Using the BigCommerce API to Get Orders in Javascript
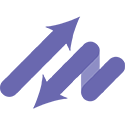
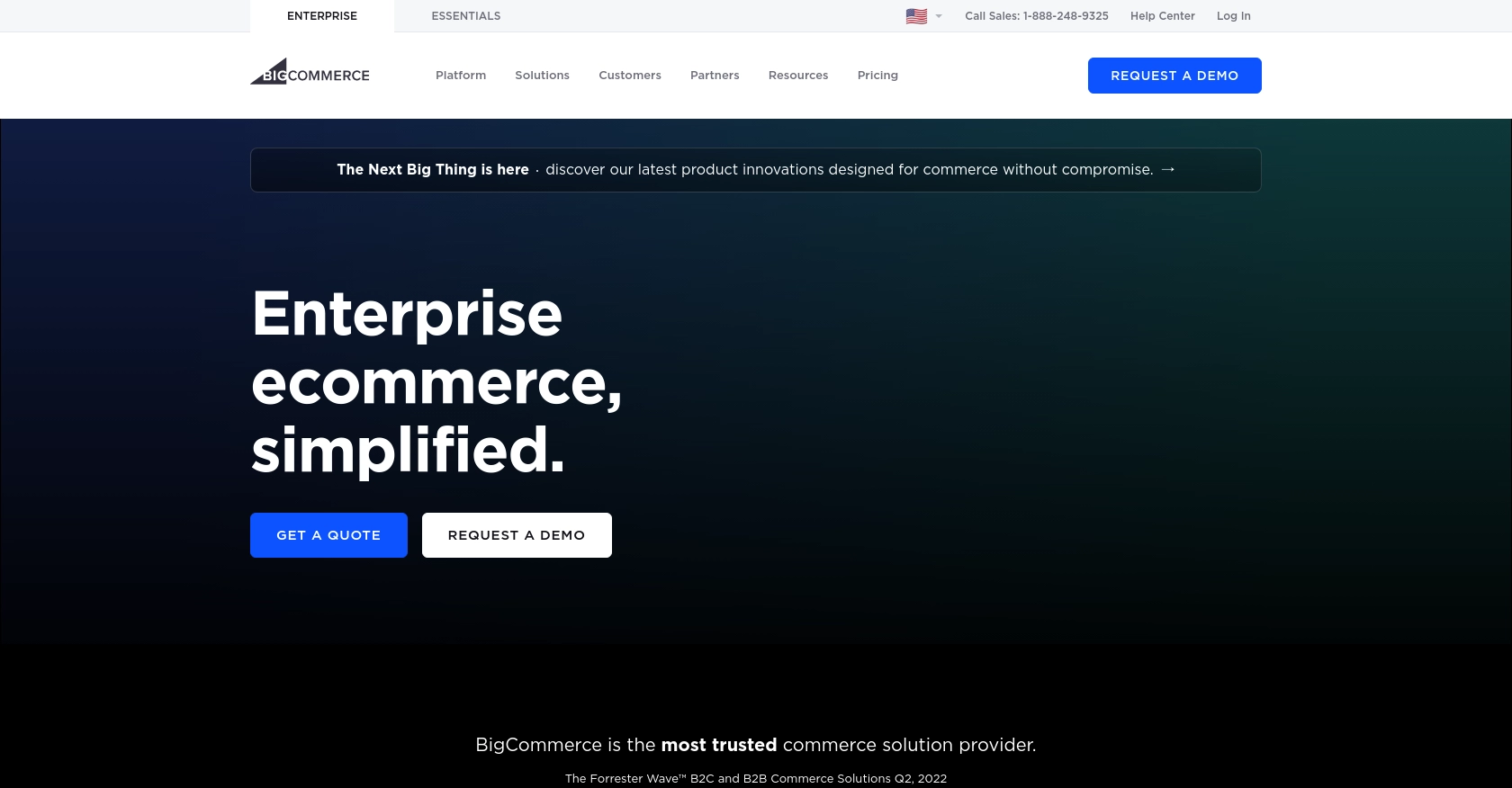
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a comprehensive suite of tools for managing products, orders, and customer relationships, making it a popular choice for businesses looking to scale their online presence.
Integrating with the BigCommerce API allows developers to automate and streamline various e-commerce operations. For example, you can use the API to retrieve order data, enabling you to analyze sales trends, manage inventory, or integrate with third-party logistics providers for efficient order fulfillment.
