Using the Keap API to Get Contacts in Javascript
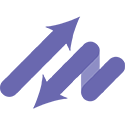
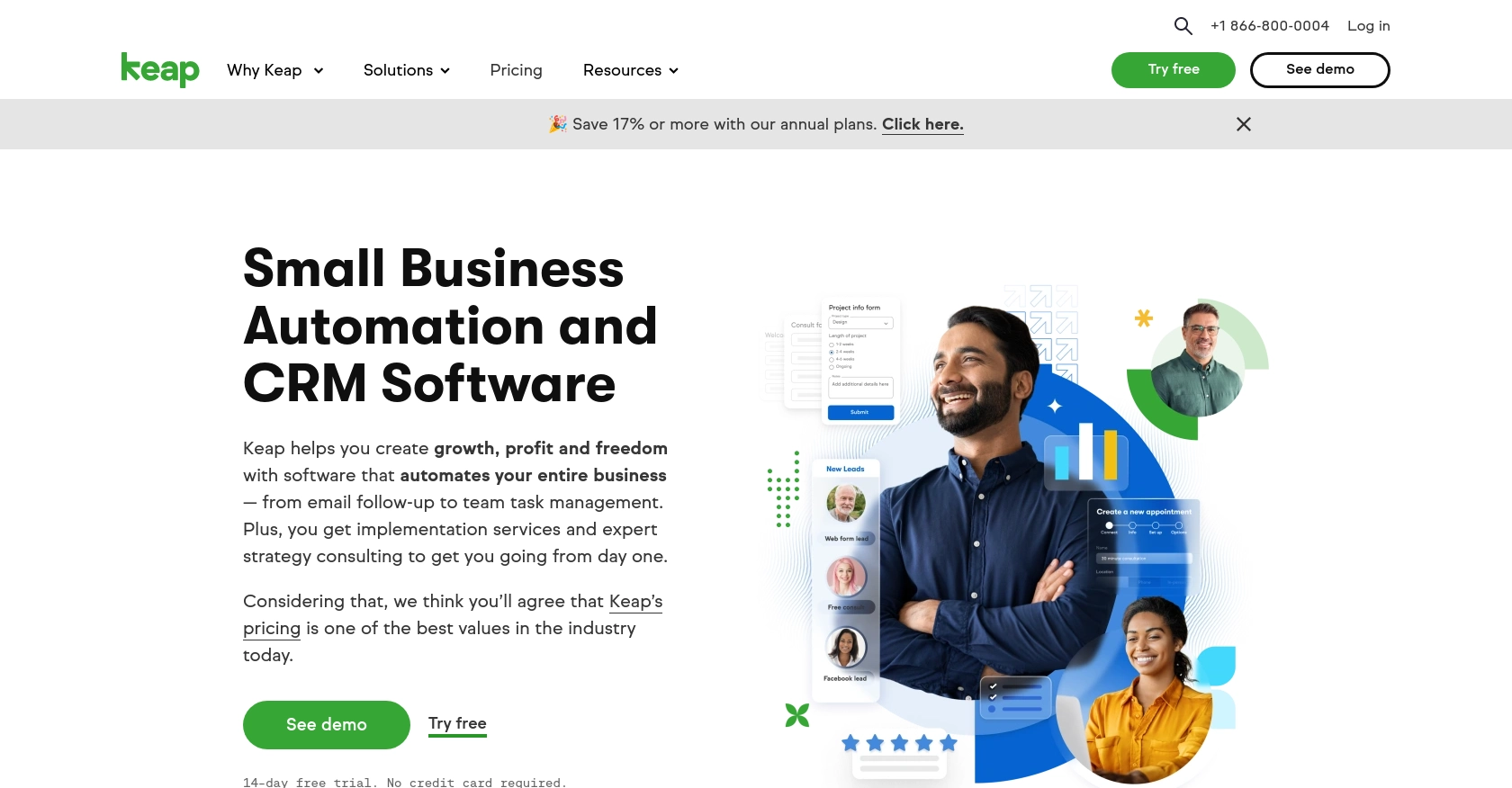
Introduction to Keap CRM
Keap, formerly known as Infusionsoft, is a robust CRM platform designed to help small businesses streamline their sales and marketing efforts. With features like contact management, email marketing, and automation, Keap empowers businesses to enhance customer relationships and drive growth.
