Using the Clickup API to Create Teams in Python
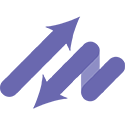
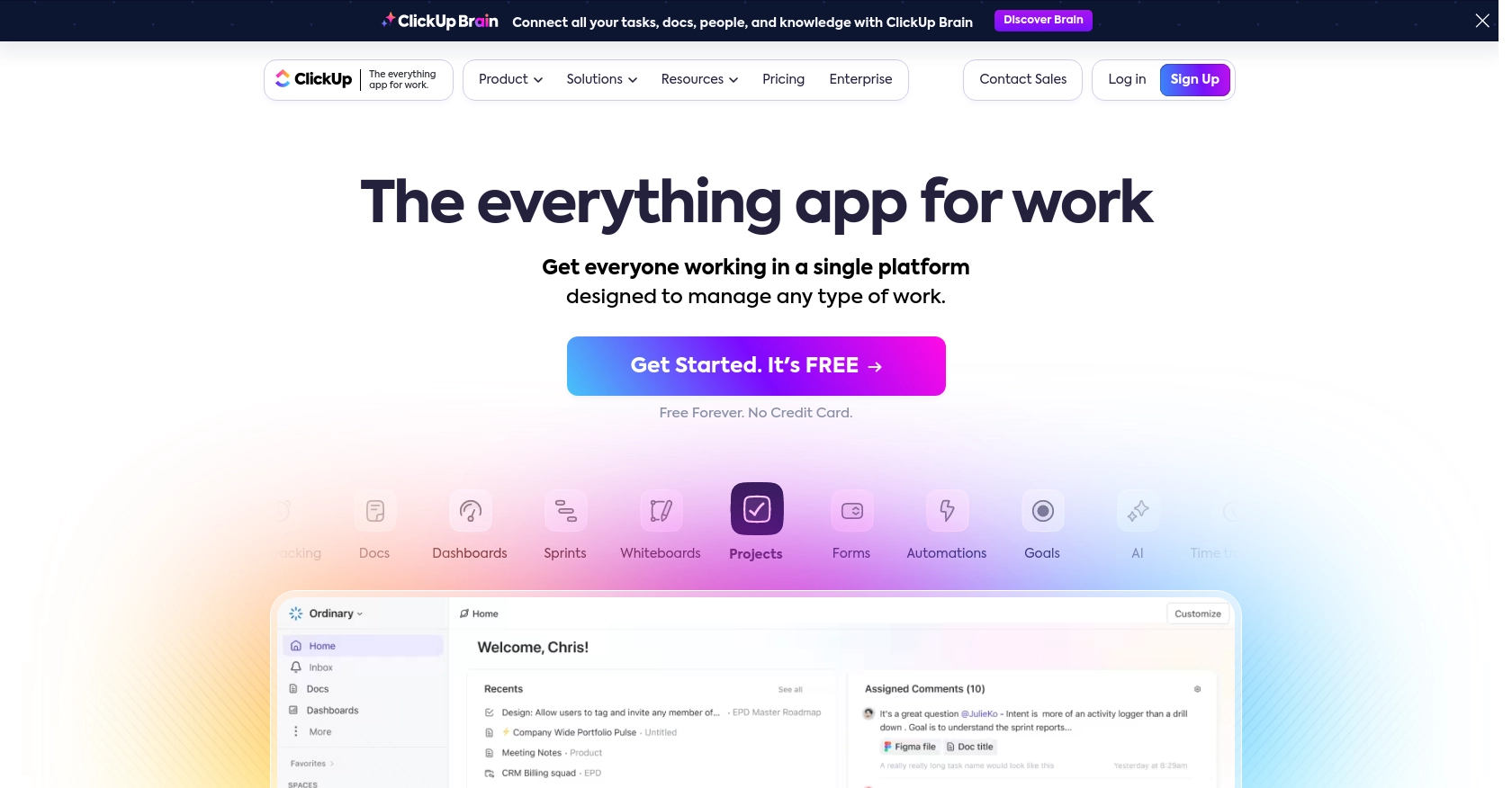
Introduction to ClickUp API
ClickUp is a versatile project management tool that offers a wide array of features to help teams collaborate and manage their workflows efficiently. From task management to time tracking, ClickUp provides a comprehensive platform for businesses to streamline their operations.
