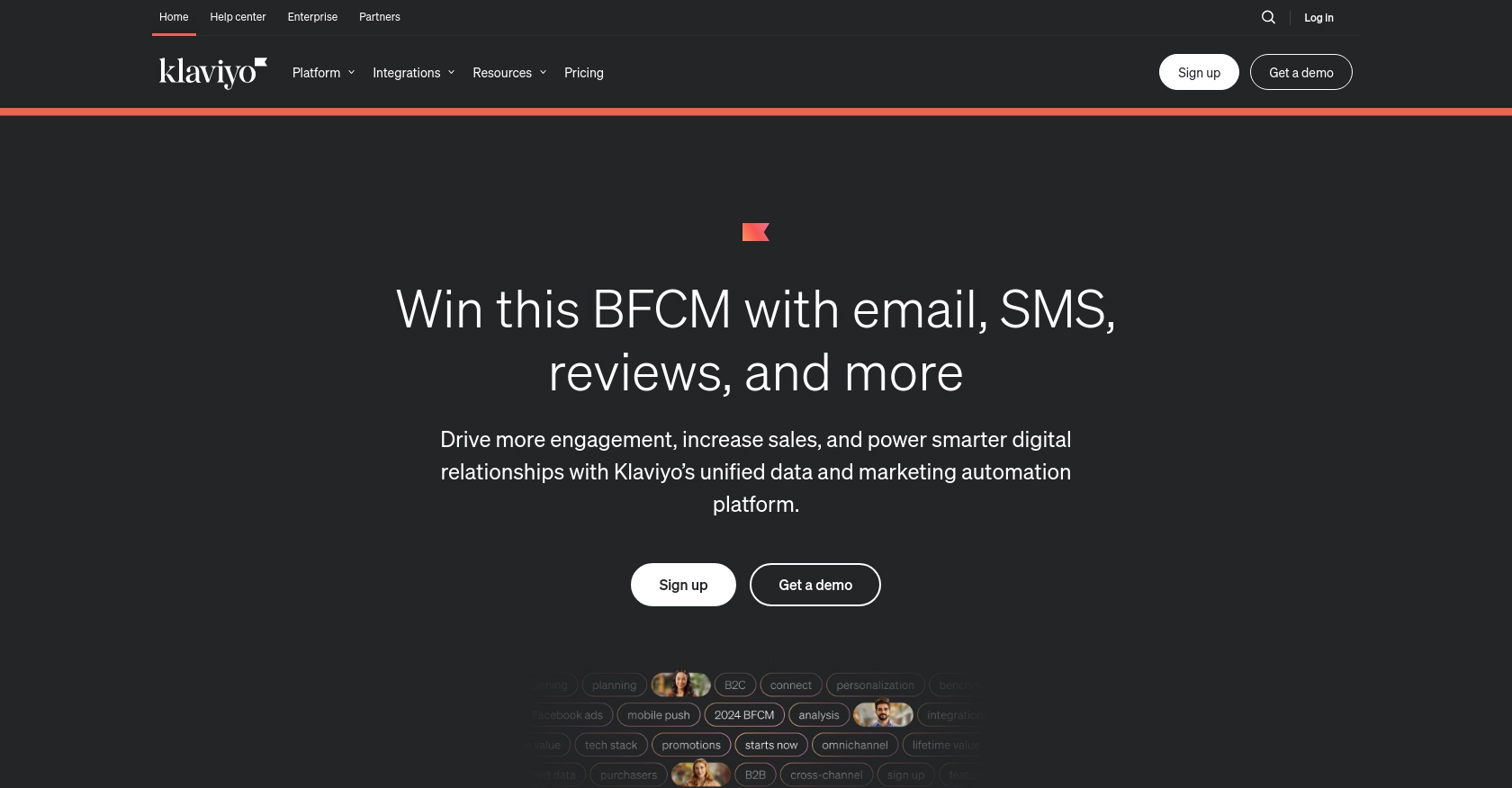
Introduction to Klaviyo API Integration
Klaviyo is a powerful email and SMS marketing platform that leverages data science to deliver personalized marketing experiences. It offers a robust API that allows developers to access and manage customer profiles, enabling businesses to create targeted marketing campaigns and enhance customer engagement.
Integrating with Klaviyo's API can be highly beneficial for developers looking to automate marketing processes. For example, you might want to retrieve customer profiles to segment your audience based on their behavior and preferences, allowing for more personalized communication strategies.
