Using the Zoho CRM API to Create Custom Objects (with Python examples)
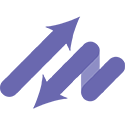
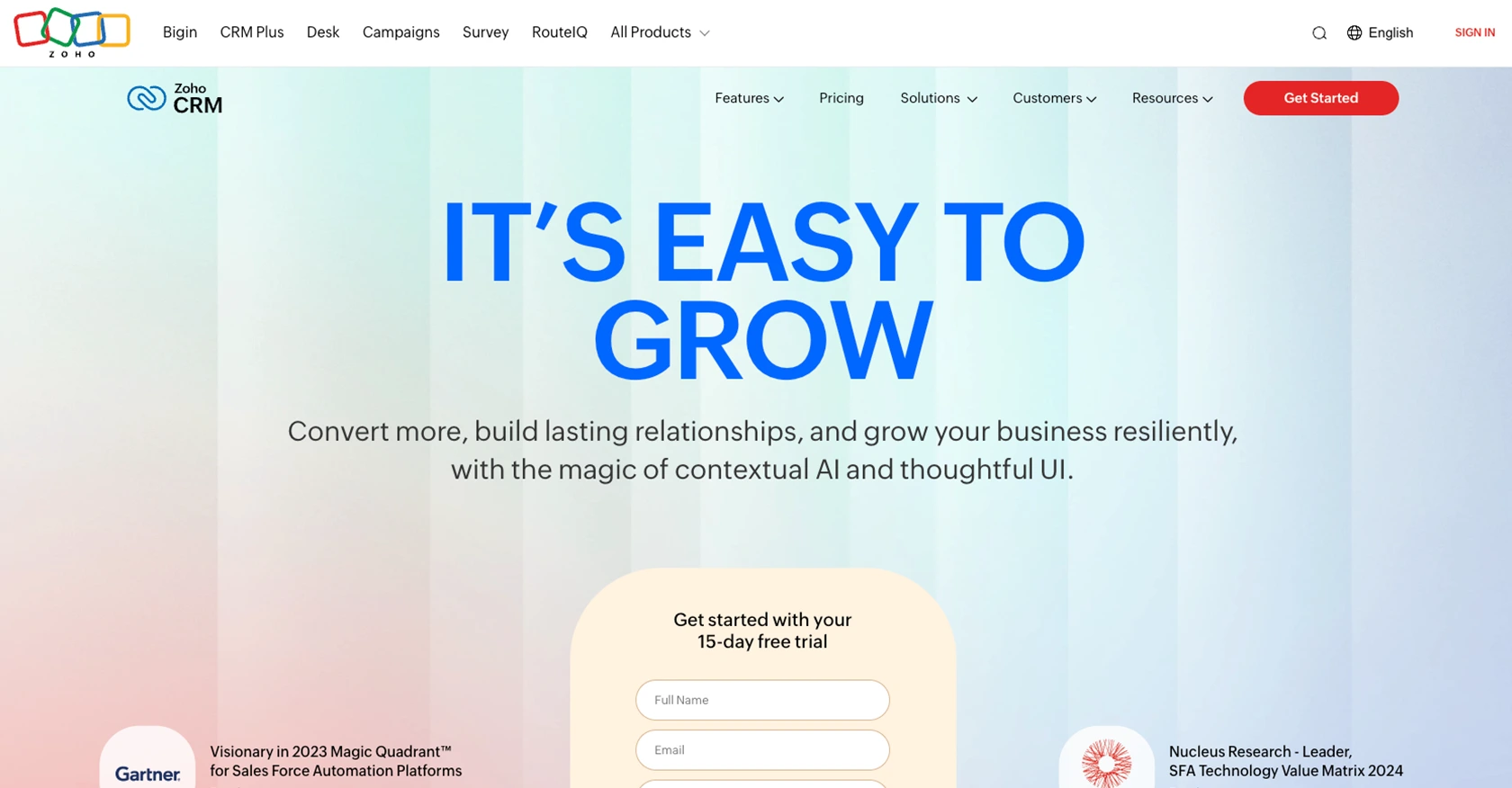
Introduction to Zoho CRM API
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in one unified system. Known for its flexibility and extensive features, Zoho CRM is a popular choice for businesses looking to enhance their customer engagement and streamline operations.
