Using the Chargebee API to Create or Update Product Catalog in PHP
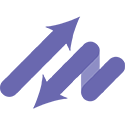
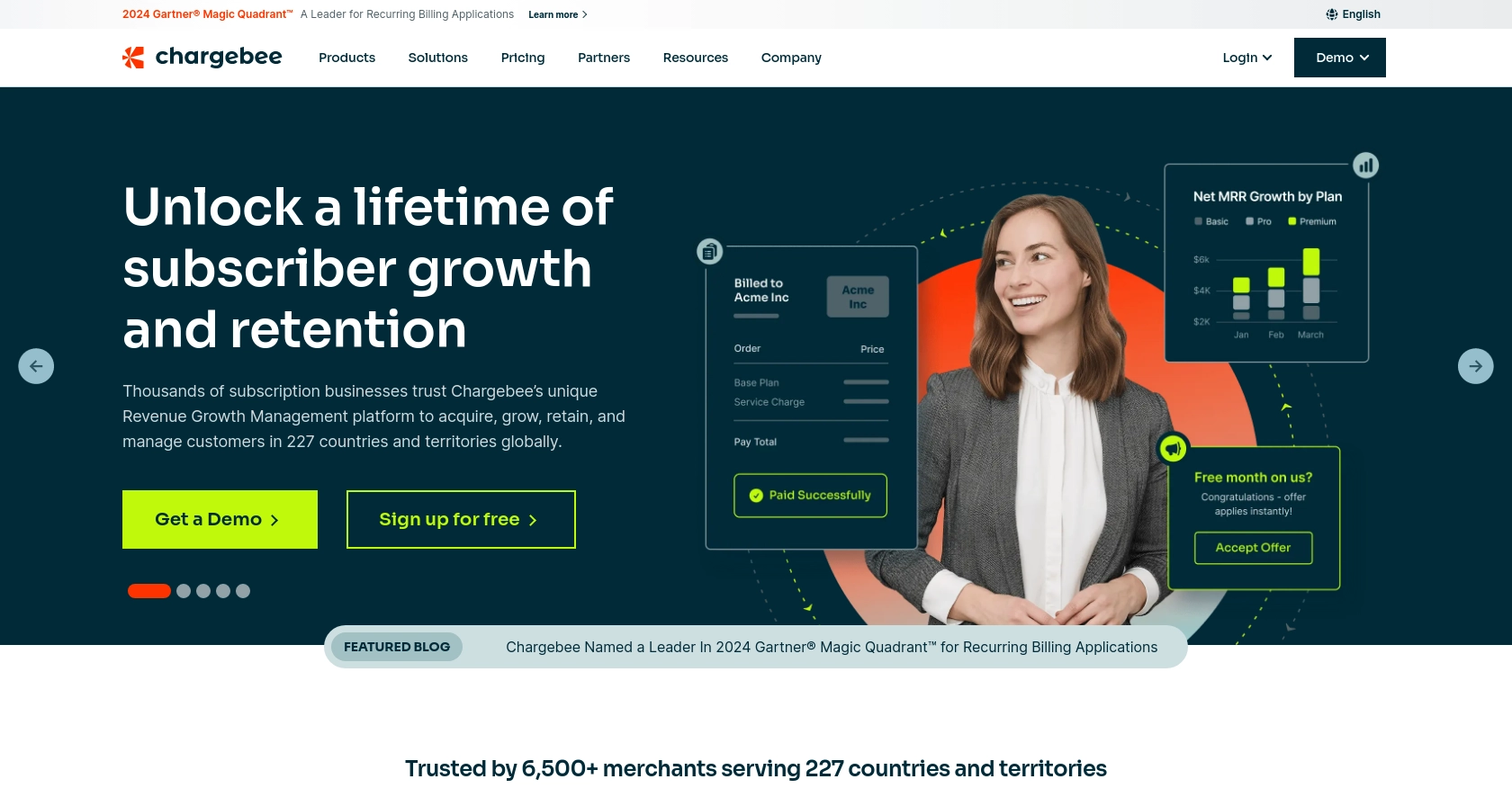
Introduction to Chargebee API for Product Catalog Management
Chargebee is a robust subscription billing and revenue management platform designed to streamline the billing processes for SaaS businesses. With its comprehensive suite of tools, Chargebee enables companies to manage subscriptions, invoicing, and revenue operations efficiently.
