Using the Sage 100 API to Get Purchase Orders (with Python examples)
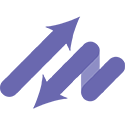
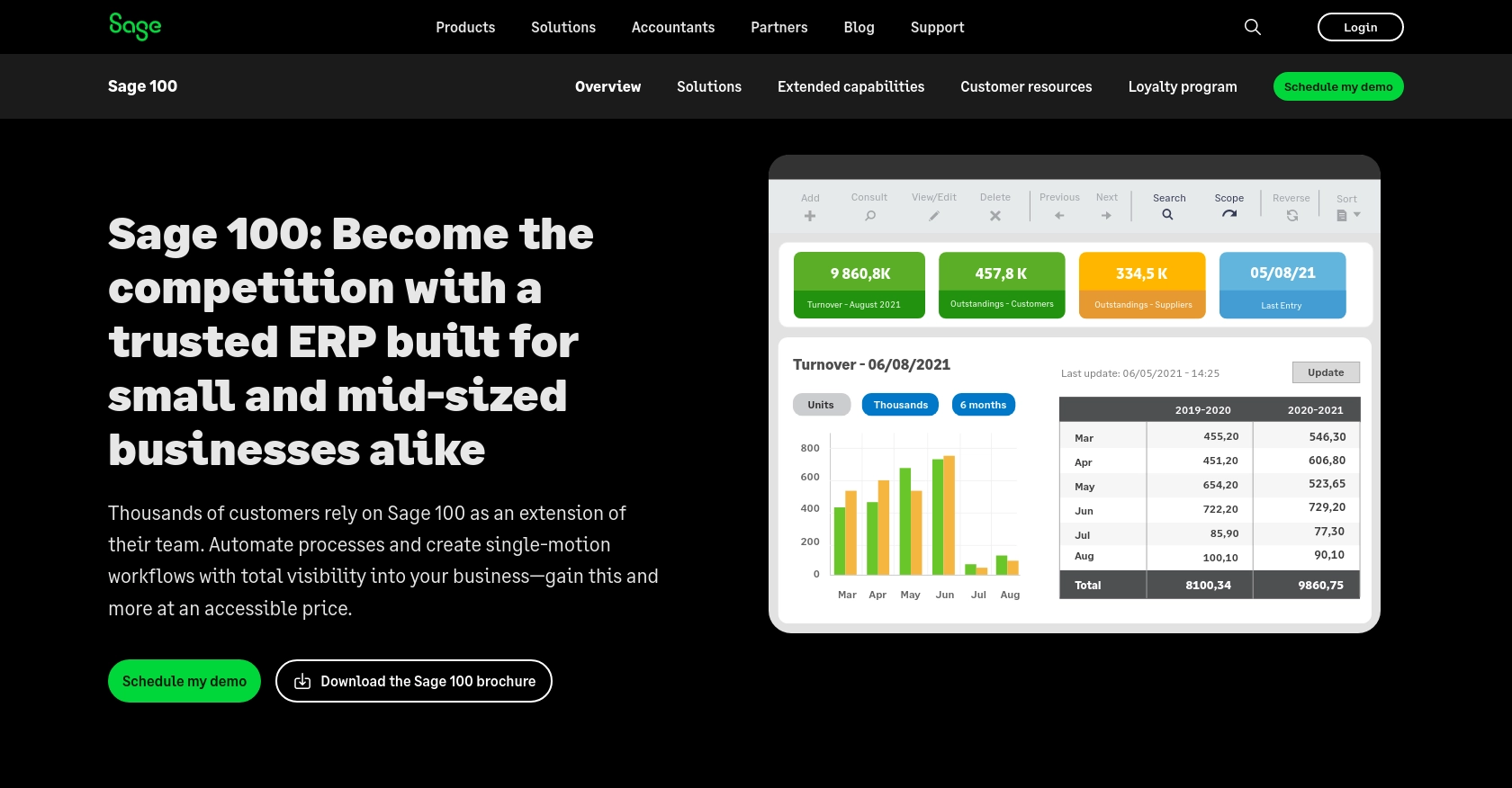
Introduction to Sage 100 API for Purchase Orders
Sage 100 is a comprehensive enterprise resource planning (ERP) solution designed to streamline business operations for small to medium-sized enterprises. It offers a robust suite of tools for managing accounting, inventory, and customer relationships, making it a popular choice for businesses looking to enhance their operational efficiency.
