Using the Keap API to Create or Update Companies (with PHP examples)
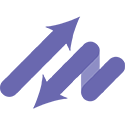
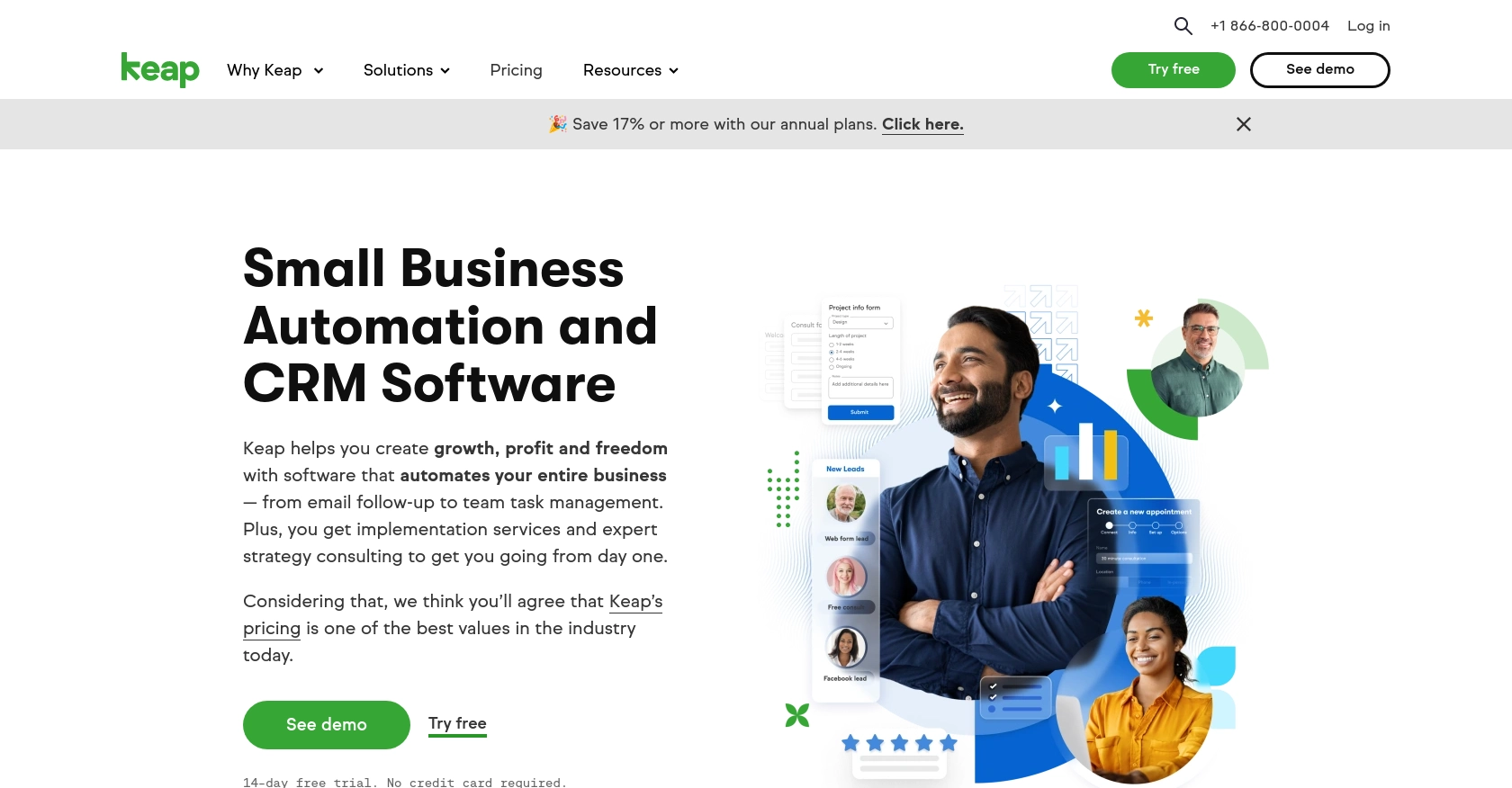
Introduction to Keap API Integration
Keap, formerly known as Infusionsoft, is a powerful CRM platform designed to help small businesses manage customer relationships, automate marketing, and streamline sales processes. With its robust suite of tools, Keap enables businesses to nurture leads, track customer interactions, and enhance overall productivity.
