Using the BigCommerce API to Get Product Categories in Python
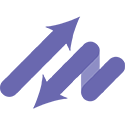
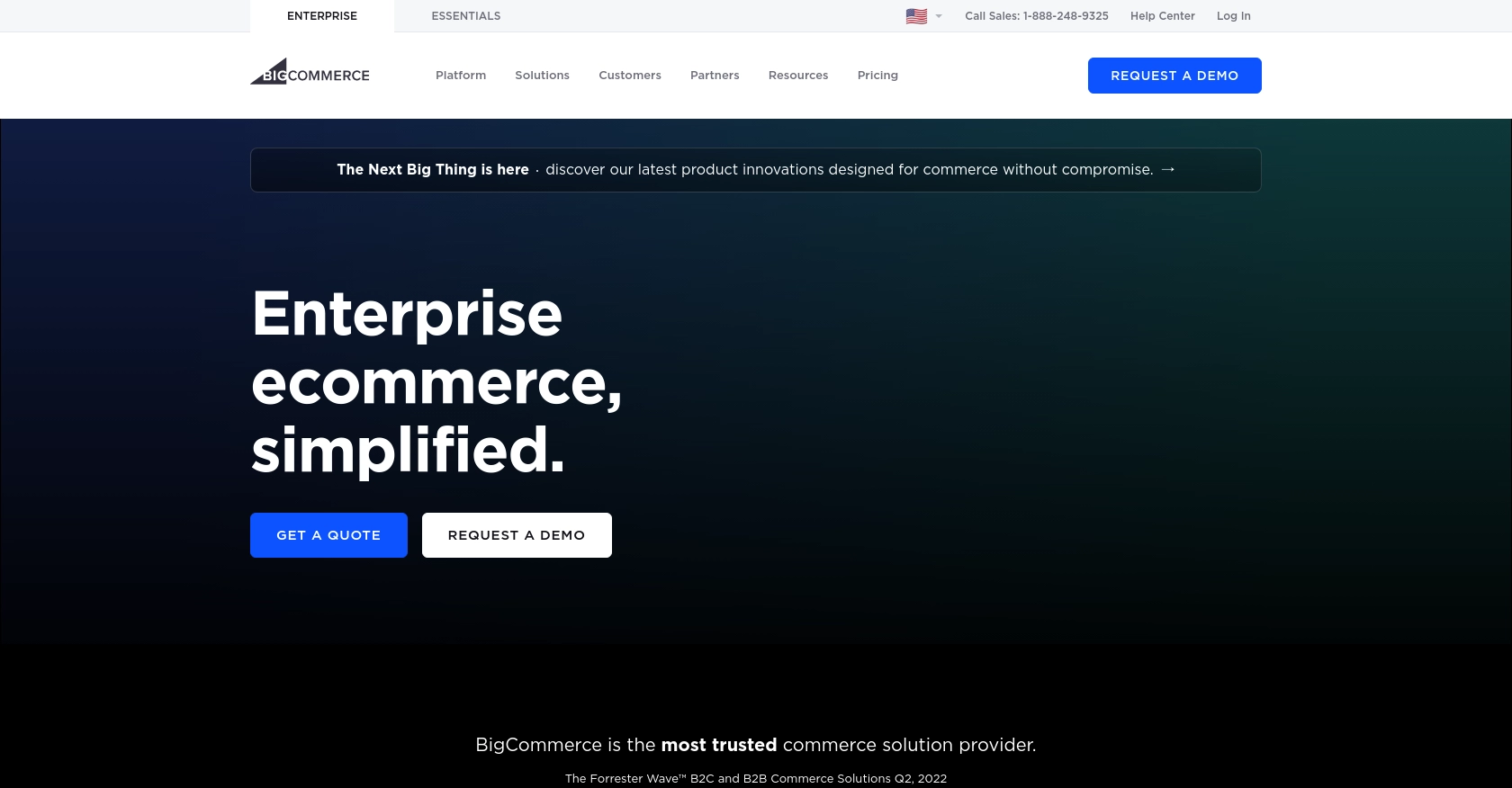
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a wide array of features, including product management, order processing, and customer engagement tools, making it a popular choice for businesses looking to expand their online presence.
