How to Get People with the Salesloft API in PHP
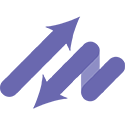
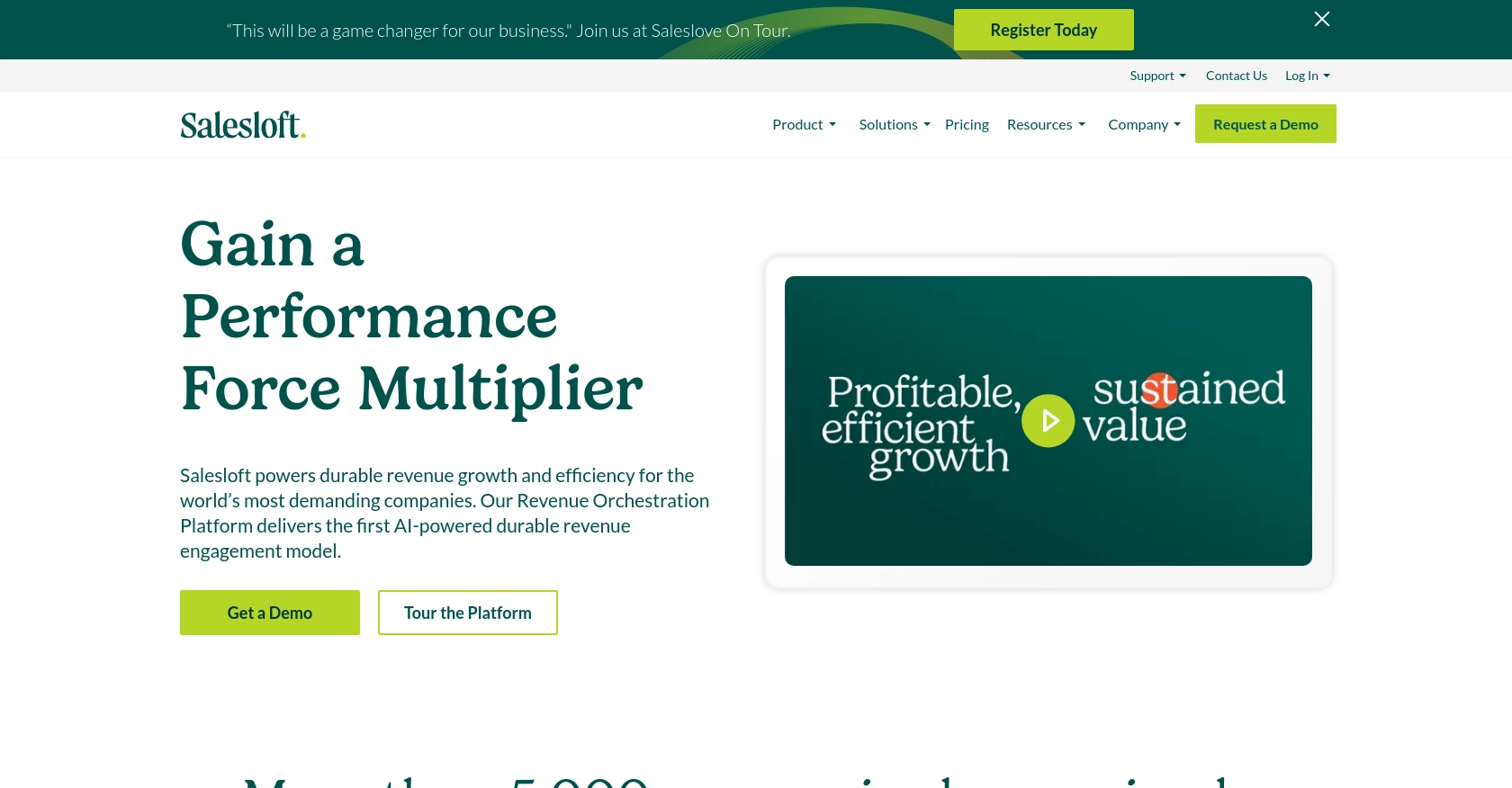
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform designed to enhance the efficiency and effectiveness of sales teams. It offers a suite of tools that streamline communication, automate workflows, and provide valuable insights into sales processes.
Integrating with the Salesloft API allows developers to access and manage sales data programmatically, enabling the automation of tasks such as retrieving and analyzing contact information. For example, a developer might use the Salesloft API to fetch a list of people and their engagement history, which can be used to tailor sales strategies and improve customer interactions.
