How to Get Leads with the Close API in Python
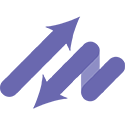
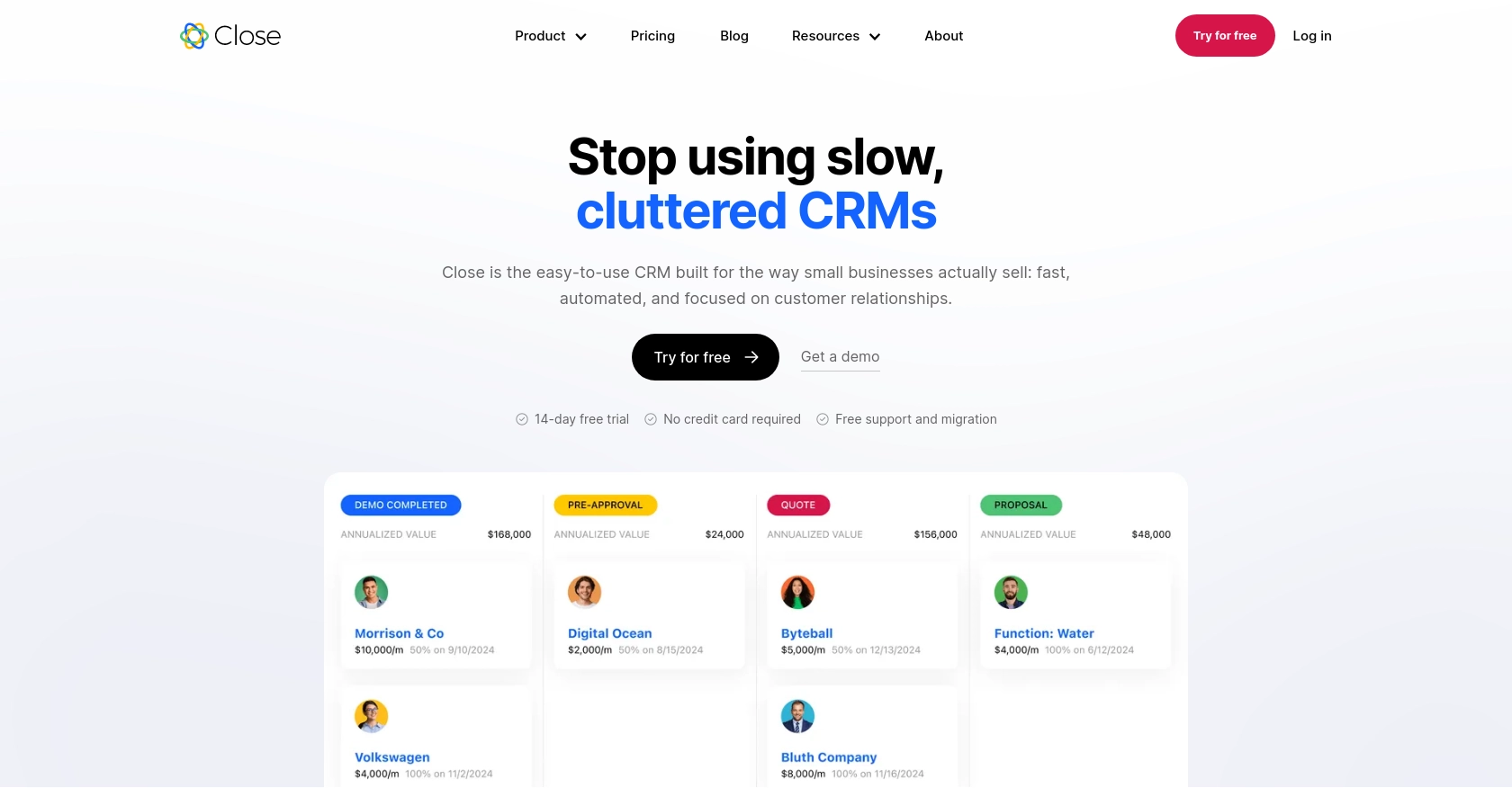
Introduction to Close CRM
Close is a powerful CRM platform designed to enhance sales productivity and streamline communication for businesses. It offers a comprehensive suite of tools that help sales teams manage leads, track opportunities, and close deals more efficiently.
