Using the Outreach API to Get Prospects (with PHP examples)
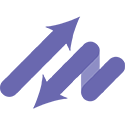
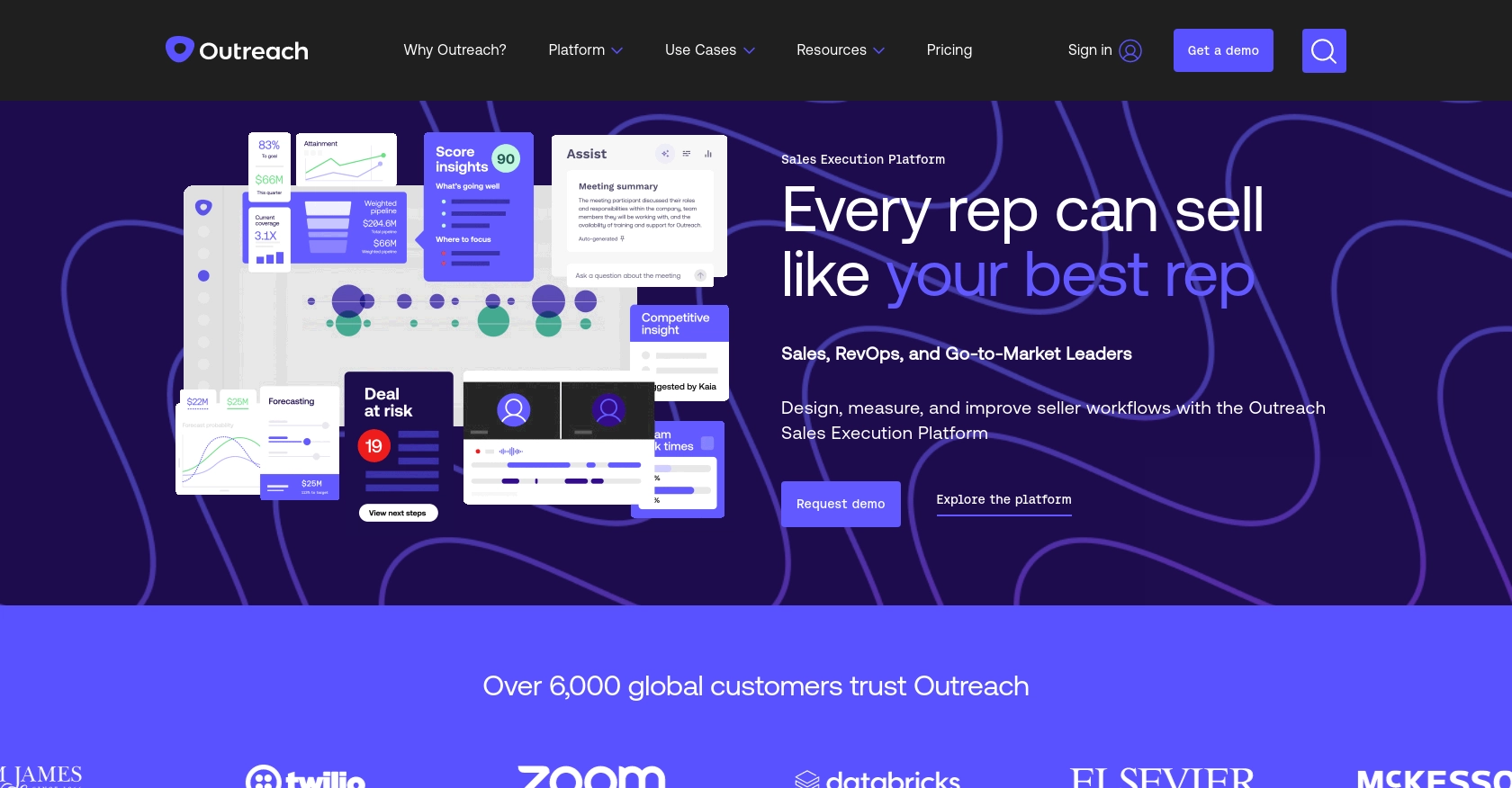
Introduction to Outreach API
Outreach is a powerful sales engagement platform that helps businesses streamline their sales processes and improve productivity. It offers a comprehensive suite of tools for managing customer interactions, tracking sales activities, and optimizing outreach strategies.
