How to Create or Update Accounts with the Zoho Books API in Python
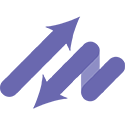
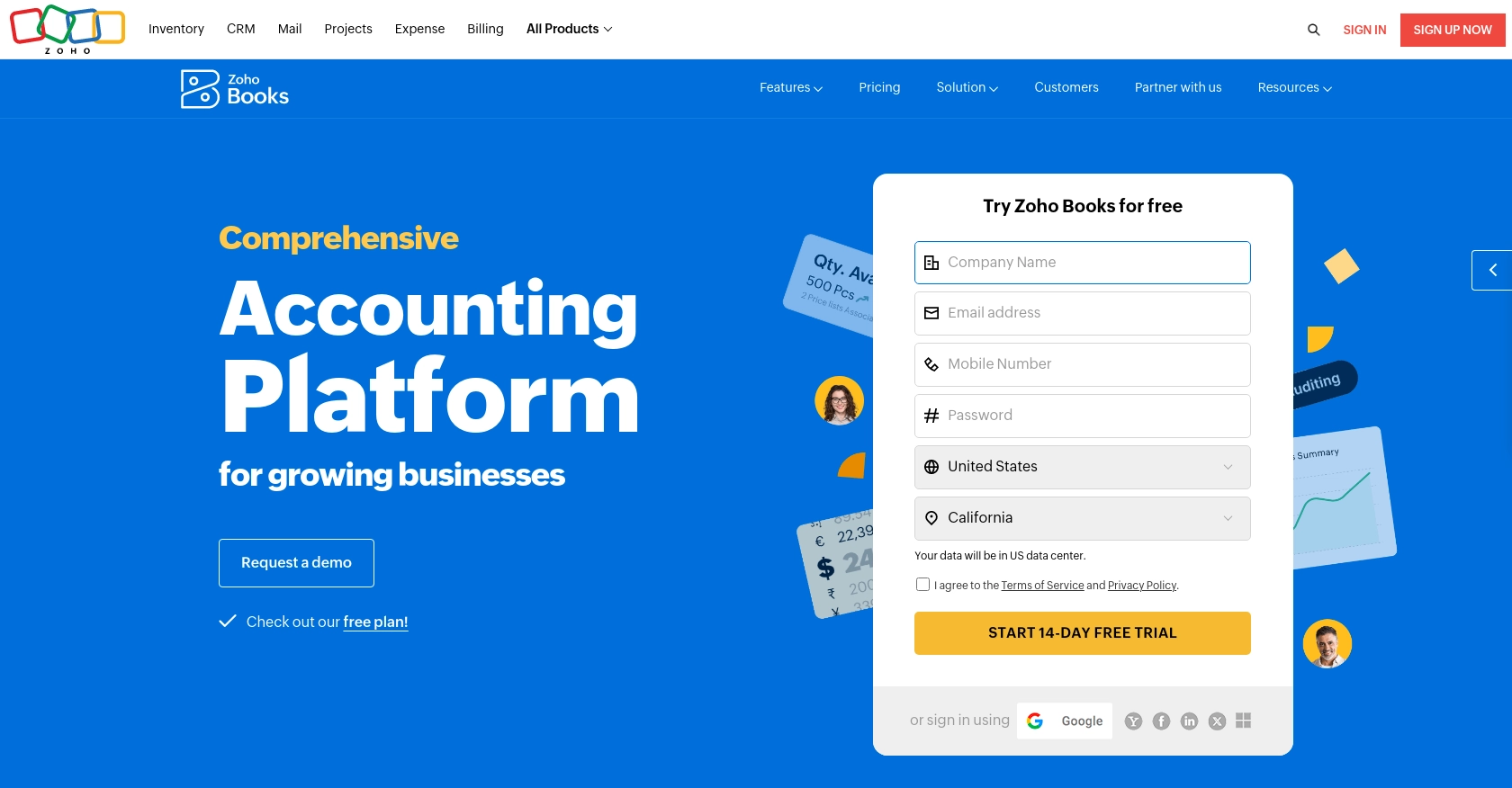
Introduction to Zoho Books API Integration
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features, including invoicing, expense tracking, and inventory management, making it a popular choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to automate and streamline accounting processes, such as creating or updating accounts. For example, a developer might use the Zoho Books API to automatically update account details when changes occur in a connected CRM system, ensuring that financial records are always up-to-date and accurate.