Integrating with Close's API allows developers to automate and optimize sales processes, such as retrieving and managing leads. For example, a developer might use the Close API to automatically fetch new leads into a custom dashboard, enabling sales teams to quickly access and act on potential opportunities.
Setting Up Your Close CRM API Test Account
Before you can start integrating with the Close API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Create a Close CRM Account
If you don't already have a Close account, you can sign up for a free trial on the Close website. This will give you access to the platform's features and allow you to generate an API key for testing purposes.
- Visit the Close website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Close dashboard.
Generate an API Key for Close CRM
Close uses API keys for authentication, which are ideal for internal scripts and integrations. Here's how to generate an API key:
- Navigate to the "Settings" page in your Close dashboard.
- Under the "API Keys" section, click on "Generate API Key."
- Copy the generated API key and store it securely, as you'll need it for making API calls.
For more information on API key authentication, refer to the Close API documentation.
Understanding Close API Authentication
Close API keys use HTTP Basic authentication. When making API requests, include an Authorization header with the word "Basic" followed by a space and a base64-encoded string of your API key followed by a colon. The API key acts as the username, and the password is left blank.
import base64
api_key = 'yourapikey:'
encoded_key = base64.b64encode(api_key.encode()).decode()
headers = {'Authorization': f'Basic {encoded_key}'}
For further details, see the Close API authentication guide.
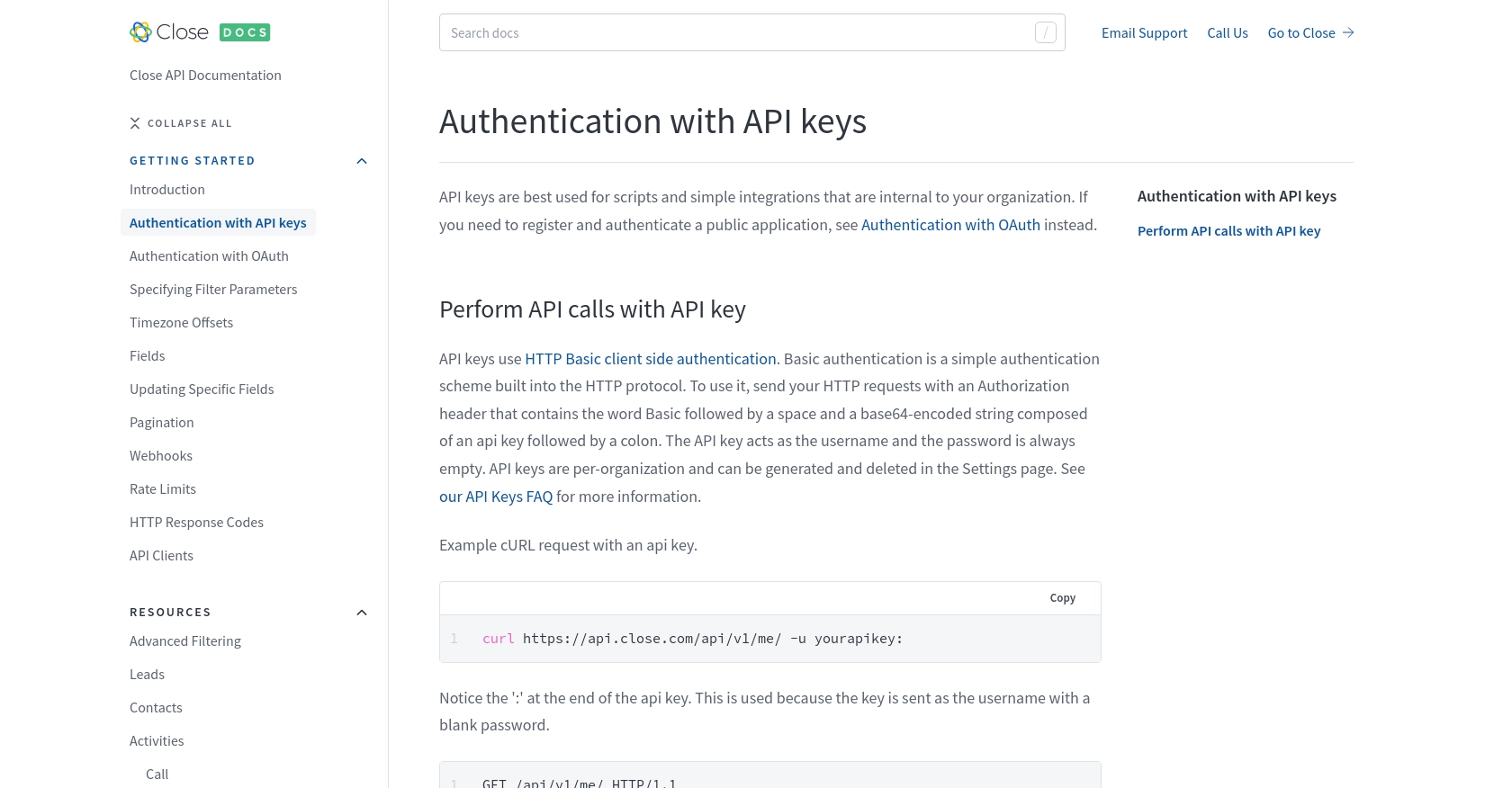
sbb-itb-96038d7
Making API Calls to Retrieve Leads from Close CRM Using Python
Now that you have your Close CRM account and API key set up, it's time to make API calls to retrieve leads using Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Close API Integration
