How to Get Questions with the Google Forms API in Javascript
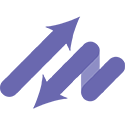
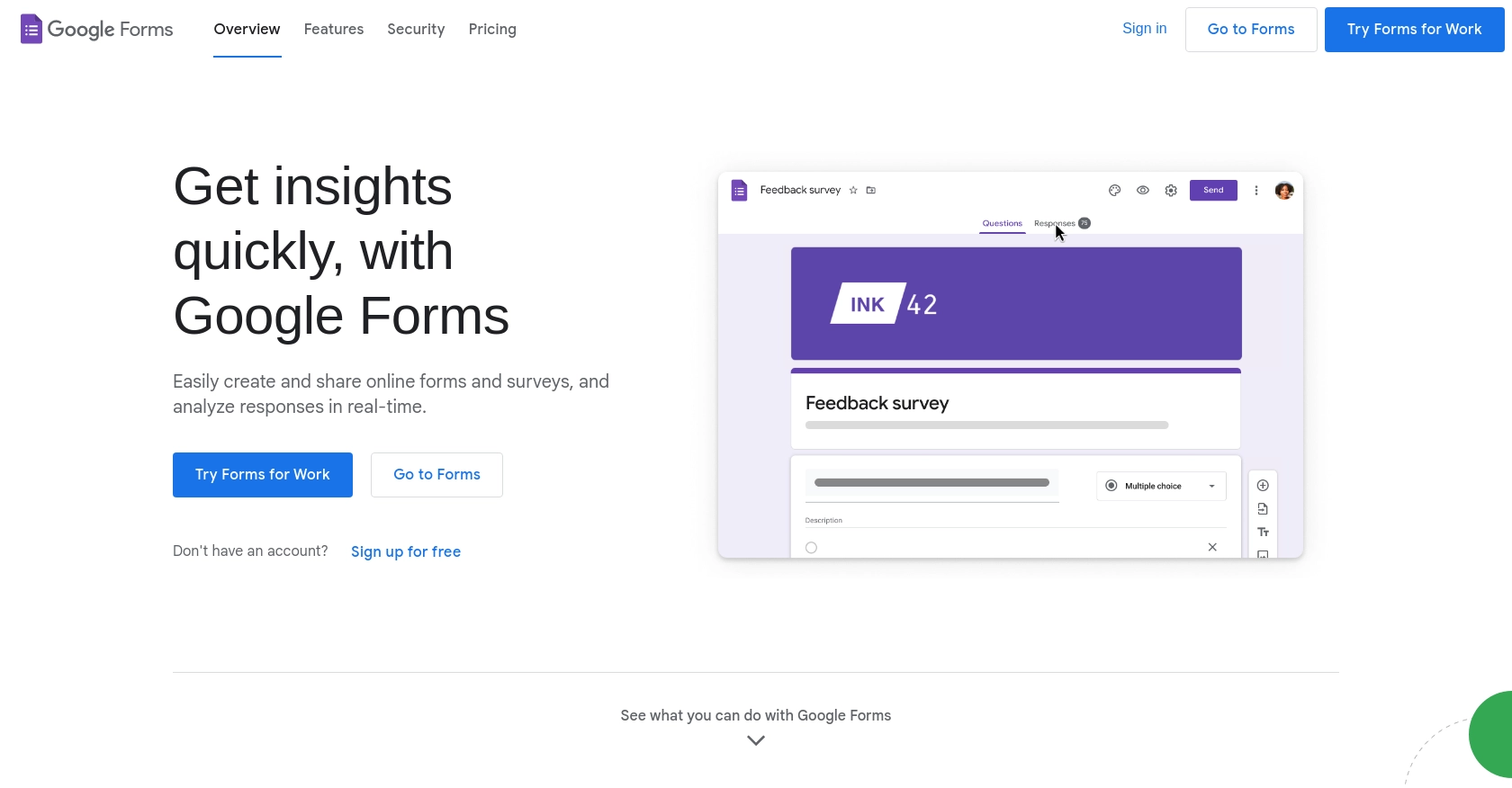
Introduction to Google Forms API Integration
Google Forms is a versatile tool within the Google Workspace suite, allowing users to create surveys, quizzes, and forms for data collection. Its seamless integration with other Google services makes it a popular choice for businesses and educators looking to streamline data gathering and analysis.
