Using the Nimble API to Get Contacts in Javascript
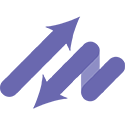
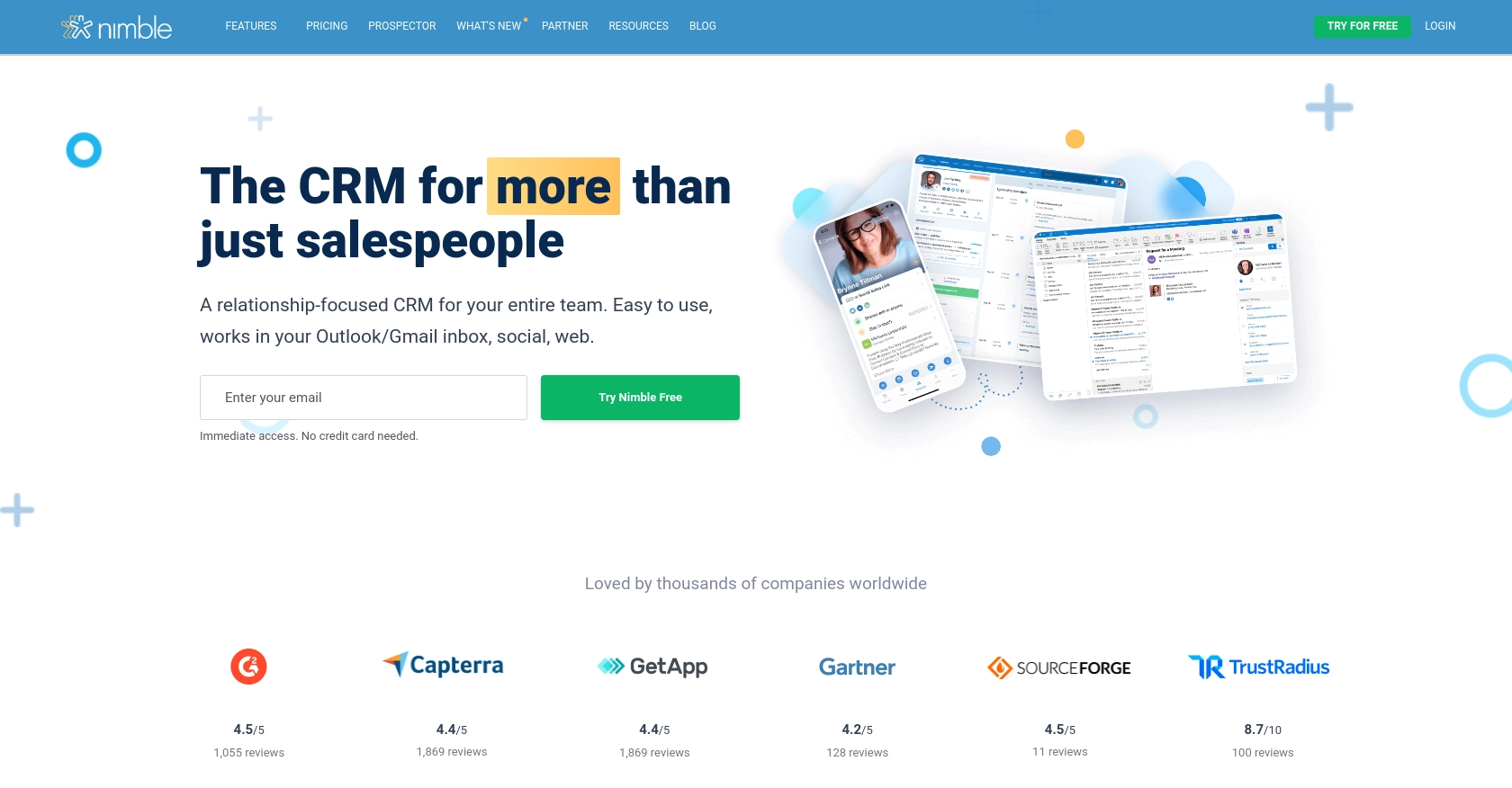
Introduction to Nimble CRM
Nimble is a relationship-focused CRM platform that helps businesses manage their contacts, tasks, and deals efficiently. With its seamless integration capabilities, Nimble connects with Microsoft 365 and Google Workspace, allowing users to gather and manage contact information from various sources effortlessly.
