Using the Front API to Get Conversations in PHP
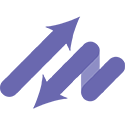
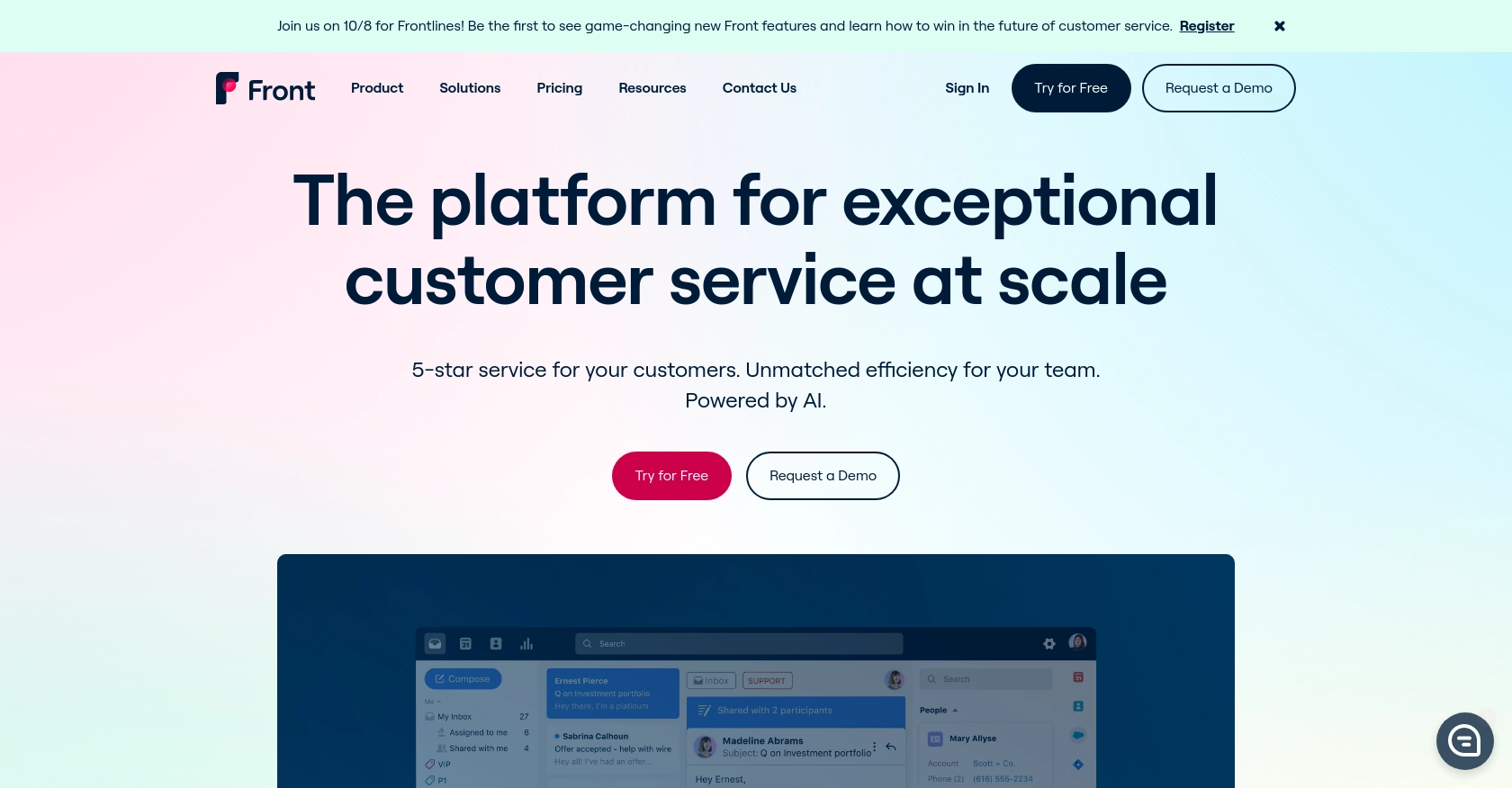
Introduction to Front API Integration
Front is a powerful communication platform that centralizes emails, messages, and other communication channels into a single collaborative space. It is widely used by businesses to enhance team collaboration and streamline customer interactions.