For developers, integrating with Keap's API offers the opportunity to automate and enhance business workflows. By connecting with Keap, developers can create or update company records programmatically, ensuring that customer data is always up-to-date and accessible. For example, a developer might use the Keap API to automatically update company information whenever a new lead is captured from a website form, thereby reducing manual data entry and improving data accuracy.
Setting Up Your Keap Developer Account and Sandbox Environment
Before diving into the Keap API, you'll need to set up a developer account and a sandbox environment. This allows you to test API interactions without affecting live data. Follow these steps to get started:
Register for a Keap Developer Account
To begin, you'll need to create a Keap developer account. This account provides access to Keap's API documentation and tools necessary for integration development.
- Visit the Keap Developer Portal.
- Click on the "Register" button to sign up for a developer account.
- Fill in the required details and submit the registration form.
- Once registered, log in to your developer account to access the dashboard.
Create a Keap Sandbox App
With your developer account set up, the next step is to create a sandbox app. This environment allows you to safely test API calls and integrations.
- Navigate to the "Sandbox" section in your developer dashboard.
- Click on "Create Sandbox App" and provide the necessary information.
- Once created, you'll receive a sandbox app ID and secret, which are essential for API authentication.
Set Up OAuth Authentication for Keap API
Keap uses OAuth 2.0 for authentication, ensuring secure access to API resources. Follow these steps to configure OAuth:
- In your sandbox app settings, locate the "OAuth" section.
- Register a new OAuth client by providing a name and redirect URI.
- After registration, note the client ID and client secret. These credentials are crucial for obtaining access tokens.
With your developer account, sandbox app, and OAuth setup complete, you're ready to start interacting with the Keap API. In the next section, we'll explore how to make API calls using PHP to create or update company records.
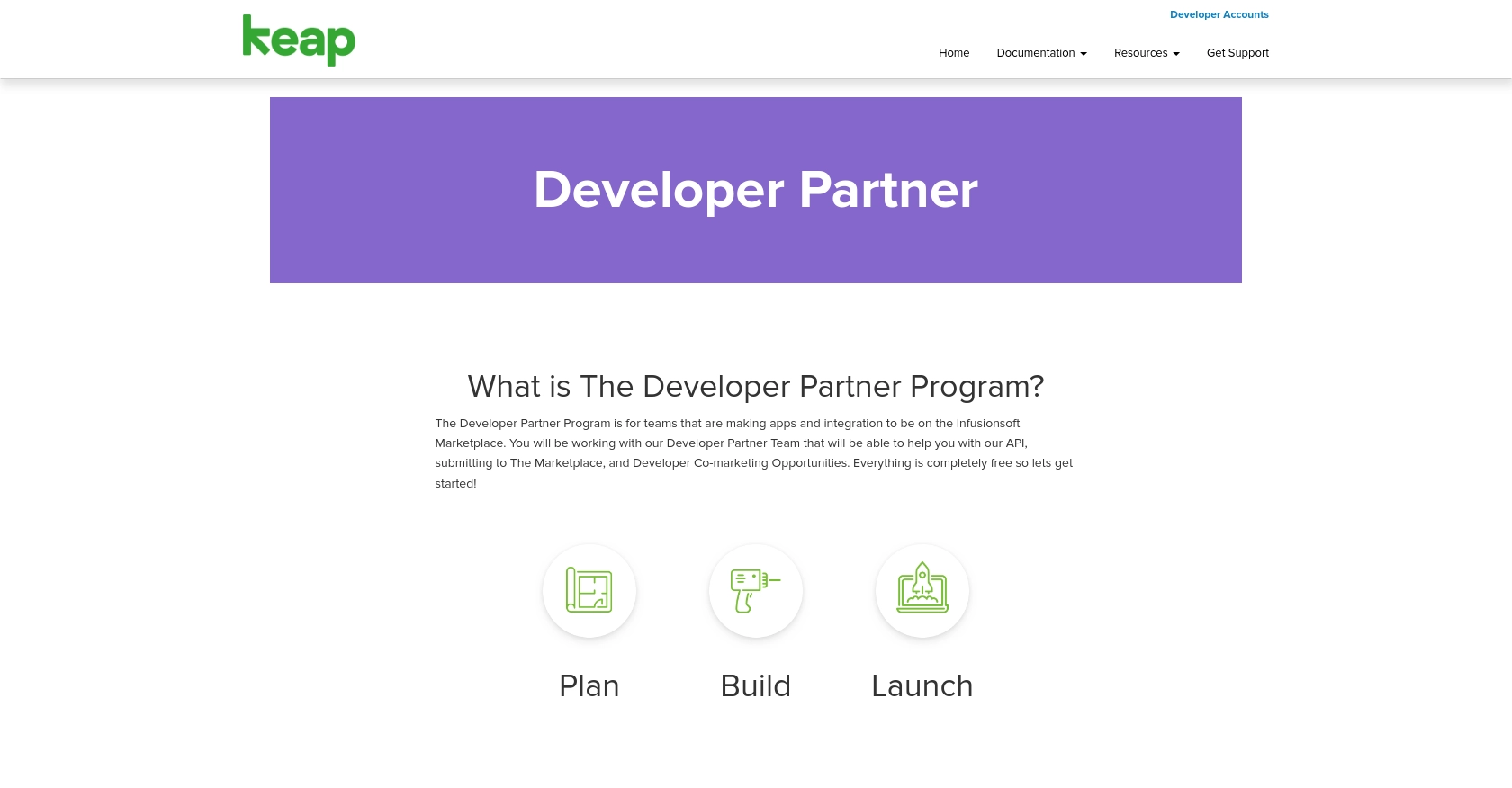
sbb-itb-96038d7
Making API Calls to Create or Update Companies with Keap Using PHP
With your Keap developer account and sandbox environment set up, you can now proceed to make API calls to create or update company records. This section will guide you through the process using PHP, ensuring you have the necessary tools and understanding to interact with the Keap API effectively.
Prerequisites for PHP and Keap API Integration
Before making API calls, ensure you have the following prerequisites installed on your development environment:
- PHP 7.4 or higher
- Composer for dependency management
- The Guzzle HTTP client library for making HTTP requests