For developers, integrating with the Google Forms API offers the ability to programmatically access and manage forms, enabling automation and enhanced functionality. For example, you might want to retrieve questions from a form to dynamically generate a quiz interface on a website, providing a more interactive user experience.
Setting Up Your Google Forms API Test Environment
Before you can start interacting with the Google Forms API using JavaScript, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup will allow you to securely access the API and manage your forms programmatically.
Create a Google Cloud Project for Google Forms API
- Go to the Google Cloud Console.
- Click on the Menu icon, navigate to IAM & Admin, and select Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Forms API
- In the Google Cloud Console, navigate to APIs & Services and select Library.
- Search for Google Forms API and click Enable.
Configure OAuth Consent Screen
- In the Google Cloud Console, go to APIs & Services and select OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the app registration form and click Save and Continue.
Create OAuth 2.0 Credentials
- Navigate to APIs & Services and select Credentials.
- Click Create Credentials and choose OAuth client ID.
- Select Web application as the application type.
- Enter a name for the credential and add authorized redirect URIs.
- Click Create to generate your Client ID and Client Secret.
Once you have your OAuth 2.0 credentials, you can use them to authenticate requests to the Google Forms API. Make sure to securely store your Client ID and Client Secret, as they are essential for accessing the API.
For more detailed steps, refer to the official Google documentation on creating a Google Cloud project and configuring OAuth consent.
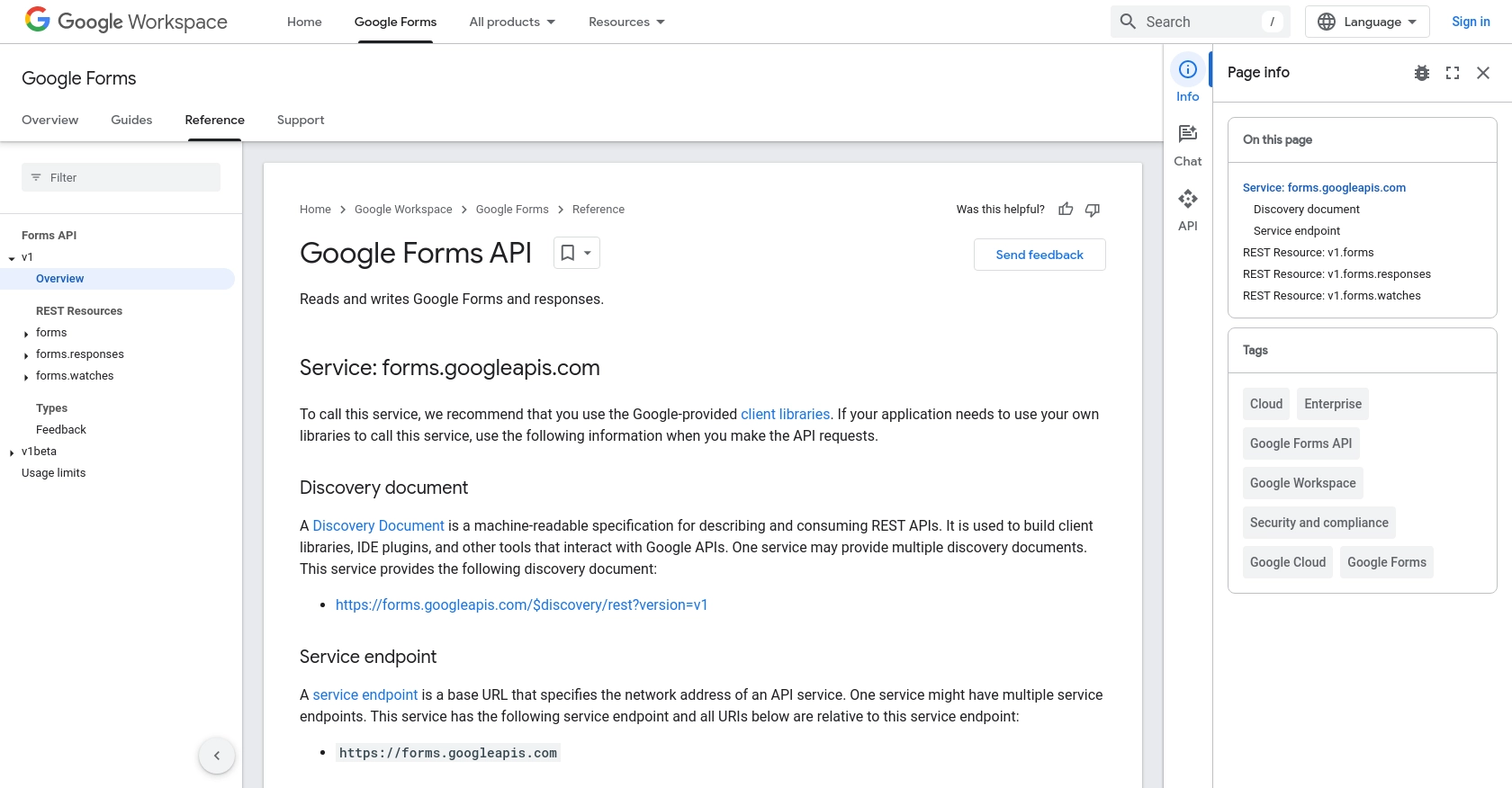
sbb-itb-96038d7
Making API Calls to Retrieve Questions from Google Forms Using JavaScript
To interact with the Google Forms API and retrieve questions from a form, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your JavaScript Environment for Google Forms API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment needed to execute JavaScript code outside of a browser. You can download it from the official Node.js website.
