Using the Apollo API to Get Contacts (with Javascript examples)
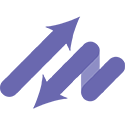
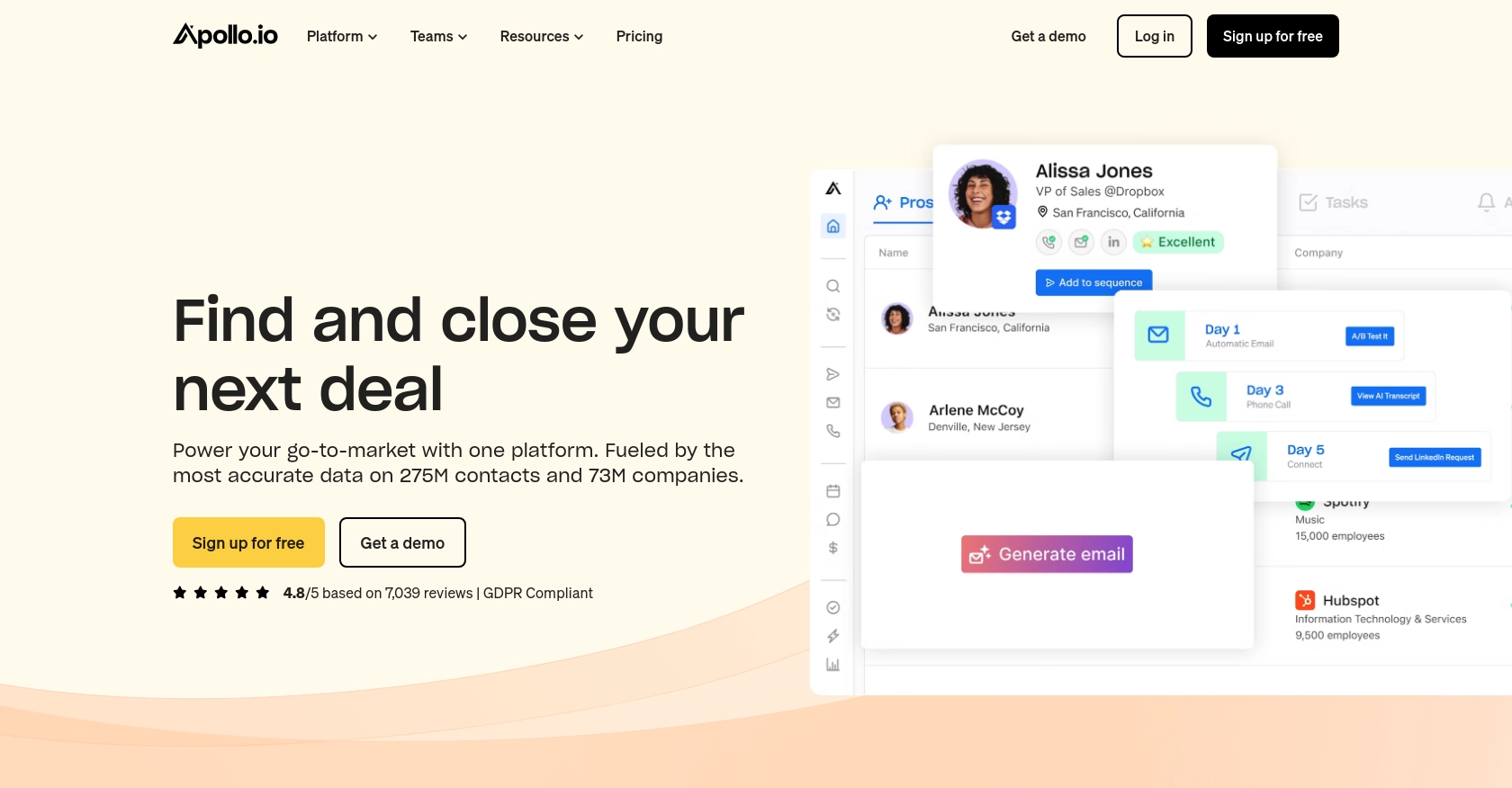
Introduction to Apollo API
Apollo is a powerful sales intelligence and engagement platform that helps businesses streamline their sales processes by providing access to a vast database of contacts and companies. With its comprehensive API, Apollo allows developers to integrate its rich data repository into their applications, enabling automation and customization of sales workflows.
