How to Get Acounts with the Sage Accounting API in Javascript
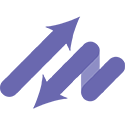
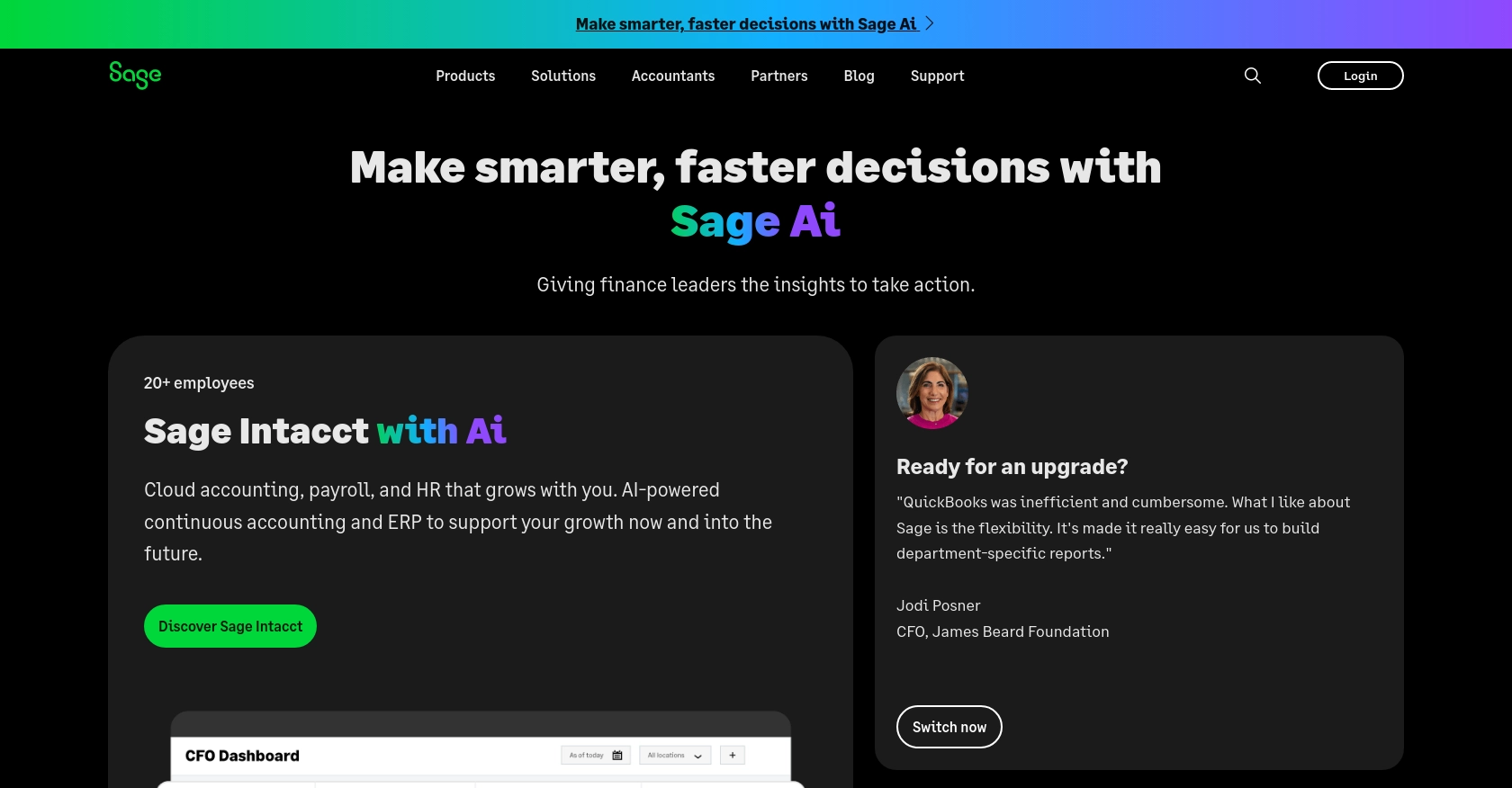
Introduction to Sage Accounting API
Sage Accounting is a comprehensive cloud-based accounting solution tailored for small to medium-sized businesses. It offers a suite of tools for managing finances, invoicing, and cash flow, making it an essential platform for businesses looking to streamline their accounting processes.