This guide will walk you through the process of using JavaScript to interact with the Klaviyo API, specifically focusing on retrieving customer profiles. By the end of this tutorial, you'll be equipped to efficiently access and manage profiles within your Klaviyo account.
Setting Up Your Klaviyo Test Account and Obtaining API Credentials
Before you can start interacting with the Klaviyo API, you'll need to set up a test account and obtain the necessary API credentials. This will allow you to authenticate your requests and access customer profiles securely.
Create a Klaviyo Test Account
If you don't already have a Klaviyo account, you can sign up for a free trial on the Klaviyo website. This will give you access to the platform's features and allow you to test API interactions without affecting live data.
- Visit the Klaviyo website and click on the "Sign Up" button.
- Fill in the required information to create your account.
- Once your account is set up, log in to access your dashboard.
Generate Klaviyo API Credentials
To interact with the Klaviyo API, you'll need to generate API credentials. Klaviyo uses API keys for authentication, which you can create from your account settings.
- Navigate to the "Account" section in your Klaviyo dashboard.
- Click on "Settings" and then select "API Keys" from the dropdown menu.
- Click on "Create API Key" to generate a new key. Make sure to copy and store this key securely, as it will be used to authenticate your API requests.
For more detailed instructions, refer to the Klaviyo API documentation.
Understanding Klaviyo API Key Scopes
When creating an API key, you can define scopes to restrict access to specific data. This is crucial for maintaining security and ensuring that only necessary data is accessible.
- Read-only: Allows viewing data without making changes.
- Full: Grants permissions to create, update, or delete data.
- Custom: Customize access based on specific needs.
Choose the appropriate scope for your API key based on your integration requirements. For more information, visit the Klaviyo API documentation.
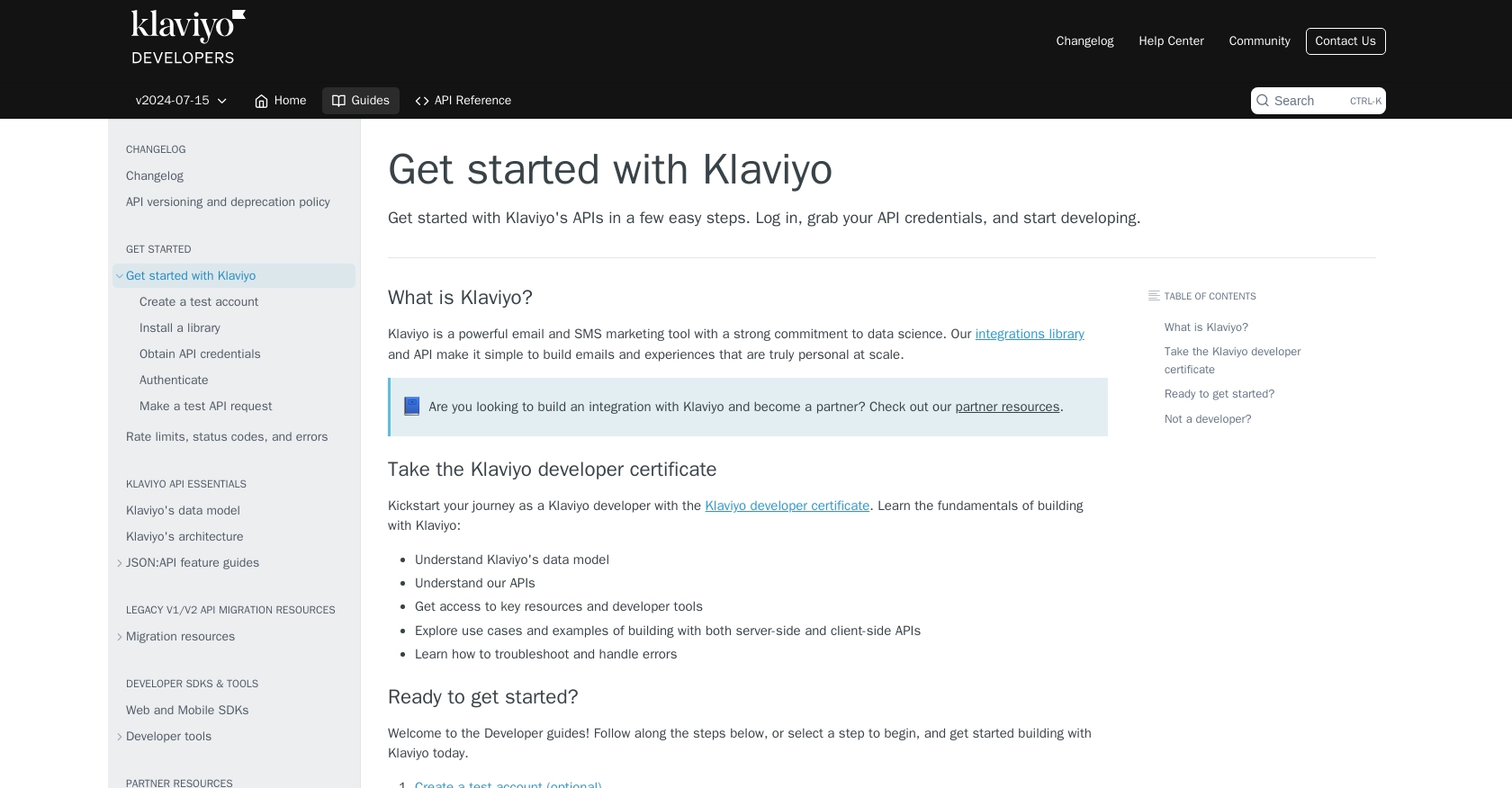
sbb-itb-96038d7
How to Make API Calls to Retrieve Profiles with Klaviyo API Using JavaScript
In this section, we will guide you through the process of making API calls to retrieve customer profiles from Klaviyo using JavaScript. This involves setting up your development environment, writing the necessary code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Klaviyo API Integration