Connecting with Apollo's API can be particularly beneficial for developers looking to enhance their CRM systems or automate outreach efforts. For example, you might want to retrieve contact information from Apollo to enrich your CRM database, ensuring your sales team has the most up-to-date information for targeted marketing campaigns.
Setting Up Your Apollo API Test Account
Before you can start using the Apollo API to retrieve contacts, you need to set up a test account. This will allow you to safely experiment with the API without affecting any live data.
Create an Apollo Account
If you don't already have an Apollo account, you can sign up for a free trial on the Apollo website. This will give you access to the API and allow you to explore its features.
- Visit the Apollo website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generate an API Key for Apollo API Access
Once your account is set up, you'll need to generate an API key to authenticate your requests to the Apollo API. This key will be used in the headers of your API calls.
- Log in to your Apollo account and navigate to the API settings section.
- Click on "Generate API Key" to create a new key.
- Copy the generated API key and store it securely, as you'll need it for authentication in your API requests.
Configure API Key Authentication
With your API key ready, you can now configure your application to authenticate requests using this key. In your JavaScript code, include the API key in the headers of your requests as shown in the example below:
// Example of setting up headers with API key
const headers = {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
'X-Api-Key': 'YOUR_API_KEY_HERE'
};
Replace YOUR_API_KEY_HERE
with the actual API key you generated.
By following these steps, you'll have your Apollo test account set up and ready to interact with the API, allowing you to retrieve and manage contact data efficiently.
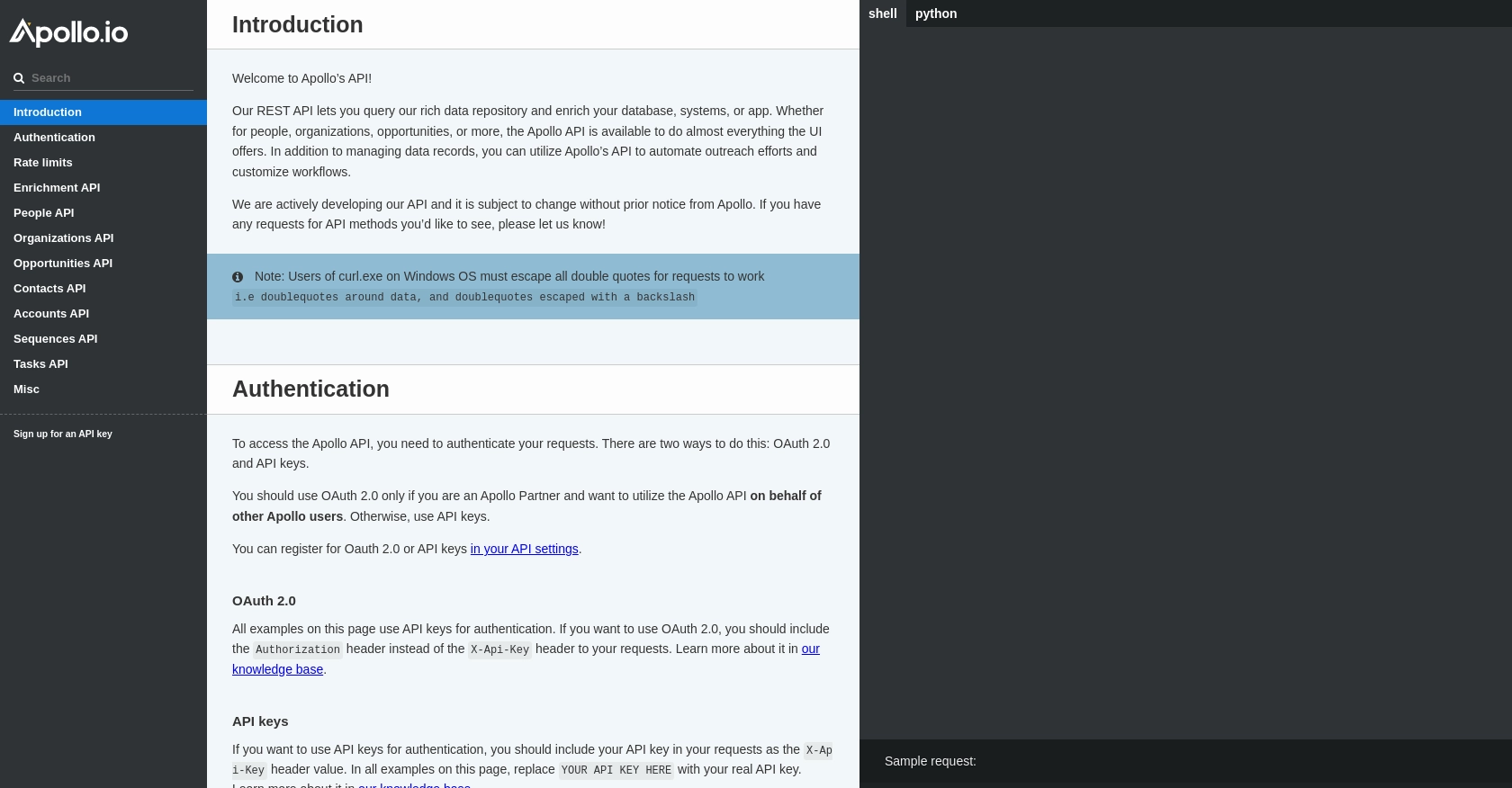
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Apollo Using JavaScript
To effectively interact with the Apollo API and retrieve contact information, you'll need to set up your JavaScript environment and make the necessary API calls. This section will guide you through the process, ensuring you have the right tools and code to get started.
Setting Up Your JavaScript Environment for Apollo API
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js or any modern browser that supports JavaScript. Additionally, you'll need to install the axios
library to simplify HTTP requests.
// Install axios using npm
npm install axios
Writing JavaScript Code to Fetch Contacts from Apollo API
With your environment ready, you can now write the JavaScript code to fetch contacts from the Apollo API. The following example demonstrates how to make a GET request to retrieve contact data:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.apollo.io/v1/contacts';
