How to Get Leads with the Zoho CRM API in Javascript
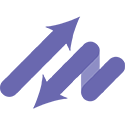
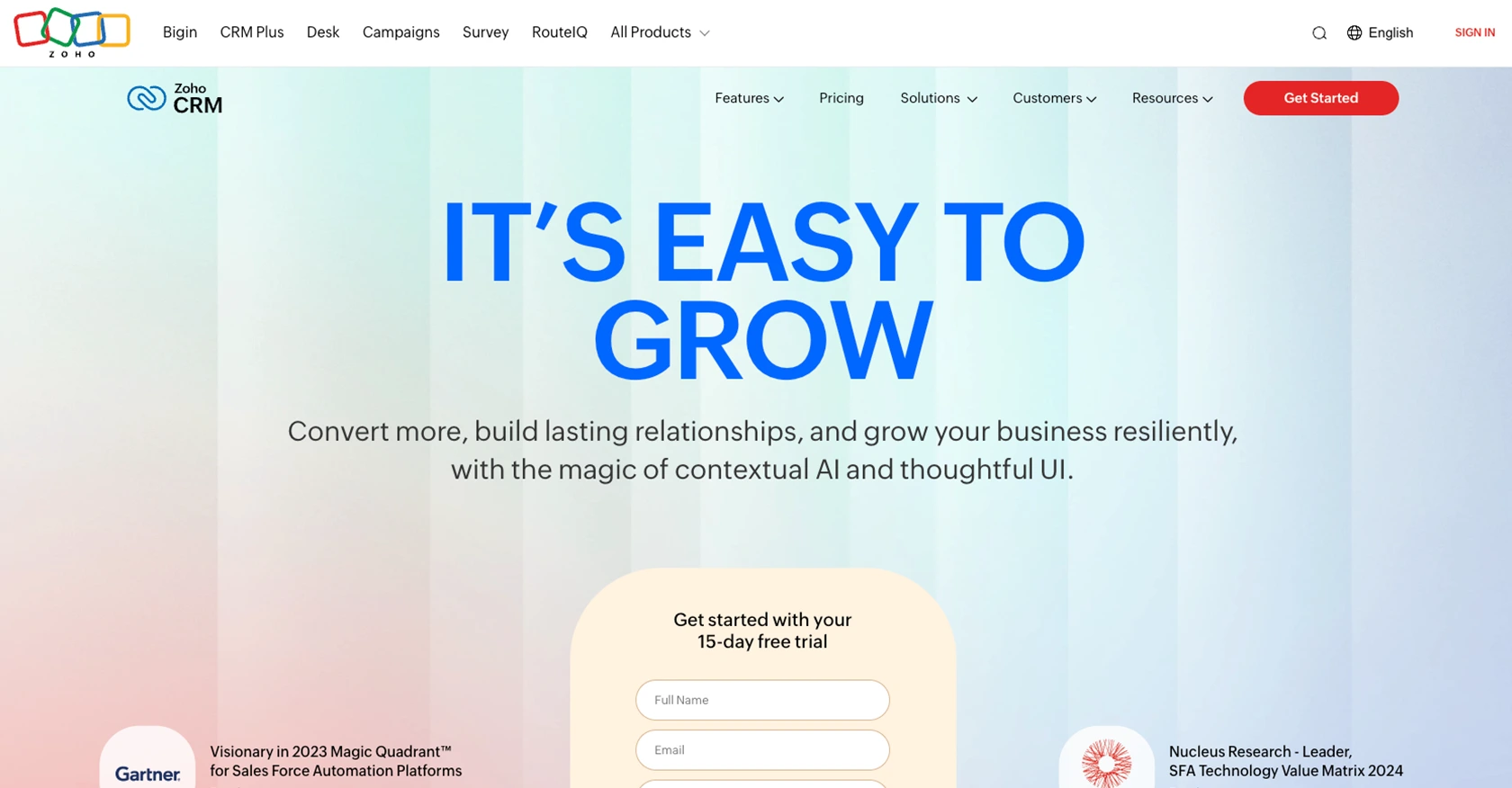
Introduction to Zoho CRM and Its API Integration
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a single system. Known for its flexibility and extensive features, Zoho CRM is a popular choice for organizations looking to enhance their customer engagement and streamline operations.