Developers often integrate with the Zoho CRM API to automate and customize CRM functionalities. For example, creating custom objects using the Zoho CRM API can help tailor the CRM to specific business needs, such as tracking unique customer interactions or managing specialized sales processes.
This article will guide you through using Python to create custom objects in Zoho CRM, providing step-by-step instructions and code examples to simplify the integration process.
Setting Up Your Zoho CRM Sandbox Account for API Integration
Before you can start creating custom objects in Zoho CRM using the API, you need to set up a sandbox account. This allows you to test and develop your integration without affecting your live data.
Step 1: Register for a Zoho CRM Developer Account
If you don't already have a Zoho CRM account, you'll need to register for one. Visit the Zoho CRM signup page and create a free account. If you already have an account, simply log in.
Step 2: Access the Zoho Developer Console
Once logged in, navigate to the Zoho Developer Console. This is where you can manage your API integrations and sandbox environment.
Step 3: Create a Sandbox Environment
In the Developer Console, select the option to create a new sandbox environment. This will allow you to test your API calls without impacting your production data.
Step 4: Register Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication. Follow these steps to register your application:
- In the Developer Console, choose "Register Client".
- Select "Web Based" as the client type.
- Enter the required details such as Client Name, Homepage URL, and Authorized Redirect URIs.
- Click "Create" to generate your Client ID and Client Secret.
For more detailed instructions, refer to the Zoho CRM OAuth Overview.
Step 5: Generate Access and Refresh Tokens
With your Client ID and Client Secret, you can now generate access and refresh tokens:
- Make an authorization request to Zoho's OAuth server.
- Use the authorization code received to request access and refresh tokens.
Ensure you securely store these tokens, as they are needed for authenticating API requests. For more details, see the Zoho CRM OAuth Documentation.
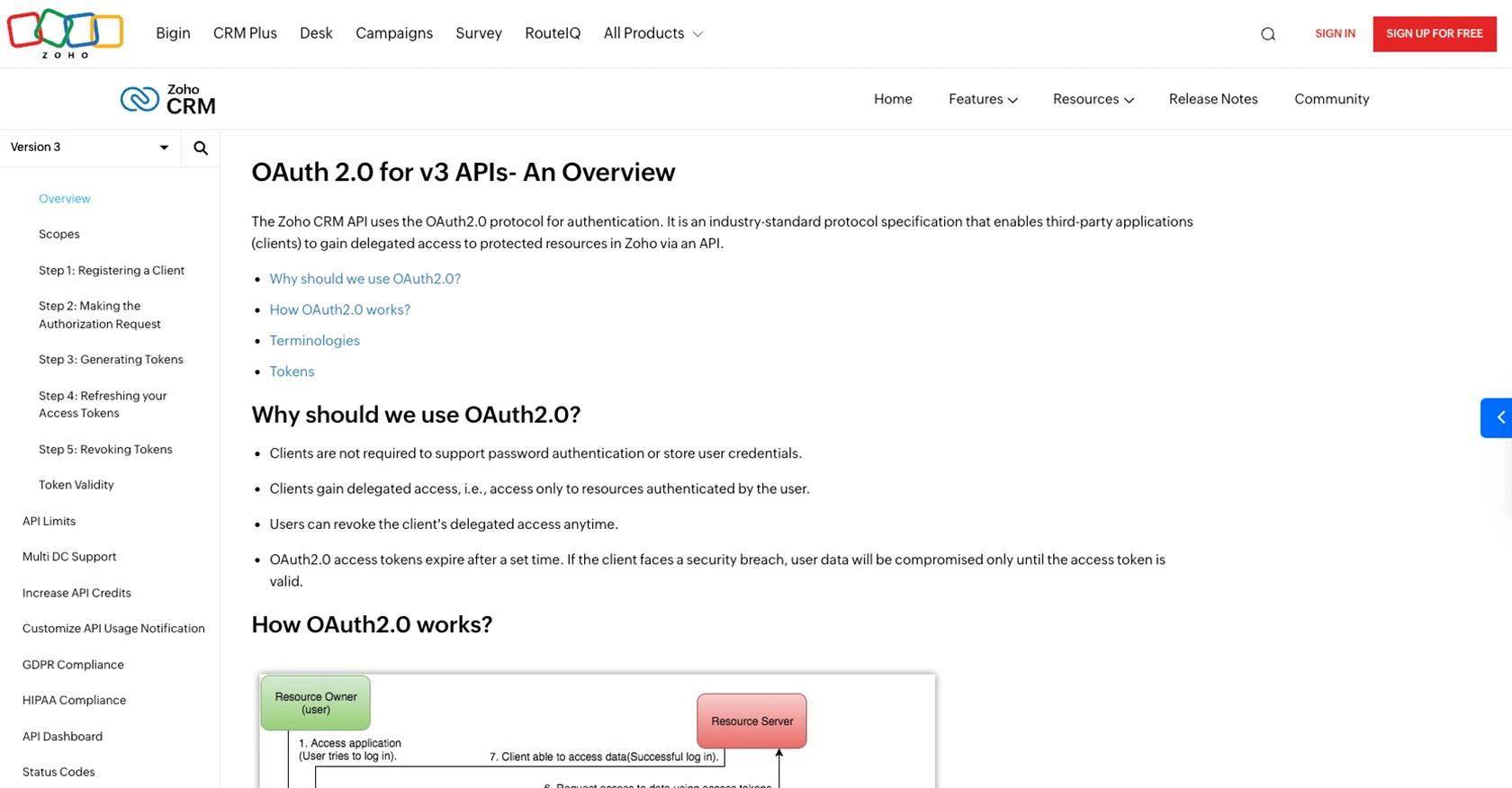
sbb-itb-96038d7
How to Make API Calls to Create Custom Objects in Zoho CRM Using Python
