Using the Salesflare API to Create or Update Accounts in PHP
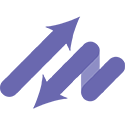
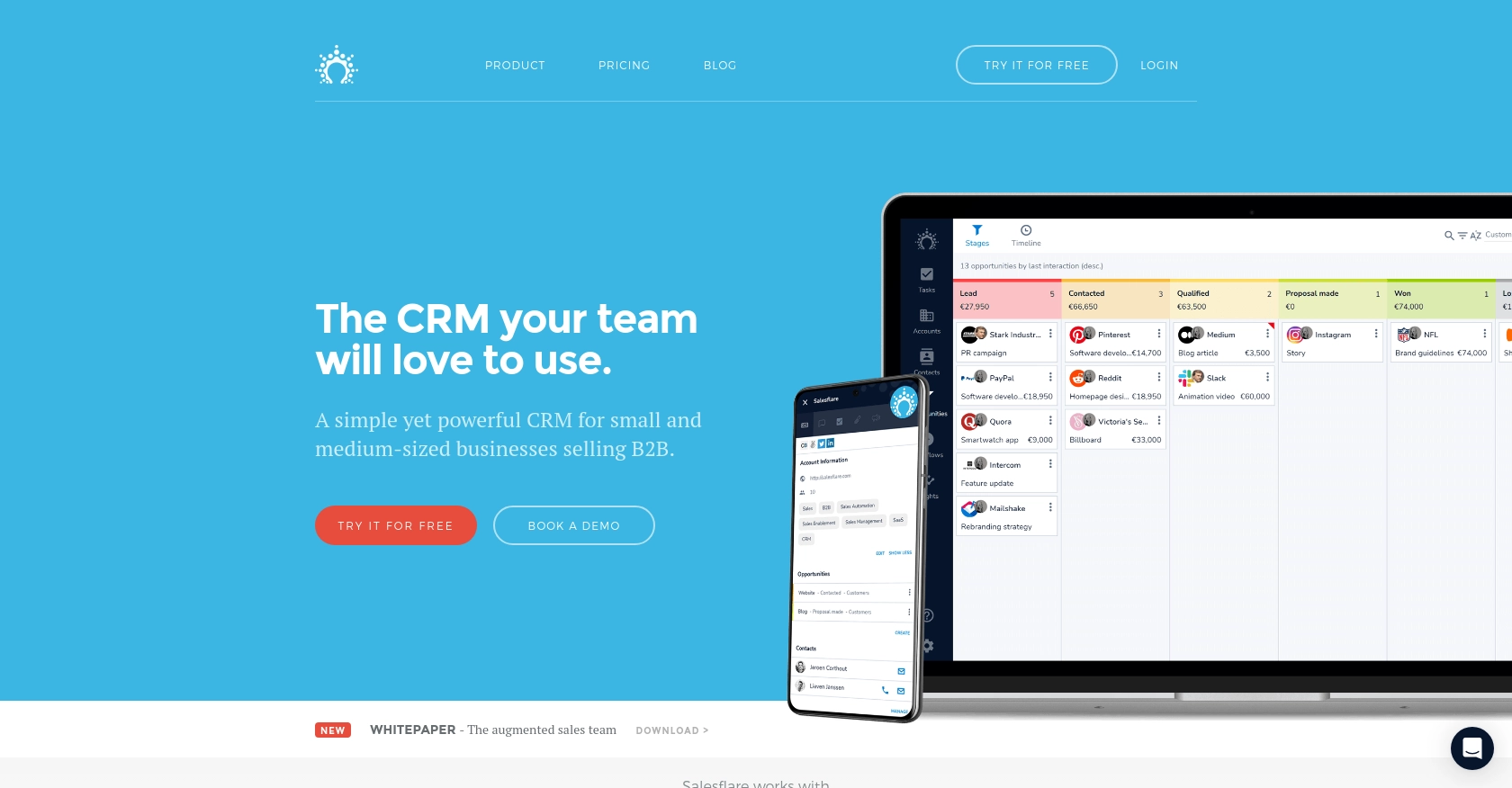
Introduction to Salesflare API Integration
Salesflare is a powerful CRM platform designed to simplify sales processes for small and medium-sized businesses. It offers a user-friendly interface and a range of features to help sales teams manage leads, track customer interactions, and automate follow-ups.
