Using the Zendesk Sell API to Create or Update Leads (with Python examples)
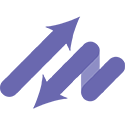
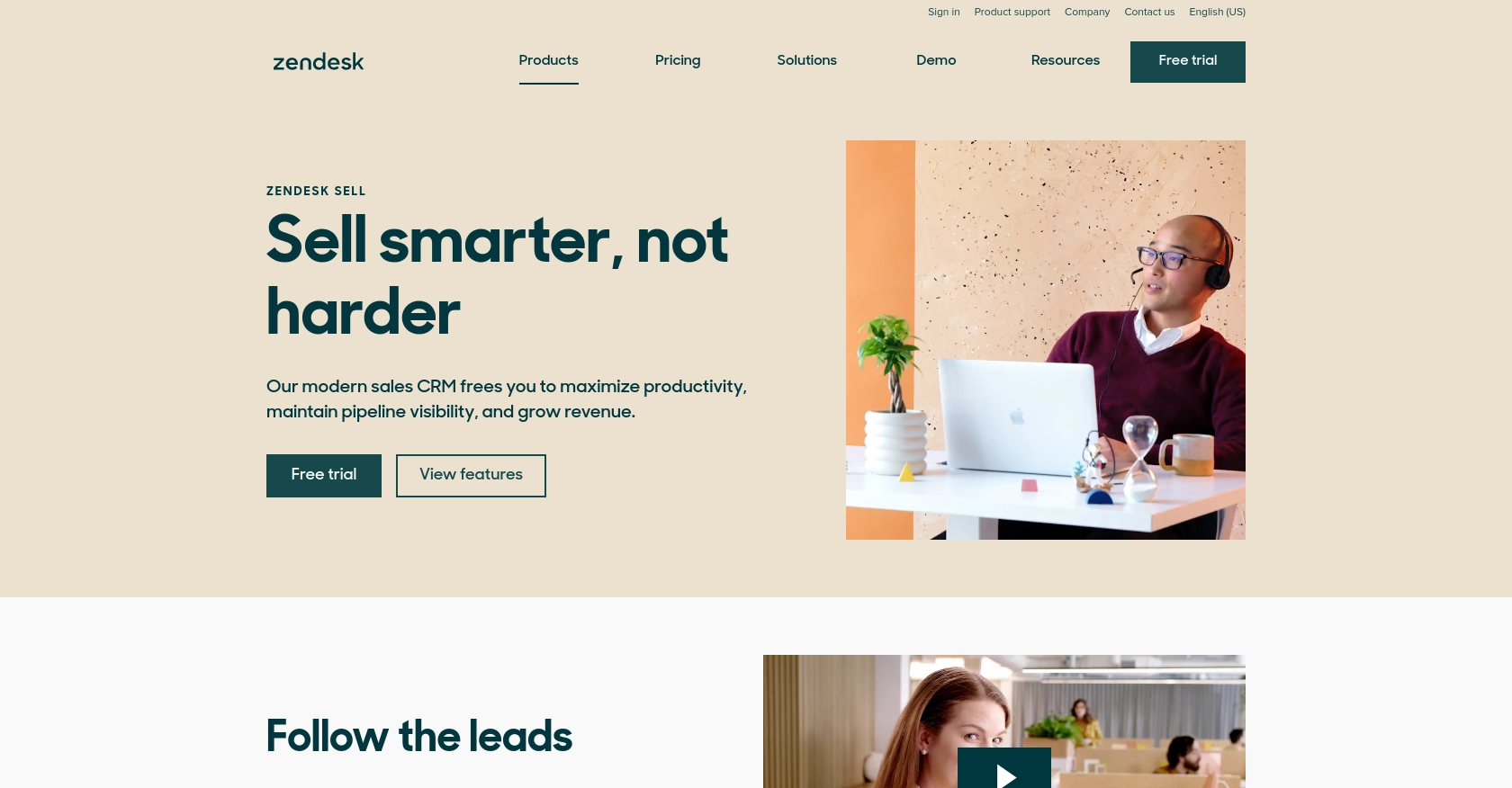
Introduction to Zendesk Sell API
Zendesk Sell is a powerful sales CRM platform designed to enhance productivity and streamline sales processes for businesses. It offers a comprehensive suite of tools to manage leads, contacts, and deals, providing sales teams with the insights they need to close more deals efficiently.
Integrating with the Zendesk Sell API allows developers to automate and optimize sales workflows. For example, by using the API, developers can create or update leads programmatically, ensuring that sales teams have the most up-to-date information at their fingertips. This can be particularly useful for syncing data from other systems or automating lead generation processes.
