Using the Quickbooks API to Create Or Update Sales Orders in Javascript
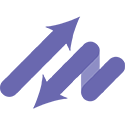
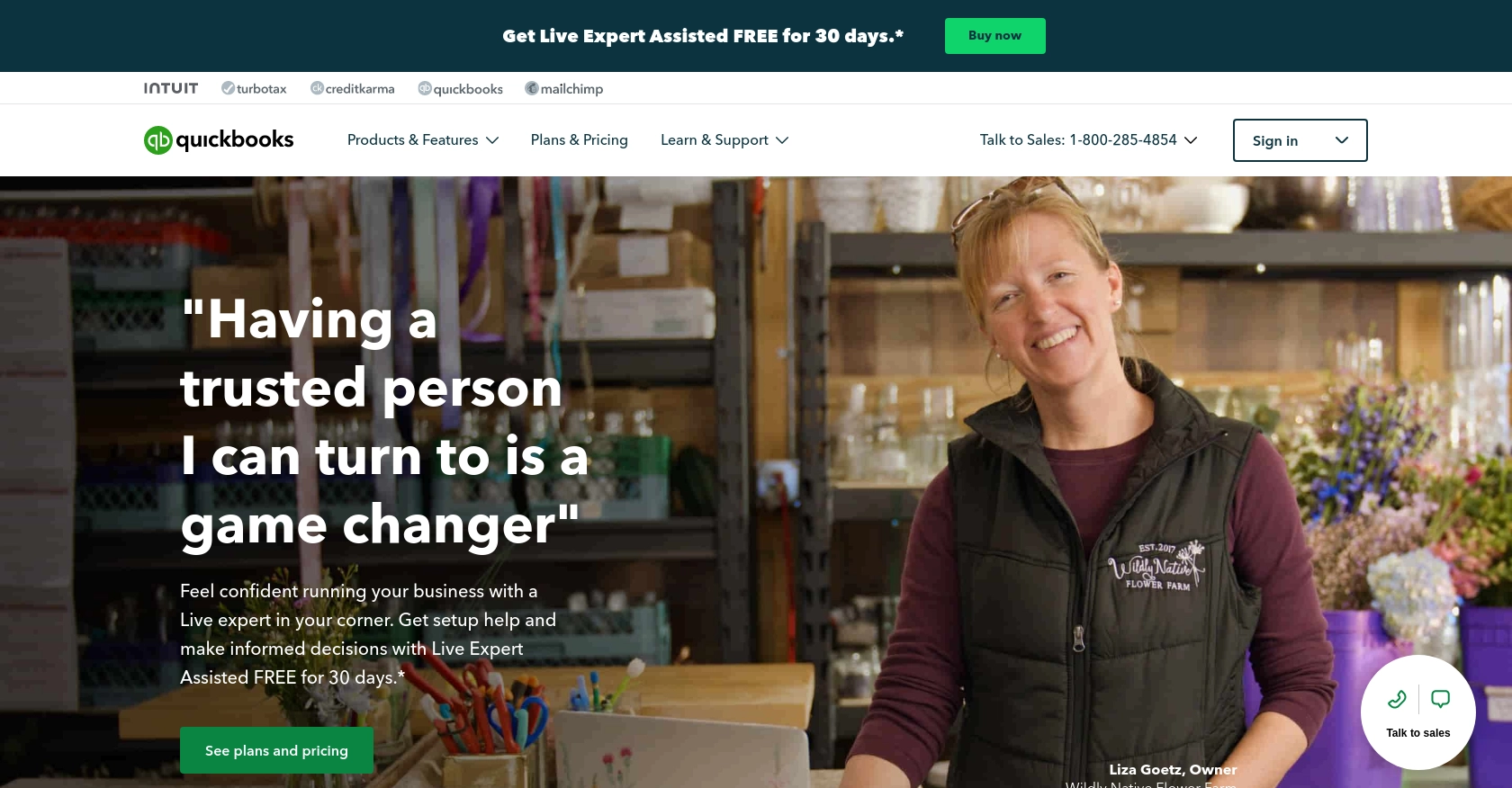
Introduction to QuickBooks API for Sales Orders
QuickBooks is a widely-used accounting software that offers robust tools for managing finances, invoicing, payroll, and more. It is a preferred choice for small to medium-sized businesses looking to streamline their financial operations.