Integrating with Chargebee's API allows developers to automate and enhance their product catalog management. For example, a developer might use the Chargebee API to create or update items in the product catalog, ensuring that the latest offerings are always available to customers. This integration can significantly reduce manual efforts and improve the accuracy of product listings.
Setting Up a Chargebee Test or Sandbox Account for API Integration
Before you can start integrating with the Chargebee API to manage your product catalog, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a Chargebee Test Account
To begin, you'll need to create a Chargebee test account. Follow these steps:
- Visit the Chargebee website and sign up for a free trial.
- Once registered, log in to your Chargebee dashboard.
- Navigate to the 'Sites' section and create a new test site. This will serve as your sandbox environment.
Generating API Keys for Chargebee Authentication
Chargebee uses HTTP Basic authentication for API calls, where your API key acts as the username and the password is left empty. Follow these steps to generate your API keys:
- In your Chargebee dashboard, go to the 'API Keys' section under 'Settings'.
- Click on 'Create a Key' and choose the appropriate permissions for your integration needs.
- Copy the generated API key. Remember, the API keys for your test site and live site are different.
Configuring OAuth for Chargebee API Access
Although Chargebee primarily uses API keys, you may need to configure OAuth for certain integrations. Here's how:
- In the Chargebee dashboard, navigate to 'OAuth Apps' under 'Integrations'.
- Click 'Create New App' and fill in the necessary details, such as the app name and redirect URL.
- Save the app to generate the client ID and client secret, which you'll use for OAuth authentication.
With your test account and API keys set up, you're ready to start integrating with the Chargebee API to manage your product catalog using PHP.
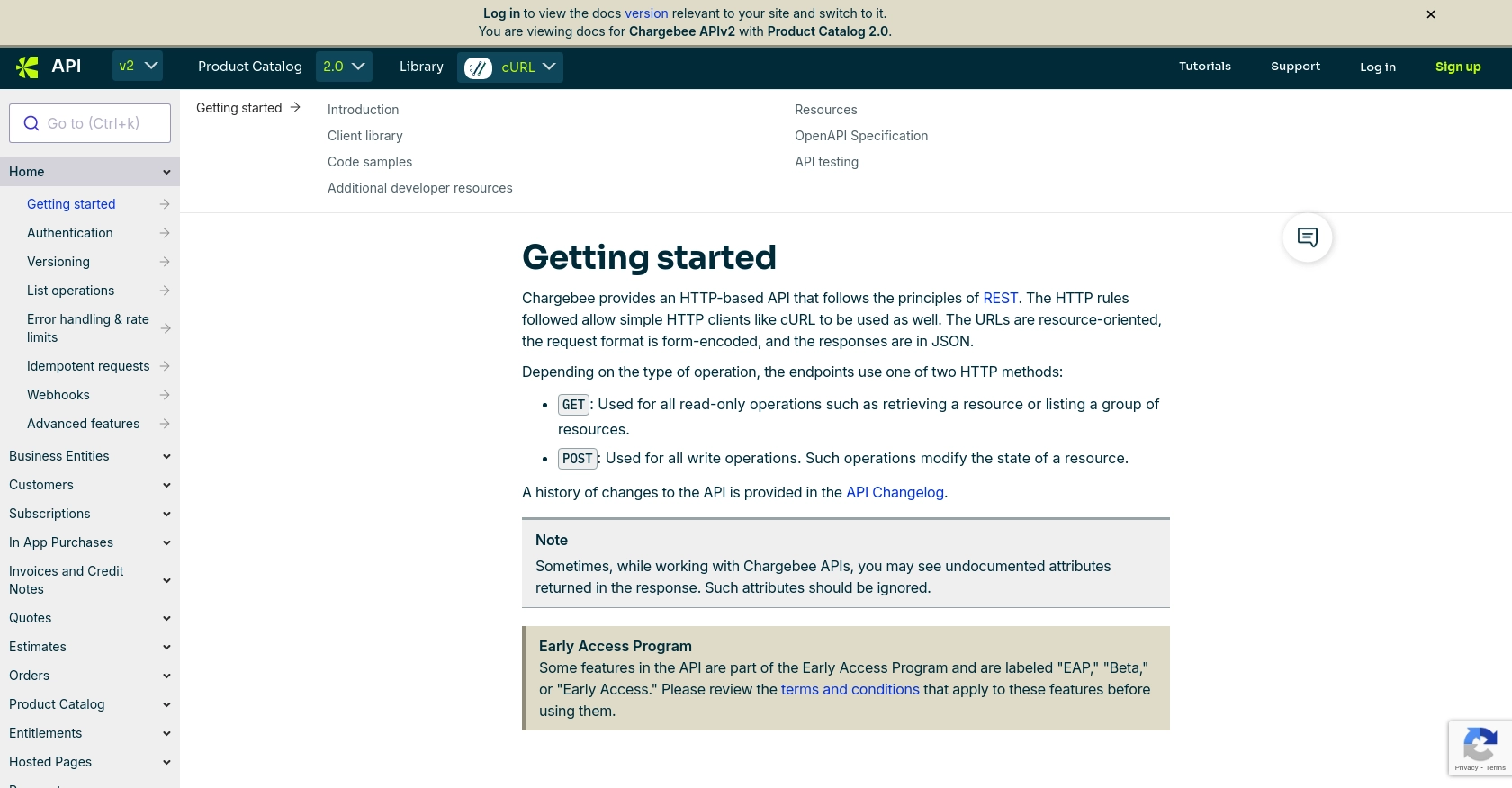
sbb-itb-96038d7
How to Make API Calls to Chargebee for Product Catalog Management Using PHP