For developers, integrating with the ClickUp API can unlock powerful capabilities to automate and enhance project management processes. By using the ClickUp API, developers can create custom applications that interact with ClickUp's features, such as creating teams or user groups within a workspace.
For example, a developer might want to use the ClickUp API to create teams in Python, allowing for dynamic team management based on project needs. This can be particularly useful for organizations that frequently adjust team structures to accommodate different projects or client requirements.
Setting Up Your ClickUp Test/Sandbox Account
Before diving into the ClickUp API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. ClickUp offers a straightforward process to get started with their API using OAuth authentication.
Creating a ClickUp Account
If you don’t have a ClickUp account, you can sign up for a free account on the ClickUp website. Follow the instructions to create your account. If you already have an account, simply log in.
Generating a Personal API Token
For personal use or testing, you can generate a personal API token. Follow these steps:
- Log into ClickUp.
- In ClickUp 3.0, click your avatar in the upper-right corner and select Settings.
- Scroll down to the Apps section in the sidebar and click it.
- Under API Token, click Generate.
- Copy and save your personal API token for later use.
Note: Personal tokens begin with pk_
and are suitable for testing purposes.
Setting Up OAuth for Application Development
If you are developing an application for others, you need to use the OAuth flow:
- Create an OAuth App:
- Log into ClickUp.
- Click on your avatar in the lower-left corner and select Integrations.
- Click on ClickUp API and then Create an App.
- Provide a name and redirect URL for your app.
- Once created, note down the
client_id
andsecret
.
- Retrieve an Authorization Code:
- Share the following URL with users to authorize your app:
https://app.clickup.com/api?client_id={client_id}&redirect_uri={redirect_uri}
. - Ensure the
redirect_uri
matches the one used during app creation.
- Share the following URL with users to authorize your app:
- Request an Access Token:
- Use the authorization code to request an access token with the
client_id
,client_secret
, andcode