Integrating with the Sage 100 API allows developers to access and manage various business data, such as purchase orders, directly from their applications. This integration can be particularly beneficial for automating procurement processes, ensuring that purchase orders are efficiently tracked and managed within the Sage 100 system.
For example, a developer might use the Sage 100 API to retrieve purchase order details and integrate them with a third-party inventory management system, providing real-time updates and improving supply chain visibility.
Setting Up Your Sage 100 Test Environment
Before you can start interacting with the Sage 100 API to retrieve purchase orders, you need to set up a test environment. This involves configuring the Sage 100 ODBC driver and creating a Data Source Name (DSN) to connect to the Sage 100 ERP system.
Installing and Configuring the Sage 100 ODBC Driver
To begin, ensure that the Sage 100 ODBC driver is installed on your system. This driver is essential for establishing a connection to the Sage 100 database. Follow these steps to configure the driver:
- Access the ODBC Data Source Administrator on your system.
- Create a new DSN that connects to the Sage 100 ERP system using the correct server, database, and authentication settings.
- Ensure that the DSN is properly configured to communicate with the Sage 100 database.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 Application for API Access
Once the ODBC driver is configured, you need to set up an application within Sage 100 to access the API. This involves creating an app and obtaining the necessary credentials:
- Log in to your Sage 100 account and navigate to the API management section.
- Create a new application and provide the required details.
- Generate the client ID and client secret for your application. These credentials will be used to authenticate API requests.
Testing the Sage 100 ODBC Connection
After setting up the DSN and application, it's crucial to test the connection to ensure everything is working correctly:
- Open the ODBC Data Source Administrator and locate your Sage 100 DSN.
- Use the "Test Connection" feature to verify that the connection to the Sage 100 database is successful.
If the connection is successful, you are ready to proceed with making API calls to retrieve purchase orders.
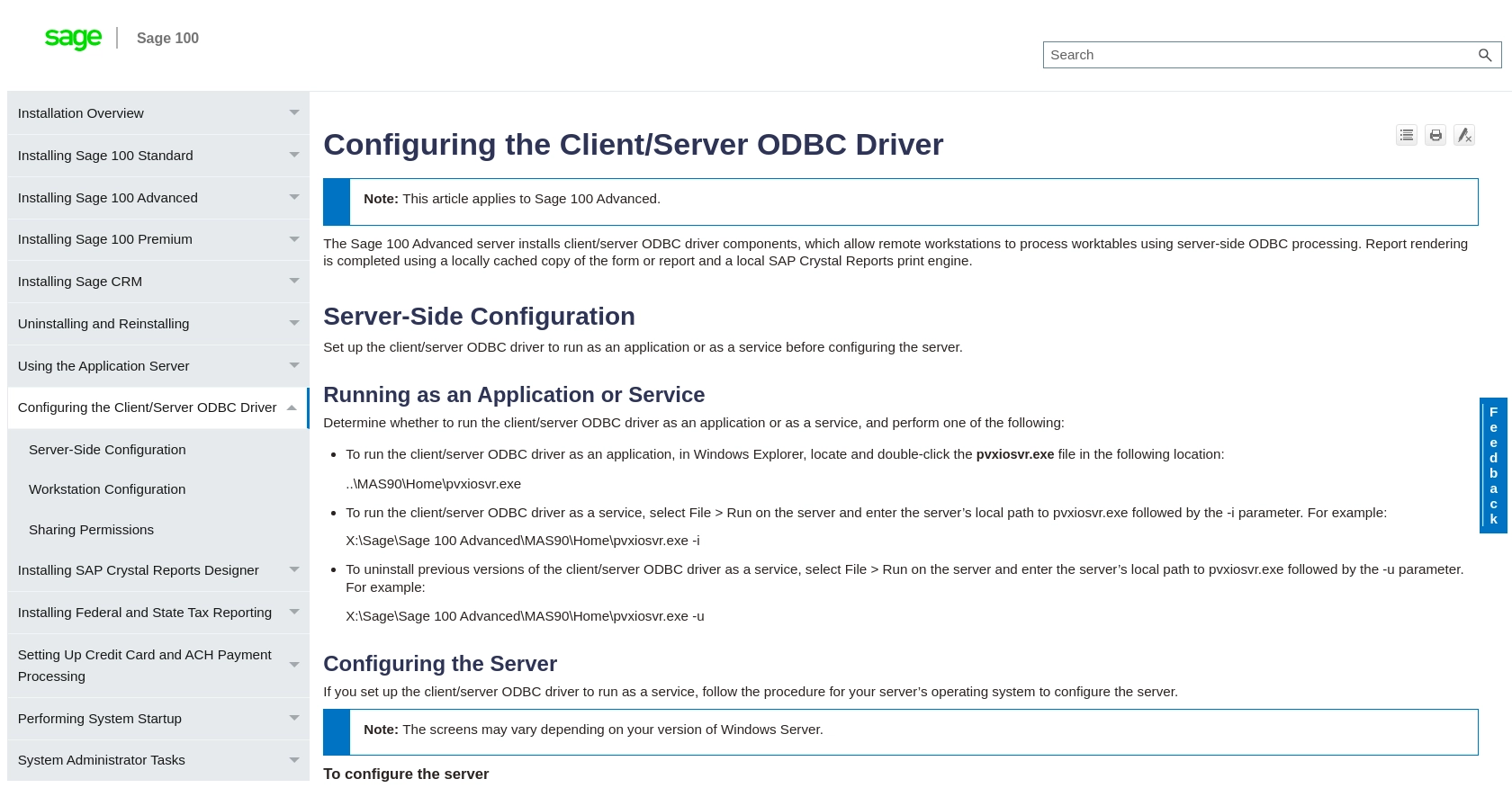
sbb-itb-96038d7