Setting Up Your Zendesk Sell Test Account
Before you can start integrating with the Zendesk Sell API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Zendesk Sell Account
If you don't already have a Zendesk Sell account, you can sign up for a free trial on the Zendesk Sell website. Follow the instructions to create your account. Once your account is set up, log in to access the dashboard.
Setting Up OAuth Authentication for Zendesk Sell API
The Zendesk Sell API uses OAuth 2.0 for authentication, which provides a secure way to access the API. Follow these steps to set up OAuth authentication:
- Register Your Application: Navigate to the Zendesk Sell developer portal and register your application. You'll receive a unique
Client ID
andClient Secret
. - Configure OAuth Settings: In your application settings, set the
Redirect URI
to a valid URL where you want to receive the authorization code. - Request Authorization: Direct users to the following URL to authorize your application:
https://api.getbase.com/oauth2/authorize?client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
- Exchange Authorization Code for Access Token: Use the authorization code to request an access token:
import requests url = "https://api.getbase.com/oauth2/token" payload = { 'grant_type': 'authorization_code', 'code': 'YOUR_AUTHORIZATION_CODE', 'redirect_uri': 'YOUR_REDIRECT_URI', 'client_id': 'YOUR_CLIENT_ID', 'client_secret': 'YOUR_CLIENT_SECRET' } response = requests.post(url, data=payload) access_token = response.json().get('access_token')
Ensure you securely store the access_token
as it will be used to authenticate your API requests.
Testing Your Zendesk Sell API Integration
With your access token in hand, you can now make API calls to the Zendesk Sell sandbox environment. This allows you to test creating or updating leads without impacting your live data. Use the following endpoint for testing:
https://api.getbase.com/v2/leads
By following these steps, you'll be ready to start integrating with the Zendesk Sell API and automating your sales workflows.
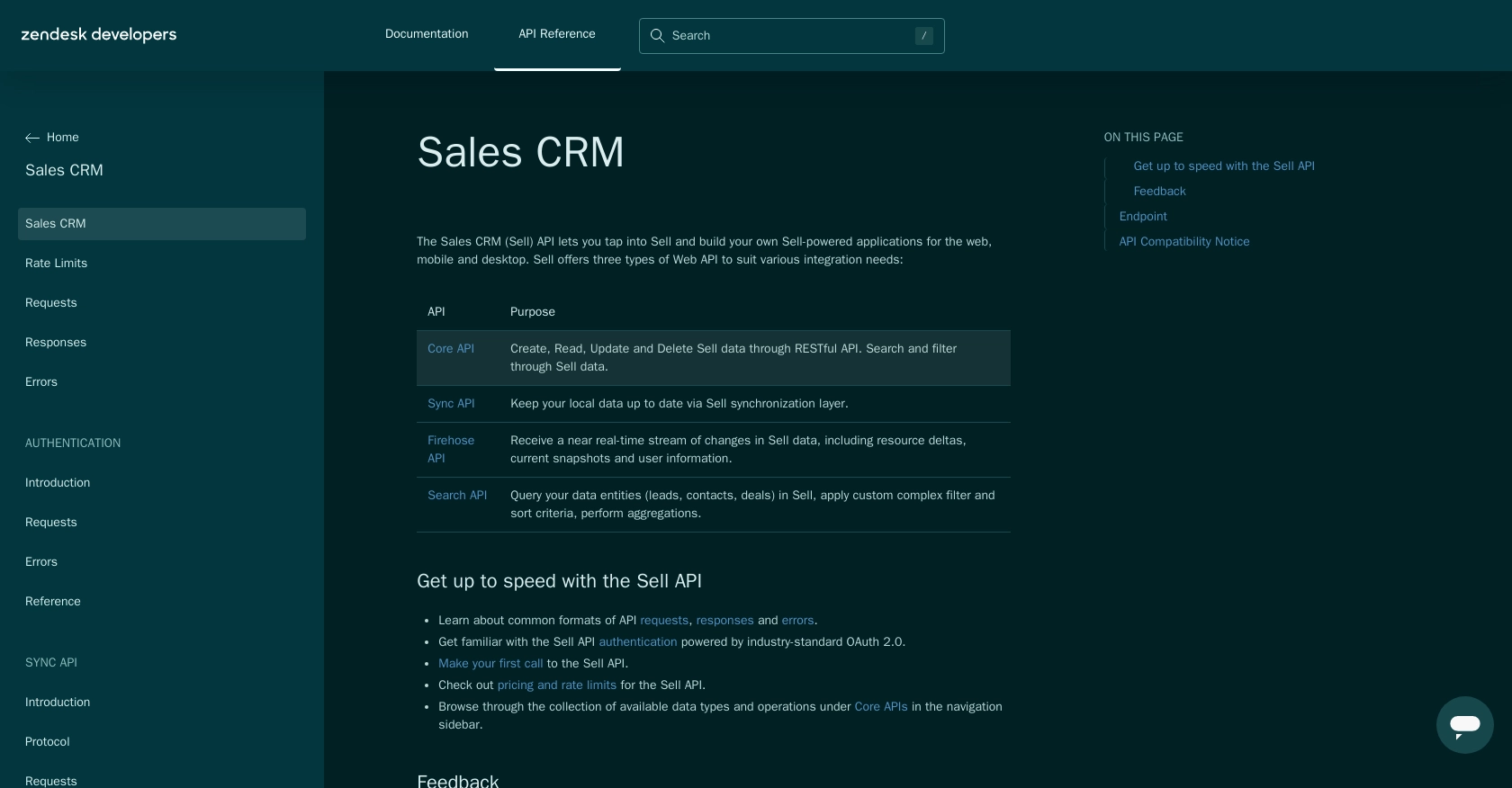
sbb-itb-96038d7
Making API Calls to Zendesk Sell for Lead Management
To interact with the Zendesk Sell API using Python, you'll need to ensure your environment is set up correctly. This section will guide you through making API calls to create or update leads, providing you with practical examples and expected outcomes.