Integrating with Salesflare's API allows developers to enhance their applications by seamlessly creating or updating account information within the Salesflare CRM. For example, a developer might want to automatically update account details from an external database to ensure that sales teams have the most current information at their fingertips.
Setting Up Your Salesflare Test Account for API Integration
Before you can start integrating with the Salesflare API, you'll need to set up a test account. This allows you to safely experiment with API calls without affecting live data. Salesflare offers a straightforward process to get started with their platform.
Creating a Salesflare Account
If you don't already have a Salesflare account, you can sign up for a free trial on their website. This trial provides access to all the features you'll need for testing and development purposes.
- Visit the Salesflare website and click on the "Try for Free" button.
- Follow the on-screen instructions to create your account. You'll need to provide basic information such as your name, email address, and company details.
- Once your account is set up, you can log in to the Salesflare dashboard.
Generating an API Key for Salesflare
Salesflare uses API key-based authentication to secure API requests. Here's how you can generate your API key:
- Log in to your Salesflare account and navigate to the settings section.
- Look for the "API" or "Integrations" tab in the settings menu.
- Click on "Generate API Key" to create a new key. Make sure to store this key securely, as it will be used to authenticate your API requests.
Configuring Your Development Environment
With your Salesflare account and API key ready, you can now configure your development environment to start making API calls. Ensure you have the necessary tools and libraries installed for PHP development.
- Ensure you have PHP installed on your machine. You can download it from the official PHP website.
- Install any required PHP libraries for making HTTP requests, such as cURL or Guzzle.
With these steps completed, you're ready to begin integrating with the Salesflare API using PHP. In the next section, we'll explore how to make API calls to create or update accounts within Salesflare.
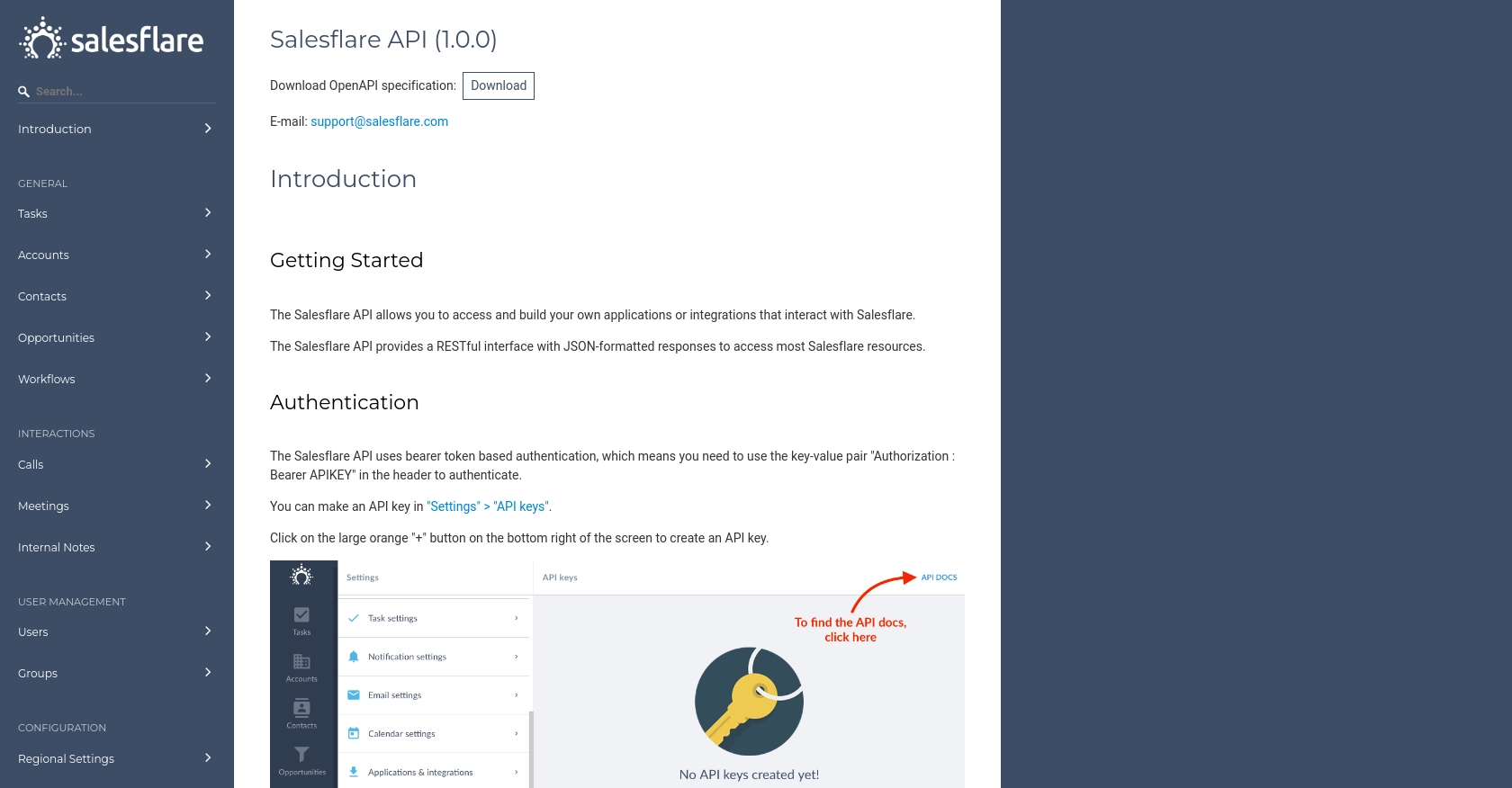
sbb-itb-96038d7
Making API Calls to Create or Update Accounts in Salesflare Using PHP
To effectively interact with the Salesflare API and manage account information, you'll need to set up your PHP environment and write code to perform API requests. This section will guide you through the process of making API calls to create or update accounts in Salesflare using PHP.
Setting Up Your PHP Environment for Salesflare API Integration
Before diving into the code, ensure your PHP environment is ready for API interaction. You'll need PHP installed on your machine, along with necessary libraries for making HTTP requests.
- Download and install PHP from the official PHP website.
- Install the Guzzle library, a popular PHP HTTP client, using Composer. Run the following command in your terminal:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update Accounts in Salesflare
