Using the Pipedrive API to Create or Update Leads (with Python examples)
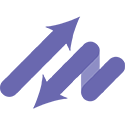
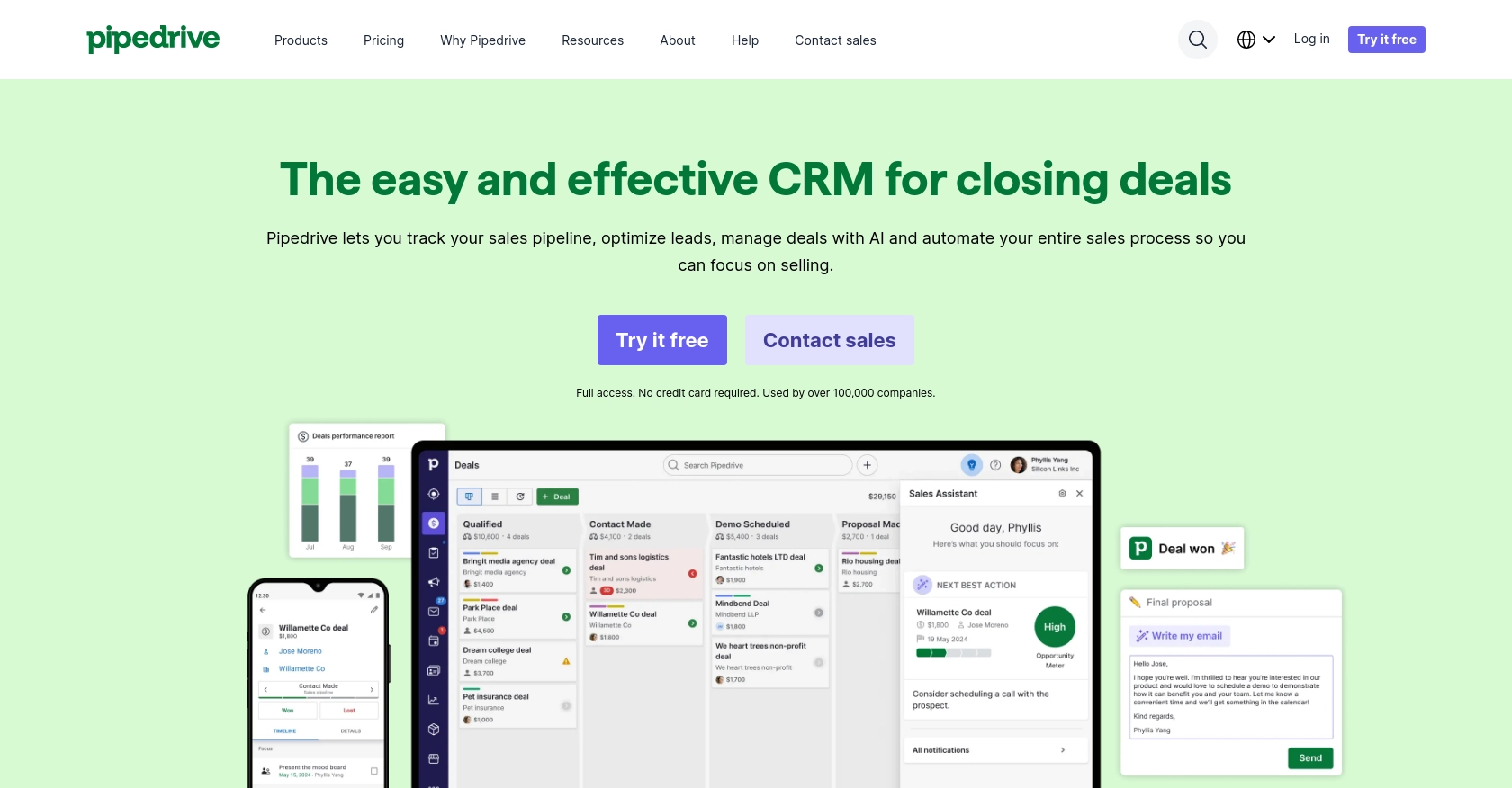
Introduction to Pipedrive CRM
Pipedrive is a robust sales CRM platform designed to help businesses manage their sales processes effectively. Known for its intuitive interface and powerful features, Pipedrive enables sales teams to track leads, manage deals, and optimize their sales pipeline.