To interact with the Close API, you'll need Python installed on your machine. This tutorial uses Python 3. Make sure you have the following prerequisites:
- Python 3.x
- pip, the Python package installer
You'll also need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Fetch Leads from Close CRM
Create a new Python file named get_close_leads.py
and add the following code:
import requests
import base64
# Your Close API key
api_key = 'yourapikey:'
encoded_key = base64.b64encode(api_key.encode()).decode()
# Set the API endpoint and headers
endpoint = "https://api.close.com/api/v1/lead/"
headers = {
'Authorization': f'Basic {encoded_key}',
'Content-Type': 'application/json'
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
leads = response.json()['data']
for lead in leads:
print(f"Lead Name: {lead['name']}")
else:
print(f"Failed to retrieve leads: {response.status_code} - {response.text}")
Replace yourapikey
with the API key you generated earlier. This script makes a GET request to the Close API to fetch leads and prints their names.
Handling API Response and Errors
After running the script, you should see a list of lead names printed in your terminal. If the request fails, the script will output the error code and message. Here are some common HTTP response codes you might encounter:
- 200: Request was successful.
- 400: There was an issue with the request.
- 401: Authentication failed. Check your API key.
- 429: Too many requests. You need to wait before retrying.
For more information on handling errors, refer to the Close API HTTP response codes documentation.
Verifying Retrieved Leads in Close CRM
To verify that the leads retrieved match those in your Close CRM account, log in to your Close dashboard and navigate to the Leads section. You should see the same leads listed there.
For more detailed information on the leads endpoint, visit the Close API leads documentation.