To interact with the Chargebee API for creating or updating your product catalog, you'll need to use PHP. This section will guide you through the process, including setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your PHP Environment for Chargebee API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- cURL extension enabled
- Composer for dependency management
Install the Chargebee PHP library using Composer:
composer require chargebee/chargebee-php
Creating or Updating Items in Chargebee Product Catalog
With your environment ready, you can now create or update items in your Chargebee product catalog. Here's a sample script to create a new item:
require 'vendor/autoload.php';
use ChargeBee\ChargeBee\Models\Item;
use ChargeBee\ChargeBee\Environment;
// Set your Chargebee site and API key
Environment::configure("{site}", "{api_key}");
// Define the item details
$itemParams = [
"id" => "silver",
"name" => "Silver Plan",
"type" => "plan",
"itemApplicability" => "all"
];
// Create the item
try {
$result = Item::create($itemParams);
echo "Item created successfully: " . $result->item()->name;
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Replace {site}
and {api_key}
with your Chargebee site name and API key.
Verifying API Call Success in Chargebee
After executing the script, verify the item creation in your Chargebee dashboard under the 'Items' section. If successful, the new item should appear in your product catalog.
Handling Errors and Understanding Chargebee API Error Codes
Chargebee uses standard HTTP status codes to indicate the success or failure of an API call. Here are some common error codes:
- 400 Bad Request: Invalid input parameters.
- 401 Unauthorized: Authentication failed. Check your API key.
- 404 Not Found: Resource not found.
- 429 Too Many Requests: Rate limit exceeded. Retry after some time.
For more details on error handling, refer to the Chargebee API documentation.
Optimizing API Calls and Managing Rate Limits with Chargebee
Chargebee imposes rate limits on API requests. For test sites, the limit is approximately 750 API calls every 5 minutes. For live sites, it's around 150 API calls per minute. To avoid hitting these limits:
- Implement exponential backoff for retries.
- Spread requests over time to prevent spikes.
- Monitor your API usage and adjust as necessary.
For more information on rate limits, visit the Chargebee API documentation.