Setting Up Your Salesloft Test Account for API Integration
Before you can start interacting with the Salesloft API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Salesloft Account
If you don't already have a Salesloft account, you can sign up for a free trial on the Salesloft website. This will give you access to the platform's features and allow you to create an OAuth application for API access.
- Visit the Salesloft website and click on "Sign Up" to create your account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is set up, log in to access the Salesloft dashboard.
Create an OAuth Application in Salesloft
To interact with the Salesloft API, you'll need to create an OAuth application. This will provide you with the necessary credentials to authenticate your API requests.
- Navigate to Your Applications in the Salesloft dashboard.
- Select OAuth Applications and click on Create New.
- Fill in the required fields, such as the application name and redirect URI, then click Save.
- After saving, you'll receive your Client ID and Client Secret. Keep these credentials secure as you'll need them for authentication.
Obtain Authorization Code and Access Tokens
With your OAuth application set up, you can now obtain the authorization code and access tokens needed to make API calls.
- Generate a request to the authorization endpoint using your Client ID and Redirect URI:
- Authorize the application when prompted. Upon approval, you'll receive a code in the redirect URI.
- Exchange the authorization code for access and refresh tokens by making a POST request:
- Store the access_token and refresh_token securely for future API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
With your Salesloft test account and OAuth application configured, you're now ready to start making API calls to retrieve and manage data programmatically.
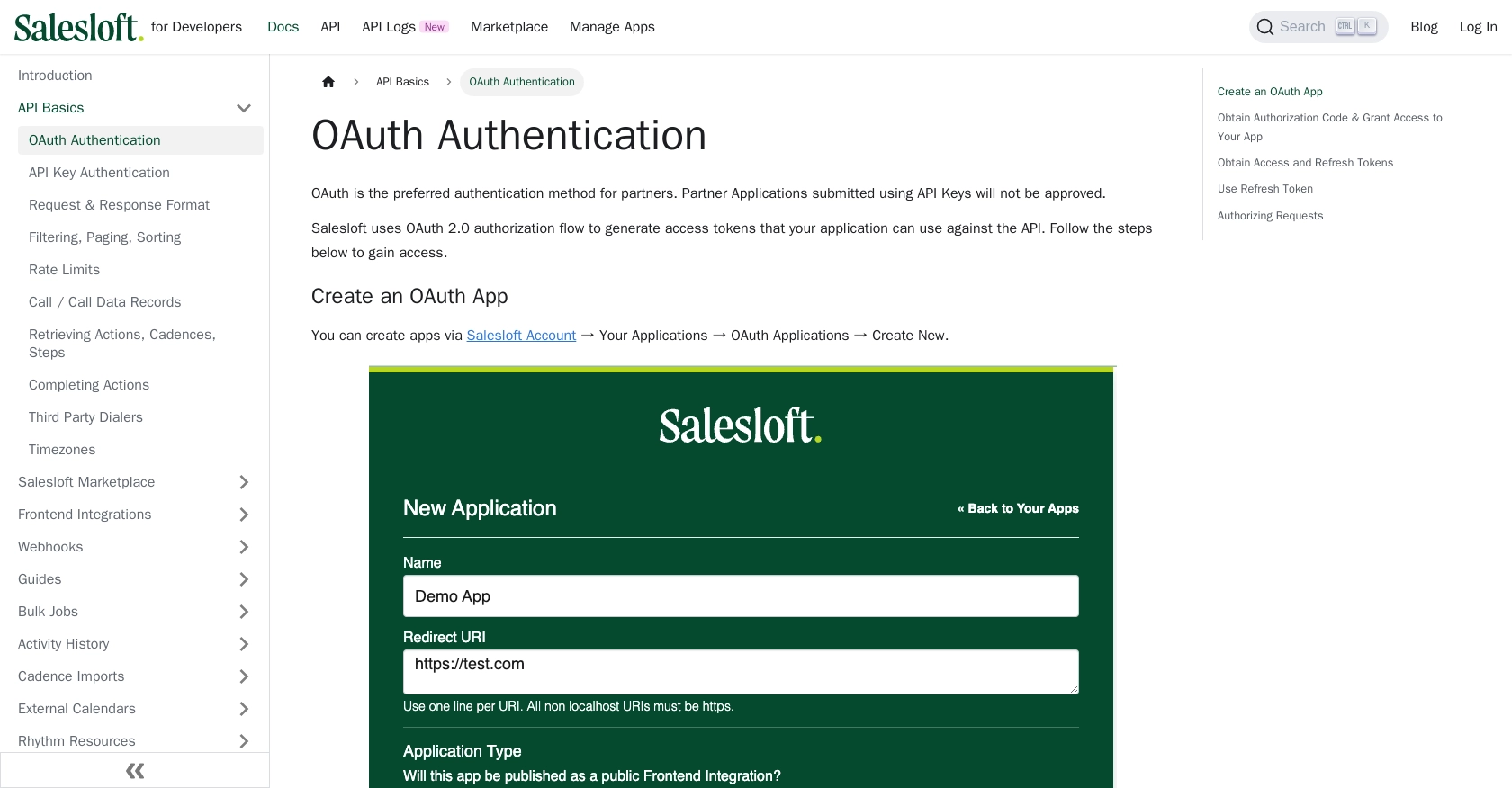
sbb-itb-96038d7
Making API Calls to Retrieve People Data from Salesloft Using PHP
With your Salesloft OAuth application set up, you can now proceed to make API calls to retrieve people data. This section will guide you through the process of setting up your PHP environment and executing the necessary API requests.