Making API Calls to Retrieve Purchase Orders from Sage 100 Using Python
To interact with the Sage 100 API and retrieve purchase orders, you'll need to use Python to establish a connection and execute the necessary queries. This section will guide you through the process of setting up your Python environment, writing the code to make API calls, and handling the responses effectively.
Setting Up Your Python Environment for Sage 100 API
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Python 3.x
- ODBC Driver for Sage 100
- Python packages:
pyodbc
andpandas
Install the required Python packages using pip:
pip install pyodbc pandas
Writing Python Code to Connect and Retrieve Purchase Orders
With your environment set up, you can now write the Python code to connect to the Sage 100 database and retrieve purchase orders. Below is a sample script demonstrating how to achieve this:
import pyodbc
import pandas as pd
# Define the DSN and connection parameters
dsn = 'Your_DSN_Name'
user = 'Your_Username'
password = 'Your_Password'
# Establish a connection to the Sage 100 database
connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}')
# SQL query to retrieve purchase orders
query = 'SELECT * FROM PO_PurchaseOrderHeader'
# Execute the query and fetch the results
purchase_orders = pd.read_sql(query, connection)
# Display the purchase orders
print(purchase_orders)
# Close the connection
connection.close()
Replace Your_DSN_Name
, Your_Username
, and Your_Password
with your actual DSN, username, and password.
Verifying API Call Success and Handling Errors
After executing the script, you should see the purchase orders printed in your console. To verify the success of your API call, cross-check the retrieved data with the records in your Sage 100 test environment.
It's crucial to handle potential errors gracefully. Use try-except blocks to catch exceptions and manage connection errors, timeouts, and query issues:
try:
# Establish a connection
connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}')
# Execute the query
purchase_orders = pd.read_sql(query, connection)
print(purchase_orders)
except pyodbc.Error as e:
print(f'Error: {e}')
finally:
# Ensure the connection is closed
if connection:
connection.close()
By following these steps, you can efficiently retrieve purchase orders from Sage 100 using Python, enabling seamless integration with your applications.