Integrating with the Outreach API allows developers to access and manage prospect data efficiently. By leveraging this API, developers can automate tasks such as retrieving prospect information, which can be used to enhance sales strategies and personalize communication efforts.
For example, a developer might use the Outreach API to fetch a list of prospects and analyze their engagement levels to tailor follow-up actions. This integration can significantly enhance the effectiveness of sales campaigns and improve customer relationship management.
Setting Up Your Outreach Test Account for API Integration
Before diving into the Outreach API, you'll need to set up a test account. This allows you to safely experiment with API calls without affecting live data. Outreach offers a development environment where you can create and manage test data.
Creating an Outreach Developer Account
If you don't have an Outreach account, start by signing up for a developer account on the Outreach website. This account will give you access to the necessary tools and resources for API integration.
- Visit the Outreach Developer Portal.
- Follow the instructions to create a new account.
- Once your account is set up, log in to access the developer dashboard.
Configuring OAuth for Outreach API Access
The Outreach API uses OAuth 2.0 for authentication. You'll need to create an app within your developer account to obtain the necessary credentials.
- Navigate to the "My Apps" section in your Outreach developer dashboard.
- Click on "Create App" and fill in the required details, such as the app name and description.
- Under the "API Access" tab, configure the OAuth settings:
- Specify one or more redirect URIs.
- Select the OAuth scopes your application will use, such as
prospects.read
. - Save your settings to generate the client ID and client secret.
Note: OAuth client secrets are displayed only once. Ensure you store them securely.
Obtaining Access and Refresh Tokens
After setting up your app, you can obtain access and refresh tokens to authenticate API requests.
// Example PHP code to obtain an access token
$client_id = 'your_client_id';
$client_secret = 'your_client_secret';
$redirect_uri = 'your_redirect_uri';
$authorization_code = 'authorization_code_from_redirect';
$ch = curl_init('https://api.outreach.io/oauth/token');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, [
'client_id' => $client_id,
'client_secret' => $client_secret,
'redirect_uri' => $redirect_uri,
'grant_type' => 'authorization_code',
'code' => $authorization_code
]);
$response = curl_exec($ch);
curl_close($ch);
$tokens = json_decode($response, true);
$access_token = $tokens['access_token'];
$refresh_token = $tokens['refresh_token'];
