Using the Chargebee API to Get Subscriptions in Javascript
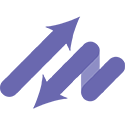
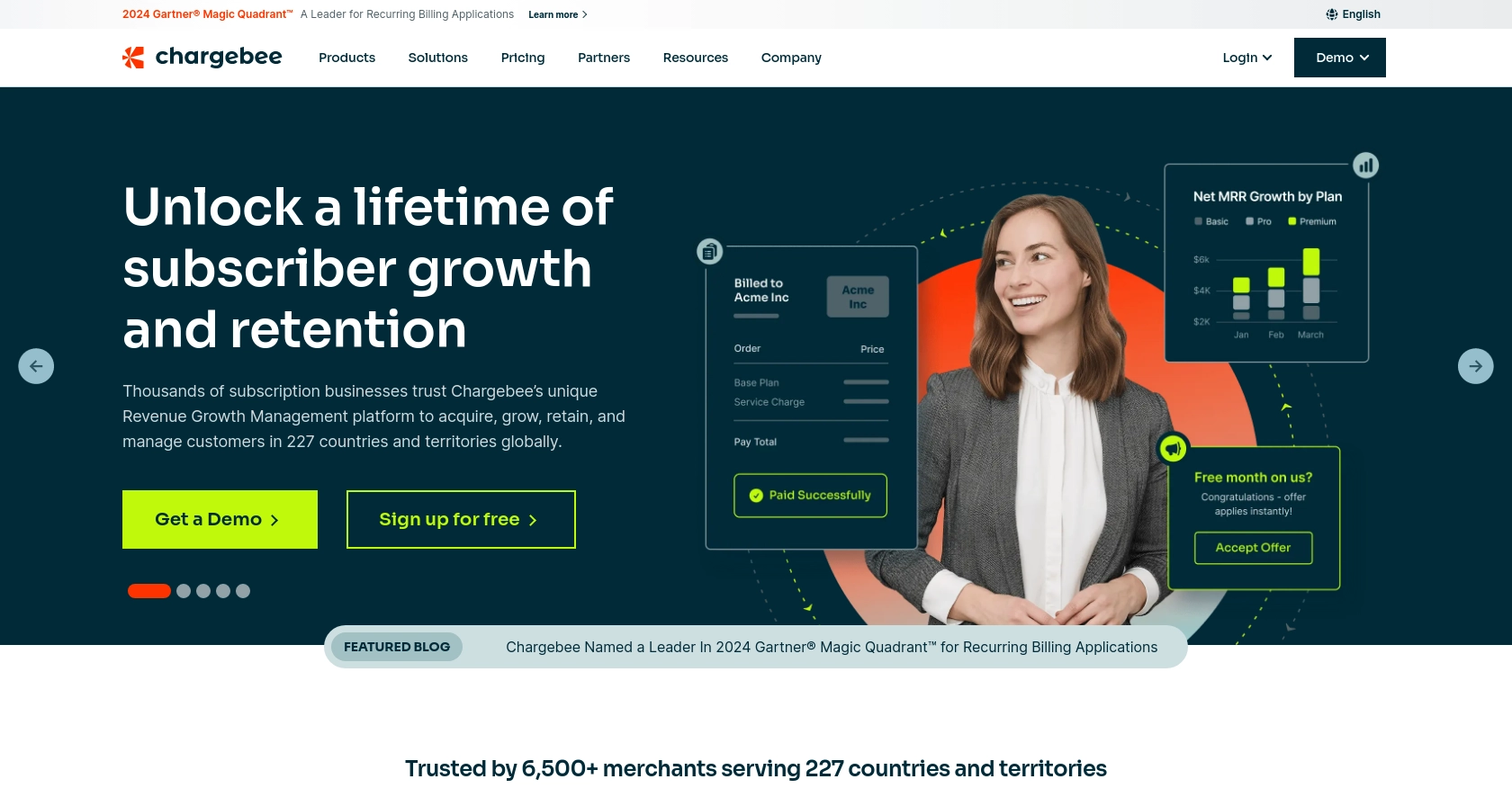
Introduction to Chargebee API Integration
Chargebee is a robust subscription management platform that empowers businesses to automate billing, invoicing, and revenue operations. It offers a comprehensive suite of tools designed to streamline subscription management for SaaS companies, e-commerce platforms, and other subscription-based businesses.