Integrating with the Sage Accounting API allows developers to automate financial tasks and access real-time financial data. For example, a developer might use the API to retrieve account information, enabling seamless integration of financial data into custom applications or dashboards.
Setting Up Your Sage Accounting Test/Sandbox Account
Before you can begin integrating with the Sage Accounting API, you need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting any live data.
Create a Sage Developer Account
To start, you'll need a Sage Developer account. This account will enable you to register and manage your applications, obtain client credentials, and specify key details such as callback URLs.
- Visit the Sage Developer Portal.
- Sign up using your GitHub account or an email address.
- Follow the guide on creating an app to get your Client ID and Client Secret.
Set Up a Trial Business for Development
Next, create a trial business account to use for development purposes. Sage offers trial accounts for various regions and subscription tiers, allowing you to test the API's functionality.
- Choose your region and subscription tier from the options available on the Sage Developer Portal.
- Sign up for a trial account using the provided links for your region.
Upgrade to a Developer Account
Once you have your trial account, you can request an upgrade to a developer account. This upgrade provides 12 months of free access to your Sage Accounting business for testing.
- Submit your upgrade request through the Sage Developer Portal.
- Provide the necessary details, including your name, email address, app name, and client ID.
- Wait for confirmation from Sage, which typically takes 3-5 working days.
Configure OAuth Authentication
Sage Accounting API uses OAuth for authentication. Follow these steps to configure it:
- Log in to your Sage Developer account and navigate to your app settings.
- Enter the callback URL for your application.
- Save your settings to generate the Client ID and Client Secret.
With your Sage Developer account and trial business set up, you're ready to start making API calls to Sage Accounting. In the next section, we'll cover how to make these calls using JavaScript.