Developers may want to integrate with Front's API to automate and manage conversations efficiently. For example, using the Front API, a developer can retrieve conversations to analyze customer interactions or integrate with other systems for enhanced customer support workflows.
Setting Up Your Front Developer Account and API Access
Before you can start integrating with the Front API, you'll need to set up a developer account and obtain an API token. This will allow you to test your integration in a safe environment without affecting production data.
Create a Front Developer Account
If you don't already have a Front account, you can sign up for a free developer account. This account provides access to a developer environment where you can build and test your integrations.
- Visit the Front Developer Portal and click on the sign-up link.
- Follow the instructions to create your account. Once completed, you'll have access to the developer environment.
Generate an API Token for Front API Access
Front uses API tokens for authentication, allowing you to securely interact with their API. Follow these steps to generate your API token:
- Log in to your Front developer account.
- Navigate to the API tokens section in your account settings.
- Click on "Create API Token" and provide a name for your token.
- Select the appropriate scopes for your token, such as "Shared Resources," to ensure you have the necessary permissions.
- Click "Generate" to create your token. Make sure to copy and store it securely, as it will be used in your API requests.
With your developer account set up and your API token generated, you're ready to start making API calls to the Front platform.
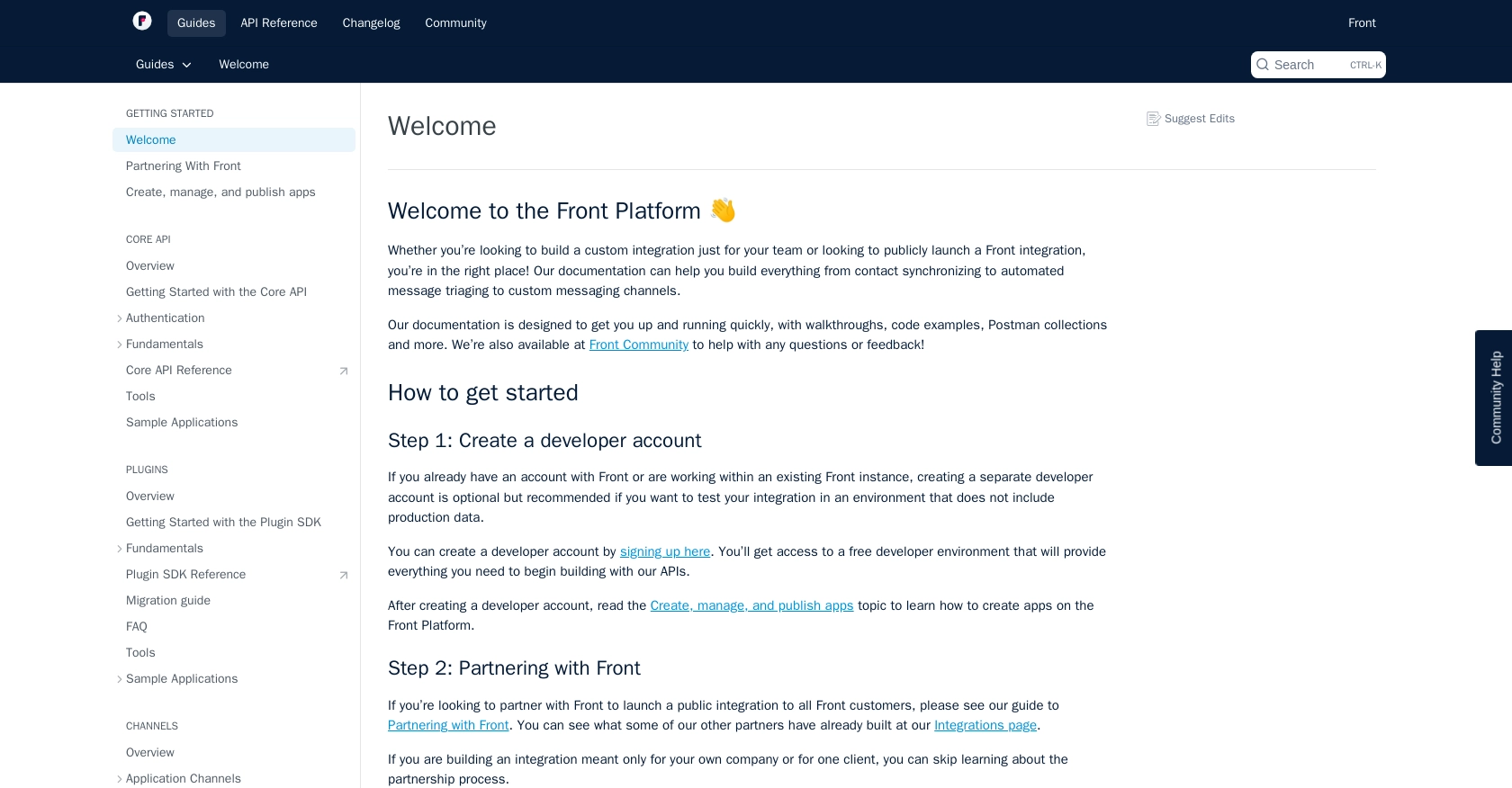
sbb-itb-96038d7
Making API Calls to Retrieve Conversations Using Front API in PHP
To interact with the Front API and retrieve conversations, you'll need to use PHP to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Front API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or later installed on your machine.
- The
cURL
