Using the Constant Contact API to Get Contacts (with Python examples)
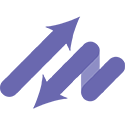
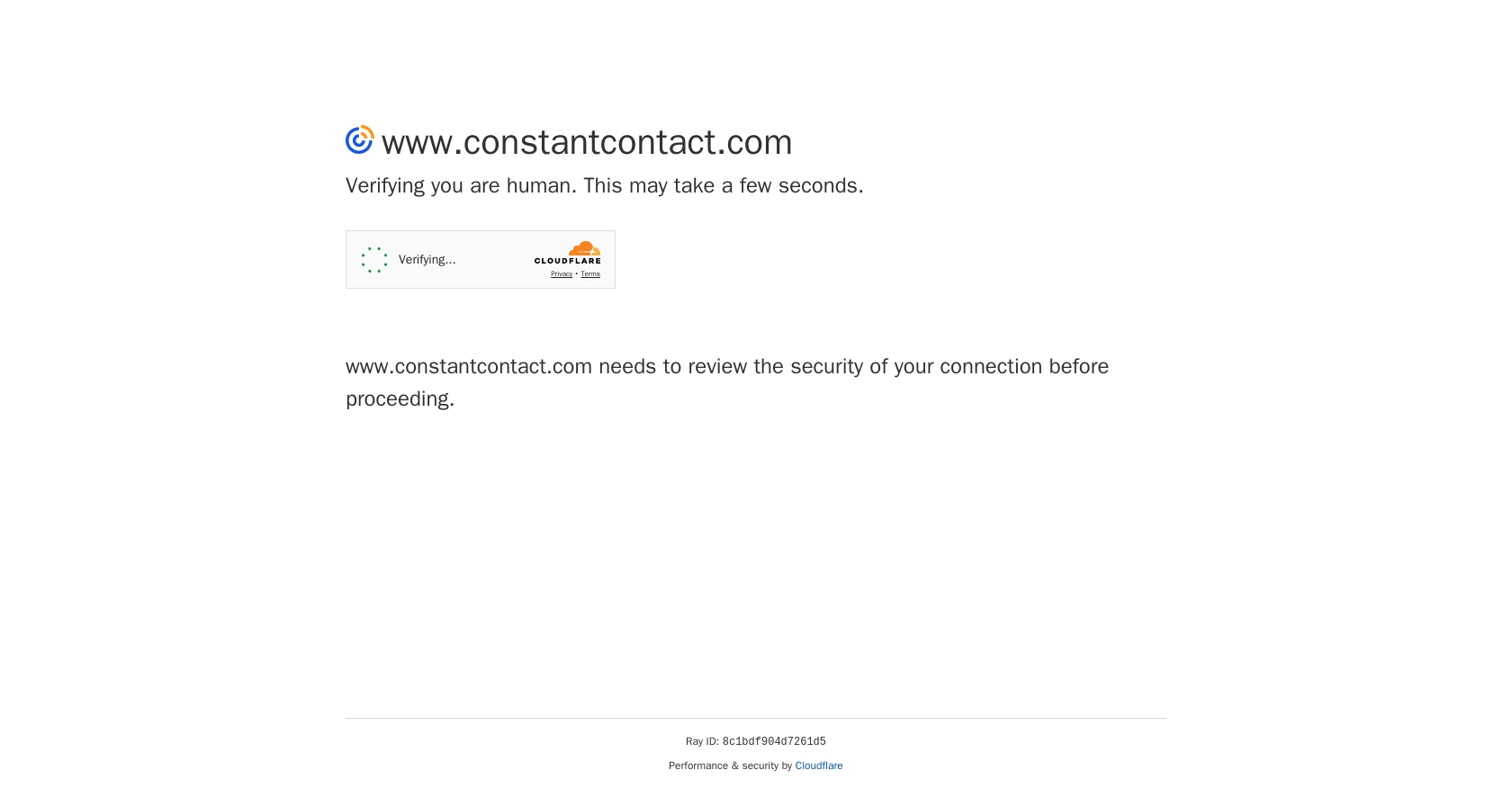
Introduction to Constant Contact API
Constant Contact is a powerful email marketing platform that enables businesses to create and manage effective email campaigns. With its robust suite of tools, Constant Contact helps organizations engage with their audience, track campaign performance, and drive business growth.