To interact with the Zoho CRM API and create custom objects, you'll need to use Python. This section will guide you through setting up your environment, writing the necessary code, and handling responses and errors effectively.
Setting Up Your Python Environment for Zoho CRM API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
pip install requests
Writing Python Code to Create Custom Objects in Zoho CRM
Follow these steps to write a Python script that creates custom objects in Zoho CRM:
- Create a new Python file named
create_custom_object.py
. - Import the
requests
library to handle HTTP requests. - Set up the API endpoint URL for creating custom objects. Replace
MODULE_HERE
with your custom module's API name. - Include the necessary headers, including the authorization token.
- Define the data for the custom object you want to create.
- Send a POST request to the Zoho CRM API.
- Handle the response to verify the success of the operation.
import requests
# Define the API endpoint and headers
url = "https://www.zohoapis.com/crm/v3/MODULE_HERE"
headers = {
"Authorization": "Zoho-oauthtoken Your_Access_Token",
"Content-Type": "application/json"
}
# Define the custom object data
data = {
"data": [
{
"Custom_Field_1": "Value1",
"Custom_Field_2": "Value2"
}
]
}
# Make the POST request to create the custom object
response = requests.post(url, json=data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Custom object created successfully.")
else:
print("Failed to create custom object:", response.json())
Verifying the Success of Your API Call in Zoho CRM
After running your script, verify the creation of the custom object in your Zoho CRM sandbox account. Navigate to the custom module to ensure the object appears as expected.
Handling Errors and Understanding Zoho CRM API Error Codes
When making API calls, it's crucial to handle potential errors. Zoho CRM API provides various error codes to help diagnose issues:
- 400 Bad Request: Invalid input data or parameters.
- 401 Unauthorized: Invalid or expired access token.
- 403 Forbidden: Insufficient permissions to perform the operation.
- 404 Not Found: Incorrect API endpoint or module name.
- 500 Internal Server Error: Unexpected server error. Contact support if this persists.
For a complete list of error codes, refer to the Zoho CRM API Status Codes Documentation.