. - This token will be used in the Authorization header for API requests.
- Use the authorization code to request an access token with the
For more details on OAuth, refer to the ClickUp Authentication Documentation.
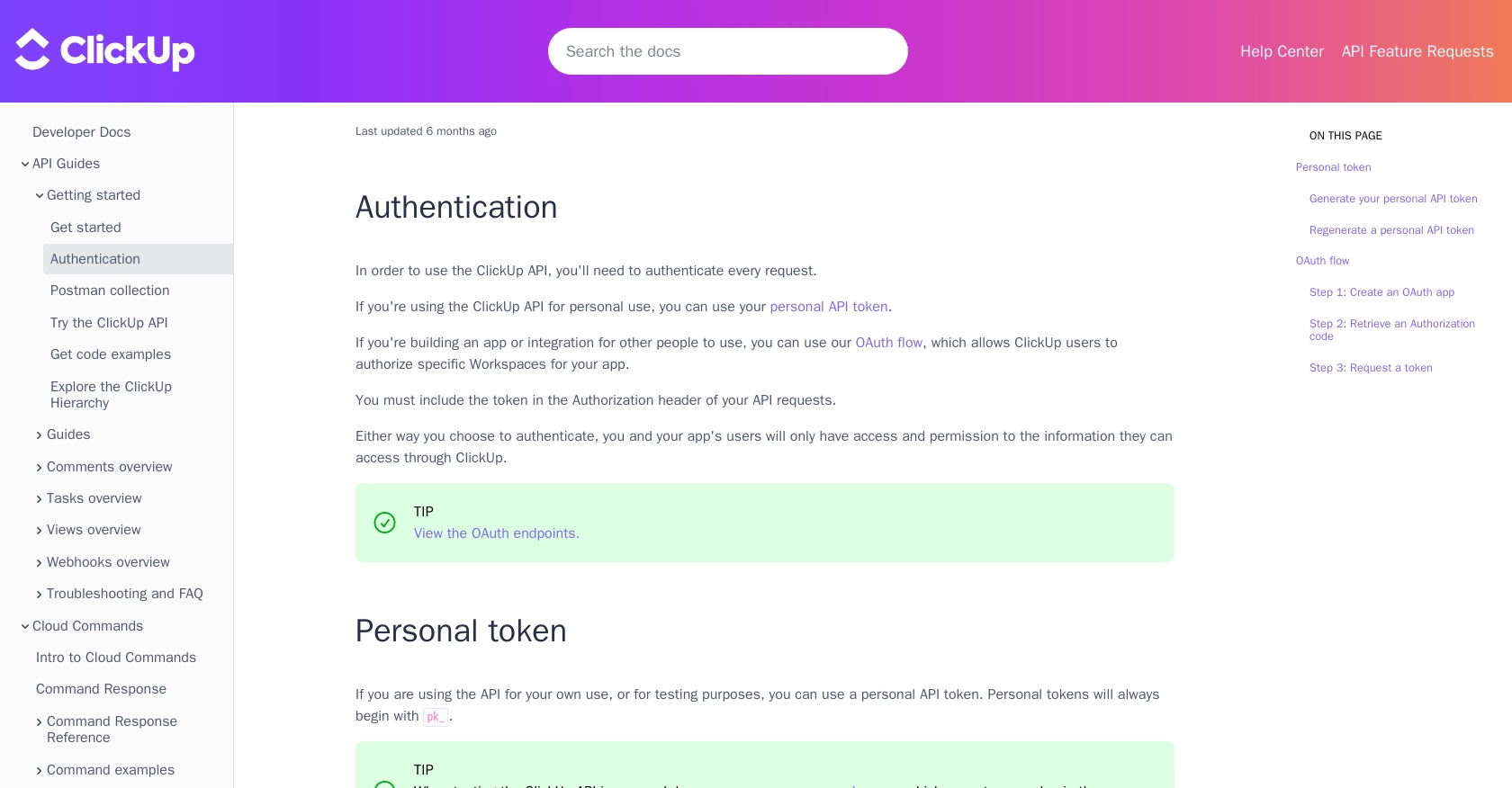
sbb-itb-96038d7
Making API Calls to Create Teams in ClickUp Using Python
To interact with the ClickUp API and create teams, you'll need to use Python to make HTTP requests. This section will guide you through the process of setting up your Python environment, writing the code to create a team, and handling potential errors.
Setting Up Your Python Environment for ClickUp API Integration
Before you start coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Create Teams in ClickUp
Now that your environment is ready, you can write the Python code to create a team in ClickUp. Create a new file named create_clickup_team.py
and add the following code:
import requests
# Set the API endpoint for creating a team
url = "https://api.clickup.com/v2/team/{team_id}/group"
# Set the request headers with your authorization token
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the team details
team_data = {
"name": "New Team Name",
"members": [123456, 987654]
}
# Make a POST request to the API
response = requests.post(url, json=team_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Team Created Successfully")
print(response.json())
else:
print("Failed to Create Team")
print(response.json())
Replace Your_Access_Token
with the access token obtained through the OAuth flow. The team_id
should be replaced with the ID of the workspace where you want to create the team.
Running the Python Script and Verifying the API Call
To execute the script, run the following command in your terminal:
python create_clickup_team.py
If successful, you should see "Team Created Successfully" along with the response data. Verify the creation by checking the ClickUp workspace for the new team.
Handling Errors and Understanding ClickUp API Responses
When making API calls, it's crucial to handle errors gracefully. The ClickUp API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
Always check the response status code and handle errors appropriately to ensure a robust integration.
For more details on API responses, refer to the ClickUp API Documentation.
