How to Get Questions with the Google Forms API in PHP
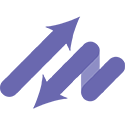
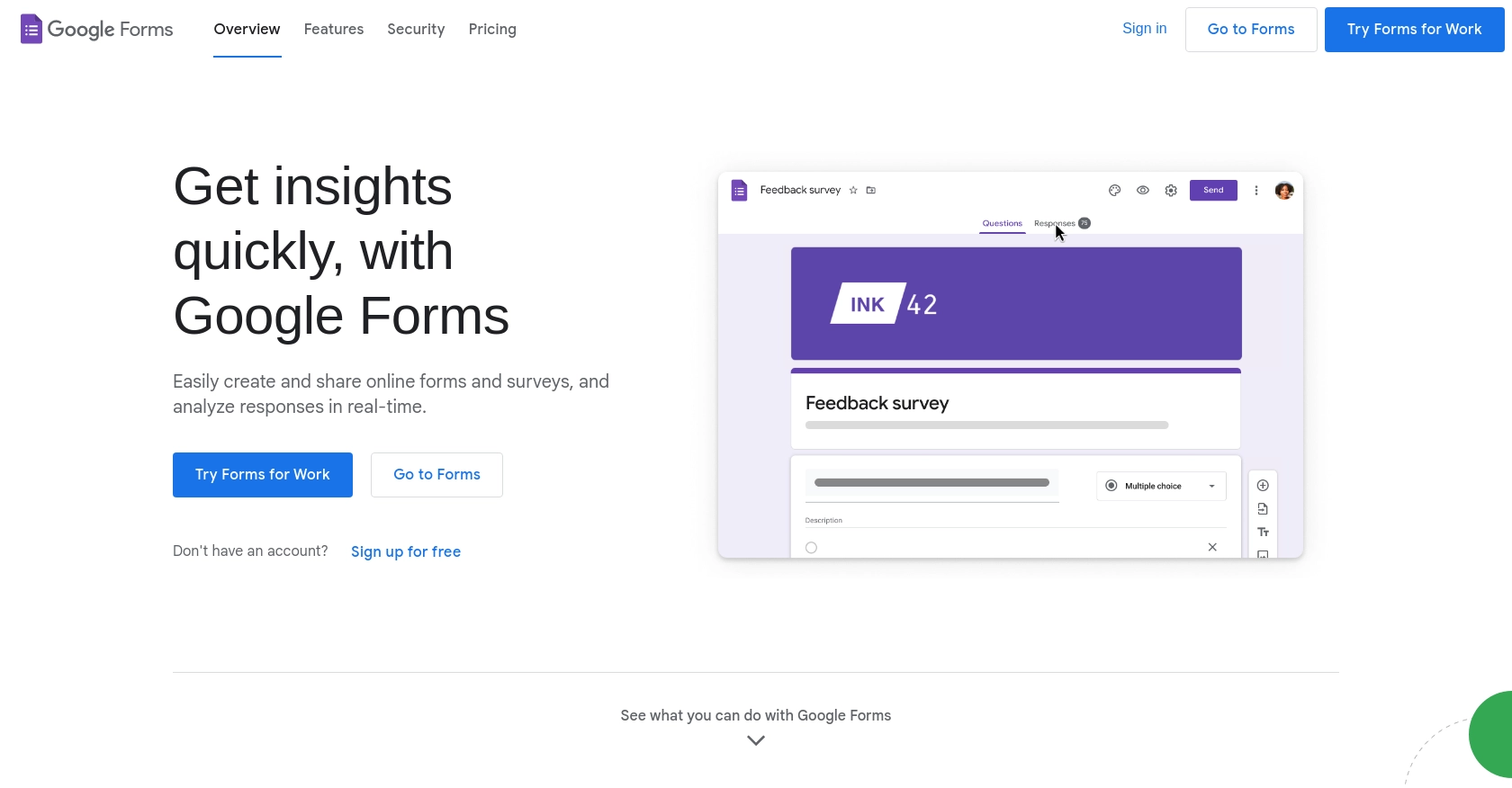
Introduction to Google Forms API
Google Forms is a versatile tool within the Google Workspace suite, allowing users to create surveys, quizzes, and forms with ease. It is widely used for collecting data, conducting research, and managing event registrations due to its user-friendly interface and seamless integration with other Google services.