This article will guide you through the process of using JavaScript to interact with the BigCommerce API, specifically focusing on retrieving order information. By the end of this tutorial, you will be able to seamlessly integrate order data into your applications, enhancing your e-commerce capabilities.
Setting Up Your BigCommerce Test or Sandbox Account
Before you can start integrating with the BigCommerce API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a BigCommerce Developer Account
If you don't already have a BigCommerce account, you'll need to create one. Visit the BigCommerce website and sign up for a developer account. This will give you access to the necessary tools and resources for API integration.
Access the BigCommerce Control Panel
Once your account is set up, log in to the BigCommerce control panel. This is where you'll manage your store settings and API credentials.
Generate API Credentials
To interact with the BigCommerce API, you'll need to generate API credentials. Follow these steps:
- Navigate to Advanced Settings in the control panel.
- Select API Accounts and click on Create API Account.
- Choose a name for your API account and set the necessary permissions for accessing orders.
- Click Save to generate your Client ID, Client Secret, and Access Token.
Make sure to store these credentials securely, as they are required for authenticating your API requests.
Configure OAuth Scopes
Ensure your API account has the appropriate OAuth scopes configured. For retrieving orders, you'll need scopes that allow read access to order data. Refer to the BigCommerce authentication documentation for detailed information on setting scopes.
Test Your API Connection
With your API credentials ready, you can test your connection to the BigCommerce API. Use a tool like Postman to send a test request to the orders endpoint:
const fetchOrders = async () => {
const response = await fetch('https://api.bigcommerce.com/stores/{store_hash}/v2/orders', {
method: 'GET',
headers: {
'X-Auth-Token': 'your_access_token',
'Accept': 'application/json'
}
});
const data = await response.json();
console.log(data);
};
fetchOrders();
Replace your_access_token
with your actual access token and {store_hash}
with your store's unique hash. If successful, you should see a list of orders returned in the console.
By following these steps, you can ensure a smooth setup process, allowing you to focus on integrating and utilizing the BigCommerce API effectively.
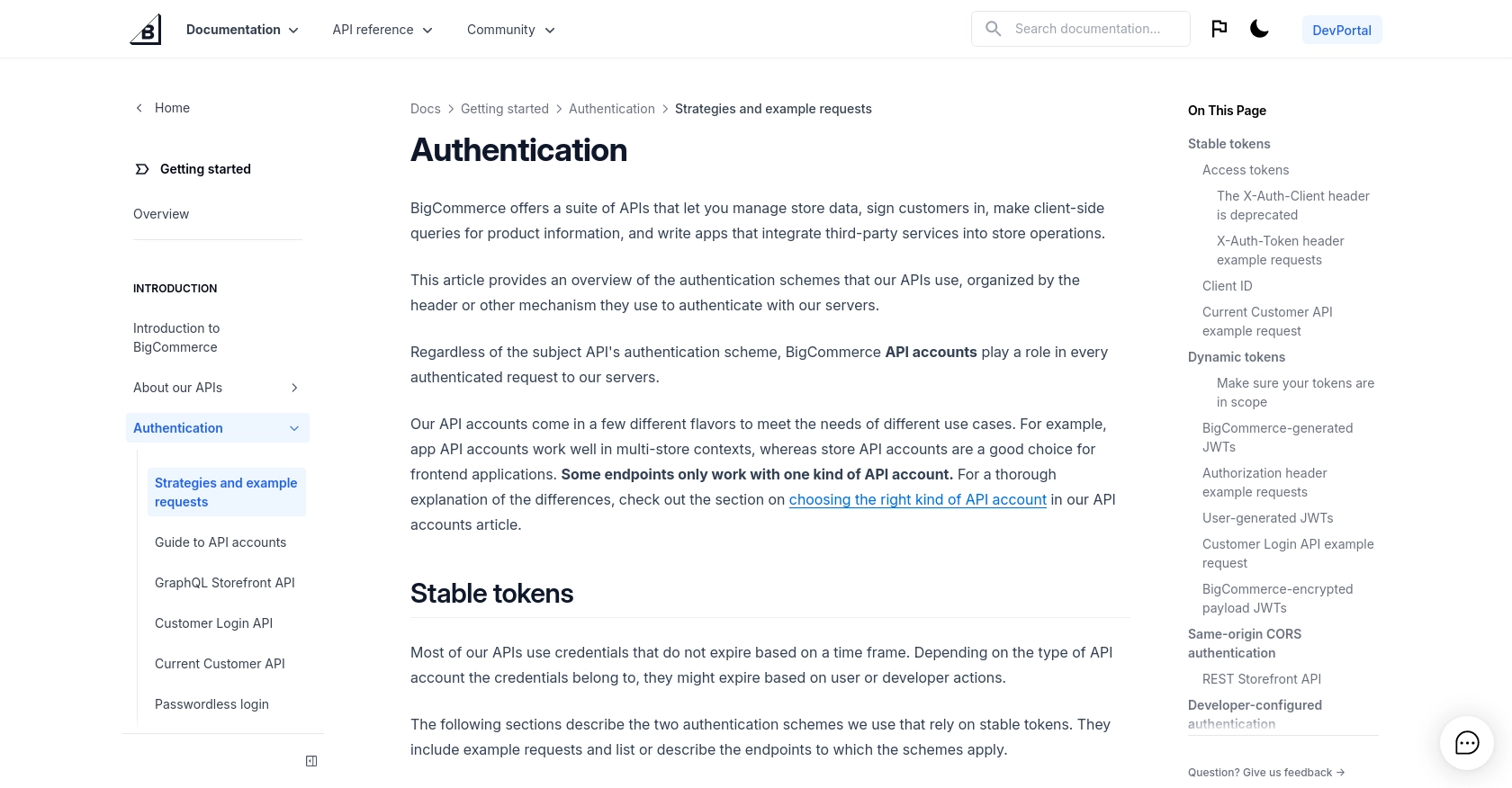
sbb-itb-96038d7
Making API Calls to Retrieve Orders from BigCommerce Using JavaScript