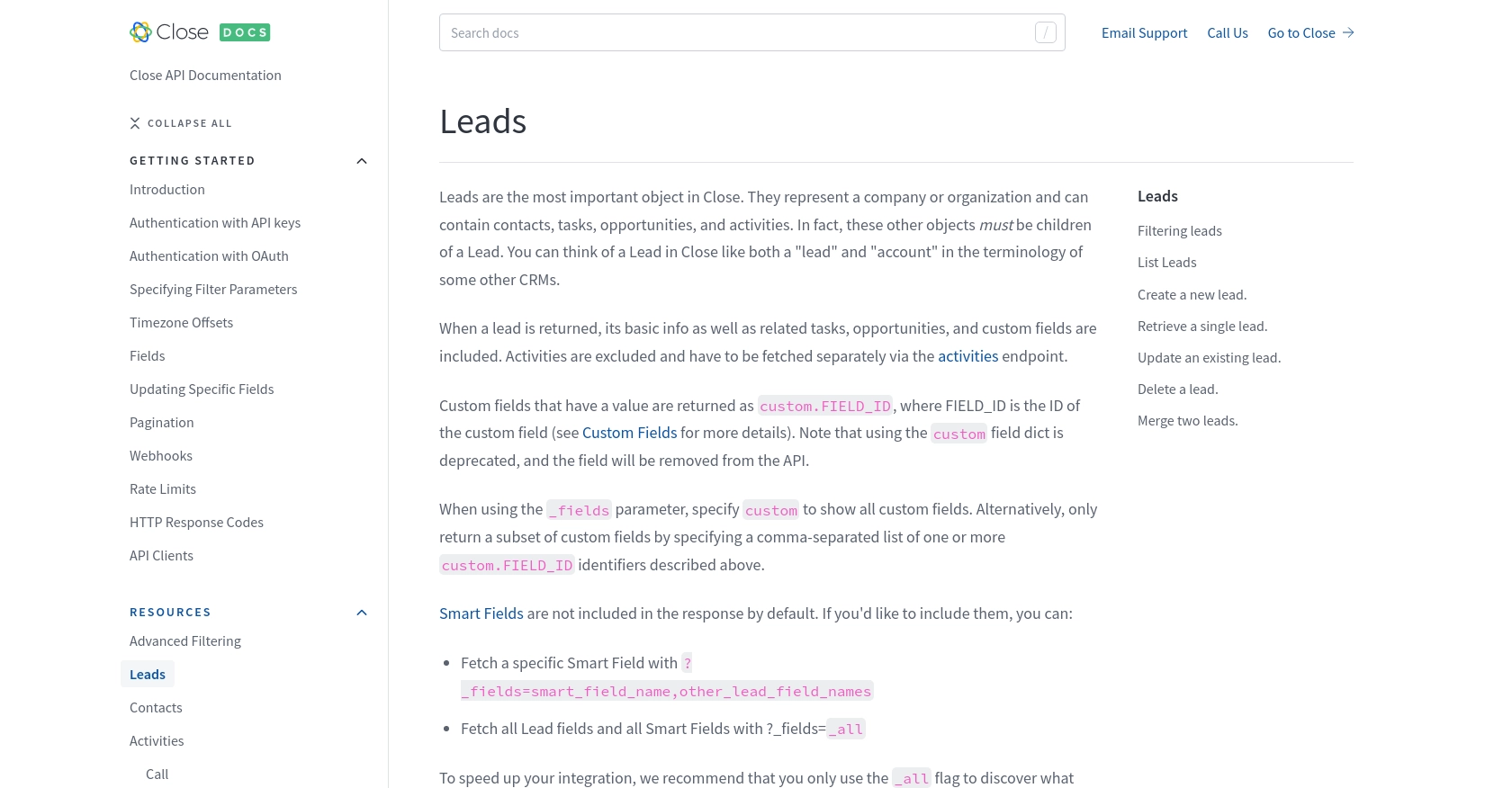
Conclusion and Best Practices for Using Close API with Python
Integrating with the Close API using Python can significantly enhance your sales processes by automating lead management and providing real-time access to crucial data. By following the steps outlined in this guide, you can efficiently retrieve leads and integrate them into your custom applications or dashboards.
Best Practices for Secure and Efficient Close API Integration
- Secure API Key Storage: Always store your API keys securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Close's rate limits. If you receive a 429 status code, pause your requests as specified by the
rate_reset
value. For more details, refer to the Close API rate limits documentation. - Data Standardization: Ensure that data retrieved from Close is standardized to fit your application's requirements. This might involve transforming field names or formats.
Enhance Your Integration Experience with Endgrate
While integrating with Close API directly is powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Close. This allows you to focus on your core product while outsourcing integration complexities.
Explore how Endgrate can simplify your integration processes by visiting Endgrate and discover how you can save time and resources while delivering a seamless experience for your users.
Read More
- https://endgrate.com/provider/close
- https://developer.close.com/topics/authentication/
- https://developer.close.com/topics/authentication-oauth2/
- https://developer.close.com/topics/pagination/
- https://developer.close.com/topics/rate-limits/
- https://developer.close.com/topics/http-response-codes/
- https://developer.close.com/resources/leads/
Ready to get started?