How to Get Calendar Events with the Google Calendar API in Python
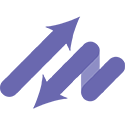
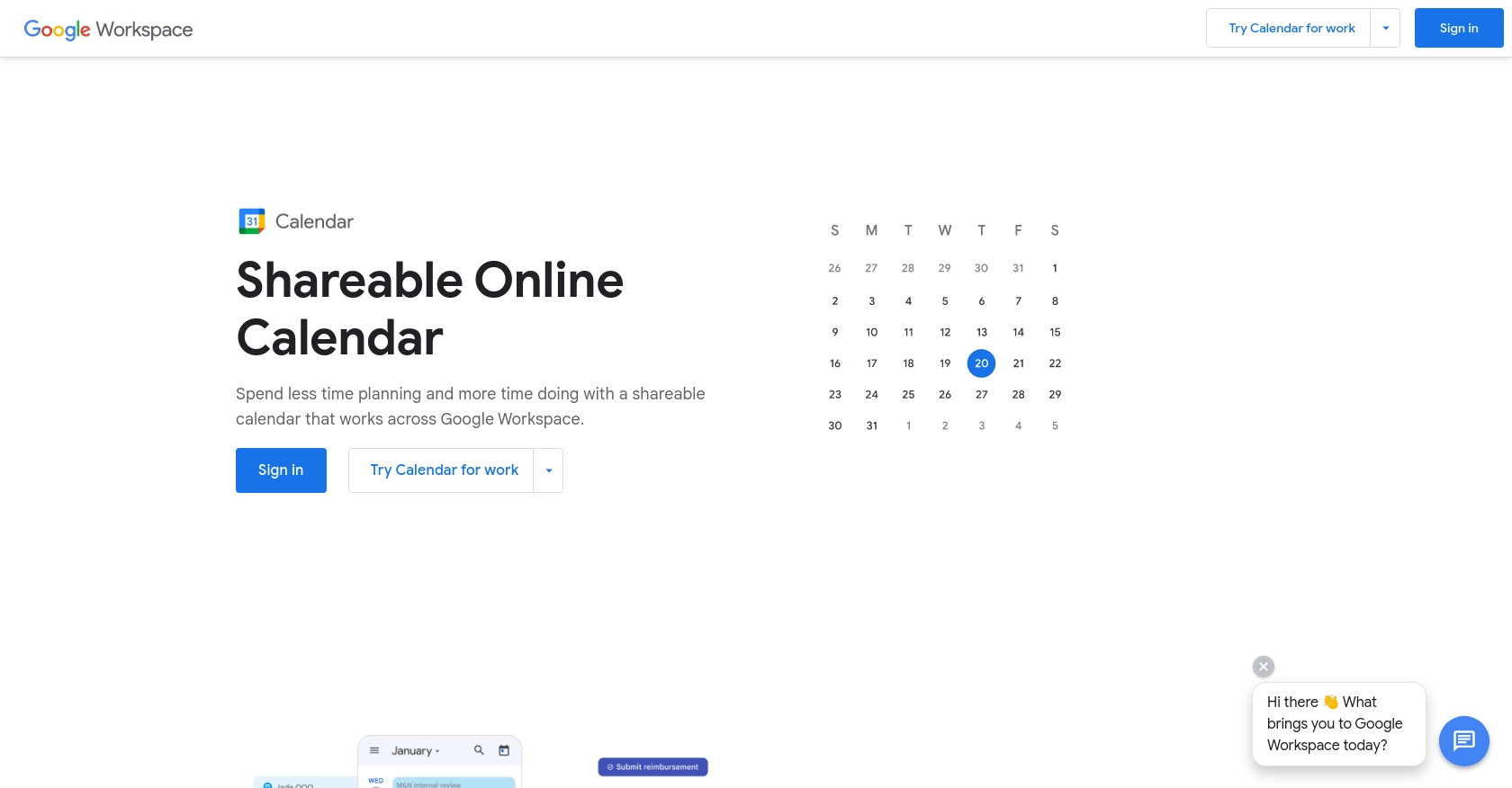
Introduction to Google Calendar API
Google Calendar is a widely used time-management and scheduling service that allows users to create and edit events. It is an essential tool for both personal and professional use, helping individuals and teams stay organized and manage their time effectively.