Setting Up Your Zoho Books Test/Sandbox Account
Before diving into the integration process, it's essential to set up a Zoho Books test or sandbox account. This will allow you to safely experiment with the API without affecting your live data. Zoho Books offers a free trial that you can use for testing purposes.
Creating a Zoho Books Account
To get started, visit the Zoho Books signup page and register for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is created, you will be logged into the Zoho Books dashboard.
Registering Your Application for OAuth Authentication
Zoho Books uses OAuth 2.0 for secure API access. Follow these steps to register your application and obtain the necessary credentials:
- Go to the Zoho Developer Console.
- Click on Add Client ID to register your application.
- Fill in the required details, such as the application name and redirect URI.
- Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure.
Generating OAuth Tokens
To interact with the Zoho Books API, you need to generate an access token using the OAuth credentials:
- Redirect users to the following URL to obtain a grant token:
- After user consent, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- Store the received access token and refresh token securely for future API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on OAuth setup, refer to the Zoho Books OAuth documentation.
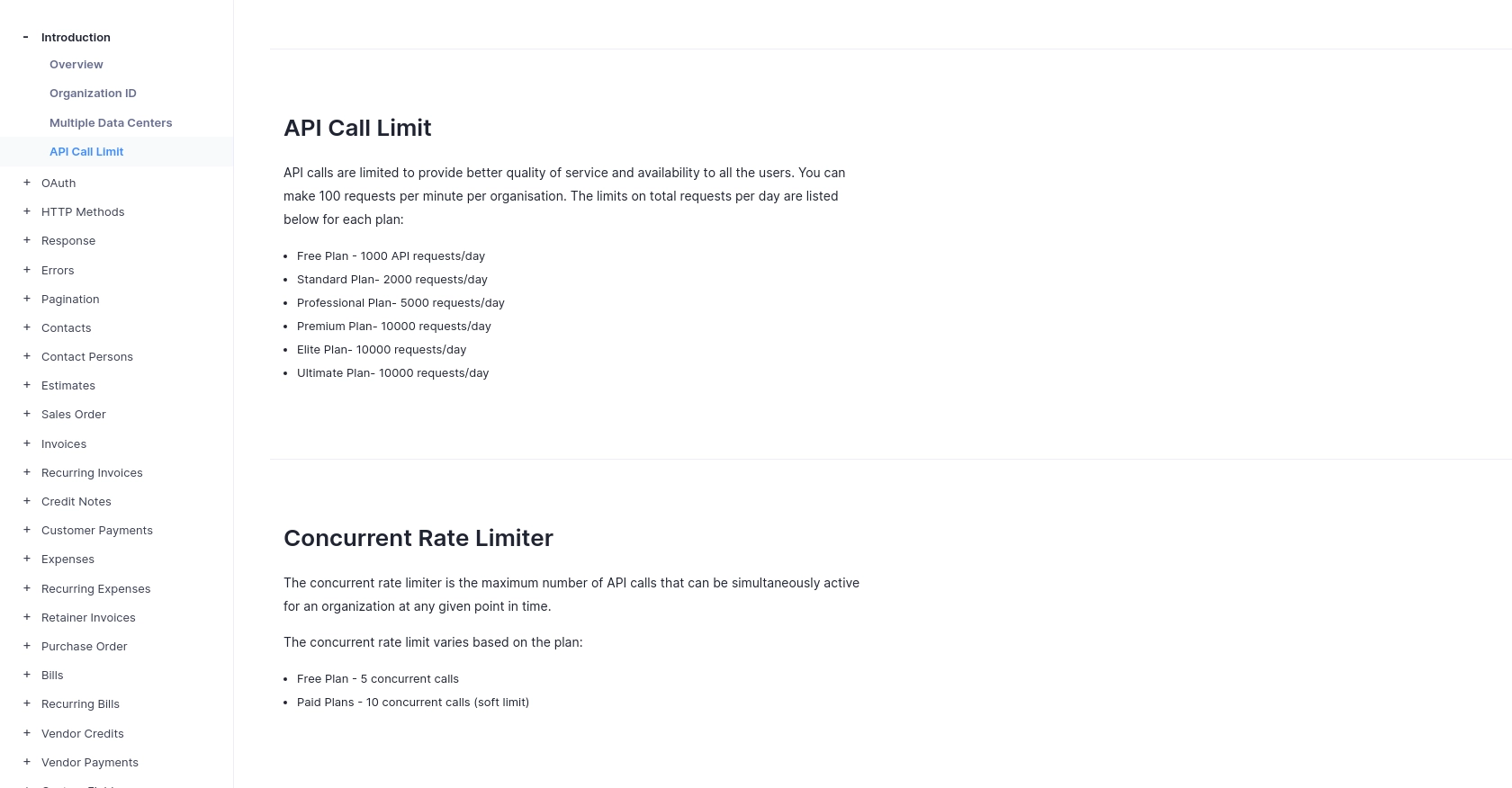
sbb-itb-96038d7
Making API Calls to Create or Update Accounts in Zoho Books Using Python
To interact with the Zoho Books API for creating or updating accounts, you'll need to use Python. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment
Before making API calls, ensure you have Python 3.x installed on your machine. You will also need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Creating an Account with Zoho Books API
To create an account in Zoho Books, you'll need to make a POST request to the API endpoint. Here's how you can do it:
import requests
# Define the API endpoint and headers
url = "https://www.zohoapis.com/books/v3/chartofaccounts?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the account details