Integrating with Pipedrive's API allows developers to automate and enhance sales operations, such as creating or updating leads. For example, a developer might use the Pipedrive API to automatically update lead information from an external source, ensuring that sales teams have the most current data at their fingertips.
Setting Up Your Pipedrive Developer Sandbox Account
Before diving into Pipedrive API integration, it's crucial to set up a developer sandbox account. This environment allows you to test and develop your application without affecting live data, providing a risk-free space to experiment and refine your integration.
Creating a Pipedrive Developer Sandbox Account
To get started, you'll need to create a developer sandbox account. Follow these steps:
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to a sandbox account. This account mimics a regular Pipedrive company account but is limited to five seats by default.
- Once your account is set up, you can import sample data to familiarize yourself with Pipedrive's features. Navigate to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app to import template spreadsheets.
Creating a Pipedrive App for OAuth Authentication
Pipedrive uses OAuth 2.0 for authentication, which requires creating an app in the Developer Hub. Here's how to do it:
- Log in to your Pipedrive sandbox account and navigate to the Developer Hub.
- Register your app by providing the necessary details. This process will generate a client ID and client secret, which are essential for OAuth authentication.
- Ensure your app's scopes are correctly set to access the required data. Only request scopes that are necessary for your integration to minimize security risks.
With your sandbox account and app set up, you're ready to start integrating with the Pipedrive API. In the next section, we'll explore how to make API calls using Python to create or update leads.
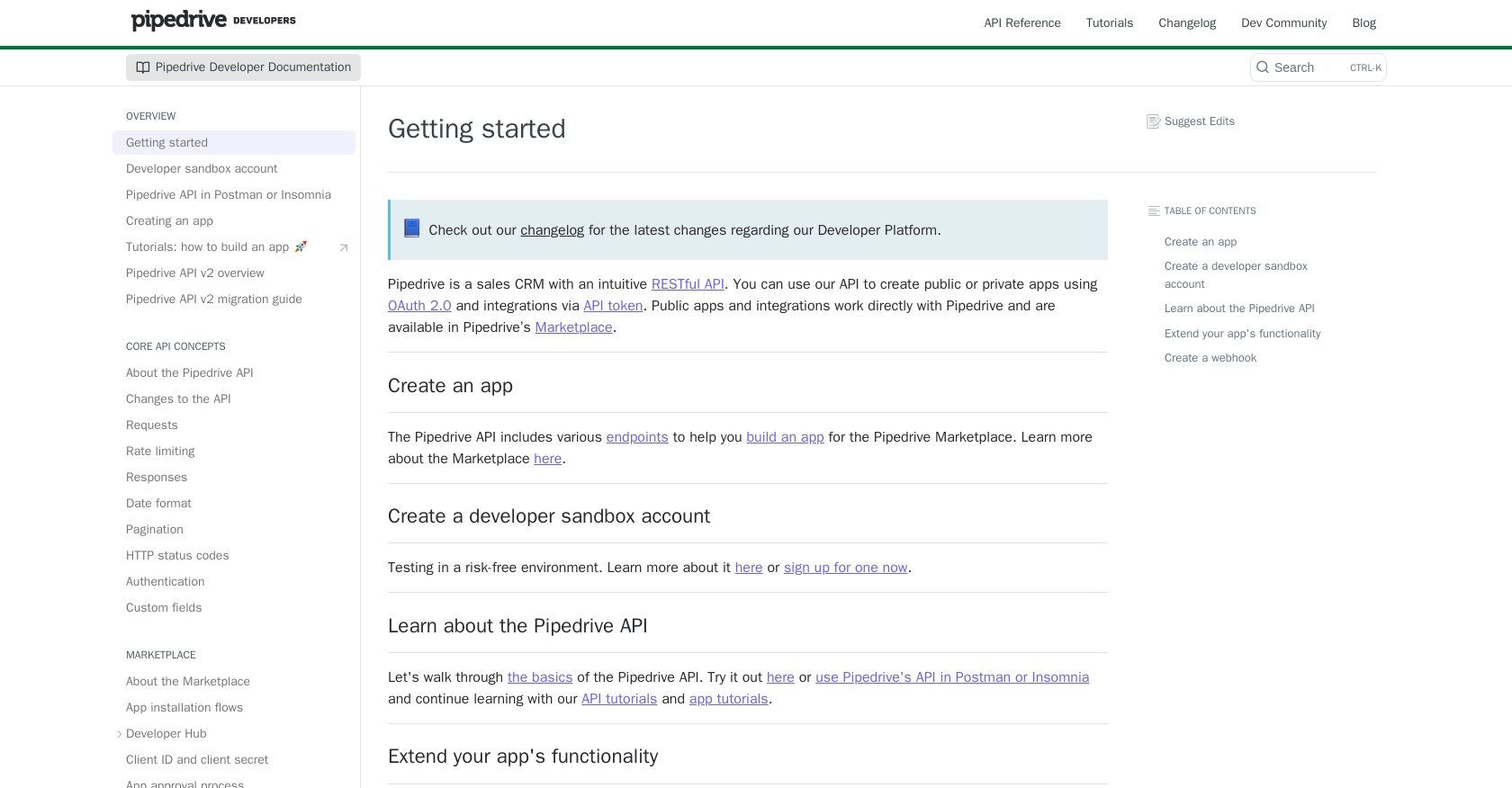
sbb-itb-96038d7
Making API Calls to Pipedrive Using Python
To interact with the Pipedrive API for creating or updating leads, you'll need to use Python. This section will guide you through setting up your environment, making API calls, and handling responses effectively.