Developers may want to integrate with Nimble's API to automate and streamline CRM processes. For example, using the Nimble API, you can retrieve contact information to synchronize with other business applications, ensuring that your team always has access to the most up-to-date customer data.
Setting Up Your Nimble API Test Account
Before you can start using the Nimble API to retrieve contacts, you need to set up a test account. This will allow you to safely experiment with the API without affecting your live data.
Create a Nimble Account
If you don't already have a Nimble account, you can sign up for a free trial on the Nimble website. This will give you access to the CRM features and allow you to generate an API key.
Generate an API Key for Nimble
Once your account is set up, follow these steps to generate an API key:
- Log in to your Nimble account.
- Navigate to Settings and select API Token.
- Click on Generate New Token.
- Provide a description for your token and click Generate.
- Copy the generated API key and store it securely. You will use this key to authenticate your API requests.
Note: Only Nimble Account Administrators can generate API keys. Ensure you have the necessary permissions or contact your account admin for access.
Grant API Access in Nimble
Ensure that API access is granted by the admin of your Nimble account. Admins can do this by navigating to Settings > Users and enabling API access for the desired users.
For more detailed instructions, refer to the Nimble API documentation.
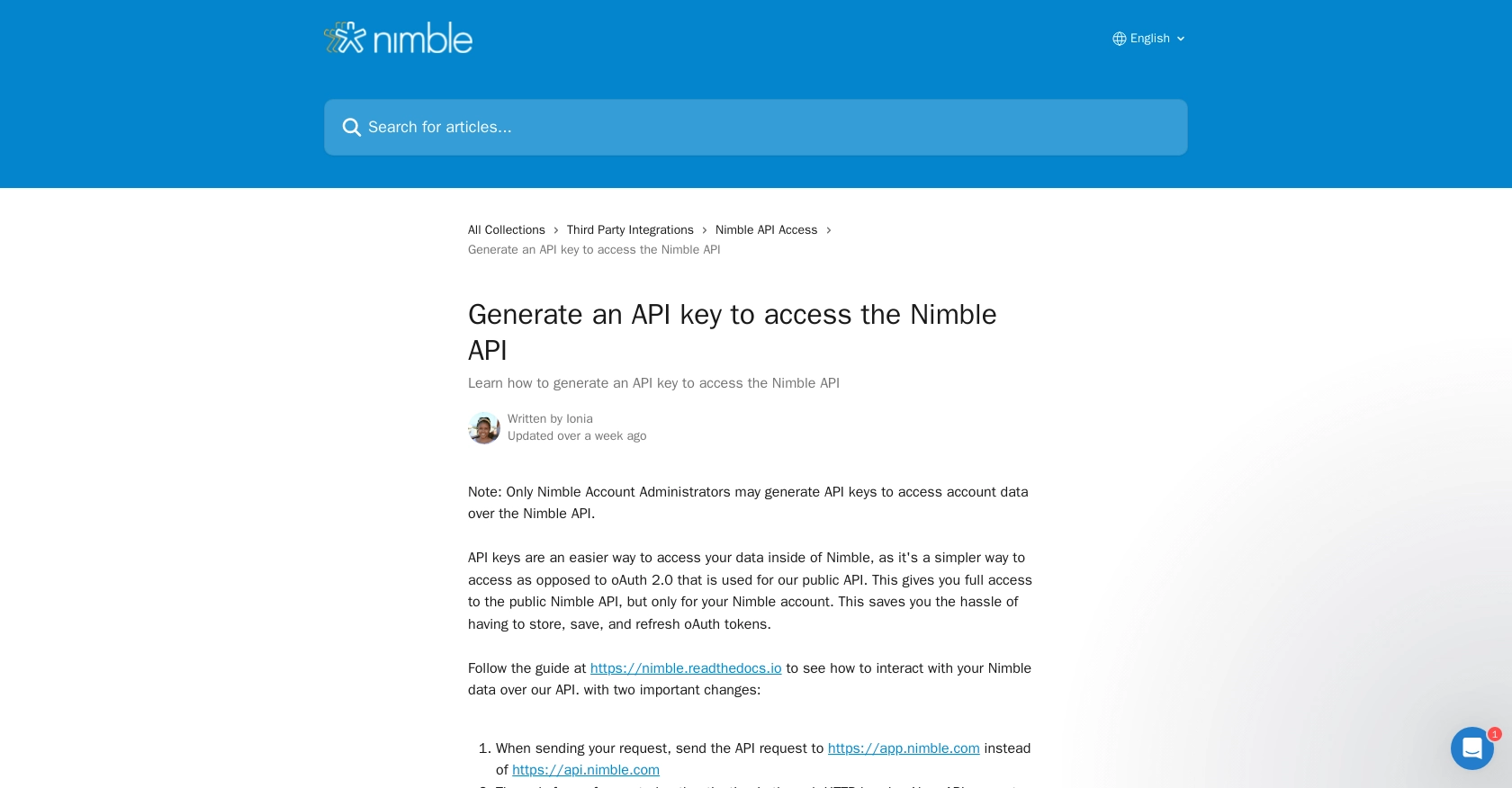
sbb-itb-96038d7
Making API Calls to Retrieve Contacts Using Nimble API in JavaScript
To interact with the Nimble API using JavaScript, you'll need to ensure you have the correct setup and understand the necessary steps to make API calls. This section will guide you through the process of retrieving contacts from Nimble using JavaScript.
Prerequisites for Using JavaScript with Nimble API
Before you begin, ensure you have the following:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- The
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Example Code to Retrieve Contacts from Nimble
Here's a sample code snippet to help you get started with retrieving contacts from Nimble:
const axios = require('axios');
// Your Nimble API key
const apiKey = 'YOUR_API_KEY';