Developers may want to integrate with Constant Contact's API to streamline the management of contact data and automate marketing processes. For example, using the Constant Contact API, a developer can retrieve contact information to personalize email campaigns or synchronize contact lists with other CRM systems.
This article will guide you through using Python to interact with the Constant Contact API, specifically focusing on retrieving contact data. By following this tutorial, you'll learn how to efficiently access and manage your contact information on the Constant Contact platform.
Setting Up Your Constant Contact Developer Account
Before you can start using the Constant Contact API, you need to set up a developer account and create an application to obtain the necessary credentials for authentication. Follow these steps to get started:
Create a Constant Contact Developer Account
- Visit the Constant Contact Developer Portal and sign up for a developer account.
- Once registered, log in to your developer account to access the dashboard.
Create a New Application for OAuth2 Authentication
Constant Contact uses OAuth2 for authentication, which requires you to create an application to obtain a client ID and client secret. Follow these steps:
- Navigate to the "My Applications" tab in the developer portal.
- Click on "Create a New Application."
- Fill in the required details, such as the application name and description.
- Specify the redirect URI, which is the URL to which users will be redirected after they authorize your application. Ensure this matches the redirect URI you will use in your code.
- Save the application to generate your client ID and client secret.
Configure OAuth2 Scopes
When creating your application, you need to specify the scopes that define the level of access your application requires. For retrieving contacts, ensure you include the contact_data
scope. This grants permission to read or write contact data.
Generate Access Tokens
With your client ID and client secret, you can now generate access tokens to authenticate API requests. Follow these steps:
- Direct users to the Constant Contact authorization endpoint to obtain an authorization code:
- Exchange the authorization code for an access token by sending a POST request to the token endpoint:
- Store the access token securely, as it will be used to authenticate your API requests.
GET https://authz.constantcontact.com/oauth2/default/v1/authorize?client_id={your_client_id}&redirect_uri={your_redirect_uri}&response_type=code&scope=contact_data
POST https://authz.constantcontact.com/oauth2/default/v1/token
Headers:
Content-Type: application/x-www-form-urlencoded
Body:
client_id={your_client_id}
client_secret={your_client_secret}
code={authorization_code}
redirect_uri={your_redirect_uri}
grant_type=authorization_code
For more details on OAuth2 authentication with Constant Contact, refer to the official authentication documentation.
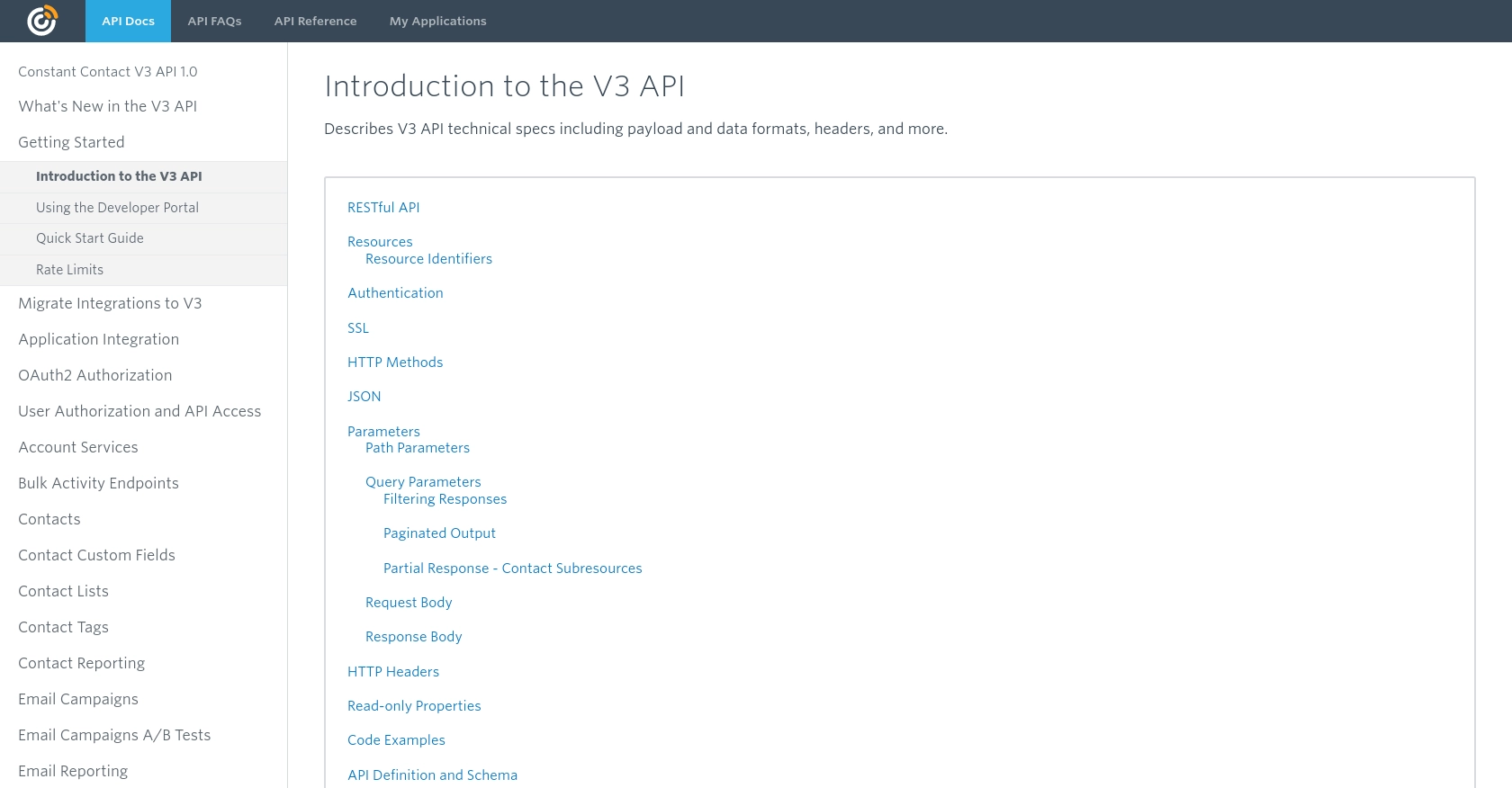
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Constant Contact Using Python
To interact with the Constant Contact API and retrieve contact data, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Constant Contact API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Retrieve Contacts from Constant Contact