Setting Up Your Python Environment for Pipedrive API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library using the following command:
pip install requests
Creating Leads in Pipedrive Using Python
To create a lead in Pipedrive, you'll need to make a POST request to the API. Here's a step-by-step guide:
import requests
# Set the API endpoint
url = "https://api.pipedrive.com/v1/leads"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the lead data
lead_data = {
"title": "New Lead",
"person_id": 12345,
"organization_id": 67890,
"value": {"amount": 500, "currency": "USD"}
}
# Make the POST request
response = requests.post(url, json=lead_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Lead created successfully:", response.json())
else:
print("Failed to create lead:", response.json())
Replace Your_Access_Token
with the token obtained during OAuth authentication. This code sends a POST request to create a new lead, and if successful, it prints the lead details.
Updating Leads in Pipedrive Using Python
To update an existing lead, use the PATCH method. Here's how you can do it:
import requests
# Set the API endpoint with the lead ID
lead_id = "your_lead_id"
url = f"https://api.pipedrive.com/v1/leads/{lead_id}"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the updated lead data
update_data = {
"title": "Updated Lead Title",
"value": {"amount": 750, "currency": "USD"}
}
# Make the PATCH request
response = requests.patch(url, json=update_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Lead updated successfully:", response.json())
else:
print("Failed to update lead:", response.json())
Replace your_lead_id
with the ID of the lead you wish to update. This code updates the lead's title and value, and prints the updated lead details if successful.
Handling API Responses and Errors in Pipedrive
When making API calls, it's crucial to handle responses and potential errors effectively. Pipedrive's API uses standard HTTP status codes to indicate success or failure:
- 200 OK: Request fulfilled successfully.
- 201 Created: New resource created successfully.
- 400 Bad Request: Request not understood by the server.
- 401 Unauthorized: Invalid API token or access token.
- 429 Too Many Requests: Rate limit exceeded.
For more details on handling errors, refer to the Pipedrive HTTP status codes documentation.
Verifying API Requests in Pipedrive Sandbox
After making API calls, verify the results in your Pipedrive sandbox account. Check if the leads are created or updated as expected. This ensures your integration is functioning correctly before deploying it to a live environment.