Store the refresh token securely and use it to obtain new access tokens when needed.
For more details, refer to the Outreach OAuth Documentation.
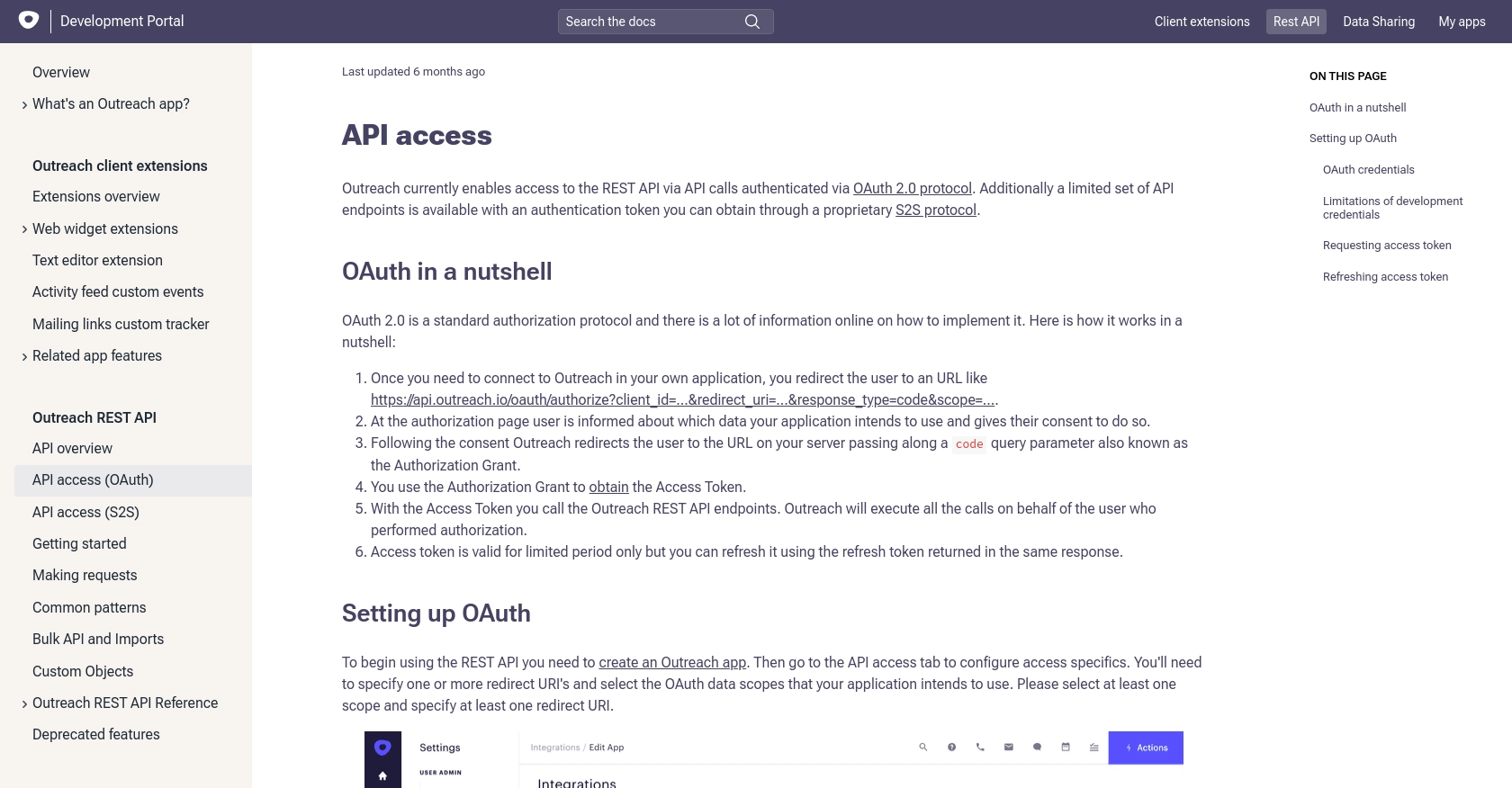
sbb-itb-96038d7
Making API Calls to Retrieve Prospects from Outreach Using PHP
To interact with the Outreach API and retrieve prospect data, you'll need to make authenticated API calls. This section will guide you through the process of setting up your PHP environment, making the necessary API requests, and handling the responses effectively.
Setting Up Your PHP Environment for Outreach API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- cURL extension for PHP
These tools will enable you to send HTTP requests and handle JSON responses from the Outreach API.
Making a GET Request to Fetch Prospects from Outreach
Once your environment is set up, you can proceed to make a GET request to the Outreach API to retrieve a list of prospects. Here's a step-by-step guide:
// Set the API endpoint and headers
$endpoint = 'https://api.outreach.io/api/v2/prospects';
$access_token = 'your_access_token';
$headers = [
'Authorization: Bearer ' . $access_token,
'Content-Type: application/vnd.api+json'
];
// Initialize cURL session
$ch = curl_init($endpoint);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode the JSON response
$data = json_decode($response, true);
// Check for errors
if (isset($data['errors'])) {
echo 'Error: ' . $data['errors'][0]['detail'];
} else {
// Loop through the prospects and print their information
foreach ($data['data'] as $prospect) {
echo 'Prospect Name: ' . $prospect['attributes']['name'] . "\n";
}
}
Replace your_access_token
with the access token obtained from the OAuth process. This script initializes a cURL session, sets the necessary headers, and executes a GET request to the Outreach API endpoint for prospects.
Verifying Successful API Requests and Handling Errors
After executing the API call, it's crucial to verify the success of the request. Check the response for any errors and handle them appropriately. The Outreach API provides detailed error messages that can help you troubleshoot issues.
If the request is successful, the response will contain a list of prospects. You can iterate through this list and extract the desired information, such as prospect names or email addresses.
Handling Outreach API Rate Limits and Best Practices
The Outreach API has a rate limit of 10,000 requests per hour. To avoid hitting this limit, implement strategies such as caching responses and minimizing unnecessary API calls. Monitor the rate limit headers in the API response to manage your request quota effectively.
For more information on handling rate limits and best practices, refer to the Outreach API Documentation.