Integrating with Chargebee's API allows developers to efficiently manage subscriptions, automate billing processes, and access detailed customer data. For example, using the Chargebee API, developers can retrieve subscription details to analyze customer behavior or generate reports for financial forecasting.
This article will guide you through using JavaScript to interact with the Chargebee API, specifically focusing on retrieving subscription data. By the end of this tutorial, you'll be equipped to seamlessly integrate Chargebee's subscription management capabilities into your applications.
Setting Up Your Chargebee Test Account for API Integration
Before diving into the Chargebee API integration, it's essential to set up a test or sandbox account. This environment allows developers to experiment and test API interactions without affecting live data. Follow these steps to get started:
Step-by-Step Guide to Creating a Chargebee Test Account
-
Sign Up for a Chargebee Account:
Visit the Chargebee signup page and register for a free trial account. This account will serve as your sandbox environment.
-
Access the Chargebee Dashboard:
Once registered, log in to your Chargebee account to access the dashboard. This is where you'll manage your test data and API keys.
-
Navigate to API Settings:
In the dashboard, go to Settings > Configure Chargebee > API Keys. Here, you can generate API keys necessary for authentication.
-
Generate API Keys:
Create a new API key by clicking on Generate Key. Ensure you save this key securely, as it will be used to authenticate your API requests.
Understanding Chargebee API Authentication
Chargebee uses HTTP Basic authentication for API calls. The API key acts as the username, while the password is left empty. This method ensures secure access to your Chargebee data.
Example: Authenticating API Requests in JavaScript
// Example of setting up authentication for Chargebee API requests
const axios = require('axios');
const site = 'your-site-name';
const apiKey = 'your-api-key';
axios.defaults.auth = {
username: apiKey,
password: ''
};
axios.get(`https://${site}.chargebee.com/api/v2/subscriptions`)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching subscriptions:', error);
});
Replace your-site-name
and your-api-key
with your actual Chargebee site name and API key. This setup allows you to make authenticated requests to the Chargebee API using JavaScript.
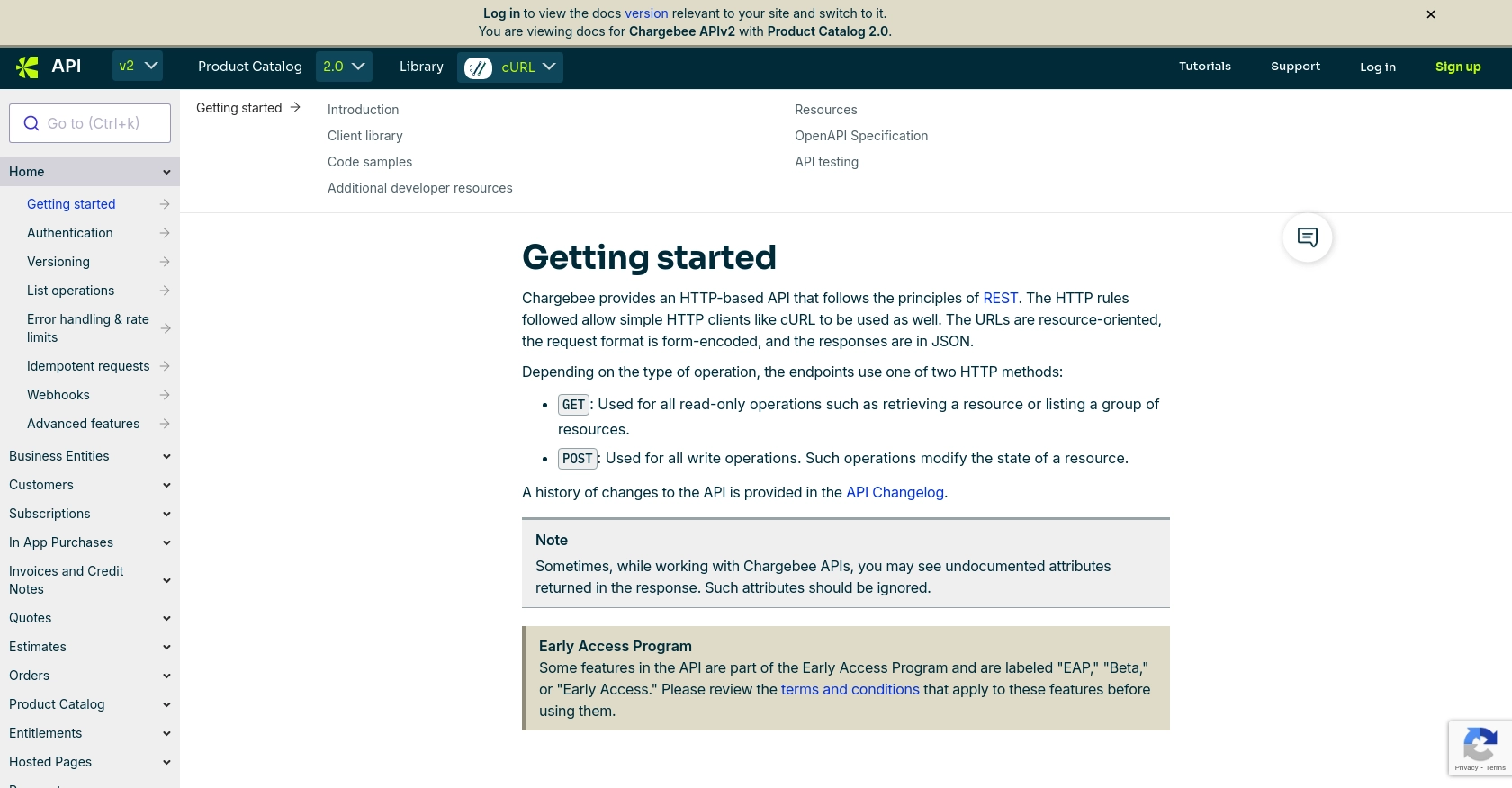
