How to Create Clients with the Applied Epic API in Python
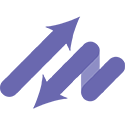
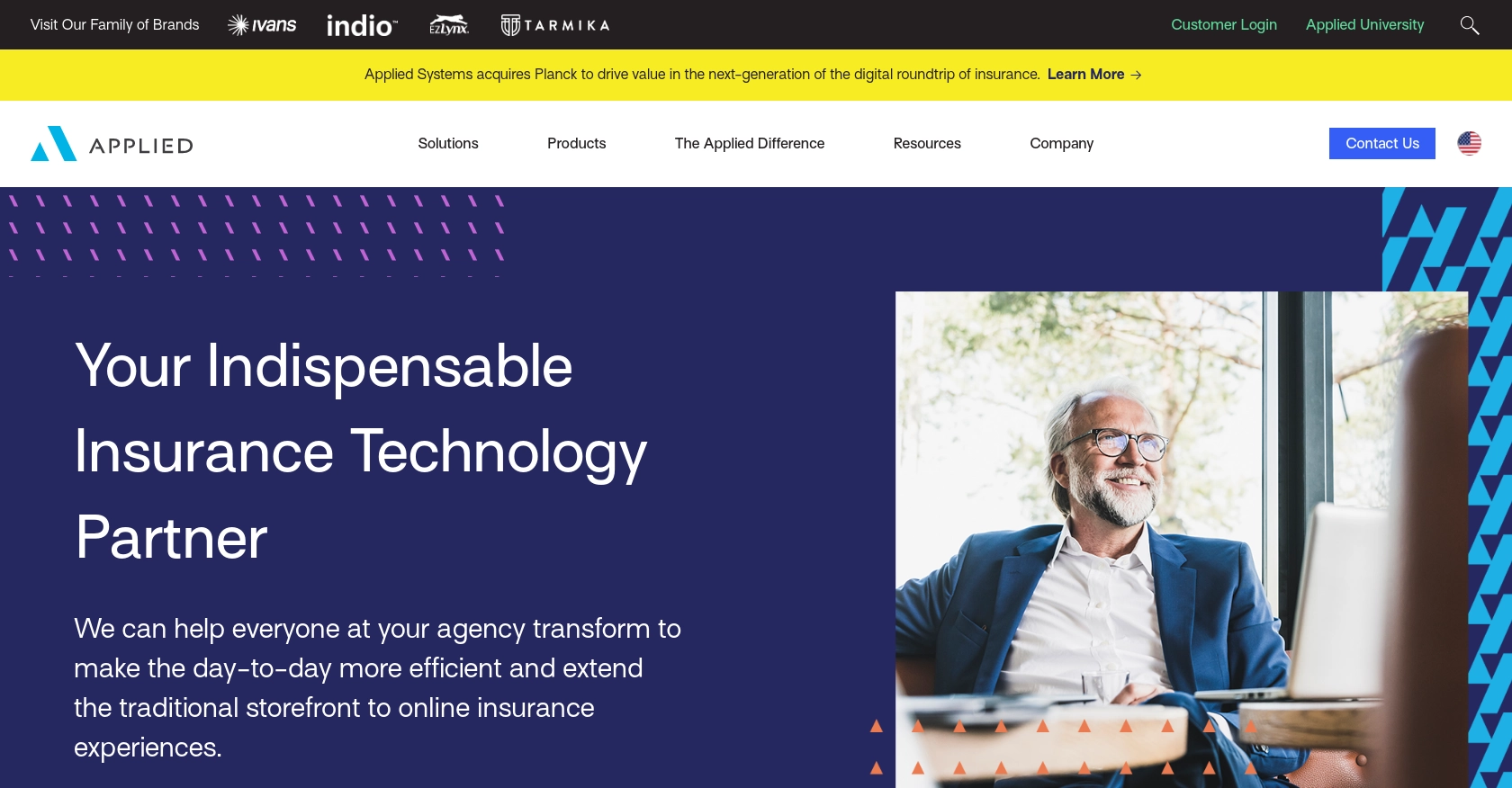
Introduction to Applied Epic API
Applied Epic is a comprehensive management system designed for insurance agencies to streamline their operations and improve client management. It offers a wide range of features that help agencies manage policies, claims, and customer interactions efficiently.