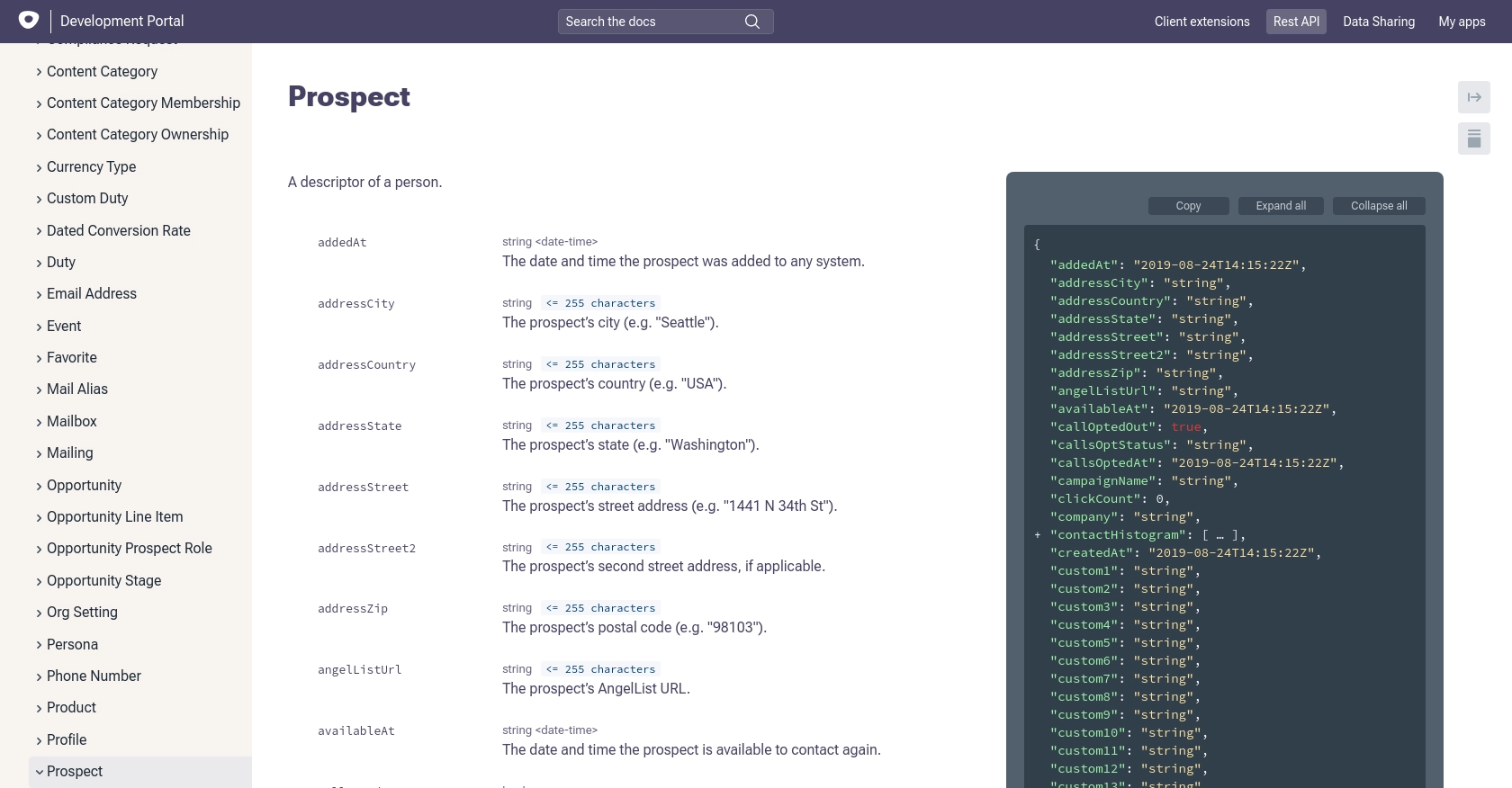
Conclusion and Best Practices for Using Outreach API with PHP
Integrating with the Outreach API using PHP can significantly enhance your sales engagement strategies by automating the retrieval and management of prospect data. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth, and make API calls to access valuable prospect information.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your OAuth client ID, client secret, and tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handling Rate Limits: Be mindful of the Outreach API's rate limit of 10,000 requests per hour. Implement caching strategies and monitor rate limit headers to optimize your API usage.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your applications and systems.
- Error Handling: Implement robust error handling to manage API errors gracefully. Utilize the detailed error messages provided by the Outreach API to troubleshoot issues effectively.
Enhancing Integration Efficiency with Endgrate
While integrating with the Outreach API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Outreach.
By leveraging Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. This allows you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?