Using the Asana API to Get Tasks (with PHP examples)
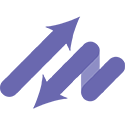
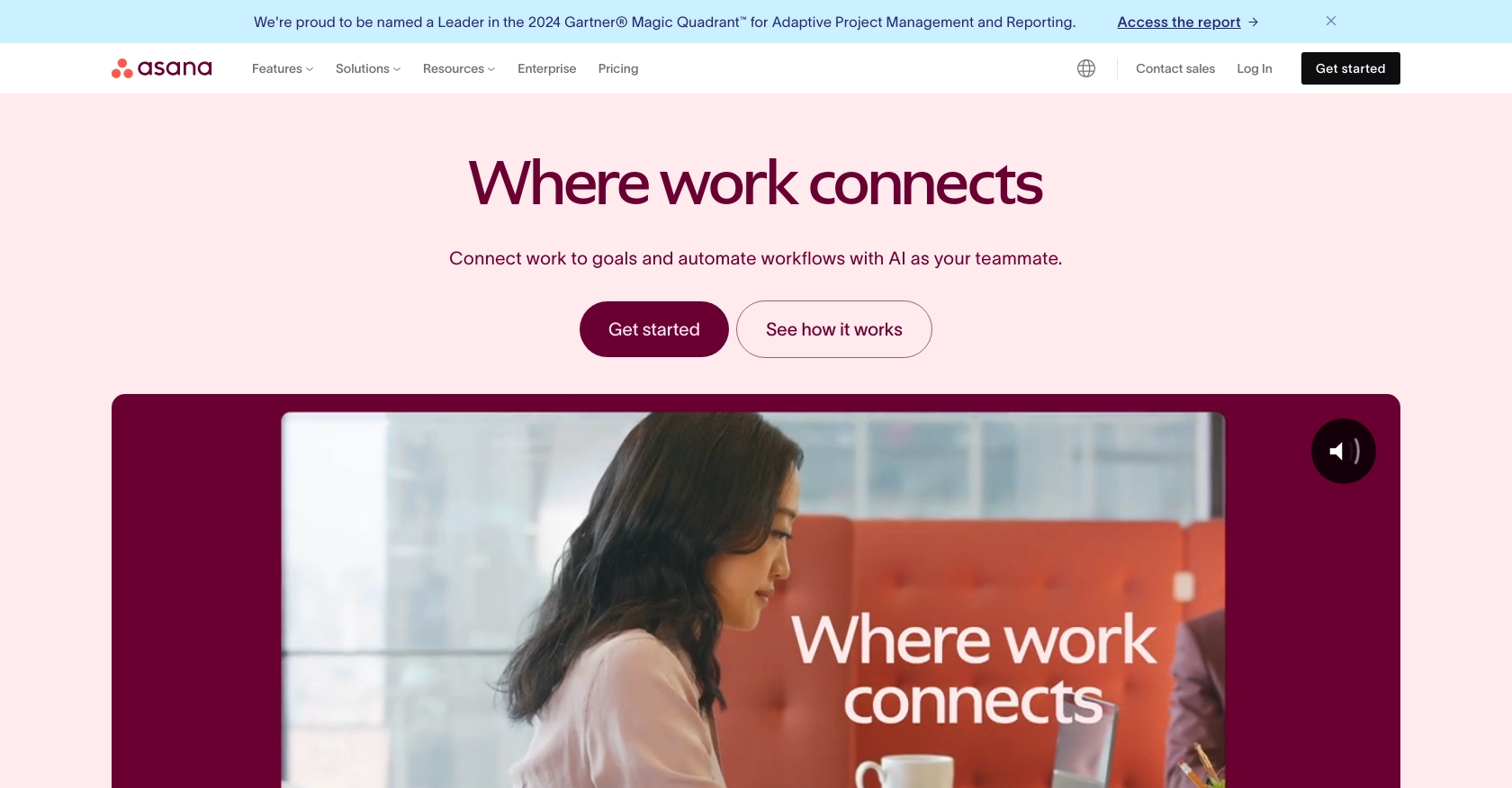
Introduction to Asana API Integration
Asana is a powerful project management tool that helps teams organize, track, and manage their work. With its user-friendly interface and robust features, Asana is widely used by businesses to enhance productivity and collaboration.