sbb-itb-96038d7
Making API Calls to Retrieve Subscriptions Using Chargebee API in JavaScript
To effectively interact with the Chargebee API and retrieve subscription data, you'll need to set up your JavaScript environment and make authenticated API calls. This section will guide you through the necessary steps to achieve this using JavaScript.
Setting Up Your JavaScript Environment for Chargebee API Integration
Before making API calls, ensure your environment is ready. You'll need Node.js installed on your machine, along with the Axios library for making HTTP requests.
-
Install Node.js:
Download and install Node.js from the official website. This will also install npm, the Node package manager.
-
Install Axios:
Open your terminal and run the following command to install Axios:
npm install axios
Example Code: Retrieving Subscriptions from Chargebee API
With your environment set up, you can now write a script to retrieve subscriptions from Chargebee. Below is an example code snippet demonstrating how to make a GET request to the Chargebee API using Axios:
// Import the Axios library
const axios = require('axios');
// Set your Chargebee site name and API key
const site = 'your-site-name';
const apiKey = 'your-api-key';
// Configure Axios for HTTP Basic authentication
axios.defaults.auth = {
username: apiKey,
password: ''
};
// Define the API endpoint for retrieving subscriptions
const endpoint = `https://${site}.chargebee.com/api/v2/subscriptions`;
// Make the GET request to the Chargebee API
axios.get(endpoint)
.then(response => {
// Handle successful response
console.log('Subscriptions:', response.data);
})
.catch(error => {
// Handle errors
console.error('Error fetching subscriptions:', error);
});
Replace your-site-name
and your-api-key
with your actual Chargebee site name and API key. This code will fetch subscription data and log it to the console.
Understanding the Response and Handling Errors
Upon a successful API call, the Chargebee API will return a JSON response containing subscription details. You can parse this data to extract the information you need.
In case of errors, the API will return an error code and message. Common error codes include:
- 401 Unauthorized: Incorrect API key or authentication details.
- 404 Not Found: The requested resource does not exist.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
For more detailed error handling, refer to the Chargebee API documentation.