Setting Up Your PHP Environment for Salesloft API Integration
Before making API calls, ensure that your PHP environment is properly configured. You will need:
- PHP 7.4 or higher
- Composer for dependency management
Install the Guzzle HTTP client, which simplifies making HTTP requests in PHP:
composer require guzzlehttp/guzzle
Executing the Salesloft API Call to List People
Now that your environment is ready, you can write a PHP script to fetch people data from Salesloft. Create a file named get_salesloft_people.php
and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN'; // Replace with your actual access token
$response = $client->request('GET', 'https://api.salesloft.com/v2/people', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
$data = json_decode($response->getBody(), true);
foreach ($data['data'] as $person) {
echo 'Name: ' . $person['first_name'] . ' ' . $person['last_name'] . "\n";
echo 'Email: ' . $person['email_address'] . "\n\n";
}
Replace YOUR_ACCESS_TOKEN
with the access token you obtained earlier. This script uses Guzzle to send a GET request to the Salesloft API endpoint for listing people. It then decodes the JSON response and prints out the names and email addresses of the people retrieved.
Verifying the API Request and Handling Errors
After running the script, you should see the list of people displayed in your terminal. To verify the request's success, check your Salesloft dashboard to ensure the data matches the retrieved information.
Handle potential errors by checking the response status code. Salesloft API may return error codes such as 403 (Forbidden), 404 (Not Found), or 422 (Unprocessable Entity). Here's how you can handle these errors:
try {
$response = $client->request('GET', 'https://api.salesloft.com/v2/people', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
} catch (\GuzzleHttp\Exception\ClientException $e) {
echo 'Error: ' . $e->getResponse()->getStatusCode() . ' - ' . $e->getMessage();
}
This code snippet uses a try-catch block to catch client exceptions and print the error status code and message, helping you diagnose issues with your API requests.