To effectively interact with the BigCommerce API and retrieve order data using JavaScript, you'll need to ensure your development environment is properly set up. This section will guide you through the necessary steps, including setting up JavaScript, installing dependencies, and executing API calls.
Setting Up Your JavaScript Environment for BigCommerce API Integration
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js or a browser-based environment. For this tutorial, we'll focus on using Node.js.
- Ensure you have Node.js installed. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
node-fetch
package to make HTTP requests by runningnpm install node-fetch
.
Executing a GET Request to Fetch Orders from BigCommerce
With your environment ready, you can now write a JavaScript function to fetch orders from BigCommerce. Use the following code as a template:
const fetch = require('node-fetch');
const fetchOrders = async () => {
const storeHash = 'your_store_hash';
const accessToken = 'your_access_token';
const url = `https://api.bigcommerce.com/stores/${storeHash}/v2/orders`;
try {
const response = await fetch(url, {
method: 'GET',
headers: {
'X-Auth-Token': accessToken,
'Accept': 'application/json'
}
});
if (!response.ok) {
throw new Error(`Error: ${response.status} ${response.statusText}`);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Failed to fetch orders:', error);
}
};
fetchOrders();
Replace your_store_hash
and your_access_token
with your actual store hash and access token. This code uses the node-fetch
library to send a GET request to the BigCommerce API, retrieving order data.
Handling API Response and Errors
After executing the API call, you should verify the response to ensure it succeeded. The code above checks the response status and throws an error if the request fails. This helps in identifying issues such as incorrect credentials or network problems.
Upon a successful request, the order data is logged to the console. You can further process this data as needed for your application.
Verifying API Call Success in BigCommerce Sandbox
To confirm that your API call is working as expected, you can cross-check the retrieved order data with the orders listed in your BigCommerce sandbox account. This ensures that the data you receive matches the actual data in your store.
Handling Common API Errors and Status Codes
When working with the BigCommerce API, you may encounter various error codes. Here are some common ones:
- 401 Unauthorized: Indicates invalid or missing authentication credentials. Ensure your access token is correct.
- 404 Not Found: The requested resource could not be found. Verify the endpoint URL and store hash.
- 500 Internal Server Error: A server-side error occurred. Try the request again later.
For more detailed information on error handling, refer to the BigCommerce API documentation.