Integrating with Keap's API allows developers to access and manage customer data programmatically. For example, you might want to retrieve contact information using JavaScript to automate follow-up emails or sync contacts with another application, enhancing workflow efficiency and data consistency.
Setting Up Your Keap Developer Account and Sandbox Environment
Before you can start interacting with the Keap API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your integrations without affecting live data.
Registering for a Keap Developer Account
To begin, you need to register for a Keap developer account. This account provides access to Keap's API documentation and tools necessary for building integrations.
- Visit the Keap Developer Portal.
- Click on the "Register" button to create a new account.
- Fill out the registration form with your details and submit it.
- Once registered, log in to your developer account to access the dashboard.
Creating a Keap Sandbox App
With your developer account set up, the next step is to create a sandbox app. This environment allows you to test API calls safely.
- In the developer dashboard, navigate to the "Sandbox Apps" section.
- Click on "Create New Sandbox App" and provide the necessary details.
- Once created, you'll receive a Client ID and Client Secret, which are essential for OAuth authentication.
Setting Up OAuth Authentication for Keap API
Keap's API uses OAuth 2.0 for authentication. Follow these steps to set up OAuth for your sandbox app:
- In your sandbox app settings, locate the "OAuth" section.
- Enter the redirect URI where users will be redirected after authentication.
- Save your changes to generate the authorization URL.
- Use the Client ID and Client Secret to request an access token by directing users to the authorization URL.
For more detailed information, refer to the Keap REST API documentation.
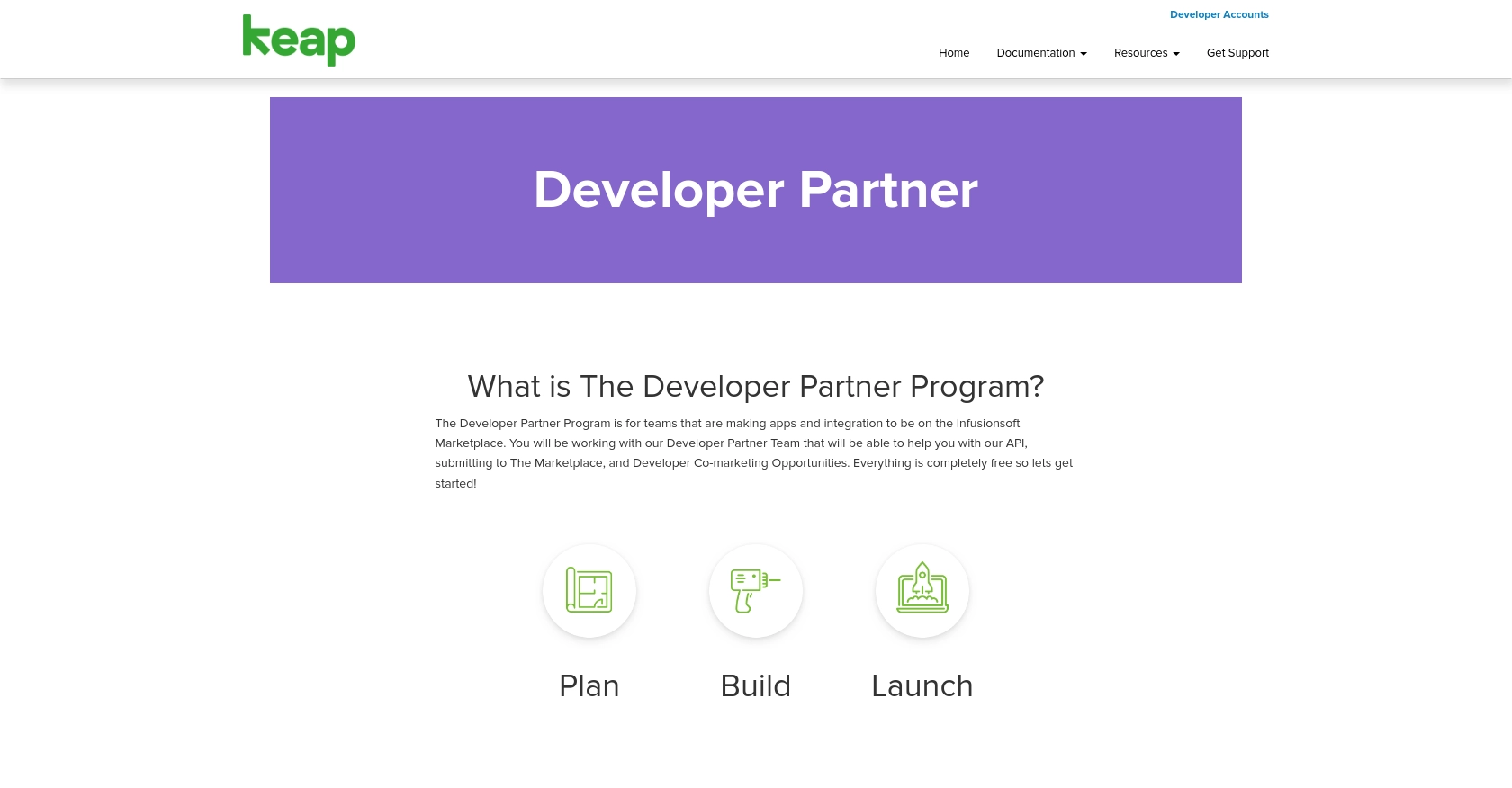
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Keap Using JavaScript
To interact with the Keap API and retrieve contacts using JavaScript, you'll need to set up your environment and write the necessary code to make API calls. This section will guide you through the process, ensuring you have the right tools and knowledge to successfully access Keap's contact data.
Setting Up Your JavaScript Environment for Keap API Integration
Before making API calls, ensure you have Node.js installed on your machine, as it provides the runtime environment for executing JavaScript code outside the browser. Additionally, you'll need the axios
