How to Get Issues with the Linear API in Python
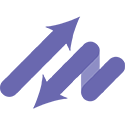
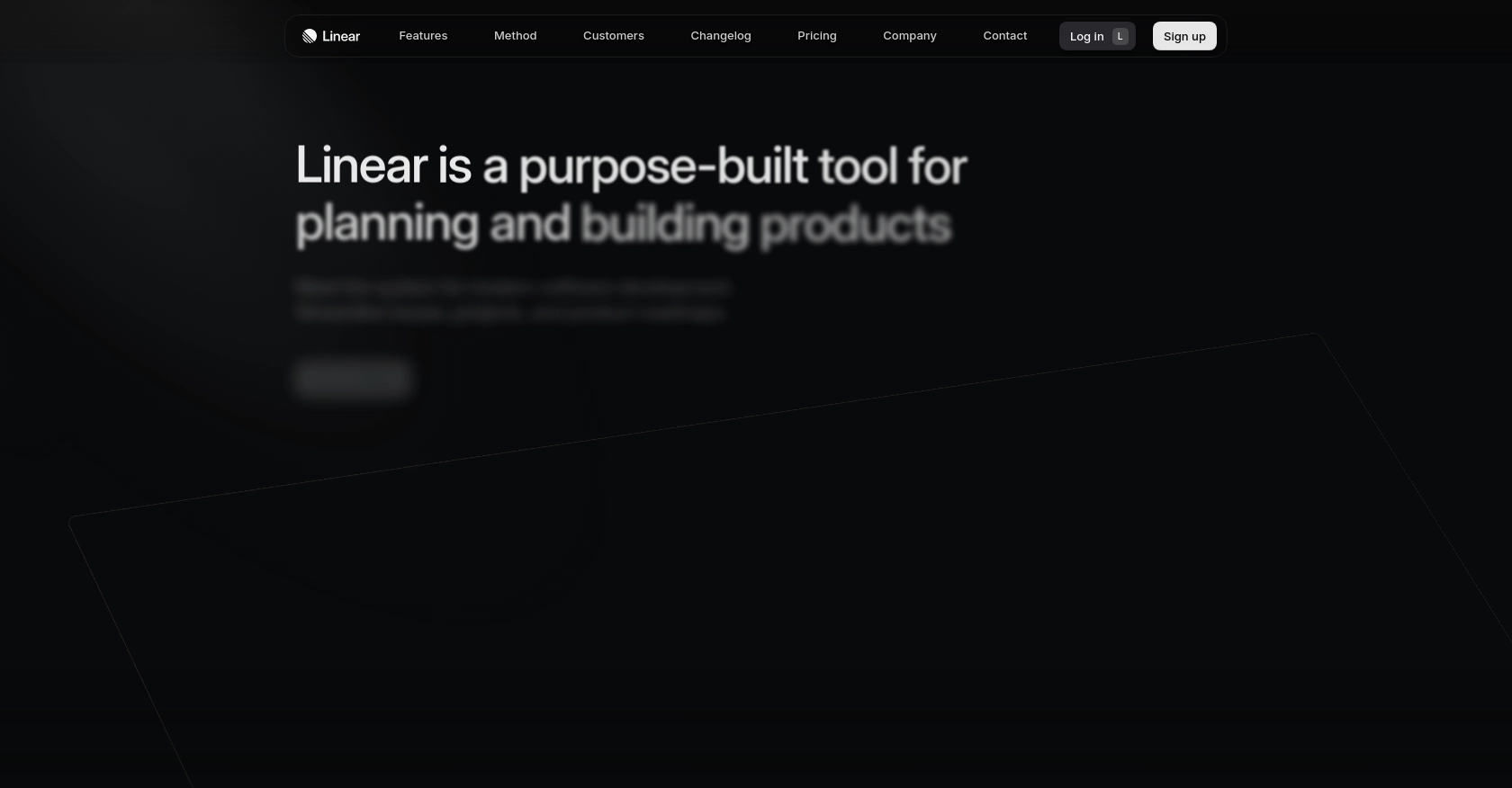
Introduction to Linear API Integration
Linear is a modern issue tracking tool designed to streamline project management and enhance team productivity. With its intuitive interface and powerful features, Linear helps development teams efficiently manage tasks, track progress, and collaborate seamlessly.