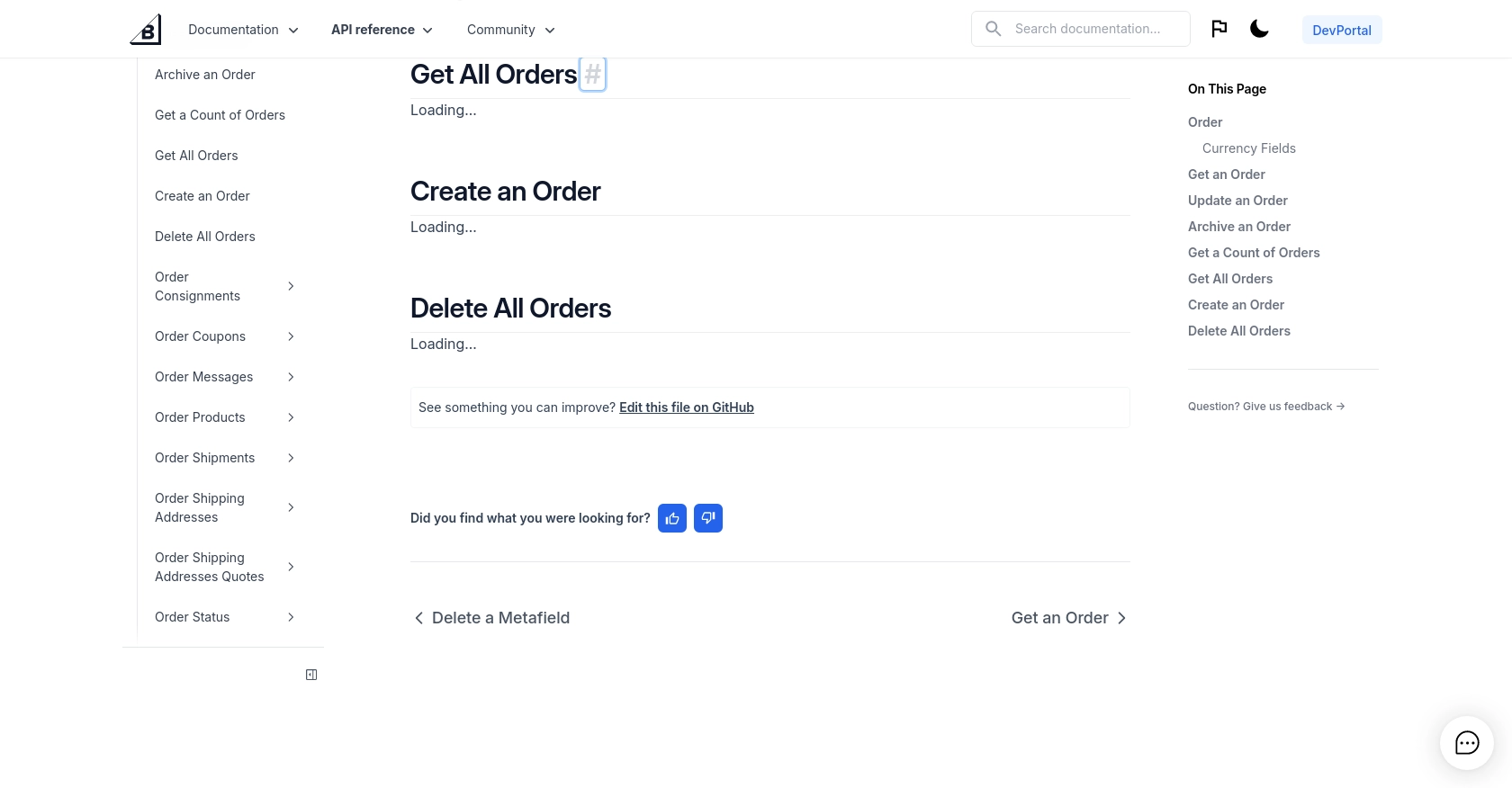
Conclusion and Best Practices for BigCommerce API Integration
Integrating with the BigCommerce API using JavaScript provides a powerful way to enhance your e-commerce operations. By automating order retrieval and processing, you can streamline workflows, improve data accuracy, and enhance customer satisfaction.
Best Practices for Secure and Efficient BigCommerce API Usage
- Securely Store API Credentials: Ensure that your API credentials, such as the access token, are stored securely and not exposed in your codebase or version control systems.
- Handle Rate Limiting: Be aware of BigCommerce's API rate limits to avoid exceeding the allowed number of requests. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Optimize Data Processing: Efficiently process and store the retrieved order data to minimize latency and improve application performance.
- Regularly Update OAuth Scopes: Periodically review and update your OAuth scopes to ensure they align with your application's needs and adhere to the principle of least privilege.
Enhance Your E-commerce Capabilities with Endgrate
While integrating with the BigCommerce API can significantly enhance your e-commerce capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including BigCommerce.
By leveraging Endgrate, you can focus on your core product development while outsourcing integration tasks. This approach allows you to build once for each use case, providing an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts and save valuable time and resources by visiting Endgrate's website.
Read More
Ready to get started?