Integrating with Asana's API allows developers to automate and streamline workflows by accessing and managing tasks programmatically. For example, you can use the Asana API to retrieve tasks and integrate them into a custom dashboard, providing real-time updates and insights into project progress.
This article will guide you through using PHP to interact with the Asana API, specifically focusing on retrieving tasks. By following this tutorial, you'll learn how to efficiently access and manage tasks within Asana, enhancing your team's workflow automation capabilities.
Setting Up Your Asana Developer Account and Sandbox
Before you can start interacting with the Asana API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test API calls without affecting your live data. Asana provides a developer console where you can manage your apps and generate the necessary credentials.
Creating an Asana Developer Account
If you don't already have an Asana account, you can sign up for a free account on the Asana website. Once your account is created, log in to access the developer console.
Generating OAuth Credentials for Asana API
Asana uses OAuth for authentication, which is ideal for applications that require multiple users to sign in. Follow these steps to create an app and obtain the necessary credentials:
- Navigate to the Asana Developer Console.
- Click on "Create App" to start the process.
- Fill in the required information, such as the app name and redirect URL.
- Once the app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating API requests.
Configuring OAuth for Asana API Access
With your client ID and client secret, you can now set up OAuth to authenticate API requests:
- Direct users to Asana's authorization URL, including your client ID and redirect URL.
- Upon user authorization, Asana will redirect to your specified URL with an authorization code.
- Exchange this authorization code for an access token by making a POST request to Asana's token endpoint.
For detailed instructions, refer to the Asana OAuth documentation.
Testing in the Asana Sandbox Environment
Asana provides a sandbox environment for developers to test API interactions without affecting live data. You can request access to a developer sandbox through the Asana Developer Console. This environment includes premium features, allowing you to fully explore the API's capabilities.