Additionally, you'll need to install the googleapis
package, which provides a client library for accessing Google APIs. Run the following command in your terminal:
npm install googleapis
Writing JavaScript Code to Access Google Forms API
Once your environment is set up, you can write the JavaScript code to authenticate and retrieve questions from a Google Form. Create a file named get_form_questions.js
and add the following code:
const { google } = require('googleapis');
const forms = google.forms('v1');
const { OAuth2 } = google.auth;
// Initialize OAuth2 client
const oauth2Client = new OAuth2(
'YOUR_CLIENT_ID',
'YOUR_CLIENT_SECRET',
'YOUR_REDIRECT_URI'
);
// Set credentials
oauth2Client.setCredentials({
refresh_token: 'YOUR_REFRESH_TOKEN'
});
// Function to get form questions
async function getFormQuestions(formId) {
try {
const response = await forms.forms.get({
auth: oauth2Client,
formId: formId,
});
const questions = response.data.items;
questions.forEach((item) => {
console.log(`Question: ${item.title}`);
});
} catch (error) {
console.error('Error retrieving form questions:', error);
}
}
// Replace with your form ID
getFormQuestions('YOUR_FORM_ID');
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, YOUR_REDIRECT_URI
, YOUR_REFRESH_TOKEN
, and YOUR_FORM_ID
with your actual credentials and form ID.
Executing the JavaScript Code and Handling Errors
Run the script using Node.js by executing the following command in your terminal:
node get_form_questions.js
If successful, the script will output the titles of the questions in the specified Google Form. If there are any errors, they will be logged to the console. Common errors include authentication issues or incorrect form IDs.
Verifying API Call Success in Google Forms
To verify that your API call was successful, you can check the console output for the list of questions. Ensure that the questions match those in your Google Form. If you encounter any issues, double-check your credentials and form ID.
For more detailed information on handling errors and understanding error codes, refer to the Google Forms API documentation.