For developers, integrating with the Google Forms API opens up opportunities to automate and enhance data collection processes. By accessing form data programmatically, developers can streamline workflows, such as automatically retrieving form questions to dynamically generate reports or dashboards.
In this article, we will explore how to interact with the Google Forms API using PHP, focusing on retrieving questions from a form. This integration can be particularly useful for developers looking to automate data analysis or integrate form responses with other applications.
Setting Up Your Google Forms API Test Account
Before you can start interacting with the Google Forms API using PHP, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup allows you to securely access the API and retrieve form questions programmatically.
Step 1: Create a Google Cloud Project
To begin, you'll need a Google Cloud project. This project will serve as the foundation for enabling APIs and managing credentials.
- Visit the Google Cloud Console and sign in with your Google account.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Step 2: Enable the Google Forms API
Once your project is created, you need to enable the Google Forms API to allow your application to interact with it.
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Google Forms API" and click on it.
- Click the Enable button to activate the API for your project.
Step 3: Configure OAuth Consent Screen
Configuring the OAuth consent screen is essential for defining what users will see when your application requests access to their data.
- Navigate to Menu > APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields, such as app name and support email, then click Save and Continue.
Step 4: Create OAuth 2.0 Credentials
To authenticate users and access the Google Forms API, you need to create OAuth 2.0 credentials.
- Go to Menu > APIs & Services > Credentials.
- Click Create Credentials > OAuth client ID.
- Select Web application as the application type.
- Enter a name for your client ID and add authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as they will be used in your PHP application to authenticate API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Access Credentials.
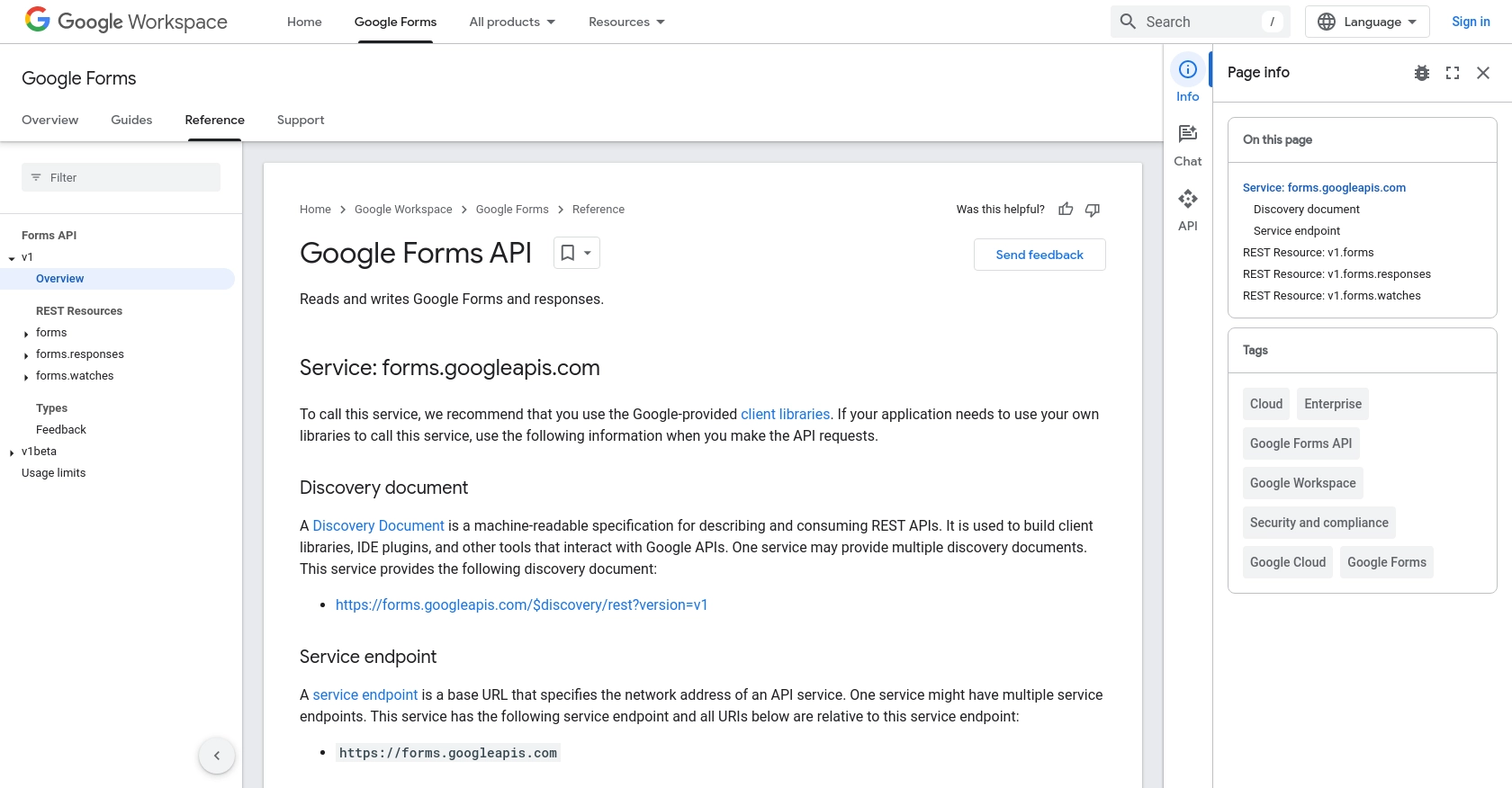
sbb-itb-96038d7
Making API Calls to Retrieve Questions from Google Forms Using PHP