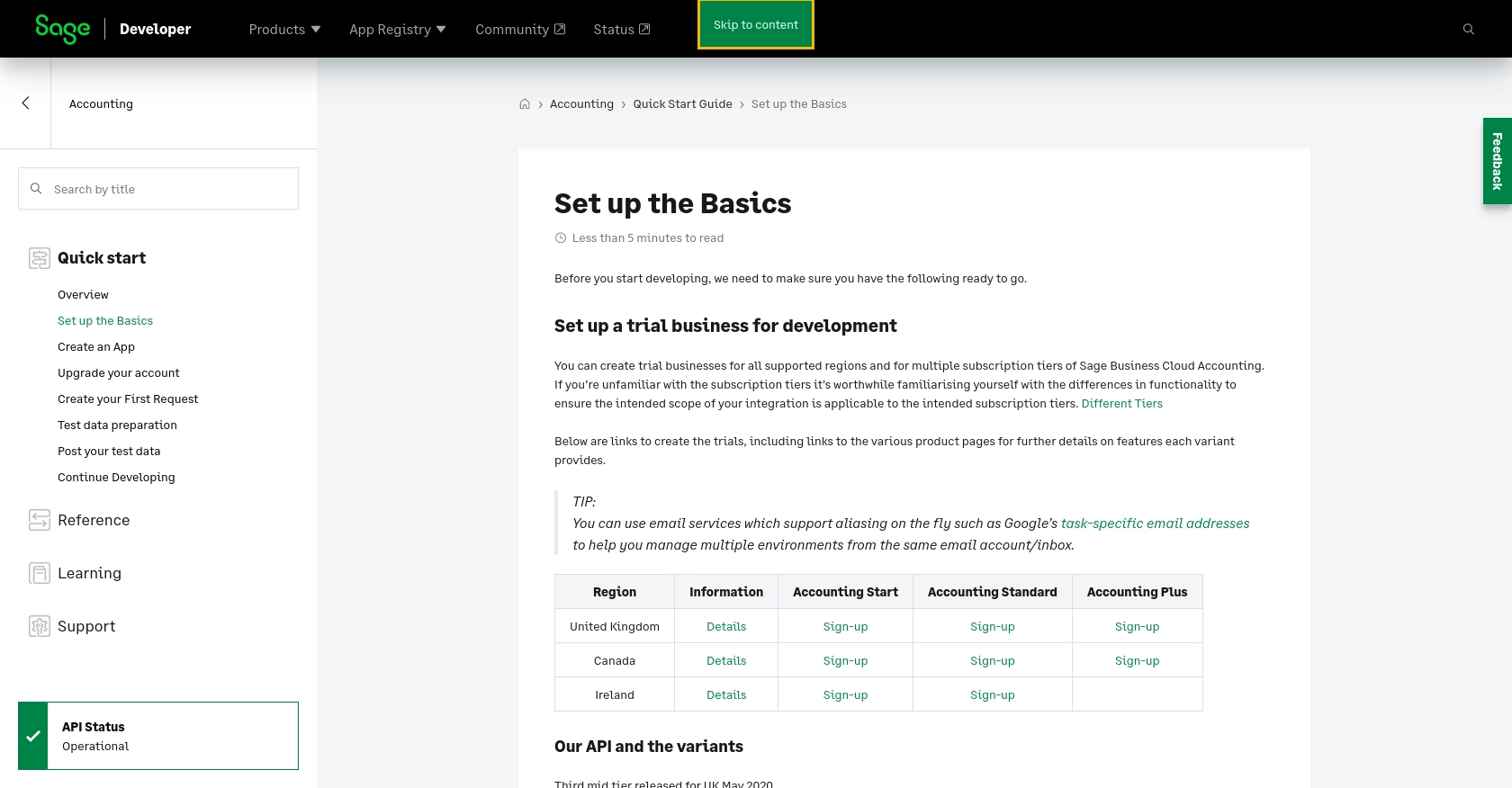
sbb-itb-96038d7
Making API Calls to Retrieve Accounts with Sage Accounting API in JavaScript
To interact with the Sage Accounting API and retrieve account information, you'll need to set up your JavaScript environment and make HTTP requests using the appropriate libraries. This section will guide you through the process of making API calls to Sage Accounting using JavaScript.
Prerequisites for Using JavaScript with Sage Accounting API
Before you begin, ensure you have the following installed on your machine:
- Node.js (version 14.x or later)
- NPM (Node Package Manager)
Once you have these installed, open your terminal and install the Axios library, which will be used to make HTTP requests:
npm install axios
Setting Up Your JavaScript Environment for Sage Accounting API
Create a new JavaScript file named getSageAccounts.js
and add the following code to it:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.sage.com/accounting/ledger-accounts';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get accounts from Sage Accounting
async function getAccounts() {
try {
const response = await axios.get(endpoint, { headers });
const accounts = response.data;
console.log(accounts);
} catch (error) {
console.error('Error fetching accounts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getAccounts();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth authentication setup.
Executing the JavaScript Code to Retrieve Accounts
Run the JavaScript file from your terminal using the following command:
node getSageAccounts.js
If successful, you should see a list of accounts retrieved from your Sage Accounting sandbox account displayed in the console.
Handling Errors and Verifying API Call Success
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a try-catch
block to catch and log any errors that occur during the API request.
To verify the success of your API call, check the response data in your console and ensure it matches the accounts data in your Sage Accounting sandbox account. If there are discrepancies, review the API documentation and your request setup.
For more detailed information on the API endpoints and error codes, refer to the Sage Accounting API documentation.