With your environment set up, you can now write the PHP code to interact with the Salesflare API. The following example demonstrates how to create or update an account using the API key for authentication.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_Salesflare_API_Key'; // Replace with your actual API key
// Define the API endpoint for creating or updating accounts
$endpoint = 'https://api.salesflare.com/accounts';
// Prepare the account data
$accountData = [
'name' => 'Example Company',
'email' => 'contact@example.com',
'phone' => '123-456-7890'
];
try {
// Make a POST request to the Salesflare API
$response = $client->request('POST', $endpoint, [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Content-Type' => 'application/json'
],
'json' => $accountData
]);
// Check if the request was successful
if ($response->getStatusCode() === 200) {
echo "Account created or updated successfully.";
} else {
echo "Failed to create or update account.";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
In this code, we use the Guzzle HTTP client to send a POST request to the Salesflare API. The API key is included in the request headers for authentication. The account data is sent as a JSON payload. If the request is successful, you'll receive a confirmation message.
Verifying API Call Success in Salesflare
After running the PHP script, verify that the account was created or updated in your Salesflare test account. Log in to your Salesflare dashboard and check the accounts section to ensure the changes are reflected.
Handling Errors and Troubleshooting
When working with API calls, it's crucial to handle potential errors gracefully. The example code includes basic error handling using a try-catch block. If an error occurs, the exception message will be displayed, helping you troubleshoot the issue.
For more detailed error information, refer to the Salesflare API documentation.
Conclusion and Best Practices for Salesflare API Integration in PHP
Integrating with the Salesflare API using PHP provides a robust solution for automating account management within your CRM. By following the steps outlined in this guide, you can efficiently create or update accounts, ensuring your sales team has access to the most accurate and up-to-date information.
Best Practices for Secure and Efficient API Integration
- Secure API Keys: Always store your API keys securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Salesflare API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized before sending them to the API. This helps maintain consistency and reduces the likelihood of errors during data processing.
- Error Handling: Implement comprehensive error handling to manage exceptions and provide meaningful feedback. This will aid in troubleshooting and ensure a smooth integration experience.
Enhance Your Integration Strategy with Endgrate
While integrating with individual APIs like Salesflare can be effective, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including Salesflare.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Explore how Endgrate can streamline your integration efforts and provide an intuitive experience for your customers by visiting Endgrate's website.
Read More
Ready to get started?