Using the Nutshell API to Get Contacts in Python
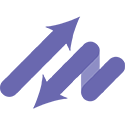
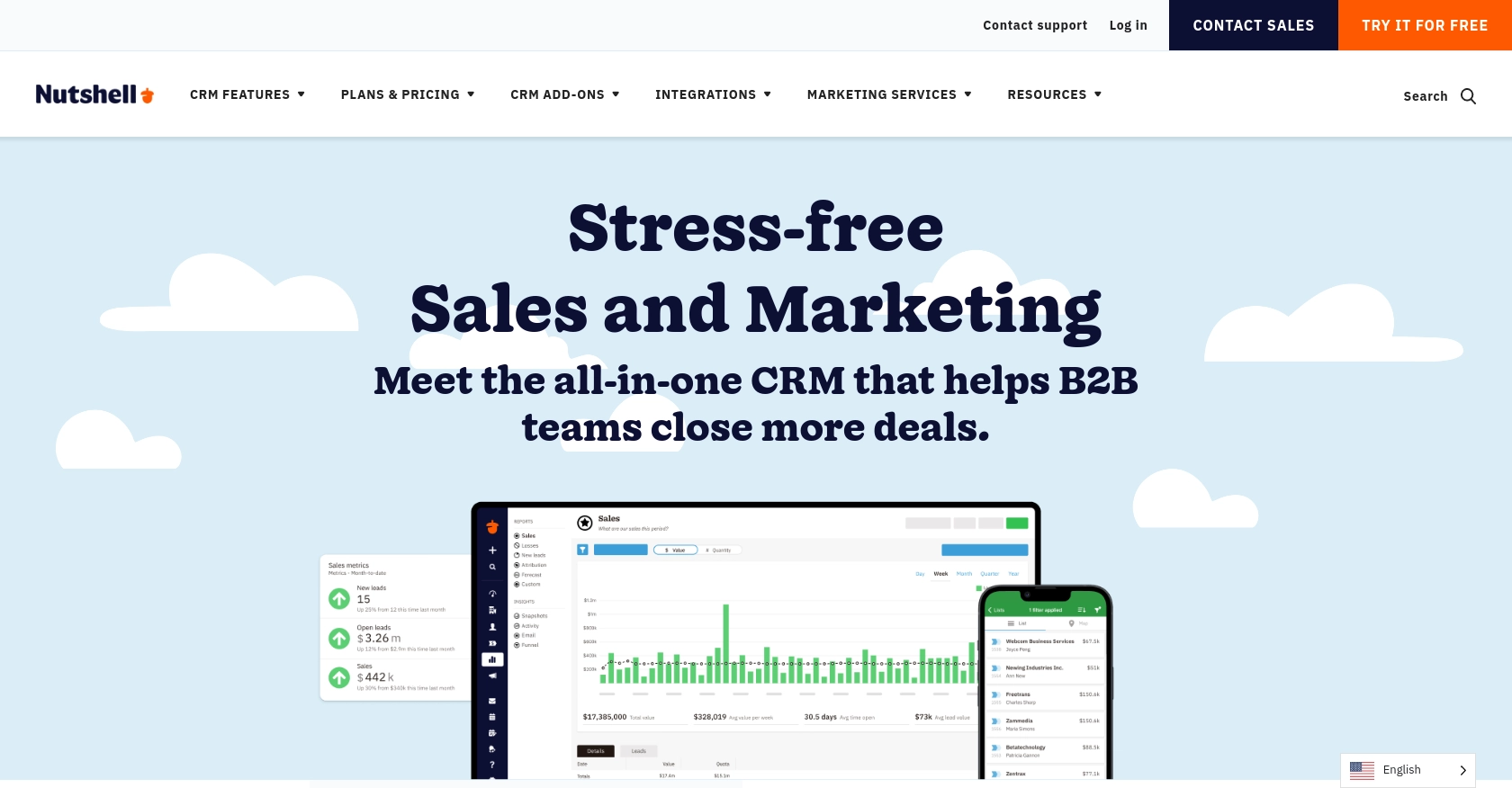
Introduction to Nutshell CRM API
Nutshell is a powerful CRM platform designed to help businesses streamline their sales processes and improve customer relationships. With its user-friendly interface and robust features, Nutshell is a popular choice for companies looking to enhance their sales and customer management efforts.