Developers often integrate with Zoho CRM to automate and optimize various business processes. By leveraging the Zoho CRM API, developers can access and manipulate data such as leads, contacts, and deals, enabling seamless data flow between Zoho CRM and other applications. For example, a developer might use the Zoho CRM API to automatically retrieve and analyze lead data, allowing sales teams to prioritize and engage with potential customers more effectively.
Setting Up Your Zoho CRM Test/Sandbox Account for API Integration
Before you can start interacting with the Zoho CRM API using JavaScript, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a Zoho CRM Developer Account
If you don't already have a Zoho CRM account, follow these steps to create one:
- Visit the Zoho CRM website and click on "Sign Up For Free."
- Fill in the required details such as your name, email, and password, then click "Sign Up."
- Verify your email address by clicking on the link sent to your inbox.
Accessing the Zoho Developer Console
Once your account is set up, you need to register your application in the Zoho Developer Console to obtain the necessary credentials for OAuth authentication:
- Go to the Zoho Developer Console and sign in with your Zoho CRM credentials.
- Select "Add Client" to register a new application.
- Choose "JavaScript" as the client type since you will be using JavaScript for API calls.
- Enter the required details such as Client Name, Homepage URL, and Authorized Redirect URIs.
- Click "Create" to generate your Client ID and Client Secret.
Configuring OAuth Scopes and Permissions
To interact with the Zoho CRM API, you need to define the appropriate scopes that determine the level of access your application will have:
- Navigate to the "Scopes" section in the Developer Console.
- Select the necessary scopes for accessing leads, such as
ZohoCRM.modules.leads.ALL
for full access. - Ensure that you save your changes to apply the selected scopes.
Generating Access and Refresh Tokens
With your application registered and scopes configured, you can now generate the tokens required for API authentication:
- Direct users to the Zoho authorization URL with the appropriate scopes and redirect URI.
- Upon successful authorization, Zoho will redirect to your specified URI with an authorization code.
