Using the PipelineCRM API to Get Users in Javascript
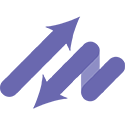
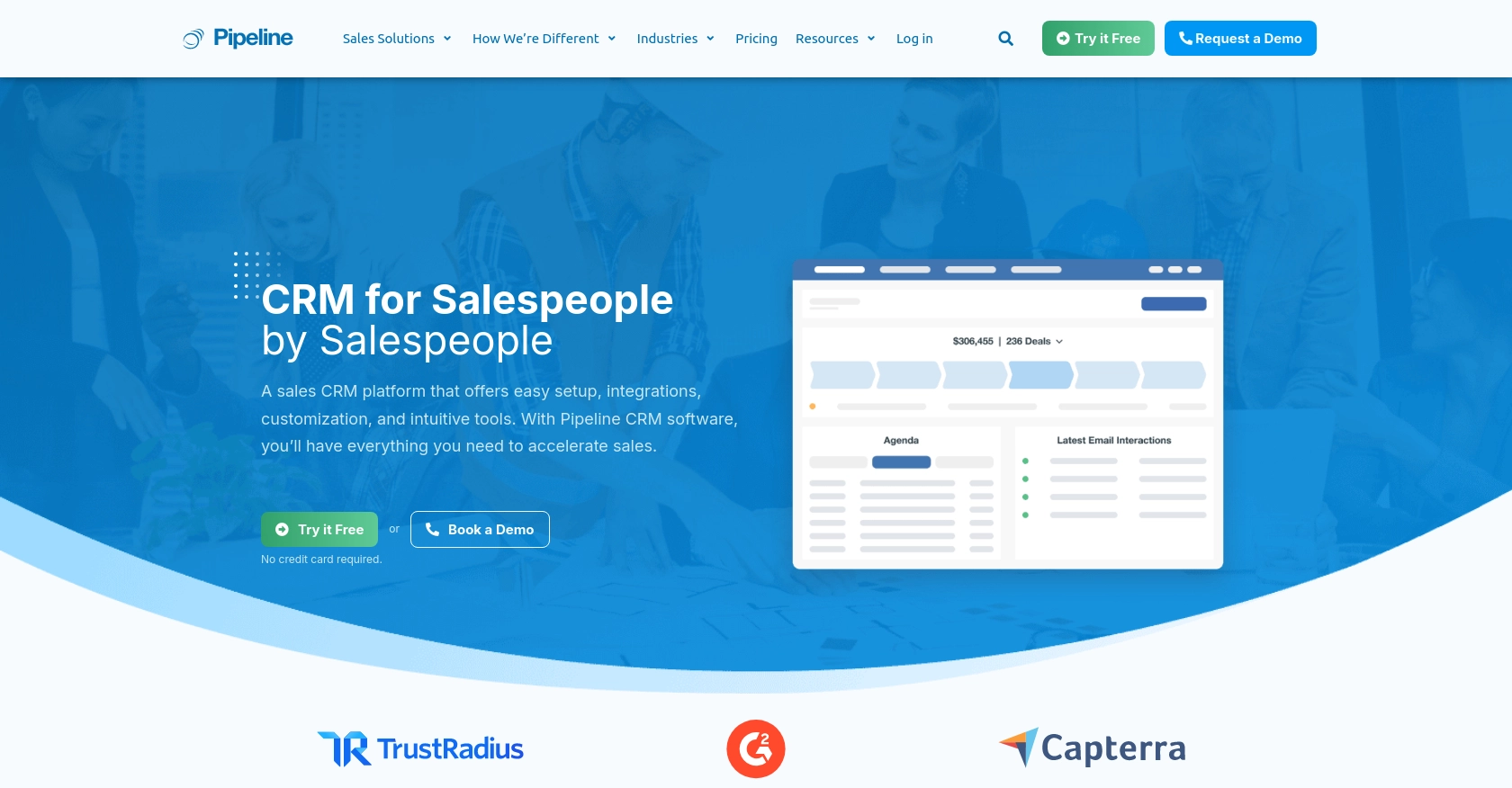
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes more effectively. With features like contact management, sales tracking, and reporting, PipelineCRM offers a comprehensive solution for sales teams looking to streamline their operations.