With your developer account and OAuth credentials set up, you're ready to start making API calls to retrieve tasks from Asana using PHP.
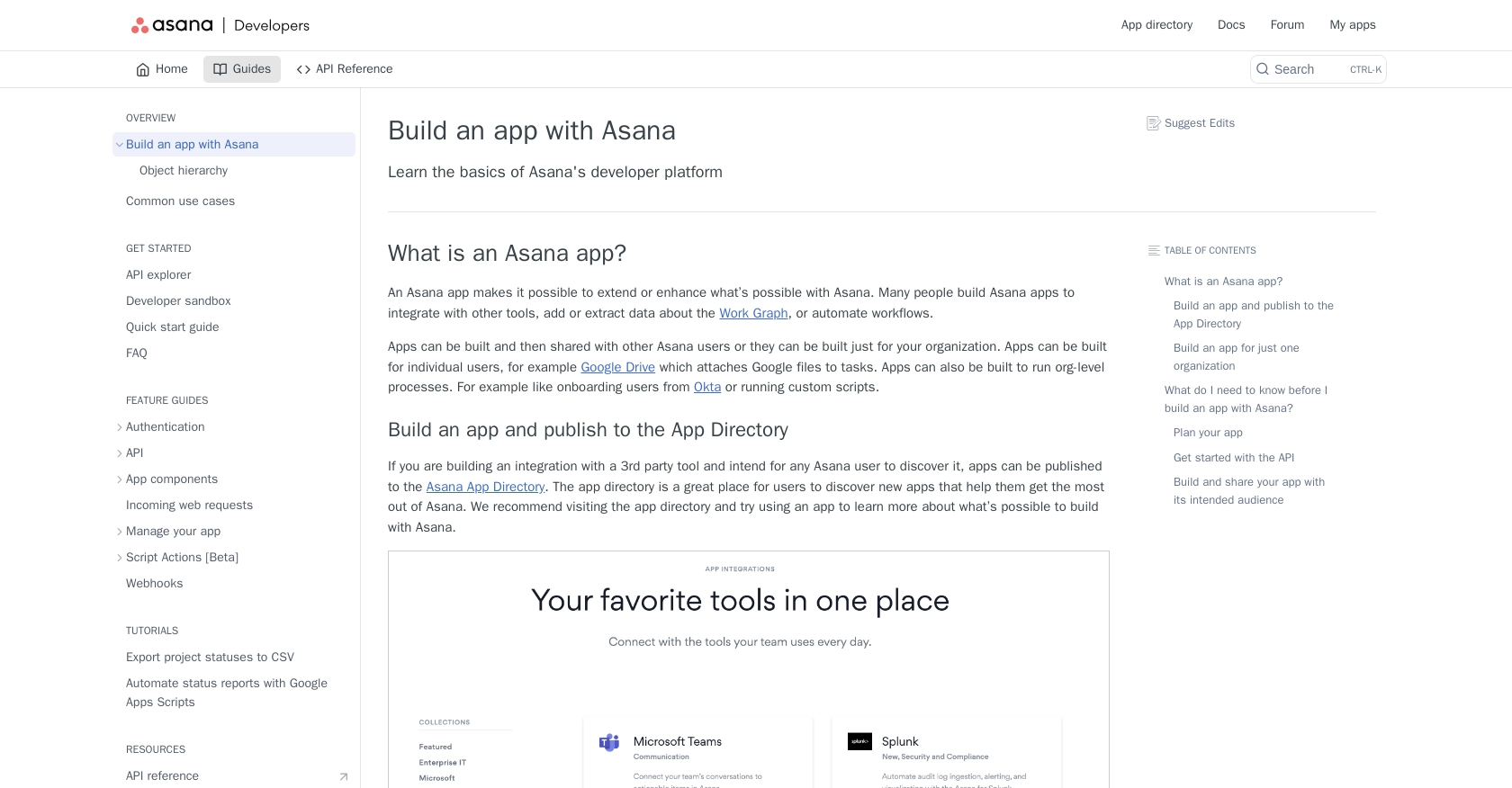
sbb-itb-96038d7
Making API Calls to Retrieve Asana Tasks Using PHP
To interact with the Asana API and retrieve tasks using PHP, you'll need to ensure you have the right setup and dependencies. This section will guide you through the process of making API calls, handling responses, and managing errors effectively.
Setting Up Your PHP Environment for Asana API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL
extension enabled. You can verify your PHP version and extensions by running the following command:
php -v
php -m | grep curl
If cURL
is not enabled, you can enable it by modifying your php.ini
file.
Installing Required PHP Libraries for Asana API
To simplify HTTP requests, you can use the Guzzle library. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Example Code to Retrieve Tasks from Asana API
Once your environment is set up, you can use the following PHP code to retrieve tasks from Asana:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$response = $client->request('GET', 'https://app.asana.com/api/1.0/tasks', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
if ($response->getStatusCode() === 200) {
$tasks = json_decode($response->getBody(), true);
foreach ($tasks['data'] as $task) {
echo 'Task Name: ' . $task['name'] . "\n";
}
} else {
echo 'Failed to retrieve tasks. Status code: ' . $response->getStatusCode();
}
Replace Your_Access_Token
with the access token obtained through OAuth. This script sends a GET request to the Asana API to retrieve tasks and prints their names.
Verifying Successful API Requests in Asana Sandbox
After running the script, verify the retrieved tasks by checking your Asana sandbox environment. Ensure the tasks match those returned by the API call.
Handling Errors and Understanding Asana API Error Codes
When making API calls, it's crucial to handle potential errors. The Asana API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. Refer to the Asana authentication documentation for detailed error handling guidelines.
By following these steps, you can efficiently retrieve tasks from Asana using PHP, enhancing your project's workflow automation capabilities.