Setting Up Your Python Environment for Zendesk Sell API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Creating a Lead with Zendesk Sell API Using Python
To create a lead in Zendesk Sell, you'll need to make a POST request to the API endpoint. Below is a sample code snippet to create a lead:
import requests url = "https://api.getbase.com/v2/leads" headers = { "Authorization": "Bearer YOUR_ACCESS_TOKEN", "Content-Type": "application/json" } data = { "data": { "first_name": "John", "last_name": "Doe", "email": "john.doe@example.com", "organization_name": "Example Corp" } } response = requests.post(url, json=data, headers=headers) if response.status_code == 201: print("Lead created successfully:", response.json()) else: print("Failed to create lead:", response.status_code, response.json())
Replace YOUR_ACCESS_TOKEN
with the access token you obtained earlier. This code will create a new lead with the specified details. If successful, the response will include the lead's information.
Updating a Lead with Zendesk Sell API Using Python
To update an existing lead, use the PUT method. Here's an example of how to update a lead's information:
import requests lead_id = "LEAD_ID" url = f"https://api.getbase.com/v2/leads/{lead_id}" headers = { "Authorization": "Bearer YOUR_ACCESS_TOKEN", "Content-Type": "application/json" } data = { "data": { "last_name": "Smith", "email": "john.smith@example.com" } } response = requests.put(url, json=data, headers=headers) if response.status_code == 200: print("Lead updated successfully:", response.json()) else: print("Failed to update lead:", response.status_code, response.json())
Replace LEAD_ID
with the ID of the lead you wish to update. This code modifies the lead's last name and email address. Verify the update by checking the response or reviewing the lead in your Zendesk Sell account.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors. The Zendesk Sell API uses standard HTTP status codes to indicate success or failure. Here are some common codes:
- 200 OK: The request was successful.
- 201 Created: A new resource was successfully created.
- 400 Bad Request: The request was malformed.
- 401 Unauthorized: Authentication failed.
- 404 Not Found: The requested resource does not exist.
- 429 Too Many Requests: Rate limit exceeded.
For a complete list of error codes, refer to the Zendesk Sell API documentation.
By following these steps, you can effectively manage leads in Zendesk Sell using Python. Ensure you test your API calls in the sandbox environment before deploying them to production.