Integrating with the BigCommerce API allows developers to access and manipulate store data programmatically, enabling automation and customization of various e-commerce processes. For example, a developer might use the BigCommerce API to retrieve product categories, which can then be used to dynamically display product listings on a custom website or application.
This article will guide you through the process of using Python to interact with the BigCommerce API, specifically focusing on retrieving product categories. By the end of this tutorial, you will be able to efficiently access and manage product categories within your BigCommerce store using Python.
Setting Up Your BigCommerce Test or Sandbox Account
Before diving into the integration process, it's essential to set up a BigCommerce test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live store data. Follow these steps to get started:
Create a BigCommerce Sandbox Account
If you don't already have a BigCommerce account, you can sign up for a sandbox account on the BigCommerce website. This account will provide you with a testing environment to explore the API functionalities.
- Visit the BigCommerce website and navigate to the sandbox account registration page.
- Fill in the required details to create your account. Once completed, you'll have access to a sandbox store where you can test API interactions.
Generate API Credentials for BigCommerce
To interact with the BigCommerce API, you'll need to generate API credentials. These credentials include an access token, which is used to authenticate your API requests.
- Log in to your BigCommerce sandbox account and navigate to the "Advanced Settings" section.
- Select "API Accounts" and click on "Create API Account."
- Choose the type of API account that suits your needs. For most cases, a "Store API Account" is appropriate.
- Configure the necessary OAuth scopes to ensure your API account has the required permissions. Refer to the BigCommerce authentication documentation for guidance on selecting the appropriate scopes.
- Once configured, save the API account details. You'll receive an access token, which you'll use in your API requests.
Understanding BigCommerce API Authentication
BigCommerce uses the X-Auth-Token header for authentication. When making API requests, include the access token as the value of this header. Ensure that your token is in scope for the actions you wish to perform.
# Example of setting the X-Auth-Token header in a Python request
headers = {