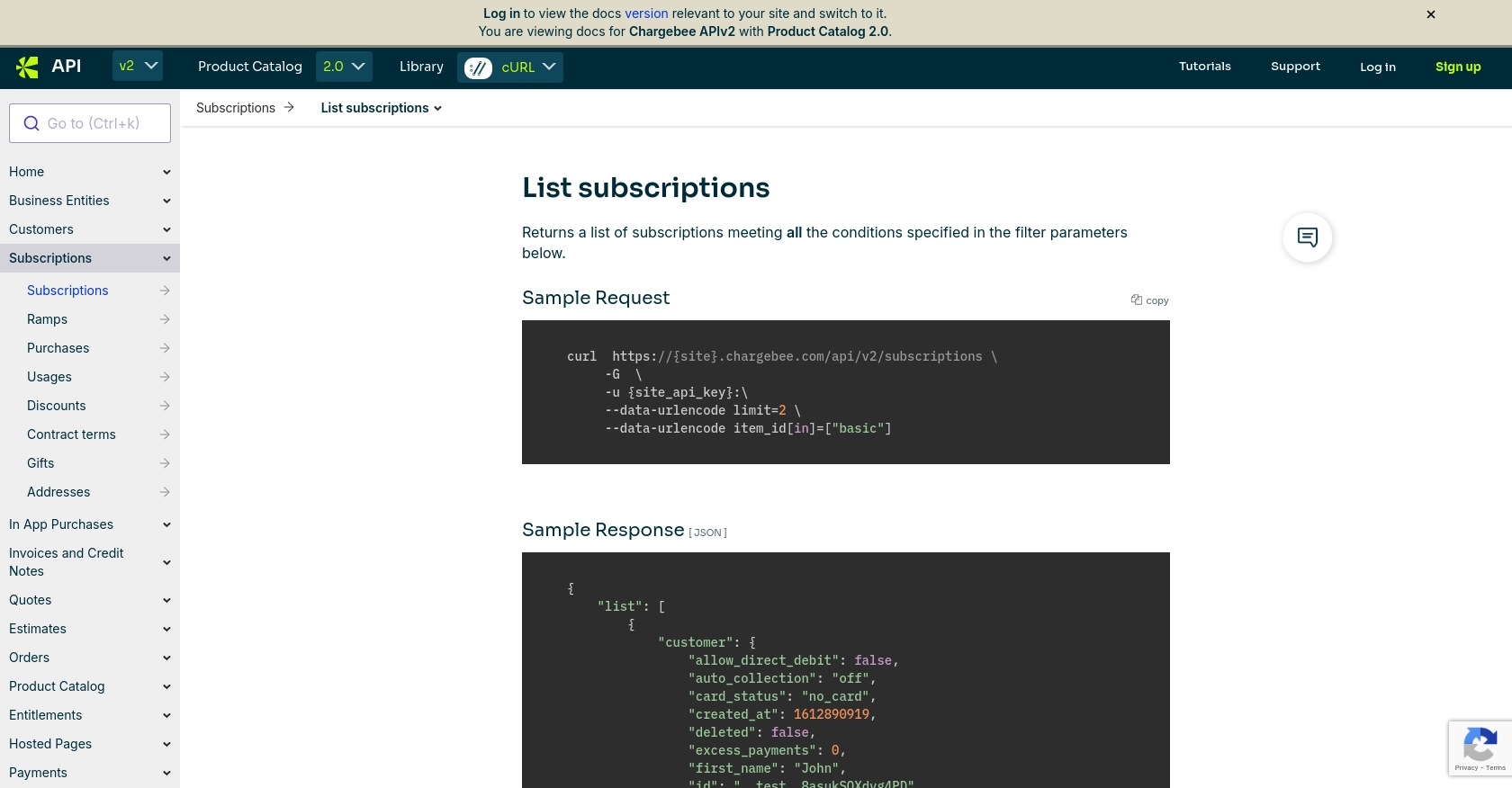
Conclusion and Best Practices for Chargebee API Integration
Integrating Chargebee's API into your JavaScript applications provides a powerful way to manage subscriptions and automate billing processes. By following the steps outlined in this guide, you can efficiently retrieve subscription data and enhance your application's functionality.
Best Practices for Secure and Efficient API Usage
- Secure API Keys: Store your API keys securely and avoid hardcoding them in your application. Consider using environment variables or a secure vault.
- Handle Rate Limits: Chargebee imposes rate limits on API requests. Implement exponential backoff strategies to handle HTTP 429 errors gracefully. For more details, refer to the Chargebee API documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your application.
Enhance Your Integration with Endgrate
For developers looking to streamline multiple integrations, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations and focusing on your core product. Build once for each use case instead of multiple times for different integrations, and provide an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration processes by visiting Endgrate.
Read More
Ready to get started?