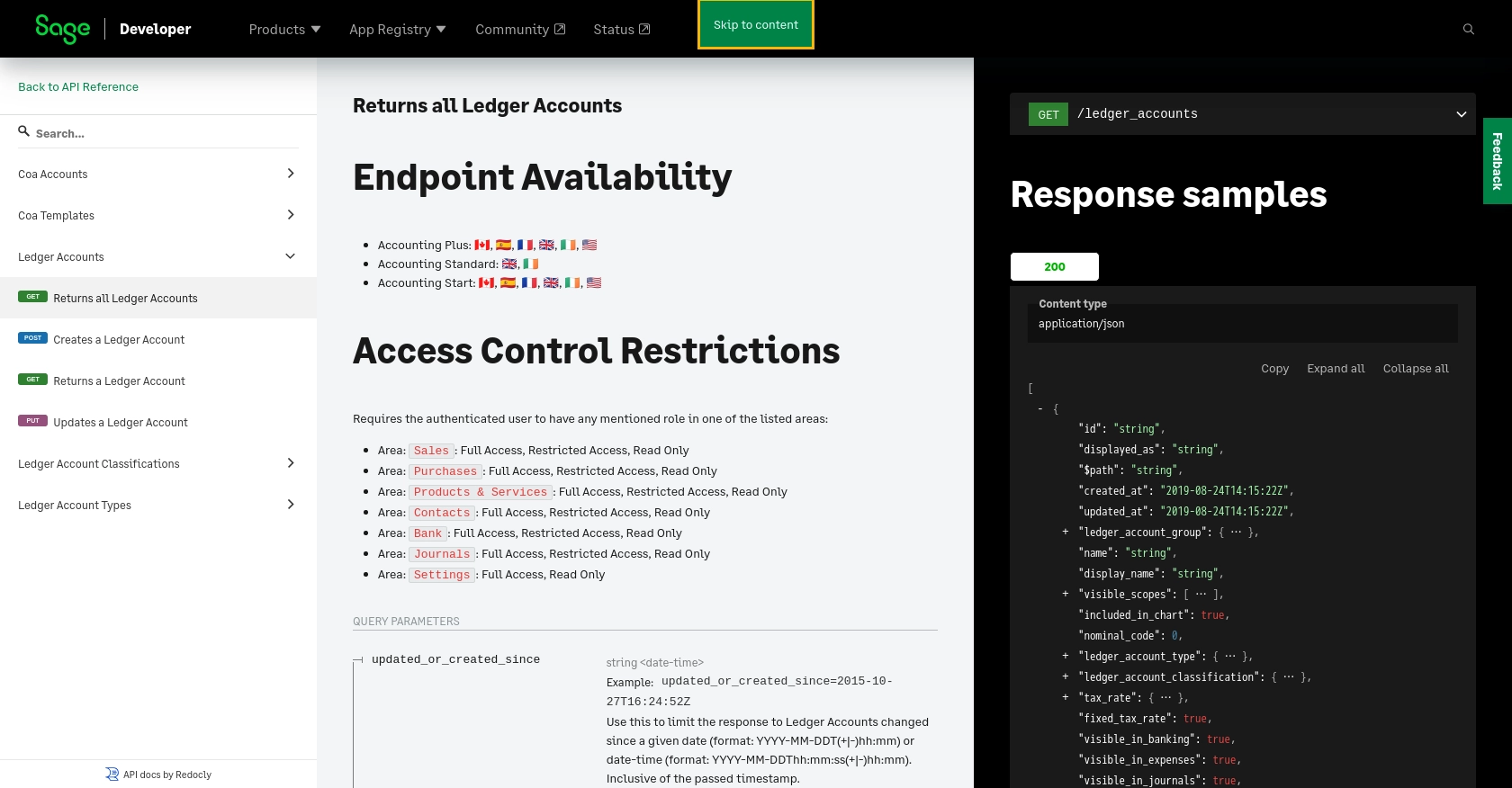
Conclusion and Best Practices for Using Sage Accounting API in JavaScript
Integrating with the Sage Accounting API using JavaScript provides a powerful way to automate financial tasks and access real-time data, enhancing the efficiency of your business operations. By following the steps outlined in this guide, you can successfully retrieve account information and integrate it into your applications.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as Client ID and Client Secret, securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your application’s data structures.
- Error Handling: Implement comprehensive error handling to manage different error codes and scenarios. Refer to the Sage Accounting API documentation for detailed error code information.
Streamline Your Integration Process with Endgrate
While integrating with Sage Accounting API can be a rewarding experience, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Sage Accounting.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration efforts.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/accounting-setup/#tag/Ledger-Accounts/operation/getLedgerAccounts
Ready to get started?