Integrating with the Google Calendar API enables developers to access and manipulate calendar events programmatically. This can be particularly useful for applications that require scheduling functionalities, such as booking systems or productivity tools. For example, a developer might use the Google Calendar API to automatically retrieve and display upcoming events in a custom dashboard, enhancing user experience by providing real-time scheduling information.
Setting Up Your Google Calendar API Test Account
Before you can start interacting with the Google Calendar API, you'll need to set up a Google Cloud project and configure OAuth 2.0 credentials. This setup allows your application to authenticate and access the Google Calendar API securely.
Create a Google Cloud Project
- Go to the Google Cloud Console.
- Click on the menu icon and navigate to IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Calendar API
- In the Google Cloud Console, go to APIs & Services > Library.
- Search for "Google Calendar API" and click on it.
- Click the Enable button to activate the API for your project.
Configure OAuth Consent Screen
- Navigate to APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields, such as app name and support email, then click Save and Continue.
Create OAuth 2.0 Credentials
- Go to APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose Web application as the application type.
- Enter a name for your OAuth client and add authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
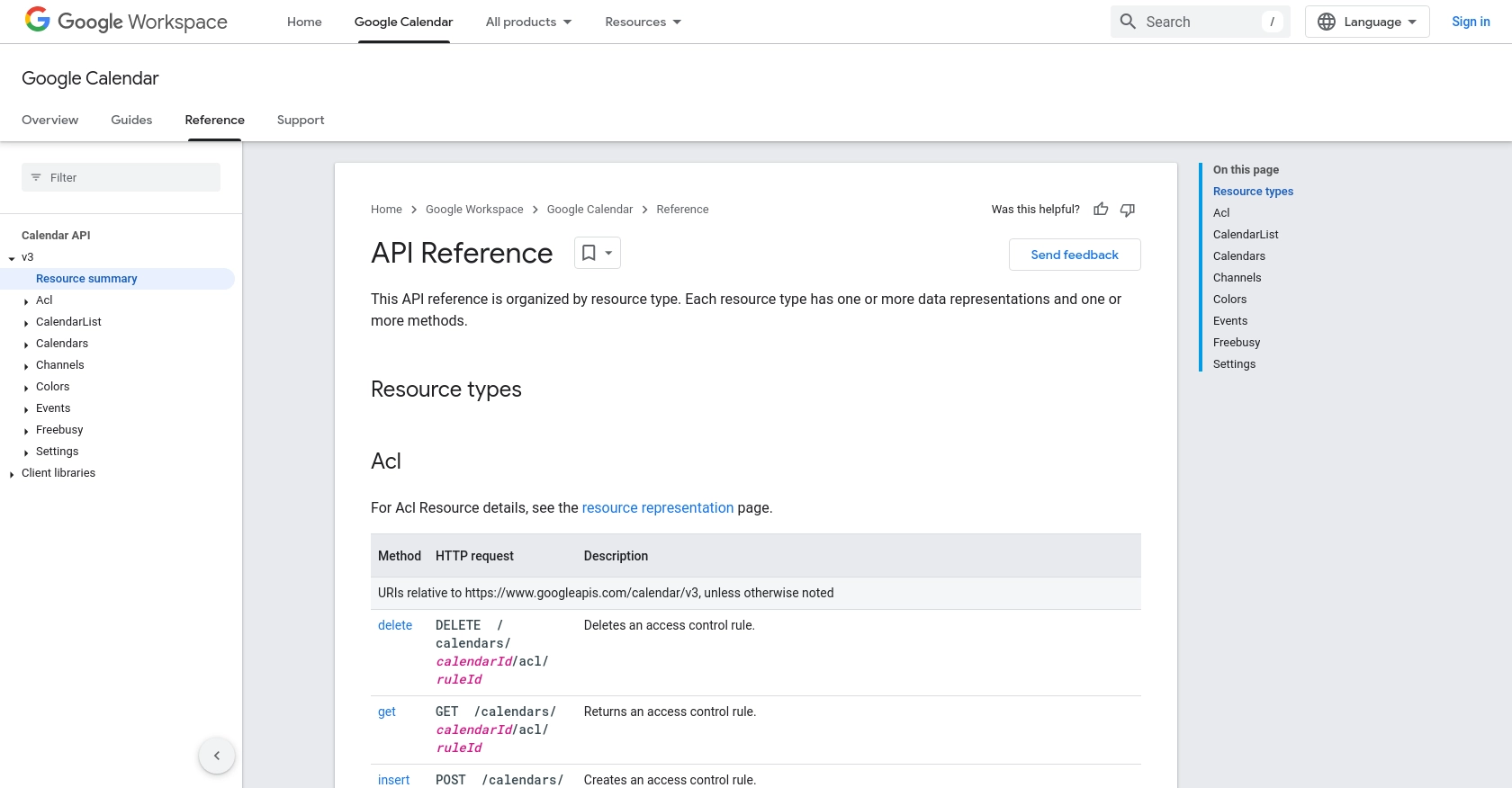
sbb-itb-96038d7
Making API Calls to Retrieve Google Calendar Events Using Python
To interact with the Google Calendar API and retrieve calendar events, you'll need to use Python. This section will guide you through the process of setting up your Python environment, installing necessary dependencies, and executing the API call to fetch events from Google Calendar.