account_data = {
"account_name": "New Account",
"account_code": "12345",
"account_type": "income",
"currency_id": "460000000000097",
"description": "Description of the new account"
}
# Make the POST request
response = requests.post(url, headers=headers, json=account_data)
# Check the response
if response.status_code == 201:
print("Account created successfully.")
else:
print("Failed to create account:", response.json())
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token. The response will confirm if the account was created successfully.
Updating an Existing Account in Zoho Books
To update an existing account, use a PUT request. Here's an example:
import requests
# Define the API endpoint and headers
account_id = "460000000038079" # Replace with your account ID
url = f"https://www.zohoapis.com/books/v3/chartofaccounts/{account_id}?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the updated account details
updated_data = {
"account_name": "Updated Account Name",
"description": "Updated description"
}
# Make the PUT request
response = requests.put(url, headers=headers, json=updated_data)
# Check the response
if response.status_code == 200:
print("Account updated successfully.")
else:
print("Failed to update account:", response.json())
Ensure you replace YOUR_ORG_ID
, YOUR_ACCESS_TOKEN
, and account_id
with the appropriate values. The response will indicate whether the update was successful.
Handling API Responses and Errors
Zoho Books API uses standard HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 201 Created: The resource was created successfully.
- 400 Bad Request: The request was invalid.
- 401 Unauthorized: Authentication failed.
- 429 Rate Limit Exceeded: Too many requests.
For more details on error codes, refer to the Zoho Books Error Documentation.
By following these steps, you can effectively create or update accounts in Zoho Books using Python. Ensure you handle errors gracefully and adhere to the API rate limits of 100 requests per minute per organization as specified in the API Call Limit Documentation.