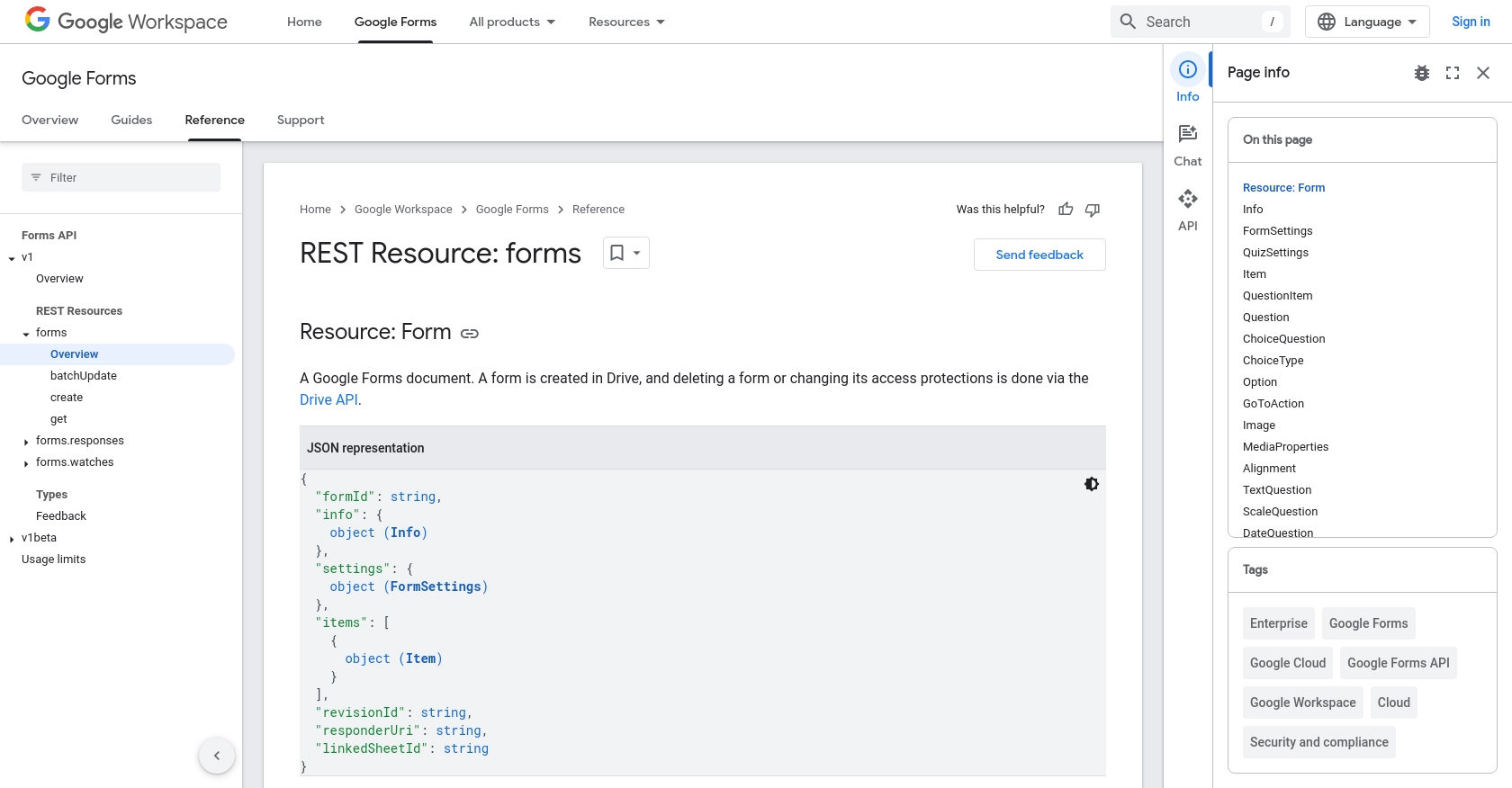
Conclusion and Best Practices for Using Google Forms API with JavaScript
Integrating with the Google Forms API using JavaScript opens up a world of possibilities for automating and enhancing data collection processes. By following the steps outlined in this guide, you can efficiently retrieve questions from Google Forms and leverage them in your applications.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid service disruptions. Implement exponential backoff strategies to handle retries gracefully. For more details, refer to the Google Forms API documentation.
- Data Transformation and Standardization: When retrieving data, ensure that it is transformed and standardized according to your application's needs. This will help maintain consistency and improve data quality.
Call to Action: Simplify Your Integrations with Endgrate
While integrating with APIs like Google Forms can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing integrations, allowing you to focus on your core product. With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how we can help you save time and resources.
Read More
- https://endgrate.com/provider/googleforms
- https://developers.google.com/forms/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/forms/api/reference/rest/v1/forms
Ready to get started?