Developers may want to integrate with the Nutshell API to access and manage customer data, such as contacts, to automate and personalize sales processes. For example, a developer could use the Nutshell API to retrieve contact information and use it to trigger personalized follow-up emails or sales calls based on customer interactions.
This article will guide you through using Python to interact with the Nutshell API, specifically focusing on retrieving contact information. By the end of this tutorial, you will be able to efficiently access and manage contacts within the Nutshell platform using Python.
Setting Up Your Nutshell API Test Account
Before you can start interacting with the Nutshell API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting any live data. Follow these steps to create your test environment:
Creating a Nutshell Account
If you don't already have a Nutshell account, you can sign up for a free trial on the Nutshell website. Follow the on-screen instructions to complete the registration process. Once your account is created, you'll be able to log in and access the Nutshell dashboard.
Generating an API Key for Authentication
The Nutshell API uses HTTP Basic authentication, which requires an API key. Follow these steps to generate your API key:
- Log in to your Nutshell account and navigate to the "Setup" tab.
- Click on "API" to access the API settings.
- Create a new API key by clicking "Add API Key." You may need to specify permissions based on your integration needs.
- Once the API key is generated, make sure to copy and store it securely, as you'll need it for authentication in your API calls.
Understanding Nutshell API Authentication
To authenticate your API requests, you'll use HTTP Basic authentication with your company domain or user email as the username and the API key as the password. Here's an example of how to structure your authentication:
import requests
# Replace with your domain or email and API key
username = 'your_domain_or_email'
api_key = 'your_api_key'
# Example of setting up authentication
auth = (username, api_key)
Ensure that all API requests are made over HTTPS to keep your credentials secure.
For more detailed information on authentication, refer to the Nutshell API documentation.
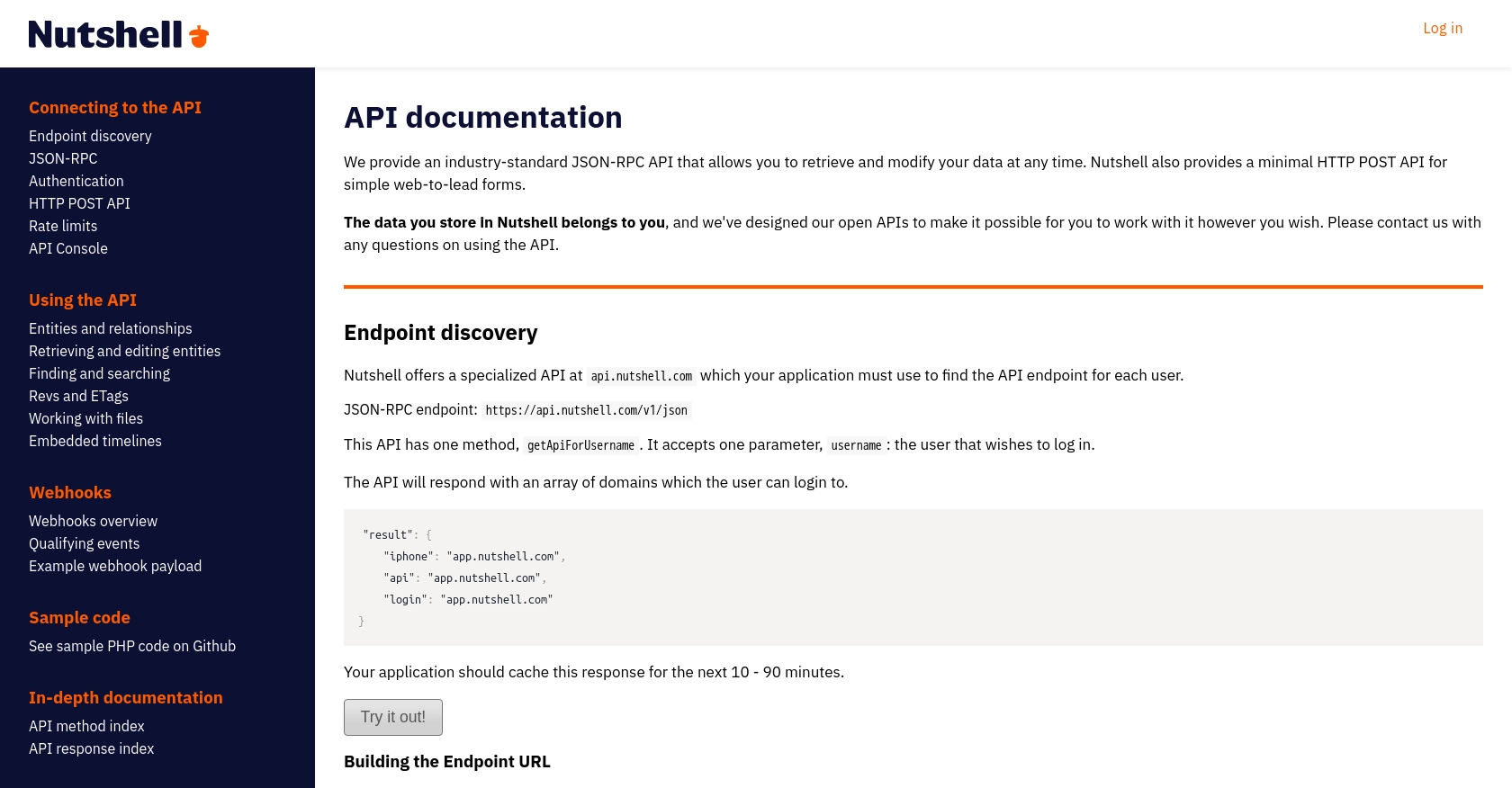
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Nutshell Using Python