Before making API calls, ensure you have a suitable JavaScript environment set up. You can use Node.js for server-side scripting or any modern browser for client-side scripting. Make sure to have the following installed:
- Node.js (if using server-side scripting)
- A code editor like Visual Studio Code
Additionally, you'll need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Retrieve Profiles from Klaviyo API
With your environment ready, you can now write the JavaScript code to interact with the Klaviyo API. Below is a sample code snippet to retrieve profiles:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://a.klaviyo.com/api/profiles/';
const headers = {
'Authorization': 'Klaviyo-API-Key your-private-api-key',
'accept': 'application/json',
'revision': '2024-07-15'
};
// Function to get profiles
async function getProfiles() {
try {
const response = await axios.get(endpoint, { headers });
const profiles = response.data.data;
console.log('Profiles retrieved:', profiles);
} catch (error) {
console.error('Error fetching profiles:', error.response.data);
}
}
// Call the function
getProfiles();
Replace your-private-api-key
with the API key you generated earlier. This code sets up the API endpoint and headers, makes a GET request to retrieve profiles, and logs the results to the console.
Handling API Responses and Errors in Klaviyo API Calls
When making API calls, it's crucial to handle responses and potential errors effectively. The Klaviyo API uses standard HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 400 Bad Request: The request is missing required parameters or has invalid parameters.
- 401 Unauthorized: Authentication failed due to invalid API key.
- 429 Rate Limit: Too many requests. Implement retry logic with exponential backoff.
For more detailed error handling, refer to the Klaviyo API documentation.
Verifying Successful API Requests in Klaviyo Dashboard
After executing the API call, verify the retrieved profiles by checking your Klaviyo dashboard. Ensure that the data matches the profiles in your account. This step helps confirm that your API integration is working correctly.
By following these steps, you can efficiently retrieve customer profiles from Klaviyo using JavaScript, enabling you to enhance your marketing strategies with personalized data insights.
