Using the Sap Business One API to Create or Update Items (with Javascript examples)
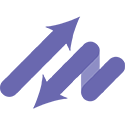
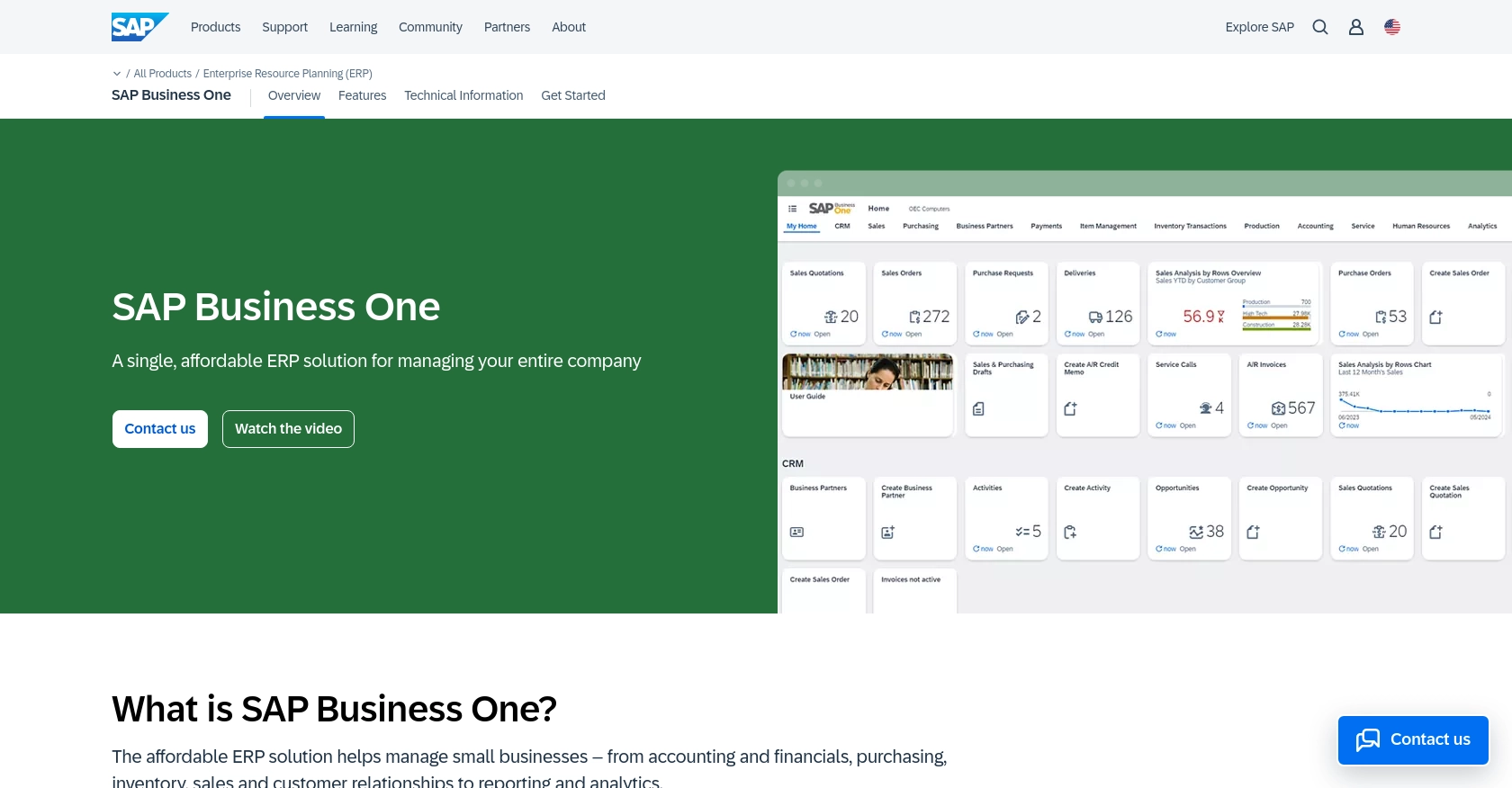
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to medium-sized businesses. It offers a range of functionalities, including financial management, sales, customer relationship management, and inventory control, all within a single platform.
Integrating with the SAP Business One API allows developers to streamline business processes by automating tasks such as creating or updating inventory items. For example, a developer might use the API to automatically update product details in the inventory system whenever changes are made in an external database, ensuring data consistency across platforms.
Setting Up Your SAP Business One Test or Sandbox Account
Before you can start integrating with the SAP Business One API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a SAP Business One Sandbox Account
To begin, you need access to a SAP Business One sandbox account. If you don't have one, you can request access through SAP's official channels or contact your SAP Business One provider for a demo environment.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method. Follow these steps to configure your authentication:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer settings.
- Create a new application and note down the Client ID and Client Secret.
- Set the necessary scopes for accessing and modifying items in your inventory.
- Save your changes and ensure you have the OAuth token ready for API calls.
For more detailed information on authentication, refer to the SAP Business One Service Layer documentation.
Generating API Keys for SAP Business One
If your integration requires API key-based access, follow these steps:
- Access the API management section in your SAP Business One account.
- Generate a new API key and restrict its permissions to only the necessary endpoints for creating or updating items.
- Store the API key securely, as you'll need it for making API requests.
With your sandbox account and authentication configured, you're ready to start making API calls to create or update items in SAP Business One using JavaScript.