- Exchange this authorization code for access and refresh tokens by making a POST request to Zoho's token endpoint.
Refer to the Zoho CRM OAuth Overview for detailed instructions on generating tokens.
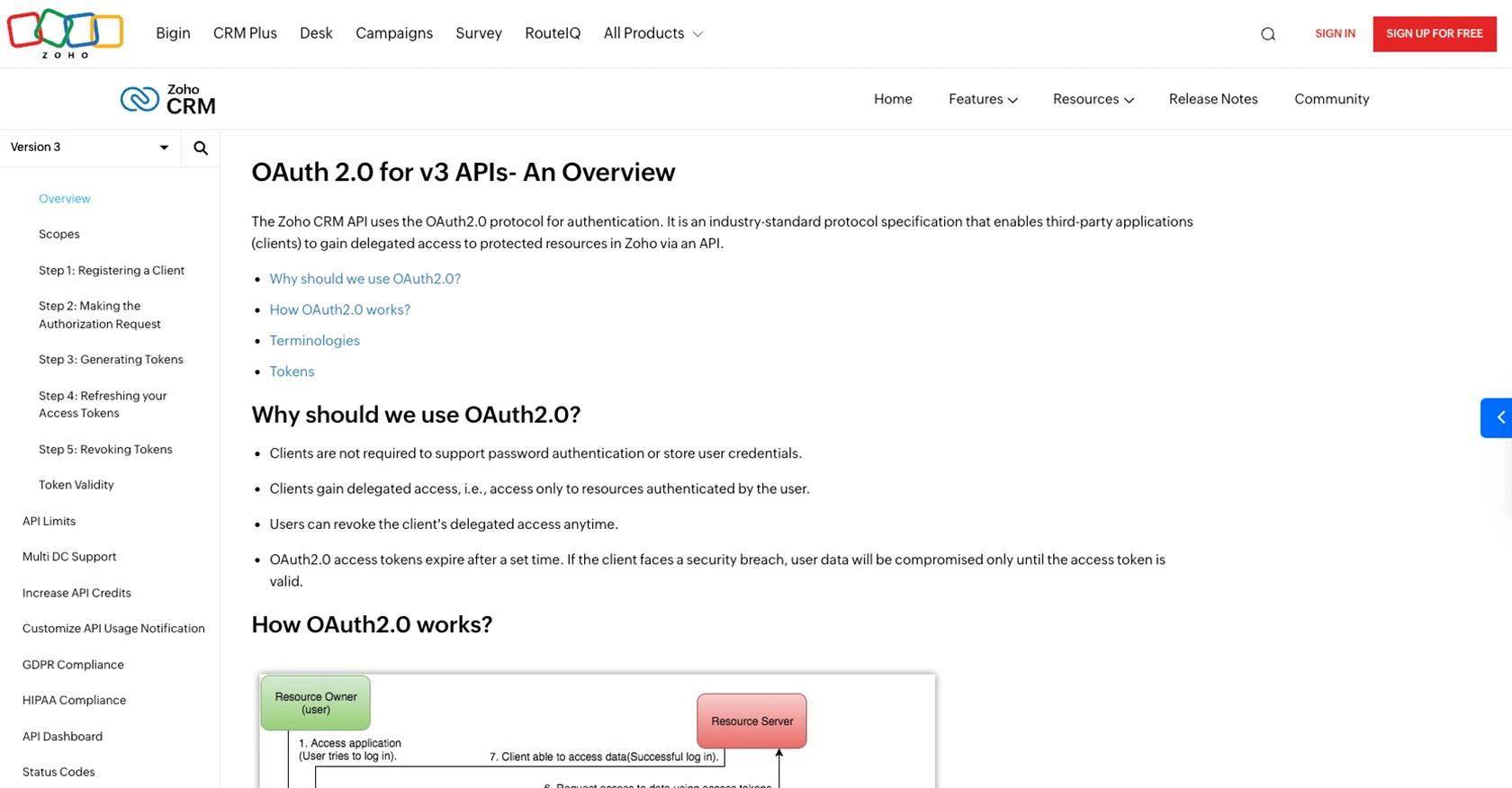
sbb-itb-96038d7
How to Make API Calls to Retrieve Leads from Zoho CRM Using JavaScript
Setting Up Your JavaScript Environment for Zoho CRM API Integration
To interact with the Zoho CRM API using JavaScript, ensure you have a suitable environment set up. You'll need Node.js installed on your machine to run JavaScript outside the browser. Additionally, you'll use the axios
library to make HTTP requests.
- Ensure Node.js is installed. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library by runningnpm install axios
.
Making a GET Request to Zoho CRM API to Fetch Leads
With your environment ready, you can now make a GET request to the Zoho CRM API to retrieve leads. The following example demonstrates how to fetch leads using the axios
library.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://www.zohoapis.com/crm/v3/Leads';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to fetch leads
async function fetchLeads() {
try {
const response = await axios.get(endpoint, { headers });
const leads = response.data.data;
console.log('Leads:', leads);
} catch (error) {
console.error('Error fetching leads:', error.response.data);
}
}
// Call the function to fetch leads
fetchLeads();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth authentication process.
Understanding the Response and Handling Errors
Upon a successful request, the API will return a JSON object containing the lead data. You can access this data through the response.data.data
property. If the request fails, the error handling block will log the error details, helping you diagnose issues such as invalid tokens or network problems.
Verifying API Call Success in Zoho CRM
To verify that your API call was successful, check the console output for the list of leads. Additionally, you can log into your Zoho CRM account and navigate to the Leads module to ensure the data matches the API response.
Handling Zoho CRM API Rate Limits
Zoho CRM imposes rate limits on API requests. If you exceed these limits, you may receive a 429 TOO_MANY_REQUESTS
error. To handle this, implement a retry mechanism with exponential backoff or consult the Zoho CRM API Limits documentation for more details.