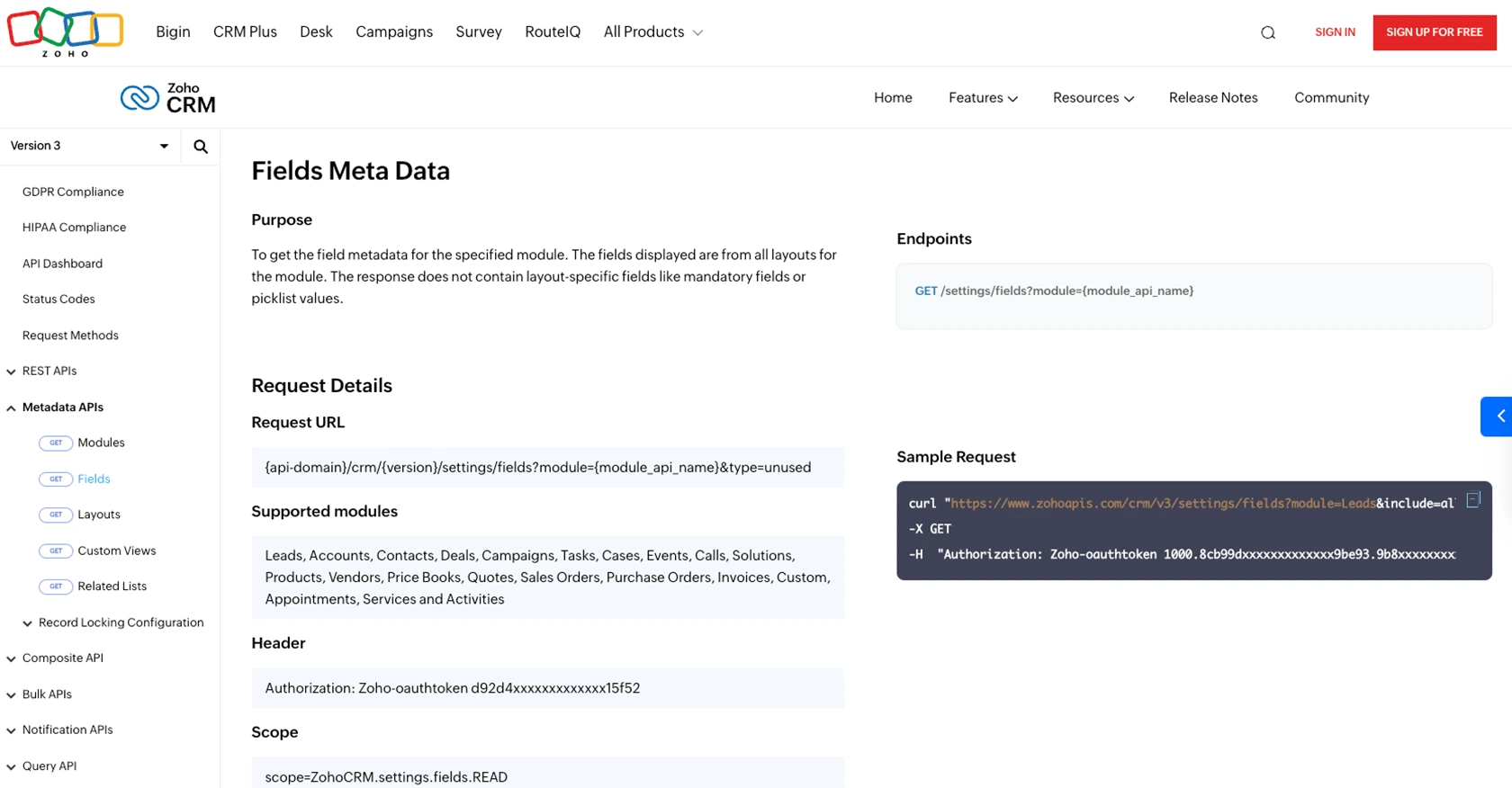
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API to create custom objects can significantly enhance your CRM's capabilities, allowing you to tailor it to your specific business needs. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth 2.0, and execute API calls using Python.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Token Storage: Always store your access and refresh tokens securely. Avoid exposing them in public repositories or client-side code.
- Handle Rate Limiting: Zoho CRM imposes API rate limits. Ensure your application handles these limits gracefully to avoid disruptions. For more details, refer to the API Limits Documentation.
- Data Standardization: Consistently format and validate data before sending it to the API to prevent errors and ensure data integrity.
- Error Handling: Implement robust error handling to manage API responses and retry logic for transient errors.
Streamline Your Integration Process with Endgrate
While integrating with Zoho CRM can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM. This allows you to focus on your core product while Endgrate handles the intricacies of integration.
Explore how Endgrate can save you time and resources by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/module-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?