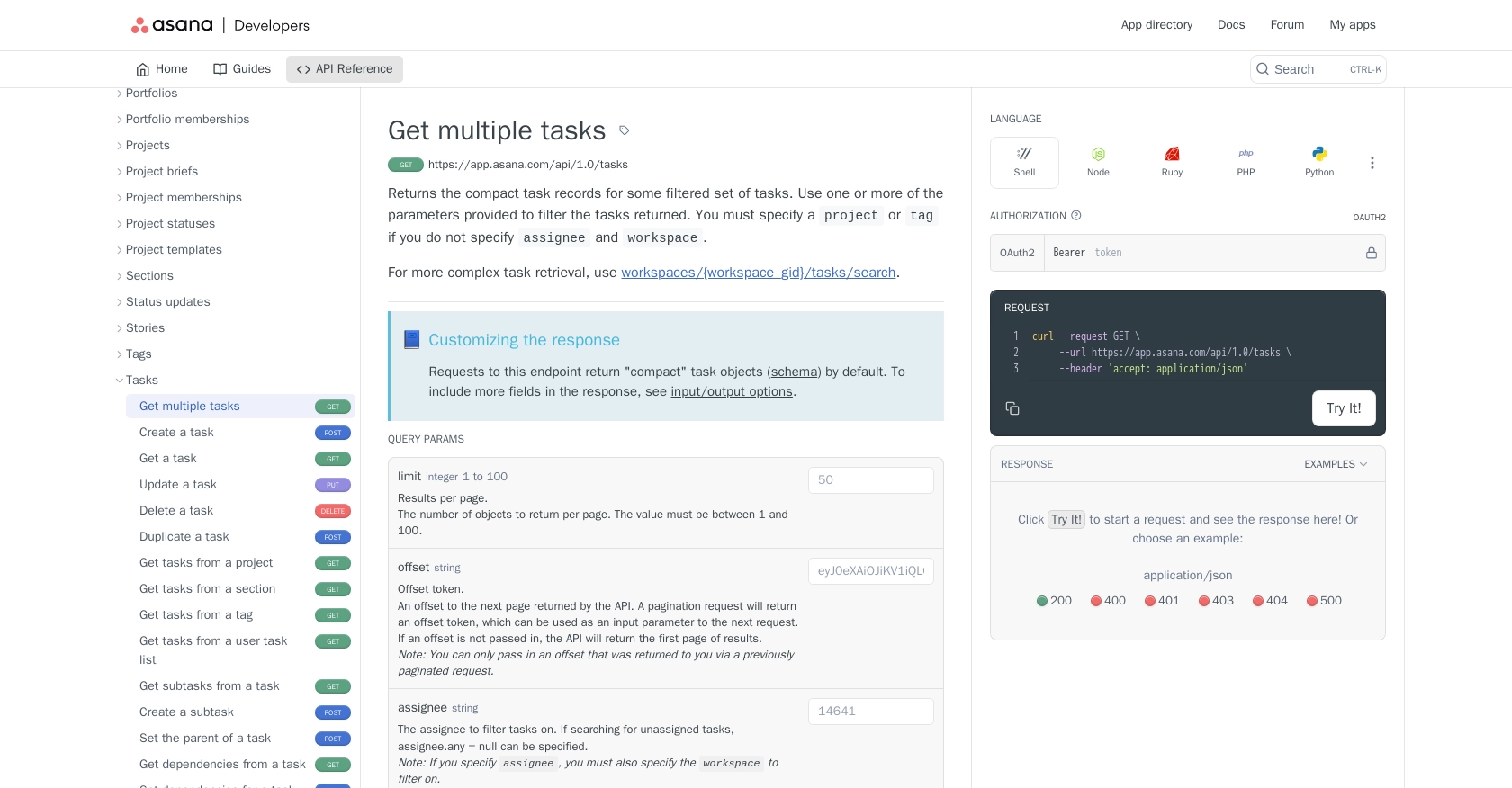
Conclusion and Best Practices for Asana API Integration Using PHP
Integrating with the Asana API using PHP provides a powerful way to automate and enhance your project management workflows. By following the steps outlined in this guide, you can efficiently retrieve tasks and integrate them into your applications, offering real-time insights and improved productivity.
Best Practices for Storing Asana API Credentials Securely
Ensure that your OAuth credentials, such as the client ID, client secret, and access tokens, are stored securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Asana API Rate Limits Effectively
Asana imposes rate limits on API requests to ensure fair usage. Be mindful of these limits and implement strategies to handle them gracefully. For instance, you can implement exponential backoff or retry mechanisms to manage rate limit errors. For more details, refer to the Asana API documentation.
Transforming and Standardizing Asana Task Data
When retrieving tasks from Asana, consider transforming and standardizing the data to fit your application's needs. This may involve mapping Asana task fields to your internal data structures or normalizing data formats for consistency.
Enhancing Integration Capabilities with Endgrate
If you're looking to streamline your integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and leverage an intuitive integration experience for your customers. Learn more about how Endgrate can simplify your integration efforts by visiting Endgrate.
By adhering to these best practices and utilizing tools like Endgrate, you can maximize the efficiency and effectiveness of your Asana API integrations, ultimately enhancing your team's productivity and collaboration.
Read More
Ready to get started?