Now that you have set up your Nutshell account and generated an API key, it's time to make API calls to retrieve contact information. We'll be using Python to interact with the Nutshell API, leveraging its JSON-RPC protocol to fetch contact data efficiently.
Setting Up Your Python Environment for Nutshell API Integration
Before diving into the code, ensure you have Python 3.x installed on your machine. Additionally, you'll need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Writing Python Code to Fetch Contacts from Nutshell API
Let's create a Python script to retrieve contacts from the Nutshell API. Follow these steps to write the code:
import requests
import json
# Replace with your domain or email and API key
username = 'your_domain_or_email'
api_key = 'your_api_key'
# Set up authentication
auth = (username, api_key)
# Define the Nutshell API endpoint
endpoint = 'https://app.nutshell.com/api/v1/json'
# Create the payload for the JSON-RPC request
payload = {
"method": "findContacts",
"params": {
"orderBy": "id",
"orderDirection": "ASC",
"limit": 10,
"page": 1
},
"id": 1
}
# Make the API call
response = requests.post(endpoint, auth=auth, json=payload)
# Check if the request was successful
if response.status_code == 200:
contacts = response.json().get('result', [])
for contact in contacts:
print(f"Contact ID: {contact['id']}, Name: {contact['name']['displayName']}")
else:
print(f"Failed to retrieve contacts: {response.status_code} - {response.text}")
Understanding the Python Code for Nutshell API Calls
In the code above, we start by importing the necessary libraries and setting up authentication using your Nutshell domain or email and API key. We define the API endpoint and create a JSON-RPC payload to specify the method and parameters for retrieving contacts.
The requests.post
method is used to send the API request, and we check the response status code to ensure the request was successful. If successful, the contacts are printed with their IDs and display names.
Handling Errors and Verifying API Call Success
It's crucial to handle errors gracefully when making API calls. The Nutshell API will return a 401 status code if authentication fails, so ensure your credentials are correct. Additionally, verify the returned data by checking the response in your Nutshell dashboard.
For more information on error codes and handling, refer to the Nutshell API documentation.