Setting Up Your Python Environment for Google Calendar API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. You will also need the Google Client Library for Python, which simplifies the process of interacting with Google APIs.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use pip to install the Google Client Library by running the following command in your terminal:
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib
Executing the API Call to Fetch Google Calendar Events
With your environment set up, you can now write a Python script to retrieve events from your Google Calendar. The following code demonstrates how to authenticate and make a request to the Google Calendar API to get events.
from google.oauth2.credentials import Credentials
from googleapiclient.discovery import build
# Load credentials from file
creds = Credentials.from_authorized_user_file('token.json', ['https://www.googleapis.com/auth/calendar.readonly'])
# Build the service
service = build('calendar', 'v3', credentials=creds)
# Call the Calendar API
events_result = service.events().list(calendarId='primary', maxResults=10, singleEvents=True, orderBy='startTime').execute()
events = events_result.get('items', [])
# Print the events
if not events:
print('No upcoming events found.')
for event in events:
start = event['start'].get('dateTime', event['start'].get('date'))
print(start, event['summary'])
In this script, we first import necessary modules and load credentials from a file named token.json
. This file is generated after the OAuth 2.0 authentication process. We then build a service object for the Google Calendar API and make a request to list events from the primary calendar.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of upcoming events printed in your terminal. If no events are found, the script will output "No upcoming events found." You can verify the success of the API call by checking your Google Calendar for the events listed.
To handle errors, ensure you include error handling in your script. The Google Calendar API may return various error codes, such as 403 for insufficient permissions or 404 if the calendar ID is incorrect. Refer to the Google Calendar API documentation for more information on error codes and handling strategies.