Once you have set up your Google Cloud project and configured OAuth 2.0 authentication, you can start making API calls to interact with the Google Forms API. In this section, we will guide you through the process of retrieving questions from a Google Form using PHP.
Prerequisites for Google Forms API Integration with PHP
Before proceeding, ensure you have the following installed on your system:
- PHP 7.4 or higher
- Composer (PHP package manager)
- Google Client Library for PHP
To install the Google Client Library, run the following command in your terminal:
composer require google/apiclient:^2.0
Setting Up Your PHP Script to Access Google Forms API
Create a new PHP file, for example, get_google_forms_questions.php
, and include the Google Client Library:
<?php
require 'vendor/autoload.php';
use Google\Client;
use Google\Service\Forms;
// Initialize the Google Client
$client = new Client();
$client->setAuthConfig('path/to/your/client_credentials.json');
$client->addScope('https://www.googleapis.com/auth/forms');
// Initialize the Google Forms service
$service = new Forms($client);
?>
Retrieving Questions from a Google Form
To retrieve questions, you need to make a GET request to the Google Forms API. Use the following code to fetch questions from a specific form:
<?php
// Replace 'your-form-id' with the actual form ID
$formId = 'your-form-id';
try {
// Get the form details
$form = $service->forms->get($formId);
// Loop through the form items to get questions
foreach ($form->items as $item) {
if (isset($item->questionItem)) {
echo "Question: " . $item->title . "\n";
}
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Replace 'your-form-id'
with the ID of the form you want to access. This script will output the titles of all questions in the specified form.
Verifying API Request Success
To ensure your API request was successful, check the output of your script. If the questions are displayed correctly, your request was successful. If you encounter errors, refer to the error message for troubleshooting.
Handling Errors and Common Error Codes
When interacting with the Google Forms API, you may encounter various error codes. Here are some common ones:
- 401 Unauthorized: Ensure your OAuth credentials are correct and have the necessary permissions.
- 404 Not Found: Verify that the form ID is correct and that the form exists.
- 500 Internal Server Error: This may indicate a temporary issue with the Google Forms service. Try again later.
For more detailed error handling, refer to the Google Forms API documentation.