Developers often integrate with QuickBooks to automate and enhance financial workflows. By using the QuickBooks API, developers can create or update sales orders directly from their applications, allowing for seamless order management and improved efficiency.
For example, a developer might want to automatically generate sales orders in QuickBooks from an e-commerce platform, ensuring that all sales data is accurately recorded and easily accessible for accounting purposes.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start creating or updating sales orders using the QuickBooks API, you'll need to set up a QuickBooks sandbox account. This environment allows developers to test their applications without affecting live data, ensuring a safe and controlled testing space.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. Follow these steps to create one:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" and fill in the required details to create your account.
- Once registered, log in to your QuickBooks Developer account.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, the next step is to set up a sandbox company:
- Navigate to the "Dashboard" in your QuickBooks Developer account.
- Click on "Sandbox" and then "Add Sandbox" to create a new sandbox company.
- Choose the type of company that best suits your testing needs and click "Create."
Your sandbox company is now ready, and you can use it to test API calls without any risk to real data.
Creating an App for OAuth Authentication
QuickBooks API uses OAuth 2.0 for authentication. To interact with the API, you'll need to create an app to obtain the necessary credentials:
- In your QuickBooks Developer account, go to the "My Apps" section.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the app details, including the name and scope of the app.
- Once the app is created, navigate to the "Keys & OAuth" section.
- Here, you'll find your Client ID and Client Secret, which are essential for OAuth authentication.
Ensure you store these credentials securely, as they will be used to authorize API requests.
Configuring OAuth Redirect URIs
To complete the OAuth setup, configure the redirect URIs:
- In the "Keys & OAuth" section of your app, locate the "Redirect URIs" field.
- Add the URI where users will be redirected after authentication. This should match the URI in your application code.
With these steps completed, your QuickBooks sandbox environment is ready for testing API interactions.