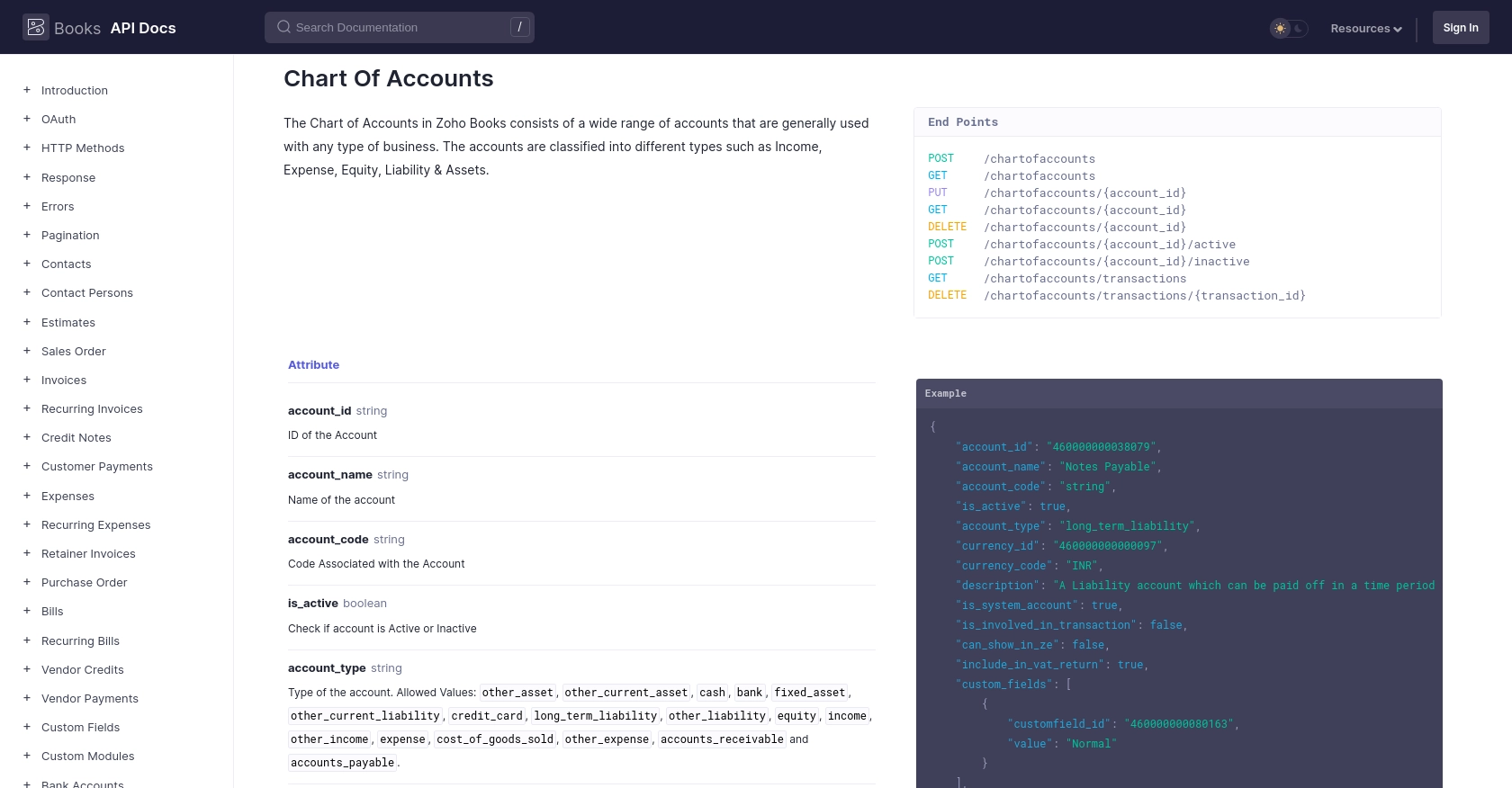
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using Python offers a powerful way to automate and streamline your accounting processes. By following the steps outlined in this guide, you can effectively create or update accounts, ensuring your financial data remains accurate and up-to-date.
Best Practices for Secure and Efficient Zoho Books API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, such as the Client ID, Client Secret, and access tokens, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Zoho Books enforces a rate limit of 100 requests per minute per organization. Implement logic to handle rate limit errors (HTTP 429) by retrying requests after a delay. For more details, refer to the API Call Limit Documentation.
- Graceful Error Handling: Implement robust error handling to manage API responses effectively. Use the error codes provided by Zoho Books to identify and resolve issues promptly. More information can be found in the Error Documentation.
- Data Transformation and Standardization: Ensure that data fields are transformed and standardized to match your application's requirements. This will help maintain consistency across different systems.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Zoho Books, allowing you to focus on your core product while outsourcing integrations. Learn more about how Endgrate can enhance your integration experience by visiting Endgrate.
By adhering to these best practices and leveraging tools like Endgrate, you can ensure a seamless and efficient integration with Zoho Books, enhancing your business operations and productivity.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/chart-of-accounts/#overview
Ready to get started?