// Set the API endpoint and headers
const endpoint = 'https://app.nimble.com/api/v1/contacts';
const headers = {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
};
// Function to get contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
const contacts = response.data.resources;
console.log('Contacts:', contacts);
} catch (error) {
console.error('Error fetching contacts:', error.response.data);
}
}
// Call the function to retrieve contacts
getContacts();
Replace YOUR_API_KEY
with the API key you generated earlier. This code sets up the necessary headers and makes a GET request to the Nimble API to fetch contacts.
Handling API Response and Errors
After making the API call, you should verify the response to ensure it succeeded. The response will contain a list of contacts if successful. If there's an error, the code will log the error message. Common error codes include:
- 409: Validation Error
- 402: Quota Error
- 500: Server Error
For more details on error handling, refer to the Nimble API documentation.
Conclusion and Best Practices for Using Nimble API in JavaScript
Integrating with the Nimble API using JavaScript can significantly enhance your CRM capabilities by automating data synchronization and ensuring your team has access to the latest customer information. By following the steps outlined in this guide, you can efficiently retrieve and manage contacts within Nimble.
Best Practices for Secure and Efficient API Integration
- Secure API Keys: Always store your API keys securely and avoid hardcoding them directly into your codebase. Consider using environment variables or a secure vault for storage.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid exceeding them. Implement exponential backoff strategies to handle retries gracefully.
- Data Standardization: Ensure that data retrieved from Nimble is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different error codes and scenarios, ensuring your application can recover gracefully from API failures.
Explore More with Endgrate for Seamless Integrations
While integrating with Nimble API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Visit Endgrate to learn how you can save time and resources by outsourcing your integrations and enhancing your product's capabilities.
Read More
Ready to get started?