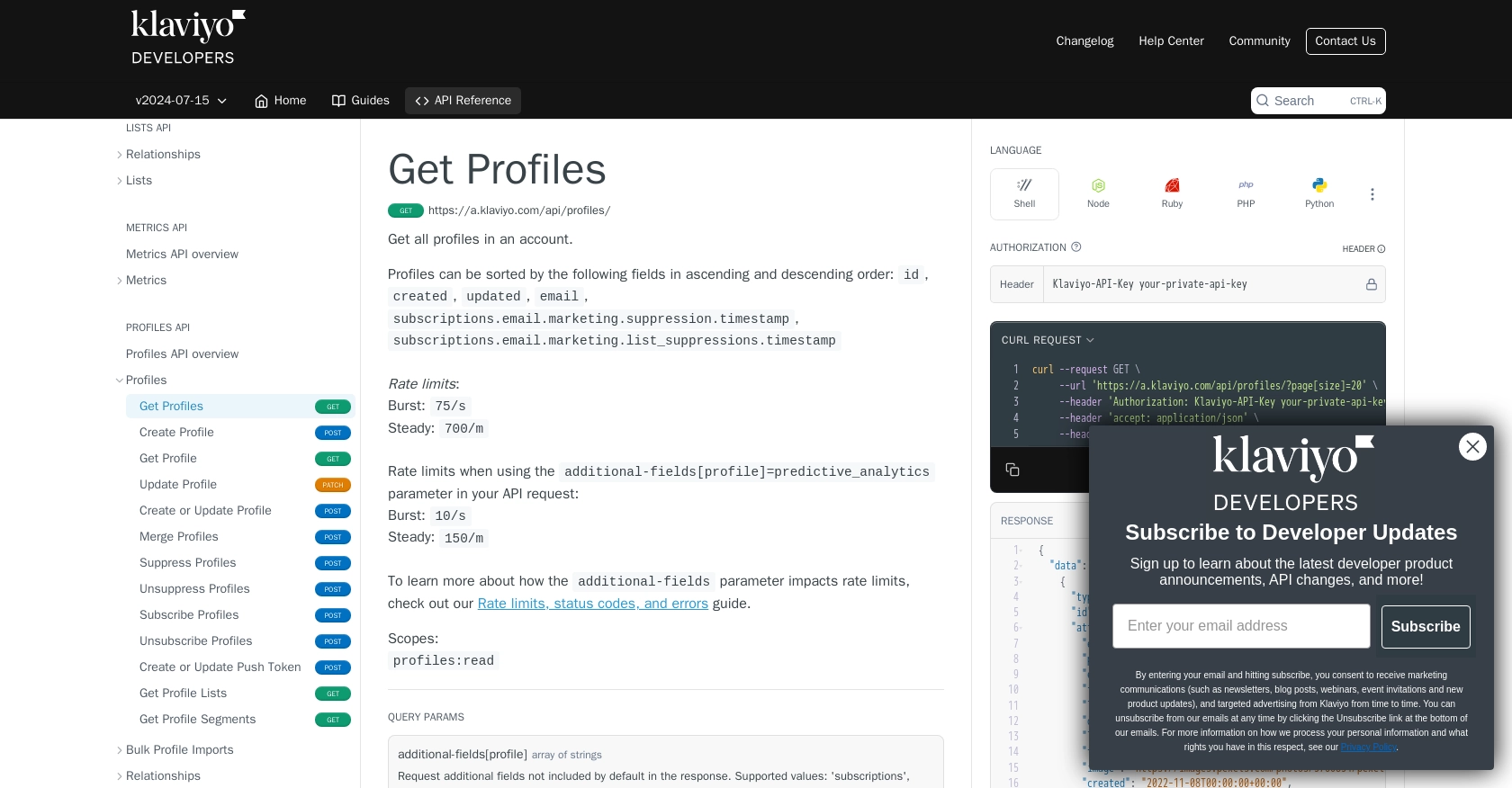
Conclusion and Best Practices for Klaviyo API Integration in JavaScript
Integrating with the Klaviyo API using JavaScript provides a powerful way to manage and retrieve customer profiles, enabling personalized marketing strategies. By following the steps outlined in this guide, you can efficiently access and handle customer data within your Klaviyo account.
Best Practices for Secure and Efficient Klaviyo API Usage
- Secure API Keys: Always store your API keys securely and avoid exposing them in client-side code or public repositories.
- Handle Rate Limits: Be mindful of Klaviyo's rate limits. Implement retry logic with exponential backoff to handle HTTP 429 errors effectively. For more details, refer to the Klaviyo API documentation.
- Optimize Data Requests: Use query parameters to request only the necessary data fields, reducing the load on your application and improving performance.
- Monitor API Usage: Regularly check your API usage and performance metrics to ensure efficient operation and identify any potential issues early.
Enhancing Integration Capabilities with Endgrate
While integrating with Klaviyo's API can be straightforward, managing multiple integrations can become complex. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Klaviyo. This allows you to streamline your integration processes, saving time and resources.
With Endgrate, you can focus on your core product development while outsourcing the complexities of integration management. Explore how Endgrate can enhance your integration experience by visiting Endgrate.
By adhering to these best practices and leveraging tools like Endgrate, you can ensure a robust and efficient integration with Klaviyo, empowering your marketing efforts with personalized customer insights.
Read More
Ready to get started?