extension enabled in your PHP configuration.
To verify that cURL is enabled, you can create a PHP file with the following content and run it:
<?php
phpinfo();
?>
Look for the cURL section in the output to confirm it's enabled.
Writing PHP Code to Make API Calls to Front
Now that your environment is ready, you can write the PHP code to make an API call to Front and retrieve conversations. Below is a sample script:
<?php
$apiToken = 'YOUR_API_TOKEN';
$endpoint = 'https://api2.frontapp.com/conversations';
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiToken,
'Accept: application/json'
]);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode JSON response
$data = json_decode($response, true);
// Display conversations
foreach ($data['_results'] as $conversation) {
echo 'Conversation ID: ' . $conversation['id'] . '<br>';
echo 'Subject: ' . $conversation['subject'] . '<br>';
echo 'Status: ' . $conversation['status'] . '<br><br>';
}
}
// Close cURL session
curl_close($ch);
?>
Replace YOUR_API_TOKEN
with the API token you generated earlier. This script initializes a cURL session, sets the necessary headers, and retrieves conversations from Front.
Handling API Responses and Errors
After executing the API call, it's crucial to handle the response properly. The sample code above checks for cURL errors and decodes the JSON response to extract conversation details. Ensure you handle potential errors by checking the HTTP status code and implementing retry logic if needed.
Verifying API Call Success in Front
To verify that your API call was successful, log in to your Front developer account and navigate to the conversations section. You should see the conversations listed in the response. If there are discrepancies, double-check your API token and endpoint URL.
Managing Rate Limits and Error Codes
Front's API has a rate limit of 50 requests per minute for the Starter plan. Monitor your API usage and handle the 429 Too Many Requests
error by implementing a retry mechanism with exponential backoff. For more details, refer to the Front API rate limiting documentation.