Integrating with the PipelineCRM API allows developers to access and manage user data programmatically, enabling automation and customization of CRM workflows. For example, a developer might use the PipelineCRM API to retrieve user information and integrate it with other business applications, enhancing data consistency and operational efficiency.
Setting Up Your PipelineCRM Test Account
Before you can start interacting with the PipelineCRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Create a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on their website. This trial will give you access to the necessary features to test API interactions.
- Visit the PipelineCRM website.
- Follow the instructions to create a new account.
- Once your account is set up, log in to access the dashboard.
Generate Your API Key for Authentication
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Navigate to the account settings in your PipelineCRM dashboard.
- Locate the API section and click on "Generate API Key."
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
Configure Your Development Environment
With your API key ready, you can now configure your development environment to start making API calls:
- Ensure you have a modern version of JavaScript installed on your machine.
- Use a code editor of your choice to write and test your JavaScript code.
- Install any necessary dependencies, such as Axios or Fetch API, to handle HTTP requests.
With these steps completed, you're ready to start interacting with the PipelineCRM API using JavaScript.
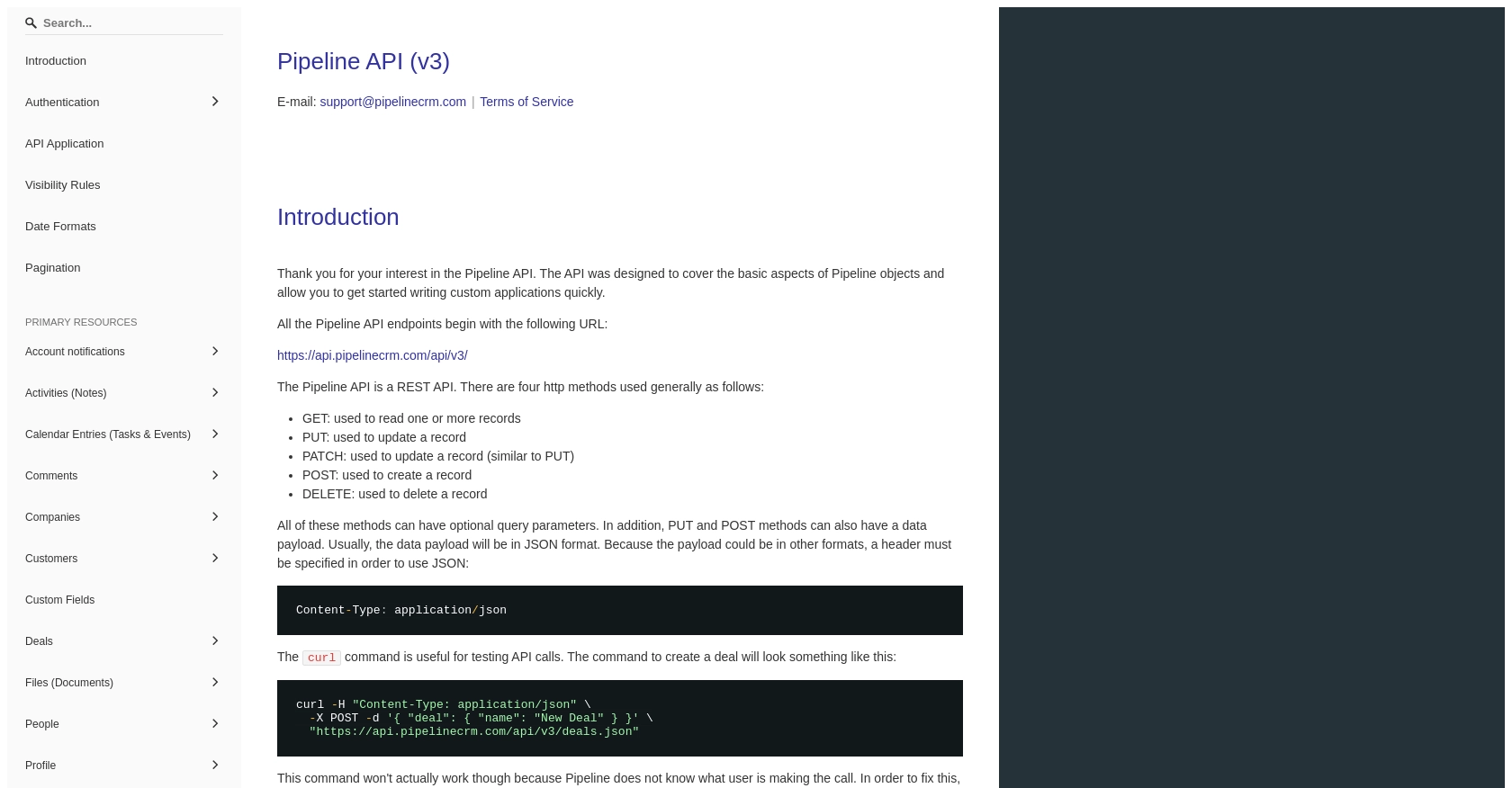
sbb-itb-96038d7
Making API Calls to Retrieve Users from PipelineCRM Using JavaScript
To interact with the PipelineCRM API and retrieve user data, you'll need to make HTTP requests using JavaScript. This section will guide you through the process of setting up your environment and executing the API call to fetch user information.
Setting Up Your JavaScript Environment for PipelineCRM API
Before making API calls, ensure your development environment is properly configured:
- Install Node.js if you haven't already, as it provides a runtime for executing JavaScript outside the browser.
- Use a package manager like npm to install necessary libraries such as Axios, which simplifies HTTP requests.
Installing Axios for HTTP Requests
To handle HTTP requests, we'll use Axios, a popular promise-based HTTP client for JavaScript:
npm install axios
Writing JavaScript Code to Fetch Users from PipelineCRM
With Axios installed, you can now write the JavaScript code to make an API call to PipelineCRM and retrieve user data:
const axios = require('axios');
// Set the API endpoint and your API key
const endpoint = 'https://api.pipelinecrm.com/v3/users';
const apiKey = 'Your_API_Key';
// Make a GET request to the PipelineCRM API
axios.get(endpoint, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => {
// Handle the successful response
console.log('Users:', response.data);
})
.catch(error => {
// Handle errors
console.error('Error fetching users:', error);
});
Replace Your_API_Key
with the API key you generated earlier. This code sets up the API endpoint and includes the necessary authorization header to authenticate your request.
Running Your JavaScript Code
To execute the code and retrieve user data from PipelineCRM, run the following command in your terminal:
node your_script_name.js
Upon successful execution, you should see a list of users printed in the console. If there are any errors, ensure your API key is correct and that you have network access to the PipelineCRM API.
Handling Errors and Validating API Responses
It's crucial to handle potential errors when making API calls. Common errors include network issues, invalid API keys, or exceeding rate limits. Check the error response for specific error codes and messages to diagnose issues.
Always validate the API response by checking the status code and the structure of the returned data. This ensures that your application can handle unexpected responses gracefully.
Conclusion and Best Practices for Using PipelineCRM API with JavaScript
Integrating with the PipelineCRM API using JavaScript can significantly enhance your CRM workflows by allowing seamless access to user data. By following the steps outlined in this guide, you can efficiently set up your development environment, authenticate API requests, and retrieve user information.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of any rate limits imposed by the PipelineCRM API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements. This can help maintain data consistency across systems.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider leveraging Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including PipelineCRM, allowing you to focus on your core product while outsourcing integration complexities.
By using Endgrate, you can build once for each use case instead of multiple times for different integrations, saving time and resources. Take advantage of an intuitive integration experience to enhance your application's capabilities effortlessly.
Visit Endgrate to learn more about how it can support your integration needs.
Read More
Ready to get started?