Integrating with the Applied Epic API allows developers to automate and enhance client management processes. For example, using the API, a developer can create new client records programmatically, ensuring that client information is consistently updated across systems. This can be particularly useful for agencies looking to automate client onboarding or synchronize data between different platforms.
Setting Up Your Applied Epic Test Account
Before you can start interacting with the Applied Epic API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Applied Epic provides developers with a sandbox environment to facilitate testing and development.
Creating an Applied Epic Developer Account
To begin, you'll need to sign up for a developer account with Applied Epic. Follow these steps:
- Visit the Applied Epic Developer Portal.
- Complete the registration form with your details and submit it.
- Once registered, log in to your developer account to access the sandbox environment.
Generating API Credentials for Applied Epic
With your developer account set up, the next step is to generate the necessary API credentials. These credentials will allow you to authenticate your API requests.
- Navigate to the API section in your developer dashboard.
- Create a new application to obtain your client ID and client secret.
- Ensure you have the appropriate permissions set for client management, such as
epic/clients:write
andepic/clients:all
.
Authenticating with Custom Authentication
The Applied Epic API uses a custom authentication method. Follow these steps to authenticate your requests:
- Use your client ID and client secret to obtain an access token.
- Include the access token in the Authorization header of your API requests.
For more detailed information on authentication, refer to the Applied Epic API Documentation.
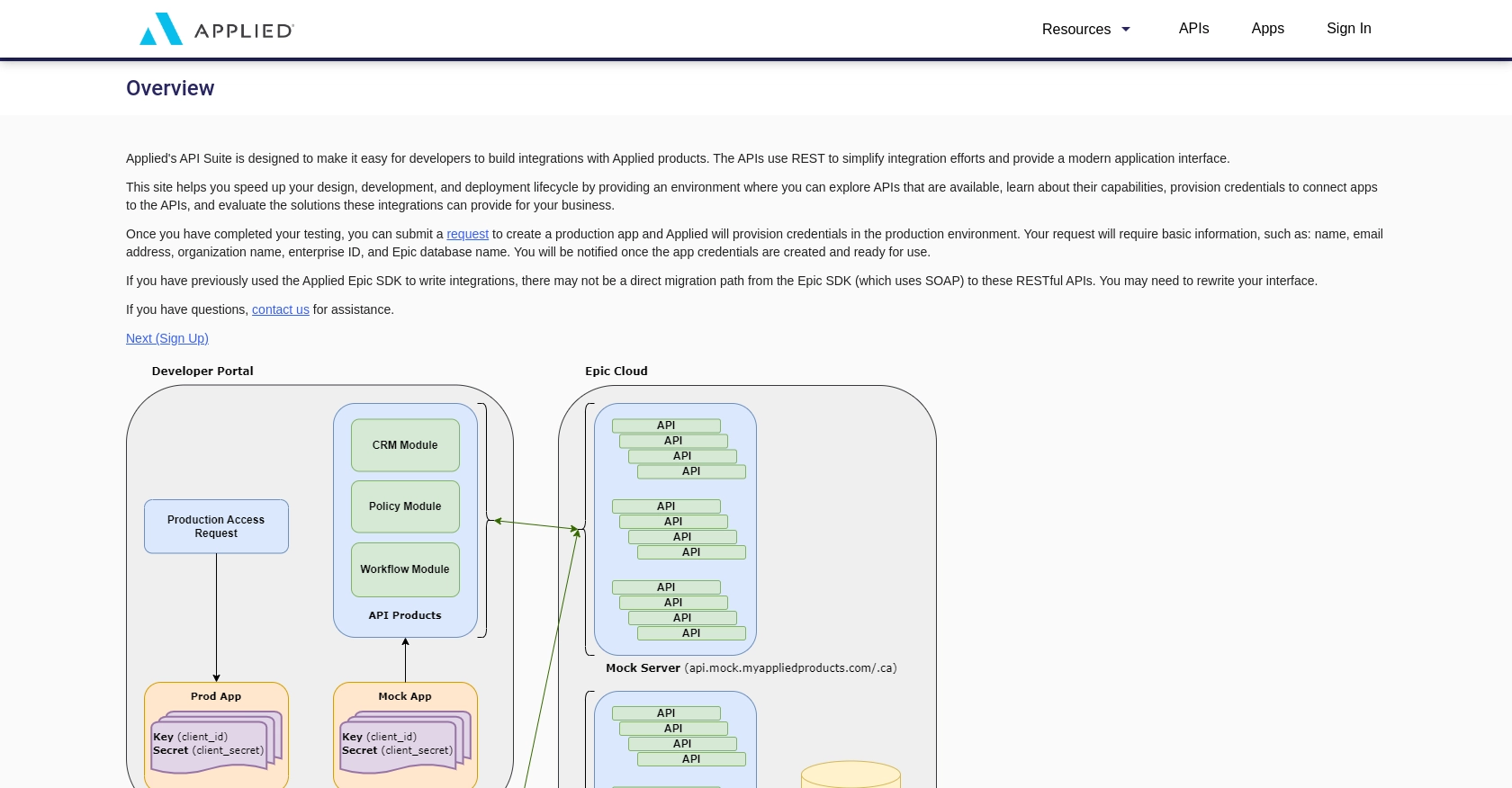
sbb-itb-96038d7
Making API Calls to Create Clients with Applied Epic API in Python
To interact with the Applied Epic API and create clients, you'll need to use Python to send HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your Python Environment for Applied Epic API
Before making API calls, ensure your Python environment is ready. You'll need Python 3.x and the requests
library to handle HTTP requests.
- Ensure Python 3.x is installed on your machine. You can download it from the official Python website.
- Install the
requests
library using pip:
pip install requests
Writing Python Code to Create a Client with Applied Epic API
Now, let's write a Python script to create a client using the Applied Epic API. We'll use the requests.post
method to send a POST request to the API endpoint.
import requests
# Set the API endpoint
url = "https://api.myappliedproducts.com/crm/v1/clients"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the client data
client_data = {
"individualOrBusiness": "INDIVIDUAL",