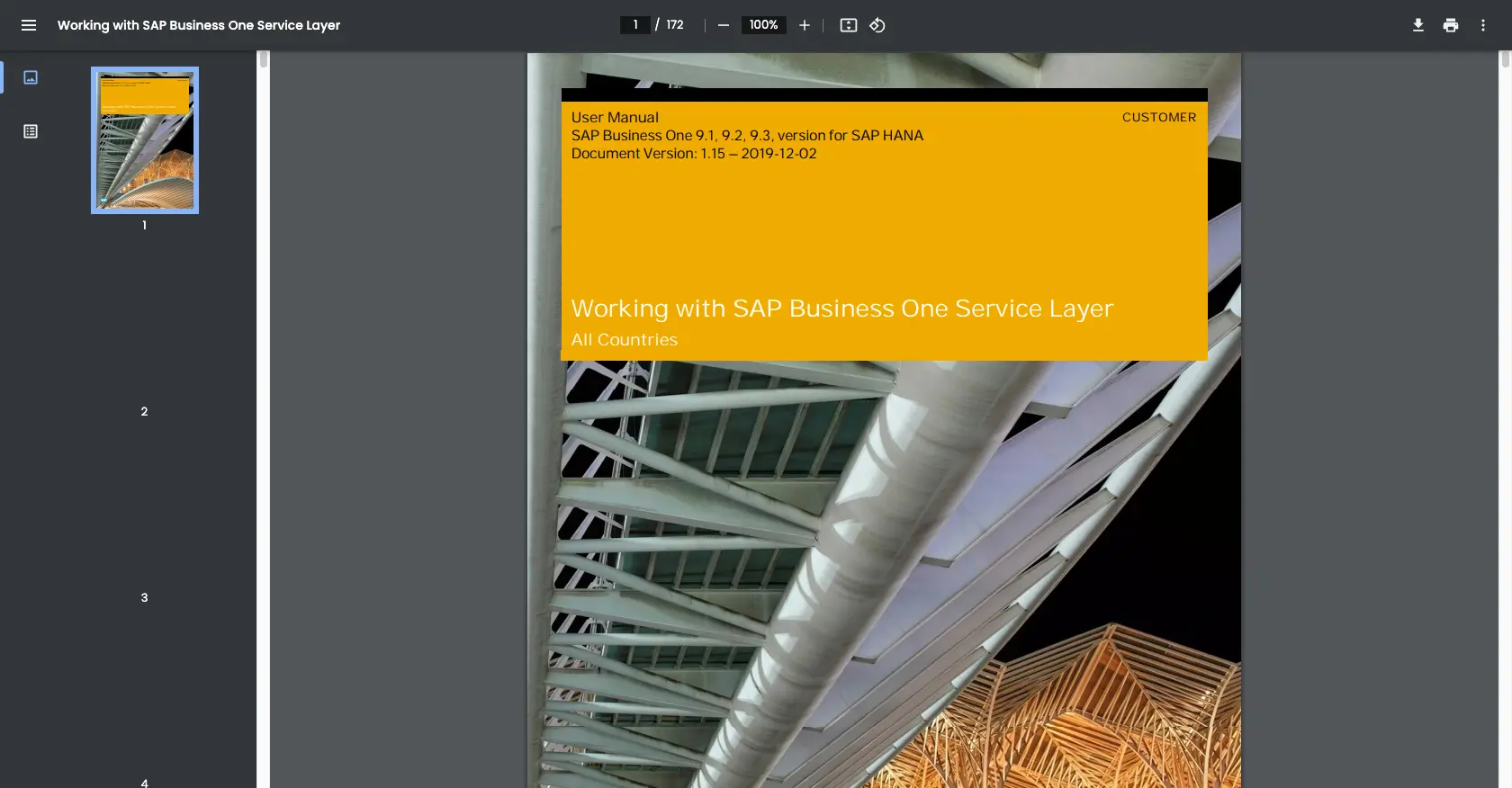
sbb-itb-96038d7
Making API Calls to SAP Business One with JavaScript
To interact with the SAP Business One API using JavaScript, you'll need to set up your development environment and write code to create or update items in your inventory. This section will guide you through the necessary steps, including setting up your JavaScript environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for SAP Business One API
Before you begin, ensure you have the following installed on your machine:
- Node.js (version 14 or later)
- NPM (Node Package Manager)
Once you have these prerequisites, install the axios
library to handle HTTP requests:
npm install axios
Creating or Updating Items Using SAP Business One API
With your environment set up, you can now write JavaScript code to create or update items in SAP Business One. Below is an example of how to perform these operations using the axios
library.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/Items';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_OAuth_Token'
};
// Define the item data
const itemData = {
ItemCode: 'A0001',
ItemName: 'Sample Item',
ItemType: 'itInventory',
InventoryItem: 'tYES'
};
// Function to create or update an item
async function createOrUpdateItem() {
try {
const response = await axios.post(endpoint, itemData, { headers });
console.log('Item created or updated successfully:', response.data);
} catch (error) {
console.error('Error creating or updating item:', error.response ? error.response.data : error.message);
}
}
// Call the function
createOrUpdateItem();
Replace Your_OAuth_Token
with the token you obtained during the authentication setup. This script sends a POST request to the SAP Business One API to create or update an item with the specified data.
Verifying API Call Success and Handling Errors
After running the script, you can verify the success of the API call by checking your SAP Business One sandbox account. The newly created or updated item should appear in your inventory.
In case of errors, the script logs the error message to the console. Common error codes include:
- 400 Bad Request: Indicates a malformed request. Check your request data and headers.
- 401 Unauthorized: Indicates an authentication issue. Verify your OAuth token.
- 404 Not Found: Indicates an incorrect endpoint URL.
Refer to the SAP Business One Service Layer documentation for more detailed error handling information.
Best Practices for Using SAP Business One API
When working with the SAP Business One API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth tokens and API keys securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by SAP Business One. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility between SAP Business One and your external systems.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for troubleshooting and alert your team to critical issues.
Streamlining Integrations with Endgrate
Building and maintaining integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including SAP Business One. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?