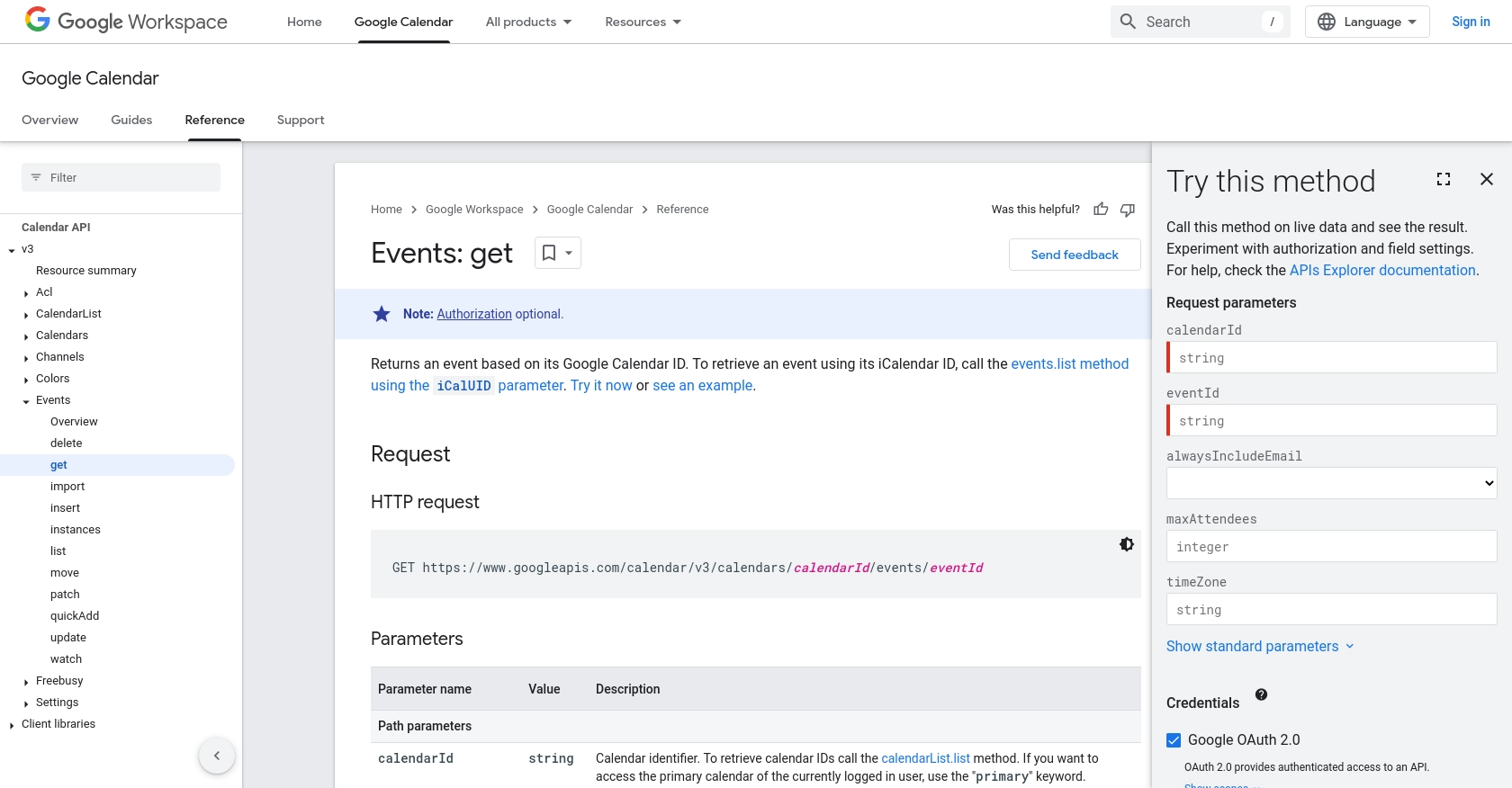
Conclusion and Best Practices for Using Google Calendar API in Python
Integrating with the Google Calendar API using Python offers a powerful way to automate and enhance scheduling functionalities within your applications. By following the steps outlined in this guide, you can efficiently retrieve calendar events and provide users with real-time scheduling information.
Best Practices for Managing Google Calendar API Integrations
- Securely Store Credentials: Always store your OAuth client ID, client secret, and token securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of the Google Calendar API's rate limits. Implement exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the Google Calendar API documentation.
- Implement Error Handling: Incorporate robust error handling to manage potential API errors, such as authentication failures or invalid requests. This ensures a smooth user experience and helps in debugging issues.
- Optimize Data Usage: Use query parameters effectively to limit the data returned by the API, such as specifying a maximum number of events or filtering by date range.
Enhance Your Integration Experience with Endgrate
While integrating with the Google Calendar API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Google Calendar.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore more about how Endgrate can streamline your integration needs by visiting Endgrate.
Read More
- https://endgrate.com/provider/googlecalendar
- https://developers.google.com/calendar/api/v3/reference
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/calendar/api/v3/reference/events/get
Ready to get started?