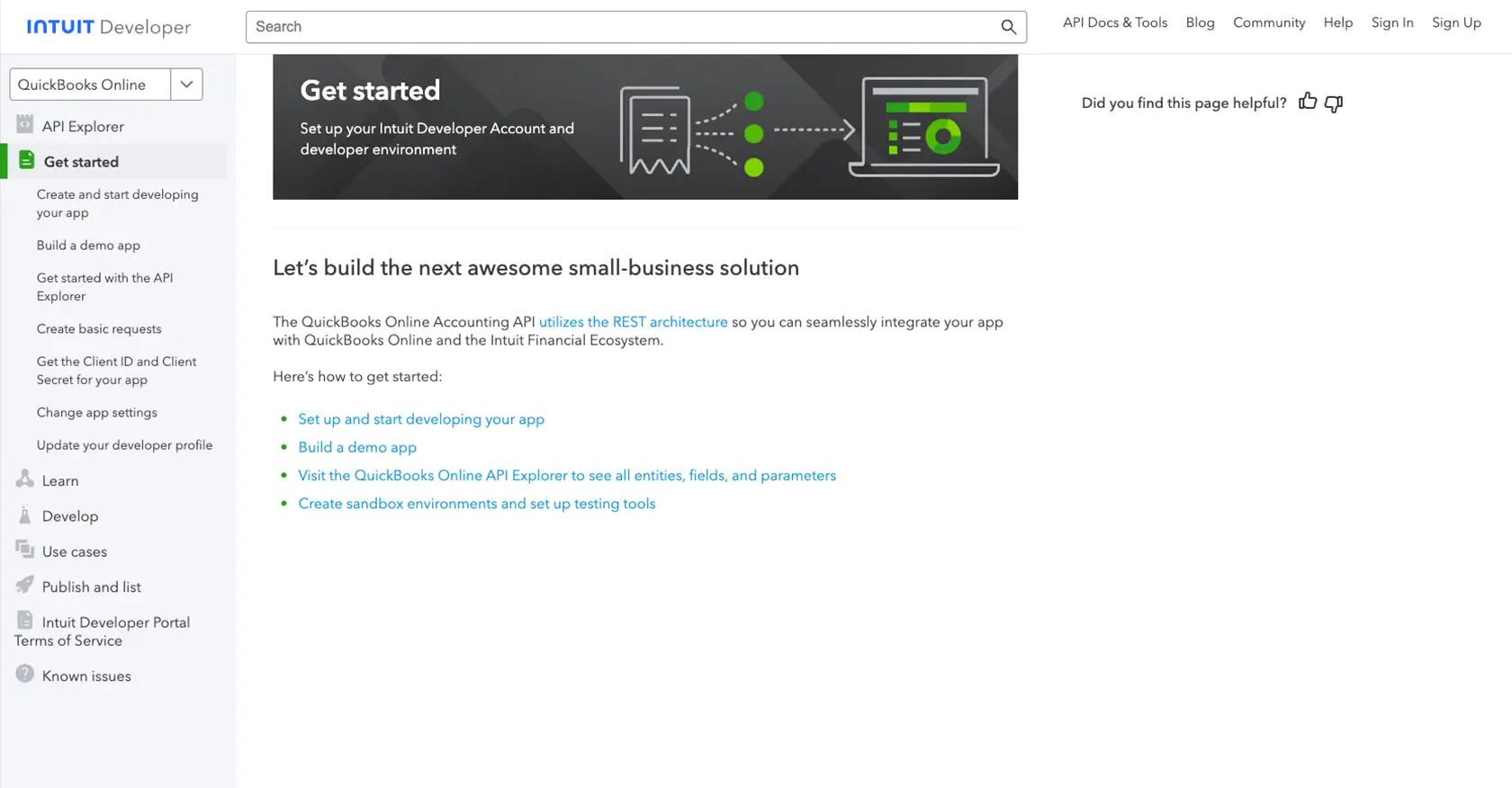
sbb-itb-96038d7
Making API Calls to Create or Update Sales Orders in QuickBooks Using JavaScript
To interact with the QuickBooks API for creating or updating sales orders, you'll need to use JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for QuickBooks API
Before making API calls, ensure you have the following prerequisites installed:
- Node.js (version 14.x or higher)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, create a new project directory and initialize it:
mkdir quickbooks-api-integration
cd quickbooks-api-integration
npm init -y
Next, install the required dependencies:
npm install axios
Axios is a promise-based HTTP client for making requests to the QuickBooks API.
Writing JavaScript Code to Create or Update Sales Orders in QuickBooks
Create a new file named salesOrder.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/salesorder';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json',
'Accept': 'application/json'
};
// Define the sales order data
const salesOrderData = {
"Line": [
{
"Amount": 100.00,
"DetailType": "SalesItemLineDetail",
"SalesItemLineDetail": {
"ItemRef": {
"value": "1",
"name": "Services"
}
}
}
],
"CustomerRef": {
"value": "1"
}
};
// Function to create or update a sales order
async function createOrUpdateSalesOrder() {
try {
const response = await axios.post(endpoint, salesOrderData, { headers });
console.log('Sales Order Created/Updated Successfully:', response.data);
} catch (error) {
console.error('Error Creating/Updating Sales Order:', error.response.data);
}
}
createOrUpdateSalesOrder();
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token.
Running the JavaScript Code and Verifying the API Call
Run the script using the following command:
node salesOrder.js
If the request is successful, you should see a confirmation message in the console. Verify the creation or update of the sales order by checking your QuickBooks sandbox account.
Handling Errors and Understanding QuickBooks API Error Codes
When making API calls, it's crucial to handle errors gracefully. The QuickBooks API may return various error codes, such as:
- 401 Unauthorized: Check your access token and ensure it's valid.
- 400 Bad Request: Verify the request payload and parameters.
- 500 Internal Server Error: Retry the request or contact QuickBooks support.
For more detailed error handling, refer to the QuickBooks API documentation.