library to handle HTTP requests.
- Download and install Node.js if you haven't already.
- Open your terminal and run the following command to install
axios
:
npm install axios
Writing JavaScript Code to Fetch Contacts from Keap API
With your environment set up, you can now write the JavaScript code to fetch contacts from the Keap API. The following example demonstrates how to make a GET request to retrieve contact data.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.infusionsoft.com/crm/rest/v1/contacts';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
const contacts = response.data.contacts;
console.log('Contacts retrieved:', contacts);
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getContacts();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth authentication process. This token is crucial for authorizing your API requests.
Verifying API Call Success and Handling Errors
After running the script, you should see the list of contacts printed in the console. If the request is successful, the contacts retrieved from your Keap sandbox environment will match those displayed in the Keap dashboard.
In case of errors, the code includes error handling to display relevant error messages. Common issues might include invalid tokens or incorrect endpoint URLs. Refer to the Keap REST API documentation for detailed error code explanations.
Conclusion and Best Practices for Keap API Integration
Integrating with the Keap API using JavaScript offers a powerful way to automate and streamline your CRM processes. By following the steps outlined in this article, you can efficiently retrieve contact data and enhance your application's functionality.
Best Practices for Secure and Efficient Keap API Usage
- Securely Store Credentials: Always store your OAuth tokens and client secrets securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be mindful of Keap's API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Standardize Data Fields: Ensure that contact data retrieved from Keap is standardized and consistent with your application's data model to maintain data integrity.
Streamline Your Integrations with Endgrate
While building integrations with the Keap API can be rewarding, it can also be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Keap. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more efficient way to manage your integrations.
Read More
Ready to get started?