"X-Auth-Token": "your_access_token",
"Accept": "application/json"
}
For more detailed information on authentication, refer to the BigCommerce authentication documentation.
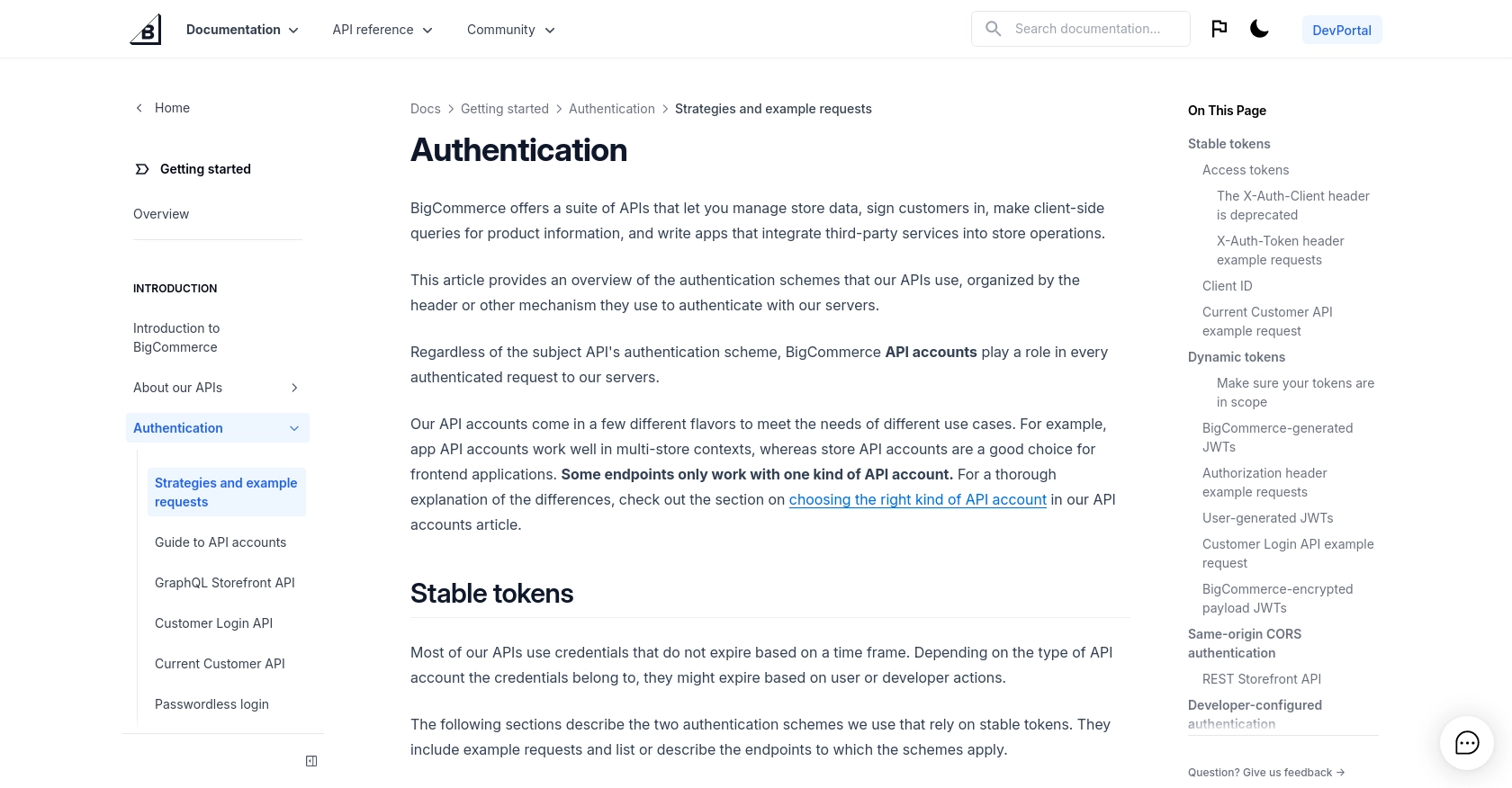
sbb-itb-96038d7
Making API Calls to Retrieve BigCommerce Product Categories Using Python
To interact with the BigCommerce API and retrieve product categories, you'll need to set up your Python environment and make HTTP requests to the appropriate endpoints. This section will guide you through the process of making these API calls effectively.
Setting Up Your Python Environment for BigCommerce API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Retrieve Product Categories from BigCommerce
Once your environment is set up, you can write a Python script to make a GET request to the BigCommerce API and retrieve product categories. Follow these steps:
import requests
# Define the API endpoint and headers
store_hash = "your_store_hash"
endpoint = f"https://api.bigcommerce.com/stores/{store_hash}/v3/catalog/categories"
headers = {
"X-Auth-Token": "your_access_token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
categories = response.json().get("data", [])
for category in categories:
print(f"Category ID: {category['id']}, Name: {category['name']}")
else:
print(f"Failed to retrieve categories. Status code: {response.status_code}")
Replace your_store_hash
and your_access_token
with your actual store hash and access token. The script sends a GET request to the BigCommerce API to fetch all product categories. If successful, it prints the ID and name of each category.
Verifying the Success of Your BigCommerce API Request
After running the script, verify the output by checking the categories listed in your BigCommerce sandbox account. The categories printed by the script should match those in your store.
Handling Errors and Understanding BigCommerce API Response Codes
It's crucial to handle potential errors when making API calls. The BigCommerce API returns various status codes to indicate the result of your request:
- 200 OK: The request was successful, and the categories were retrieved.
- 401 Unauthorized: Authentication failed. Check your access token.
- 404 Not Found: The endpoint or resource was not found. Verify the URL and store hash.
- 429 Too Many Requests: You've hit the rate limit. The default rate limit is 40 concurrent requests.
Refer to the BigCommerce API documentation for more details on handling errors and understanding response codes.