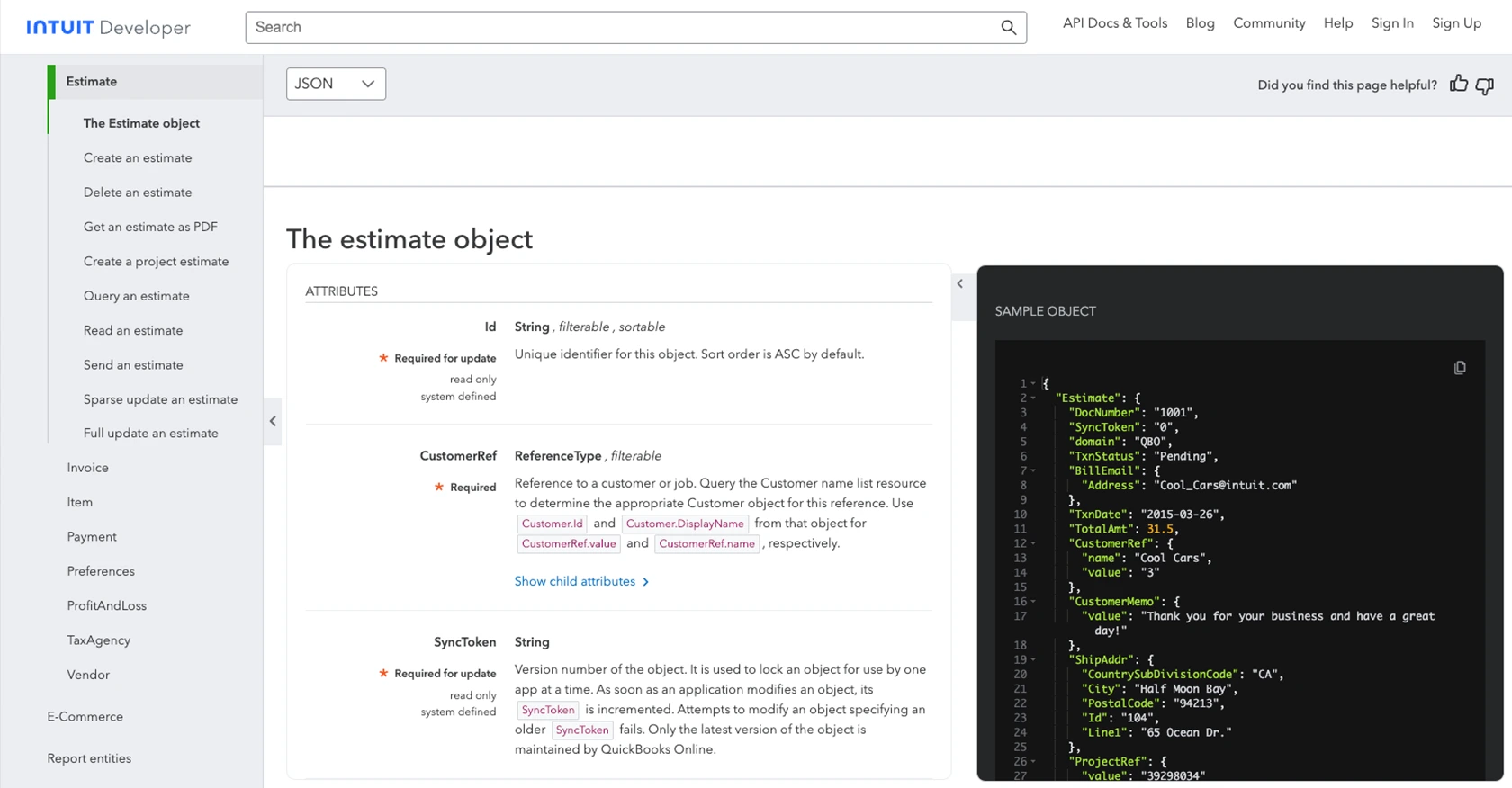
Best Practices for QuickBooks API Integration
When integrating with the QuickBooks API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key considerations:
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send to QuickBooks is standardized and validated to avoid errors and maintain data integrity.
Enhancing Integration Efficiency with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including QuickBooks.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Visit Endgrate to learn more about how you can streamline your integration processes and improve your application's capabilities.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/estimate
Ready to get started?