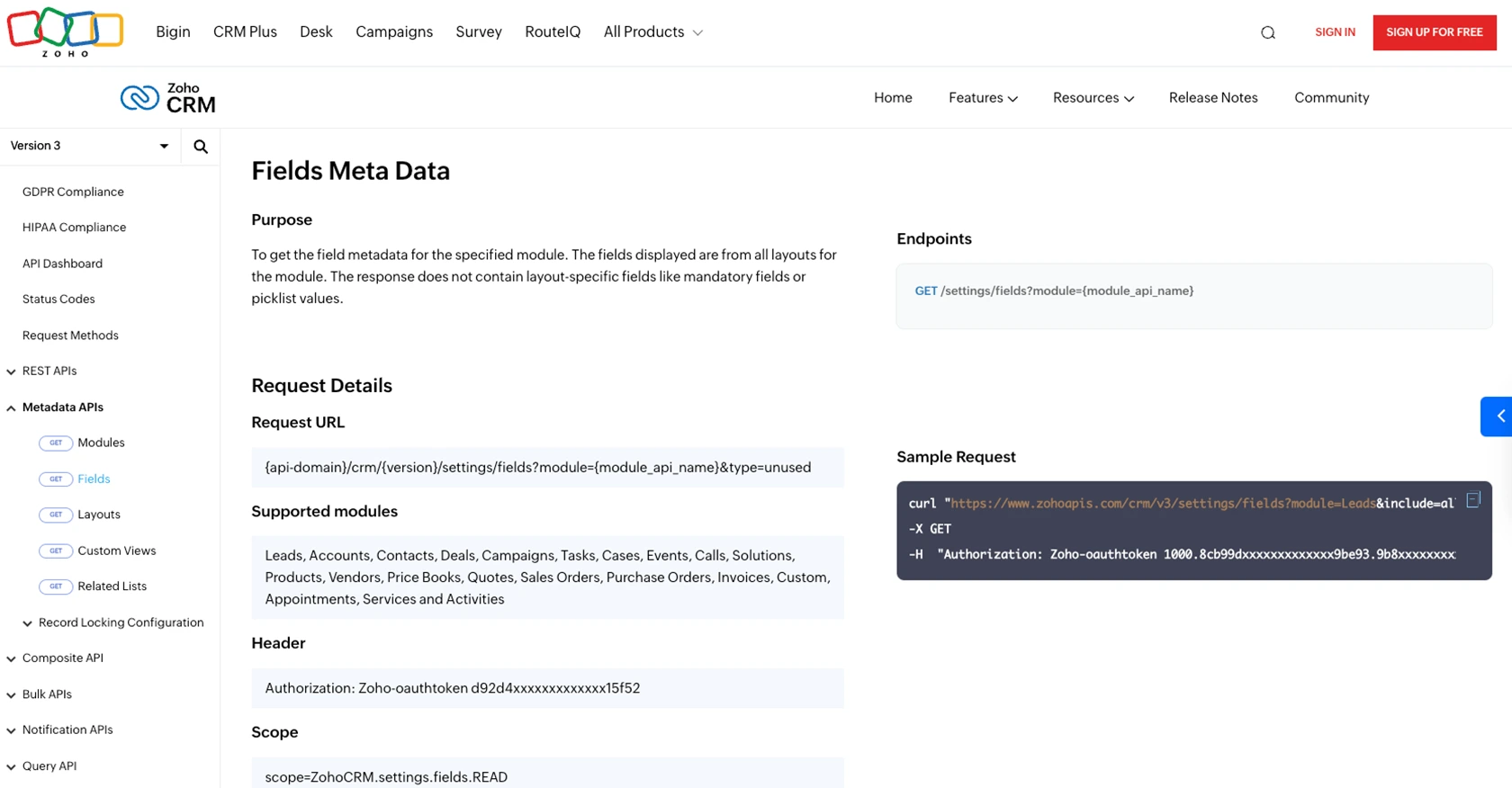
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using JavaScript provides a powerful way to automate and enhance your business processes. By following the steps outlined in this guide, you can efficiently retrieve lead data and integrate it with your existing systems, improving your sales team's ability to engage with potential customers.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Securely Store Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Zoho CRM's API rate limits. Implement retry mechanisms with exponential backoff to gracefully handle
429 TOO_MANY_REQUESTS
errors. Refer to the API Limits documentation for more information. - Data Standardization: Ensure that data retrieved from Zoho CRM is standardized and transformed as needed to fit your application's requirements.
- Regularly Refresh Tokens: Since access tokens have a limited lifespan, implement a mechanism to refresh them using the refresh token to maintain uninterrupted API access.
Enhancing Your Integration Strategy with Endgrate
For developers looking to streamline their integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate offers a unified API endpoint that simplifies interactions with multiple platforms, including Zoho CRM, providing an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can optimize your integration strategy and enhance your application's capabilities.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?