Integrating with Linear's API allows developers to automate and optimize their workflow by accessing and managing issues programmatically. For example, you might want to retrieve a list of issues assigned to a specific team member to generate custom reports or trigger notifications when new issues are created.
This article will guide you through the process of using Python to interact with the Linear API, focusing on retrieving issues efficiently. By the end of this tutorial, you'll be equipped to leverage Linear's capabilities to enhance your development projects.
Setting Up Your Linear Test Account and OAuth Application
Before you can start interacting with the Linear API using Python, you'll need to set up a test account and configure an OAuth application. This will allow you to authenticate your API requests securely and access the necessary data.
Creating a Linear Account
If you don't already have a Linear account, you can sign up for a free trial on the Linear website. Follow the instructions to create your account and log in. If you already have an account, simply log in to proceed.
Setting Up an OAuth2 Application in Linear
Linear supports OAuth2 authentication, which is recommended for building applications that integrate with Linear. Follow these steps to create an OAuth2 application:
- Navigate to the Linear application settings.
- Under the integrations section, select "OAuth2 Applications" and click on "Create New Application."
- Fill in the required details, including the application name and description.
- Configure the redirect callback URLs to your application. This is crucial for handling authorization responses.
Authorizing User Access to Linear API
To authorize user access, you'll need to redirect users to Linear's authorization URL with the appropriate parameters:
GET https://linear.app/oauth/authorize?response_type=code&client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URL&scope=read,write
Replace YOUR_CLIENT_ID
and YOUR_REDIRECT_URL
with your application's client ID and redirect URI.
Exchanging Authorization Code for Access Token
Once the user authorizes your application, they will be redirected back to your application with an authorization code. Use this code to request an access token:
POST https://api.linear.app/oauth/token
Content-Type: application/x-www-form-urlencoded
Parameters:
- code: Authorization code from the previous step
- redirect_uri: Same redirect URI used previously
- client_id: Your application's client ID
- client_secret: Your application's client secret
- grant_type: authorization_code
Upon successful request, you'll receive an access token, which you can use to authenticate your API calls.
For more detailed information on setting up OAuth2 authentication with Linear, refer to the official Linear OAuth documentation.
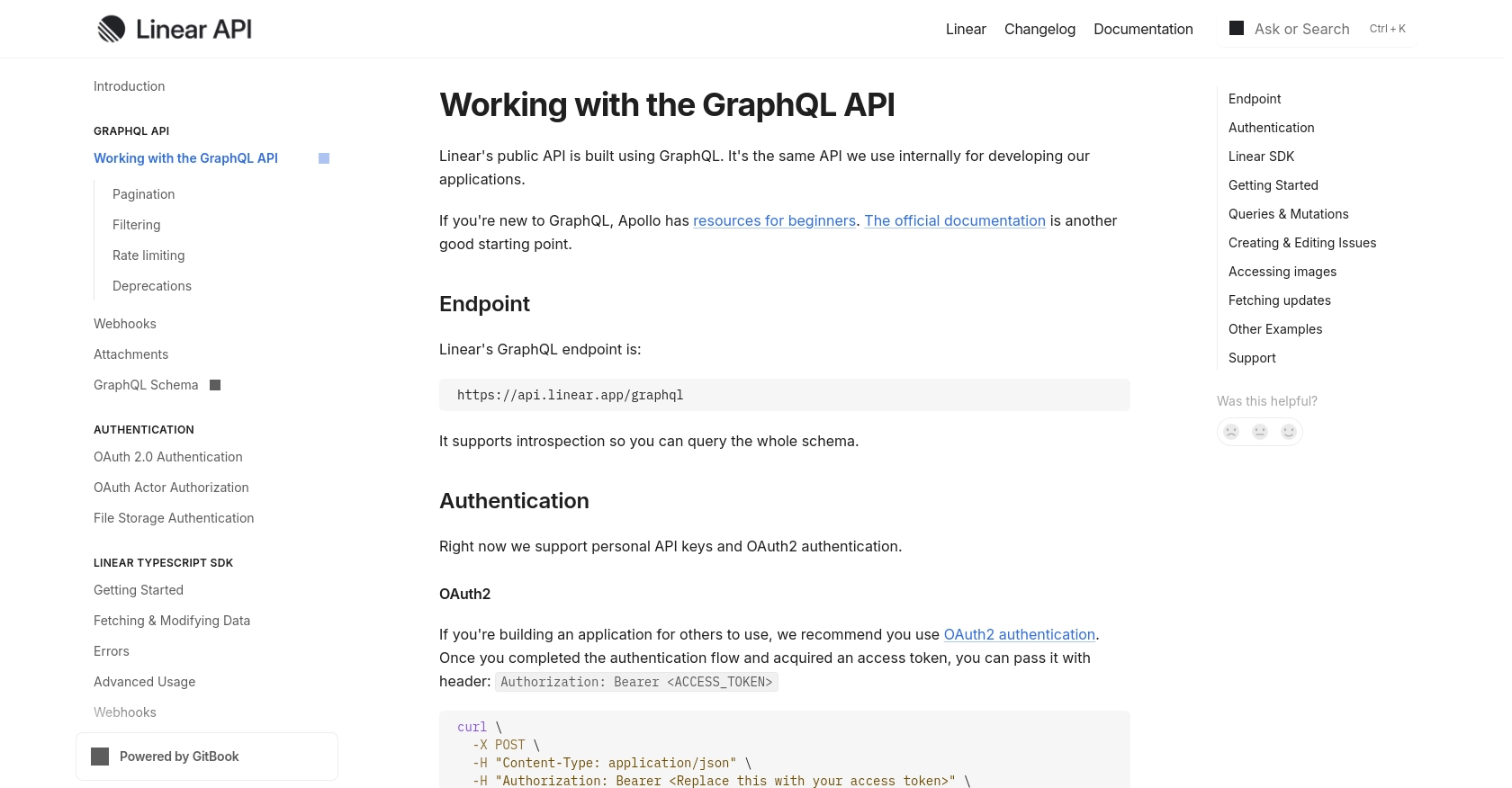
sbb-itb-96038d7
Making API Calls to Retrieve Issues from Linear API Using Python