Create a new Python file named get_constant_contact_contacts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.cc.email/v3/contacts"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the contacts and print their information
for contact in data.get("contacts", []):
print(contact)
else:
print(f"Failed to retrieve contacts: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token you obtained during the OAuth2 authentication process.
Running the Python Script to Fetch Contacts
Execute the script from your terminal or command line:
python get_constant_contact_contacts.py
If successful, the script will print out the contact information retrieved from Constant Contact.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The Constant Contact API uses standard HTTP response codes to indicate success or failure:
- 200 OK: The request was successful, and the contacts were retrieved.
- 401 Unauthorized: The access token is invalid or expired. Ensure you have a valid token.
- 403 Forbidden: The request lacks the necessary permissions. Verify your scopes.
- 429 Too Many Requests: You have exceeded the rate limit. Wait before retrying.
For more details on error codes, refer to the Constant Contact API documentation.
By following these steps, you can efficiently retrieve contact data from Constant Contact using Python, enabling you to automate and streamline your marketing processes.
Conclusion and Best Practices for Using Constant Contact API with Python
Integrating with the Constant Contact API using Python provides a powerful way to automate and enhance your marketing processes. By retrieving contact data programmatically, you can personalize email campaigns, synchronize contact lists, and streamline your marketing efforts.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Constant Contact enforces rate limits to ensure fair usage. Be mindful of the limit of 10,000 requests per day and 4 requests per second. Implement retry logic with exponential backoff to handle
429 Too Many Requests
errors gracefully. - Refresh Tokens Appropriately: Use refresh tokens to extend the lifespan of access tokens without requiring user re-authentication. Only request a new access token when the current one is expired or about to expire.
- Transform and Standardize Data: Ensure that the data retrieved from Constant Contact is transformed and standardized to fit your application's needs. This may involve mapping fields or converting data formats.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Constant Contact. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
By following these best practices and leveraging tools like Endgrate, you can ensure a robust and efficient integration with Constant Contact, empowering your marketing efforts and driving business growth.
Read More
- https://endgrate.com/provider/constantcontact
- https://developer.constantcontact.com/api_guide/v3_technical_overview.html
- https://developer.constantcontact.com/api_guide/auth_overview.html
- https://developer.constantcontact.com/api_guide/scopes.html
- https://developer.constantcontact.com/api_guide/glossary_responses.html
Ready to get started?