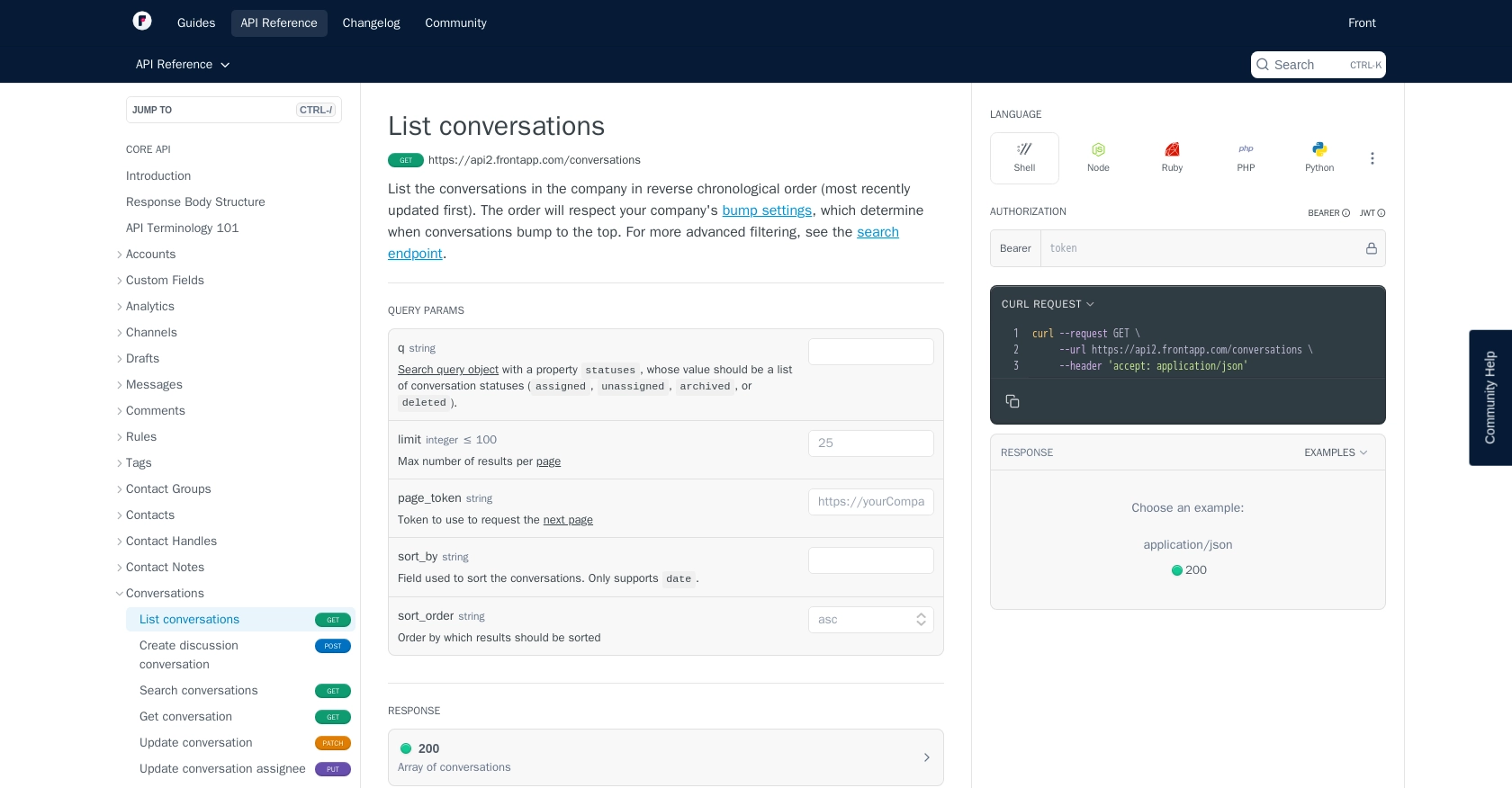
Best Practices for Front API Integration in PHP
When integrating with the Front API using PHP, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Secure API Tokens: Store your API tokens securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Be mindful of Front's rate limits, which start at 50 requests per minute for the Starter plan. Implement a retry mechanism with exponential backoff to handle
429 Too Many Requests
errors gracefully. - Handle Errors Gracefully: Always check the HTTP status codes and handle errors appropriately. Implement logging to capture error details for troubleshooting.
- Optimize Data Handling: Use pagination to efficiently handle large datasets and reduce the load on your application and Front's servers.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your systems and maintain data integrity.
Leveraging Endgrate for Seamless Front API Integrations
While building integrations with the Front API can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution to streamline this process by providing a unified API endpoint that connects to multiple platforms, including Front.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product by outsourcing integration development to Endgrate, reducing the need for in-house expertise.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across multiple platforms, simplifying maintenance and updates.
- Enhance Customer Experience: Provide your customers with a seamless and intuitive integration experience, improving satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/front
- https://dev.frontapp.com/docs/welcome
- https://dev.frontapp.com/docs/core-api-getting-started
- https://dev.frontapp.com/docs/oauth
- https://dev.frontapp.com/docs/rate-limiting
- https://dev.frontapp.com/docs/pagination
- https://dev.frontapp.com/reference/list-conversations
Ready to get started?