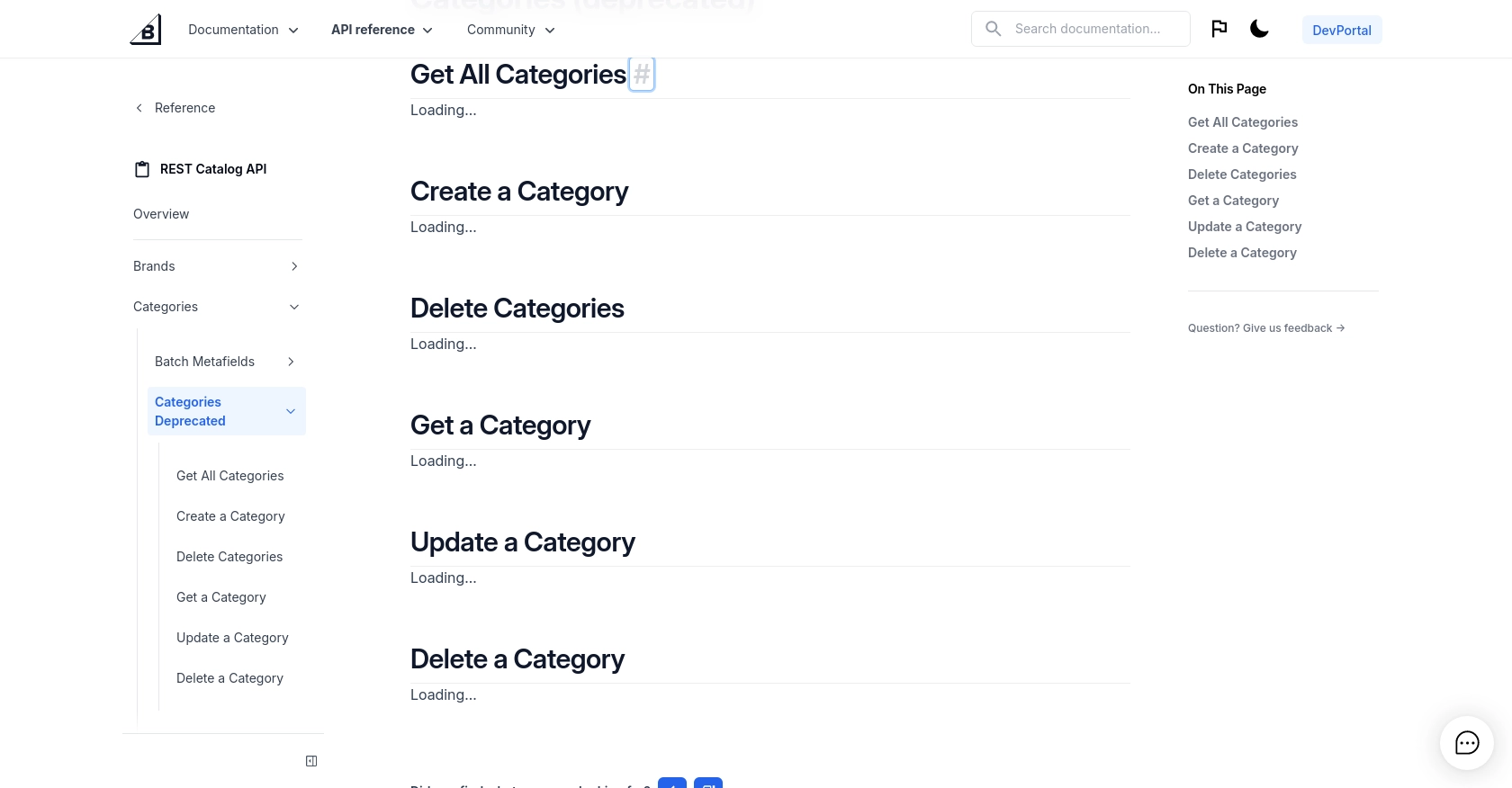
Conclusion and Best Practices for BigCommerce API Integration Using Python
Integrating with the BigCommerce API using Python provides a powerful way to automate and customize your e-commerce operations. By following the steps outlined in this guide, you can efficiently retrieve product categories and leverage this data to enhance your online store's functionality.
Best Practices for Secure and Efficient BigCommerce API Usage
- Securely Store Credentials: Always store your API credentials securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API rate limits. The default limit is 40 concurrent requests. Implement logic to handle rate limiting gracefully, such as retry mechanisms with exponential backoff.
- Data Transformation: Consider transforming and standardizing data fields to match your application's requirements. This ensures consistency and reliability in data handling.
- Monitor API Usage: Regularly monitor your API usage and performance. This helps in identifying potential issues and optimizing your integration for better efficiency.
Enhance Your Integration Strategy with Endgrate
While building integrations with the BigCommerce API can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution to manage multiple integrations effortlessly. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case and apply it across different integrations, reducing redundancy.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can simplify your integration processes and help you scale your e-commerce solutions efficiently. Visit Endgrate to learn more and get started today.
Read More
Ready to get started?