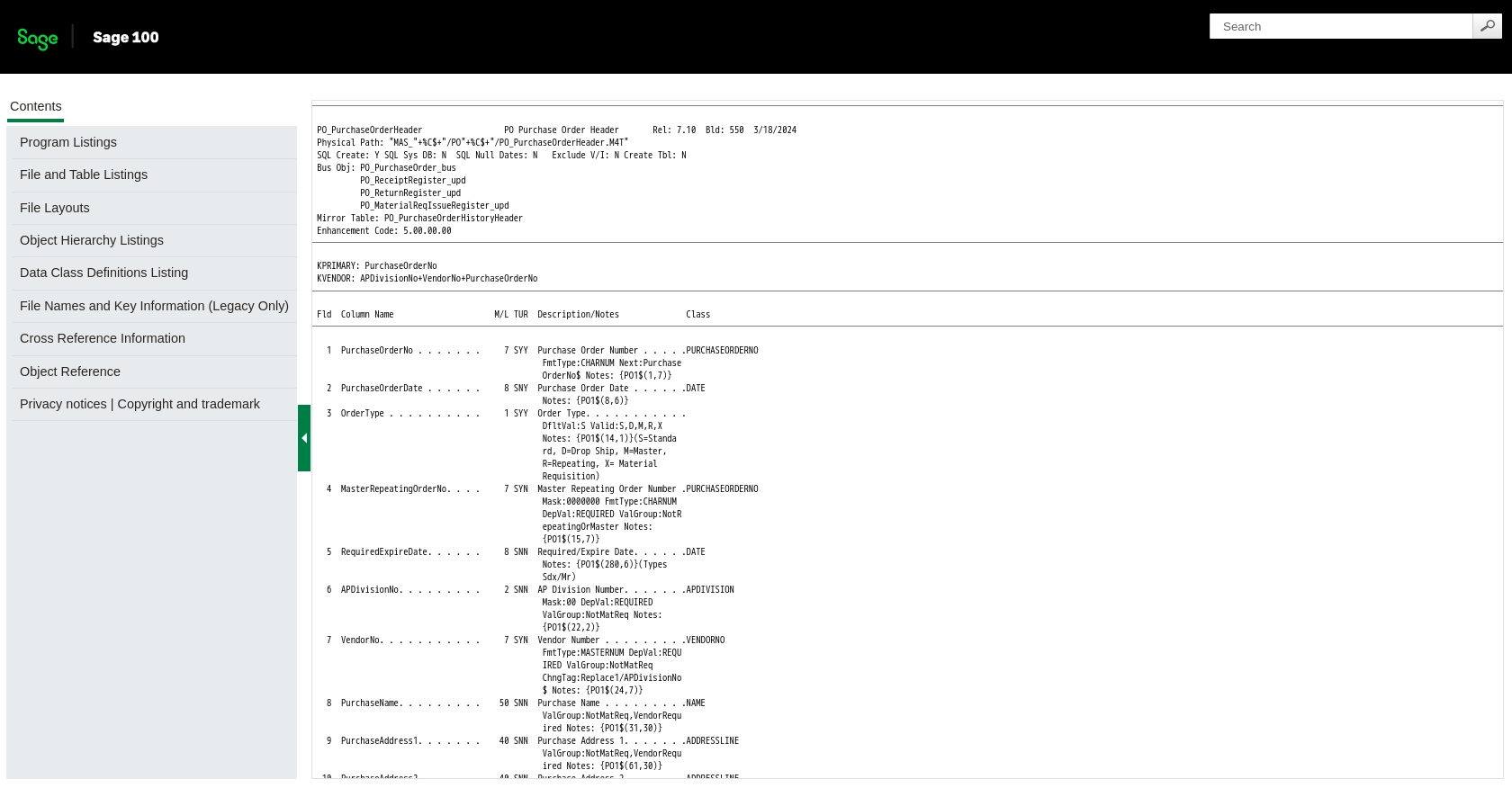
Conclusion and Best Practices for Using Sage 100 API with Python
Integrating with the Sage 100 API to retrieve purchase orders can significantly enhance your business operations by automating procurement processes and improving data visibility. By following the steps outlined in this article, you can efficiently connect to the Sage 100 ERP system using Python and manage purchase orders seamlessly.
Best Practices for Secure and Efficient Sage 100 API Integration
- Secure Credentials: Always store your DSN, username, and password securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from Sage 100 is standardized and transformed as needed to integrate smoothly with other systems.
- Error Handling: Use try-except blocks to manage exceptions and log errors for troubleshooting and auditing purposes.
Streamline Your Integrations with Endgrate
While integrating with Sage 100 API using Python is a powerful solution, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API that simplifies the integration process, allowing you to focus on your core product development. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Purchase_Order/PO_PurchaseOrderHeader.htm?Highlight=PO_PurchaseOrderHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Purchase_Order/PO_PurchaseOrderDetail.htm?Highlight=PO_PurchaseOrderDetail
Ready to get started?