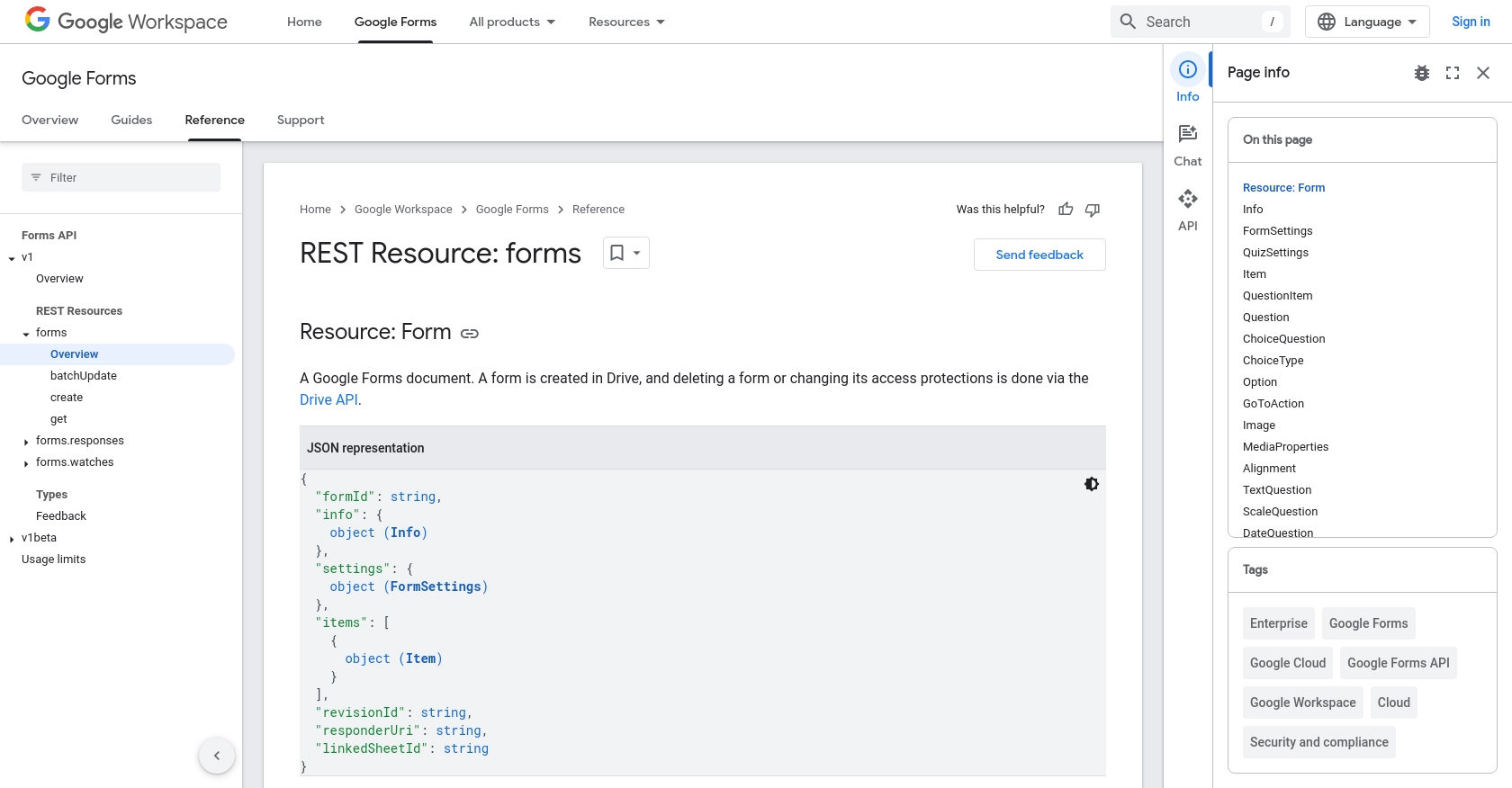
Conclusion and Best Practices for Google Forms API Integration in PHP
Integrating with the Google Forms API using PHP offers developers a powerful way to automate and enhance data collection processes. By programmatically accessing form questions, you can streamline workflows, generate dynamic reports, and integrate form responses with other applications.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth client ID and client secret securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies for retrying requests when encountering rate limit errors.
- Standardize Data Fields: Ensure that data retrieved from Google Forms is standardized and transformed as needed for seamless integration with other systems.
- Error Handling: Implement robust error handling to manage API errors gracefully. Log errors for monitoring and debugging purposes.
By following these best practices, you can ensure a secure, efficient, and reliable integration with the Google Forms API.
Streamline Your Integrations with Endgrate
While integrating with Google Forms API can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Google Forms.
With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case instead of multiple times for different integrations, and provide an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate.
Read More
- https://endgrate.com/provider/googleforms
- https://developers.google.com/forms/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/forms/api/reference/rest/v1/forms
Ready to get started?