const headers = {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
'X-Api-Key': 'YOUR_API_KEY_HERE'
};
// Function to get contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
console.log('Contacts retrieved:', response.data.contacts);
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getContacts();
Replace YOUR_API_KEY_HERE
with your actual API key. This code sets up the necessary headers and makes a GET request to the Apollo API to retrieve contacts. The response data is logged to the console.
Handling API Response and Errors
After making the API call, it's important to handle the response and any potential errors. The example above includes error handling using a try-catch block, which logs any errors encountered during the request.
Check the response data to ensure the request was successful. You can verify the retrieved contacts by comparing them with the data in your Apollo test account.
Understanding Apollo API Rate Limits
When interacting with the Apollo API, be mindful of the rate limits. According to Apollo's documentation, the API allows up to 50 requests per minute. Exceeding this limit may result in temporary access restrictions.
To manage rate limits effectively, consider implementing a delay between requests or using a queue system to control the flow of API calls.
By following these steps, you can successfully make API calls to retrieve contacts from Apollo using JavaScript, enhancing your application's data integration capabilities.
Conclusion: Best Practices for Using Apollo API with JavaScript
Integrating Apollo's API into your applications can significantly enhance your data management and sales processes. By following the steps outlined in this guide, you can efficiently retrieve and manage contact information using JavaScript.
Securely Storing API Keys and Credentials
Always ensure that your API keys and credentials are stored securely. Avoid hardcoding them directly into your source code. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Apollo API Rate Limits Effectively
Be mindful of Apollo's API rate limits, which allow up to 50 requests per minute. Implement strategies such as request throttling or queuing to prevent exceeding these limits and ensure smooth API interactions.
Data Transformation and Standardization
When integrating data from Apollo into your systems, consider transforming and standardizing data fields to match your application's requirements. This ensures consistency and improves data usability across platforms.
Explore Further with Endgrate
For developers looking to streamline integration processes, consider using Endgrate. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Endgrate provides a unified API endpoint that simplifies interactions with multiple platforms, including Apollo, offering an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can optimize your integration efforts and enhance your application's capabilities.
Read More
Ready to get started?