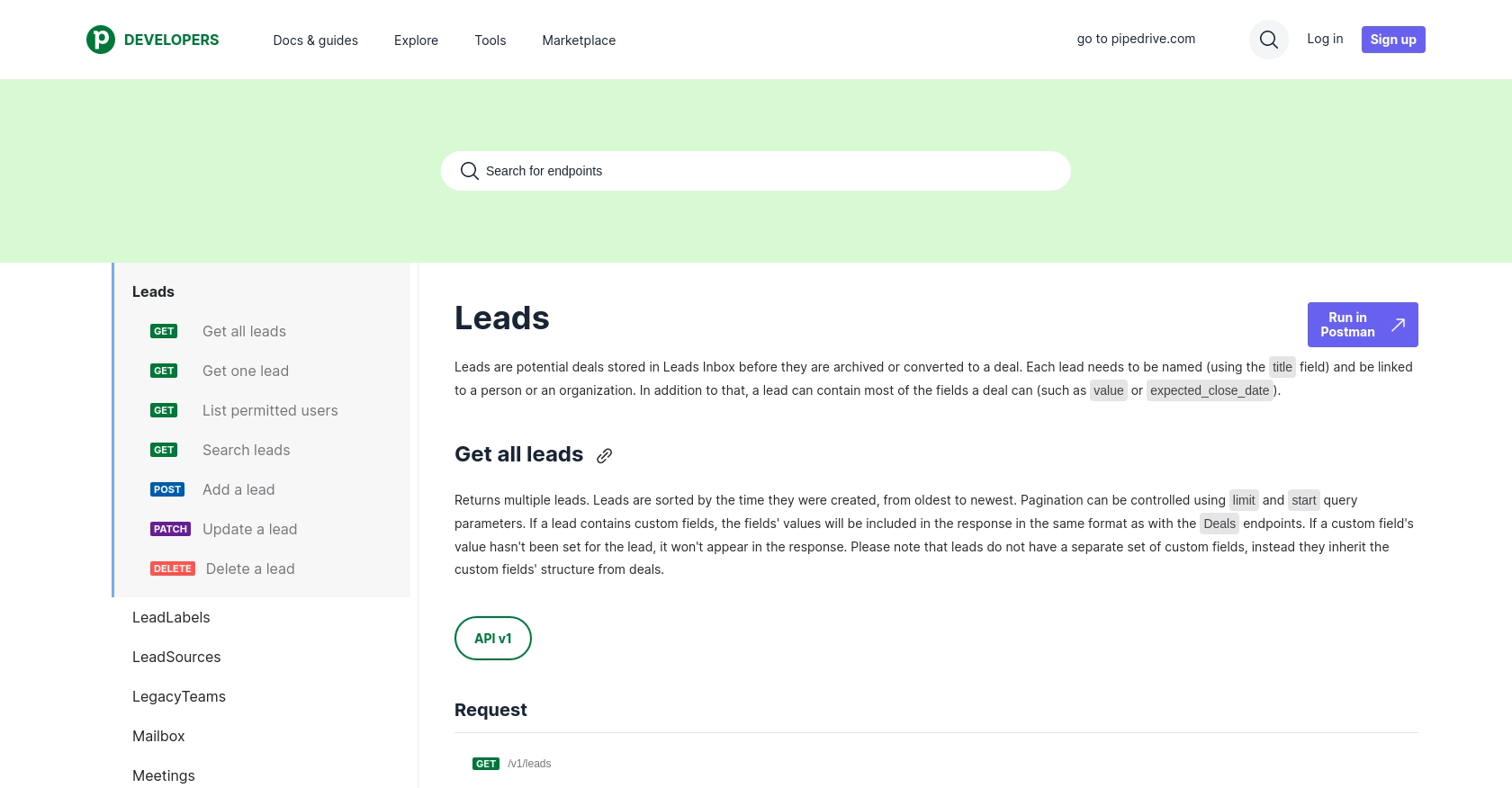
Conclusion: Best Practices for Pipedrive API Integration
Integrating with the Pipedrive API can significantly enhance your sales operations by automating lead management processes. By following the steps outlined in this guide, you can efficiently create and update leads using Python, ensuring your sales team always has access to the most current information.
Best Practices for Storing User Credentials Securely
When working with OAuth tokens, it's crucial to store them securely. Avoid hardcoding tokens in your source code. Instead, use environment variables or secure vaults to manage sensitive information. This practice helps protect your application from unauthorized access.
Handling Pipedrive API Rate Limiting
Pipedrive's API enforces rate limits to ensure fair usage. For OAuth apps, the rate limit is 80 requests per 2 seconds per access token. To avoid hitting these limits, consider implementing exponential backoff strategies or using webhooks to receive updates instead of polling the API frequently. For more details, refer to the Pipedrive rate limiting documentation.
Transforming and Standardizing Data Fields
When integrating with Pipedrive, ensure that data fields are standardized and transformed as needed. This might involve converting currencies, normalizing date formats, or mapping custom fields to match Pipedrive's structure. Consistent data handling ensures seamless integration and accurate reporting.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Pipedrive. By leveraging Endgrate, you can focus on your core product while outsourcing integration management, saving time and resources. Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
By adhering to these best practices, you can ensure a robust and efficient integration with Pipedrive, enhancing your sales processes and driving business success.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Leads
Ready to get started?