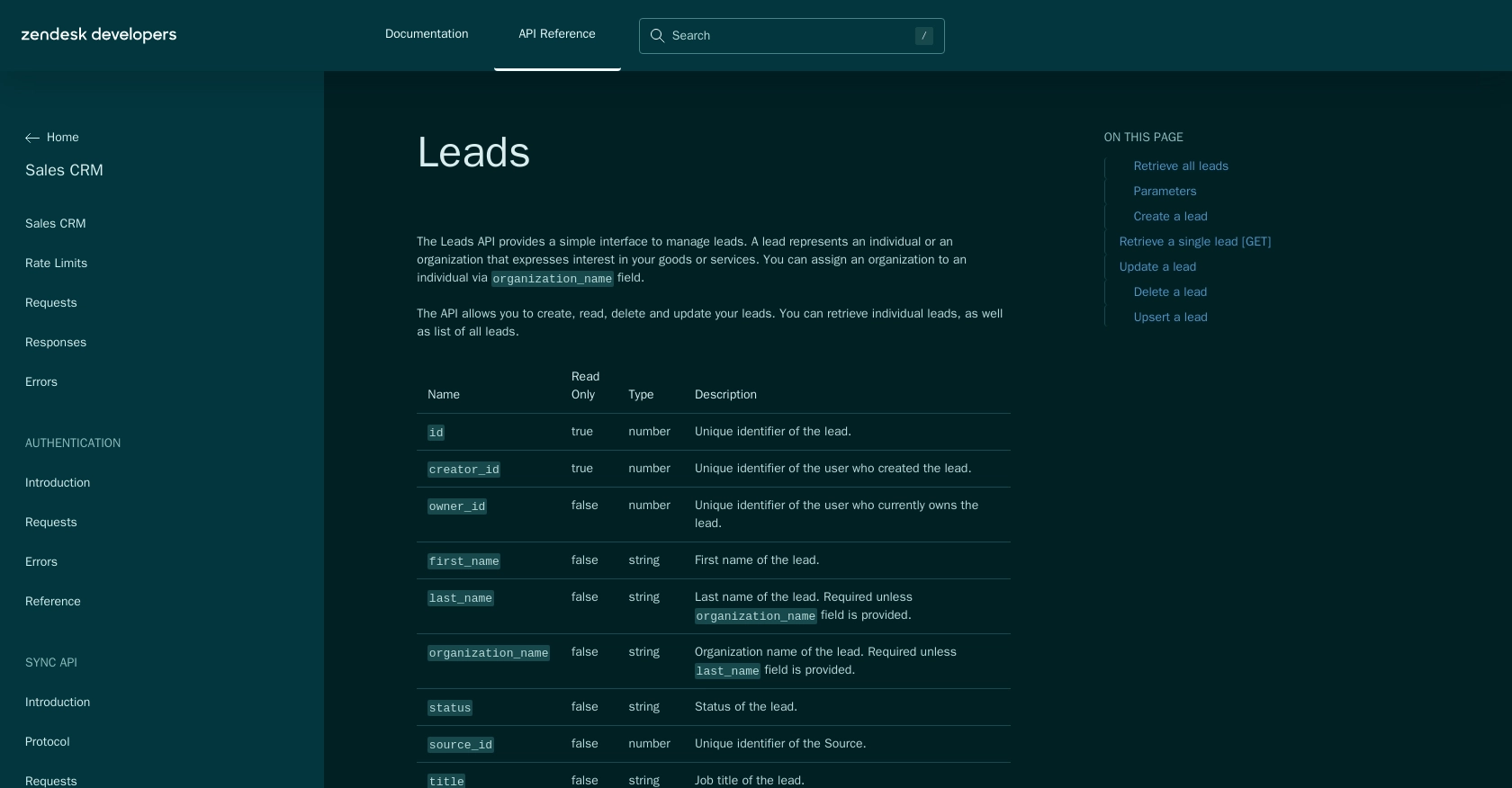
Conclusion and Best Practices for Zendesk Sell API Integration
Integrating with the Zendesk Sell API can significantly enhance your sales processes by automating lead management and ensuring your sales team has access to the most current data. By following the steps outlined in this guide, you can efficiently create and update leads using Python, streamlining your workflow and improving productivity.
Best Practices for Secure and Efficient Zendesk Sell API Usage
- Securely Store Credentials: Always store your OAuth tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the Zendesk Sell API rate limit of 36,000 requests per hour. Implement logic to handle the
429 Too Many Requests
error by retrying requests after a delay. - Data Transformation: Standardize and transform data fields as needed to ensure consistency across different systems.
- Test Thoroughly: Always test your API calls in a sandbox environment before deploying them to production to avoid unintended data changes.
Streamline Your Integrations with Endgrate
While integrating with individual APIs like Zendesk Sell can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product. By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
- https://endgrate.com/provider/zendesksell
- https://developer.zendesk.com/api-reference/sales-crm/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/rate-limits/
- https://developer.zendesk.com/api-reference/sales-crm/requests/
- https://developer.zendesk.com/api-reference/sales-crm/errors/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/requests/#client-authentication
- https://developer.zendesk.com/api-reference/sales-crm/resources/leads/
Ready to get started?