"businessTypes": ["PERSONAL"],
"name": "Alice Smith",
"lookupCode": "SMITALI-01",
"type": "INSURED",
"accountSource": {
"id": "65536",
"description": "Cold Call"
},
"address": {
"streets": ["123 Main St."],
"city": "Schenectady",
"zipOrPostalCode": "12345",
"stateOrProvince": "NY",
"countryCode": "USA"
},
"contact": {
"prefix": "Ms.",
"firstName": "Alice",
"lastName": "Smith",
"email": [{
"emailAddress": "AliceSmith@wahoo.com",
"isPrimary": True
}]
}
}
# Send the POST request
response = requests.post(url, json=client_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Client created successfully.")
else:
print(f"Failed to create client. Status code: {response.status_code}")
print(response.json())
Replace Your_Access_Token
with the access token obtained during authentication. The code above sets up the necessary headers and client data, sends the POST request, and checks the response status.
Verifying the API Call Success in Applied Epic Sandbox
After running the script, verify the success of the API call by checking the Applied Epic sandbox environment. The newly created client should appear in the client list.
Handling Errors and Understanding Applied Epic API Response Codes
When making API calls, it's crucial to handle potential errors. The Applied Epic API may return various status codes:
- 201 Created: The client was successfully created.
- 400 Bad Request: The request was malformed. Check the request data.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 403 Forbidden: You do not have permission to perform this action.
Refer to the Applied Epic API Documentation for more details on error handling.
Conclusion and Best Practices for Using Applied Epic API in Python
Integrating with the Applied Epic API can significantly enhance the efficiency of client management processes within insurance agencies. By automating tasks such as client creation, developers can ensure data consistency and streamline operations across various platforms.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API credentials, such as client ID and secret, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the API to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Data Standardization: Ensure that data fields are standardized across systems to maintain consistency. This can help in seamless data integration and reporting.
- Error Handling: Implement robust error handling to manage different response codes effectively. Log errors for troubleshooting and ensure that your application can gracefully handle failures.
Streamline Your Integration Process with Endgrate
While building integrations with the Applied Epic API can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Applied Epic. This allows developers to focus on core product development while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
Ready to get started?