To install Guzzle, run the following command in your terminal:
composer require guzzlehttp/guzzle
Creating or Updating Companies with Keap API Using PHP
Now that your environment is ready, you can create or update company records in Keap. Below is a step-by-step guide with example code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle HTTP client
$client = new Client();
// Set the API endpoint for creating or updating companies
$endpoint = 'https://api.infusionsoft.com/crm/rest/v1/companies';
// Set your access token
$accessToken = 'Your_Access_Token';
// Define the company data
$companyData = [
'company_name' => 'Example Company',
'email' => 'contact@example.com',
'phone' => '123-456-7890'
];
try {
// Make the API request
$response = $client->request('POST', $endpoint, [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => $companyData
]);
// Check if the request was successful
if ($response->getStatusCode() === 200) {
echo "Company created or updated successfully.";
} else {
echo "Failed to create or update company.";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the access token obtained during the OAuth setup. The code above initializes a Guzzle client, sets the API endpoint, and sends a POST request with the company data. If successful, it confirms the creation or update of the company.
Verifying API Call Success in Keap Sandbox
To verify that your API call was successful, log in to your Keap sandbox account and navigate to the companies section. You should see the newly created or updated company record reflecting the data you provided in the API call.
Handling Errors and Common Error Codes
When interacting with the Keap API, it's crucial to handle potential errors gracefully. Common error codes include:
- 400 Bad Request: The request was invalid. Check your data and try again.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
Implement error handling in your code to manage these scenarios effectively and ensure a robust integration.
Best Practices for Keap API Integration
When integrating with the Keap API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth client ID, client secret, and access tokens securely. Use environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be aware of Keap's API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are consistent and standardized across your application to prevent data discrepancies.
Enhance Your Integration with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. With Endgrate, you can streamline this process by leveraging a unified API endpoint that connects to various platforms, including Keap.
Endgrate allows you to focus on your core product by outsourcing integrations, saving both time and resources. By building once for each use case, you can provide an intuitive integration experience for your customers without the hassle of managing multiple APIs.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?