To interact with the Linear API and retrieve issues, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the necessary steps to set up your Python environment, make API calls, and handle responses effectively.
Setting Up Your Python Environment for Linear API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing Python Code to Fetch Issues from Linear API
With your environment set up, you can now write a Python script to fetch issues from Linear using the GraphQL API. Create a file named get_linear_issues.py
and add the following code:
import requests
# Set the GraphQL endpoint and headers
url = "https://api.linear.app/graphql"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
# Define the GraphQL query to fetch issues
query = """
{
issues {
nodes {
id
title
description
createdAt
}
}
}
"""
# Make a POST request to the Linear API
response = requests.post(url, json={"query": query}, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for issue in data["data"]["issues"]["nodes"]:
print(f"Issue ID: {issue['id']}, Title: {issue['title']}")
else:
print(f"Failed to fetch issues: {response.status_code}")
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup.
Running the Python Script and Verifying Results
Execute the script from your terminal or command line using the following command:
python get_linear_issues.py
If successful, the script will output a list of issue IDs and titles from your Linear account. Verify the results by cross-checking with the issues in your Linear dashboard.
Handling Errors and Understanding Linear API Response Codes
It's crucial to handle potential errors when making API calls. The Linear API may return various HTTP status codes indicating the success or failure of your request:
- 200 OK: The request was successful, and the issues were retrieved.
- 401 Unauthorized: Authentication failed. Check your access token.
- 400 Bad Request: The request was malformed. Verify your query syntax.
For more detailed error handling, refer to the Linear API documentation.
Conclusion and Best Practices for Using Linear API with Python
Integrating with the Linear API using Python can significantly enhance your team's productivity by automating issue tracking and management. By following the steps outlined in this article, you can efficiently retrieve and manage issues, allowing for seamless project management and collaboration.
Best Practices for Secure and Efficient Linear API Integration
- Secure Storage of Access Tokens: Always store your OAuth access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Be mindful of Linear's rate limits to avoid disruptions. Implement exponential backoff strategies for handling rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from Linear is standardized and transformed as needed to fit your application's requirements.
Enhancing Your Integration Strategy with Endgrate
While integrating with Linear's API can be powerful, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Linear. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration strategy by visiting Endgrate.
Read More
Ready to get started?