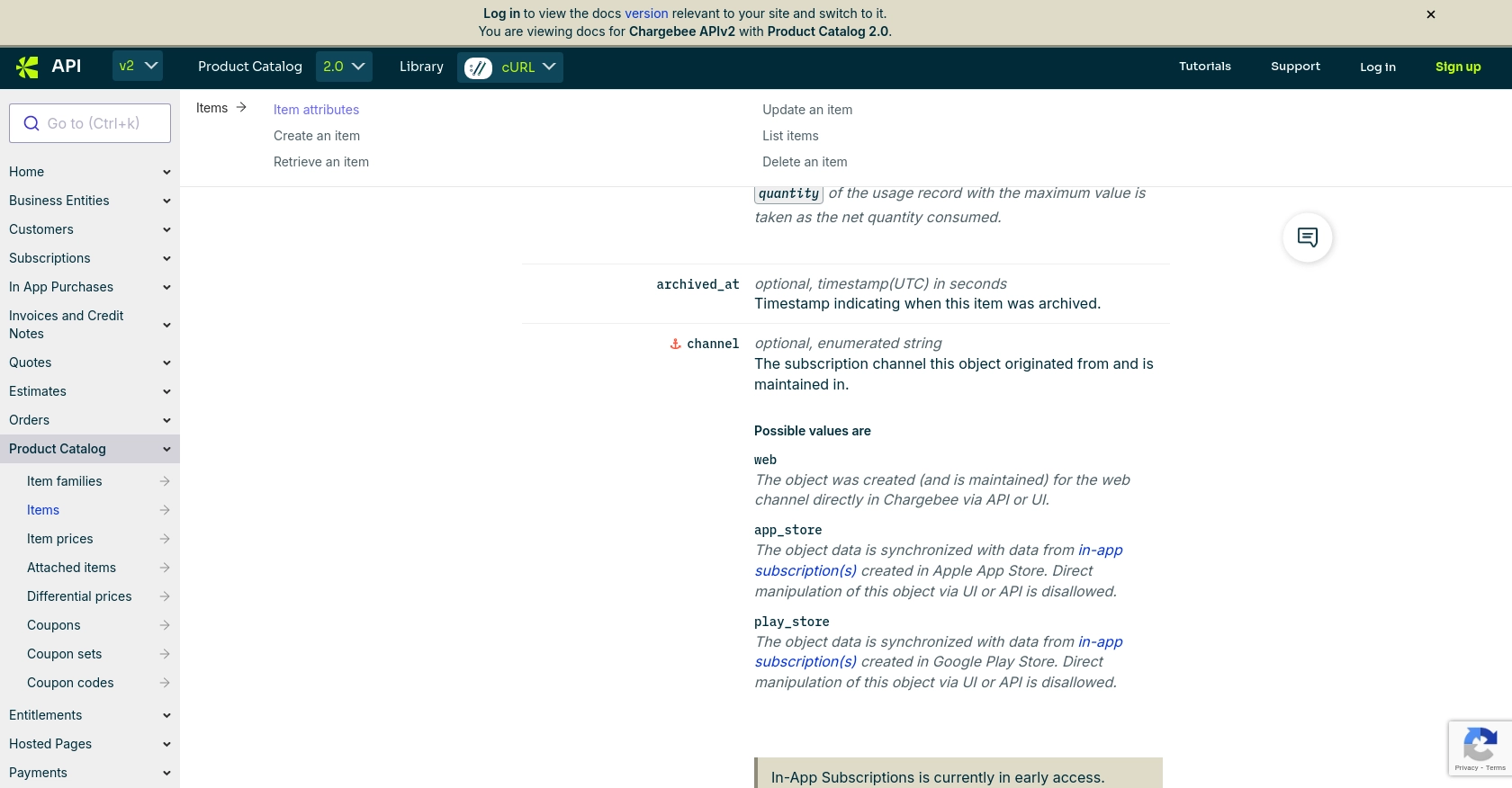
Conclusion and Best Practices for Chargebee API Integration in PHP
Integrating with the Chargebee API for product catalog management in PHP can significantly streamline your subscription billing processes. By automating the creation and updating of items, you ensure that your product offerings are always current and accurate, reducing manual errors and saving valuable time.
Best Practices for Secure and Efficient Chargebee API Integration
- Secure API Keys: Store your API keys securely and avoid hardcoding them in your application. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Implement strategies like exponential backoff and request spreading to manage API rate limits effectively. This ensures your application remains responsive and avoids unnecessary throttling.
- Data Standardization: Ensure that data fields are standardized and consistent across your application to facilitate seamless integration and data management.
- Error Handling: Implement robust error handling to manage API responses effectively. Use Chargebee's error codes to identify and resolve issues promptly.
Enhance Your Integration Strategy with Endgrate
While integrating with Chargebee's API offers numerous benefits, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Chargebee. This allows you to focus on your core product while outsourcing integration management.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can optimize your integration strategy and enhance your product offerings.
Read More
Ready to get started?