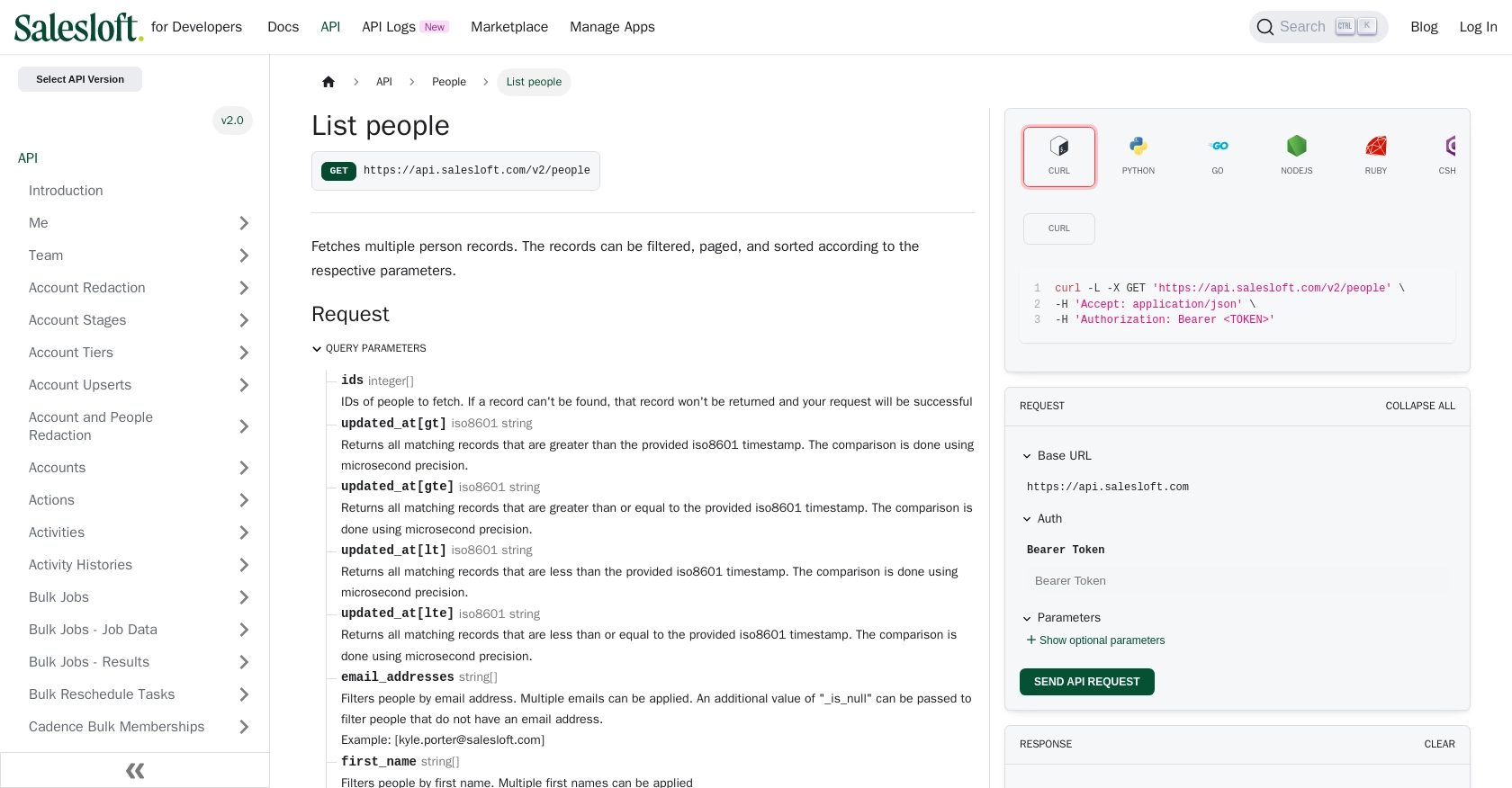
Conclusion and Best Practices for Salesloft API Integration in PHP
Integrating with the Salesloft API using PHP provides a powerful way to automate and enhance your sales processes. By following the steps outlined in this guide, you can efficiently retrieve and manage people data, allowing your sales team to focus on strategic tasks rather than manual data entry.
Best Practices for Storing Salesloft API Credentials
It's crucial to store your API credentials securely to prevent unauthorized access. Consider using environment variables or secure vault services to manage your Client ID
, Client Secret
, and Access Token
. Avoid hardcoding these values directly in your scripts.
Handling Salesloft API Rate Limits
The Salesloft API enforces a rate limit of 600 cost per minute per team. To avoid hitting this limit, implement logic to monitor the x-ratelimit-remaining-minute
header in your API responses. If you're approaching the limit, consider implementing a retry mechanism with exponential backoff to manage requests efficiently. For more details, refer to the Salesloft API Rate Limits documentation.
Transforming and Standardizing Salesloft Data
When working with data retrieved from Salesloft, consider transforming and standardizing fields to match your application's data model. This might involve mapping Salesloft fields to your internal database schema or converting data formats to ensure consistency across systems.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, consider using Endgrate. By providing a unified API endpoint, Endgrate allows you to connect to multiple platforms, including Salesloft, with ease. This approach reduces development time and allows you to focus on building your core product. Learn more about how Endgrate can enhance your integration strategy by visiting Endgrate's website.
By adhering to these best practices and leveraging tools like Endgrate, you can ensure a robust and efficient integration with the Salesloft API, ultimately driving better sales outcomes for your organization.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/people-index/
Ready to get started?