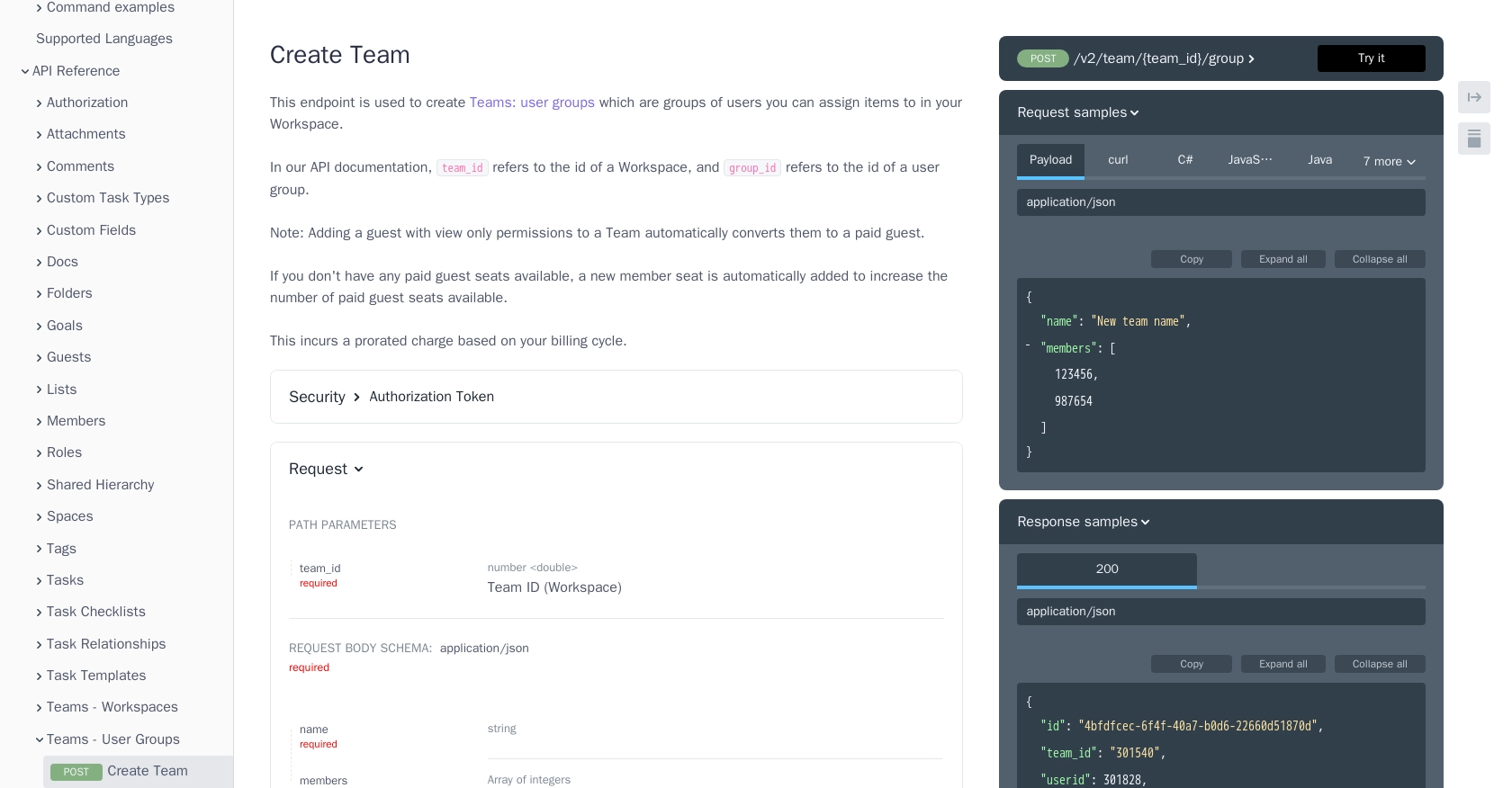
Conclusion and Best Practices for Using ClickUp API in Python
Integrating with the ClickUp API to create teams in Python can significantly enhance your project management capabilities by allowing dynamic team management and automation. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth, and make API calls to manage teams within your ClickUp workspace.
Best Practices for Secure and Efficient ClickUp API Integration
- Secure Storage of Credentials: Always store your API tokens and OAuth credentials securely. Consider using environment variables or secure vaults to keep sensitive information safe.
- Handle Rate Limiting: Be aware of ClickUp's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure that data fields are standardized and consistent across your application to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling to manage API response codes effectively. This will help maintain a smooth user experience and prevent unexpected failures.
Enhancing Integration with Endgrate
While integrating with ClickUp directly offers powerful capabilities, managing multiple integrations can be complex and time-consuming. Endgrate provides a unified API endpoint that simplifies the integration process across various platforms, including ClickUp.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. With Endgrate, you build once for each use case, reducing the need for multiple integrations and providing an intuitive experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
Ready to get started?