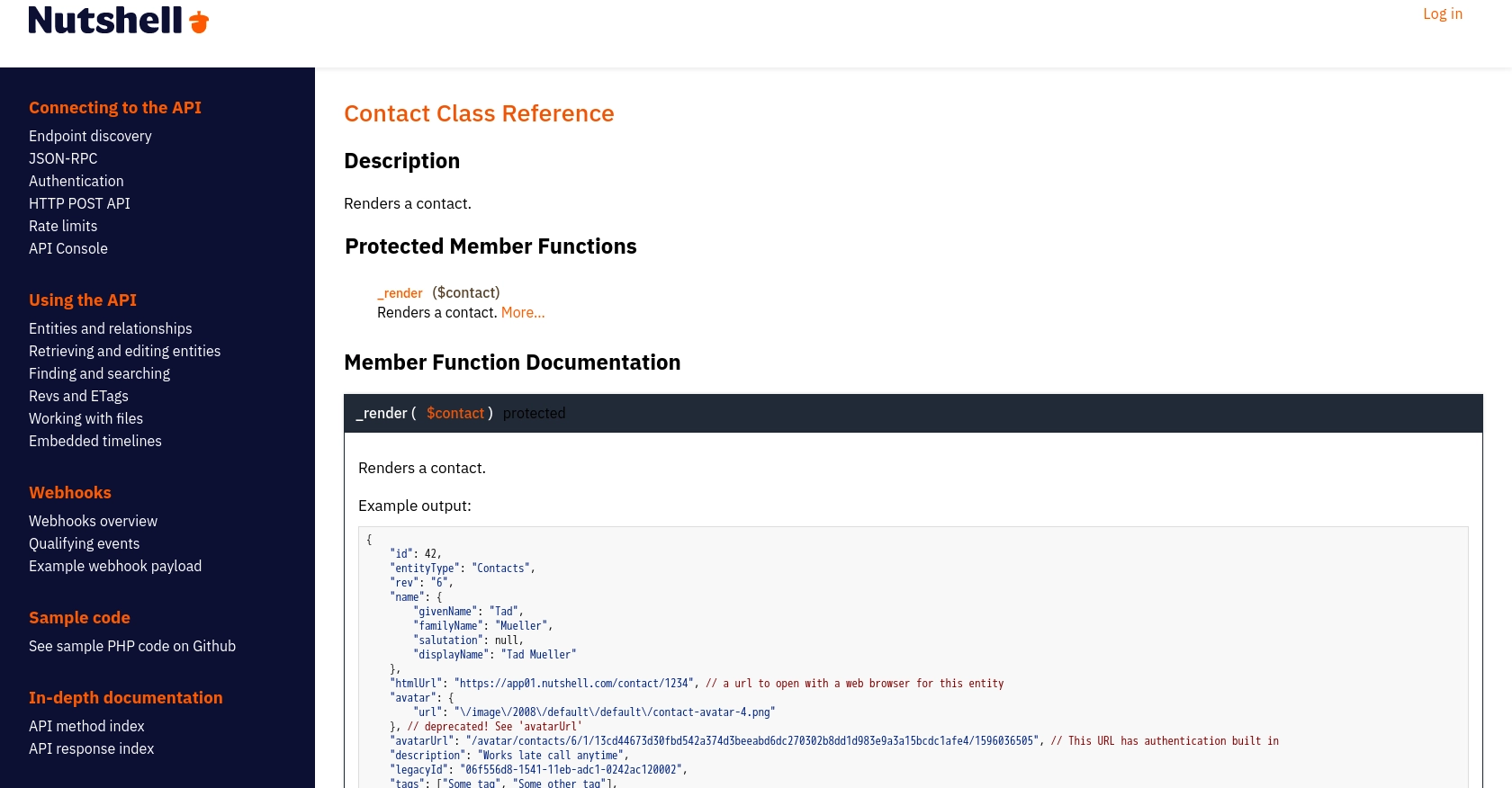
Conclusion and Best Practices for Using the Nutshell API with Python
Integrating with the Nutshell API using Python allows developers to efficiently manage and retrieve contact information, enhancing sales and customer relationship processes. By following the steps outlined in this guide, you can seamlessly connect to the Nutshell platform and automate various tasks.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API keys and credentials securely, using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and API-specific error messages effectively.
Explore More with Endgrate for Seamless Integrations
While integrating with the Nutshell API can be straightforward, managing multiple integrations across various platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple services, including Nutshell.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while ensuring a smooth integration experience for your customers. Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?