How to Get Leads with the Close API in Javascript
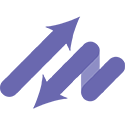
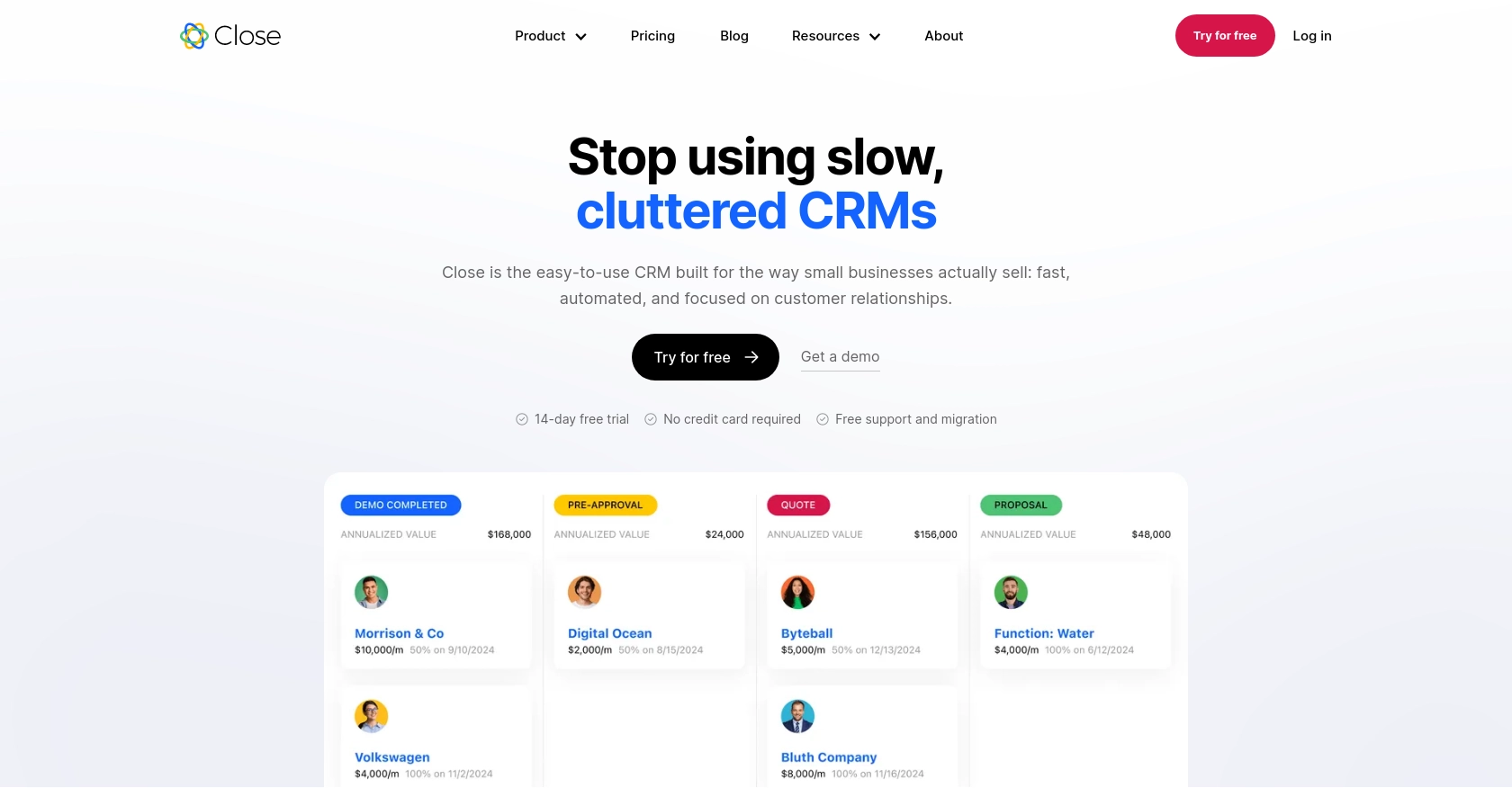
Introduction to Close CRM and API Integration
Close is a powerful CRM platform designed to help businesses streamline their sales processes and improve customer relationship management. It offers a comprehensive suite of tools for managing leads, contacts, and sales activities, making it a popular choice for sales teams looking to enhance their productivity and efficiency.
Integrating with Close's API allows developers to automate and optimize various sales operations. For example, by using the Close API, developers can programmatically retrieve leads, enabling seamless integration with other business systems and enhancing data-driven decision-making.
This article will guide you through the process of using JavaScript to interact with the Close API, specifically focusing on retrieving leads. By the end of this tutorial, you'll be equipped with the knowledge to efficiently access and manage lead data within the Close platform.
Setting Up Your Close CRM Account for API Access
Before you can start retrieving leads using the Close API, you need to set up your Close CRM account and obtain the necessary API key for authentication. This section will guide you through the steps to create a Close account and generate an API key.
Step 1: Create a Close CRM Account
If you don't already have a Close account, you can sign up for a free trial or use the free version by visiting the Close website. Follow the instructions to create your account and log in to the Close dashboard.
Step 2: Generate an API Key for Close API Authentication
Close uses API keys for authentication, which are ideal for internal scripts and integrations. Follow these steps to generate your API key:
- Log in to your Close account and navigate to the Settings page.
- Under the API Keys section, click on Create API Key.
- Provide a name for your API key to help you identify it later.
- Click Generate to create the API key.
- Copy the generated API key and store it securely, as you will need it to authenticate your API requests.
For more detailed information on API key authentication, refer to the Close API Authentication Documentation.
Step 3: Prepare Your Development Environment
Ensure you have a suitable development environment set up for JavaScript. You can use Node.js or any other JavaScript runtime that suits your needs. Make sure to have a code editor and terminal ready for executing your scripts.
With your Close account and API key ready, you're now set to start making API calls to retrieve leads using JavaScript. In the next section, we'll walk through the process of making these API calls.
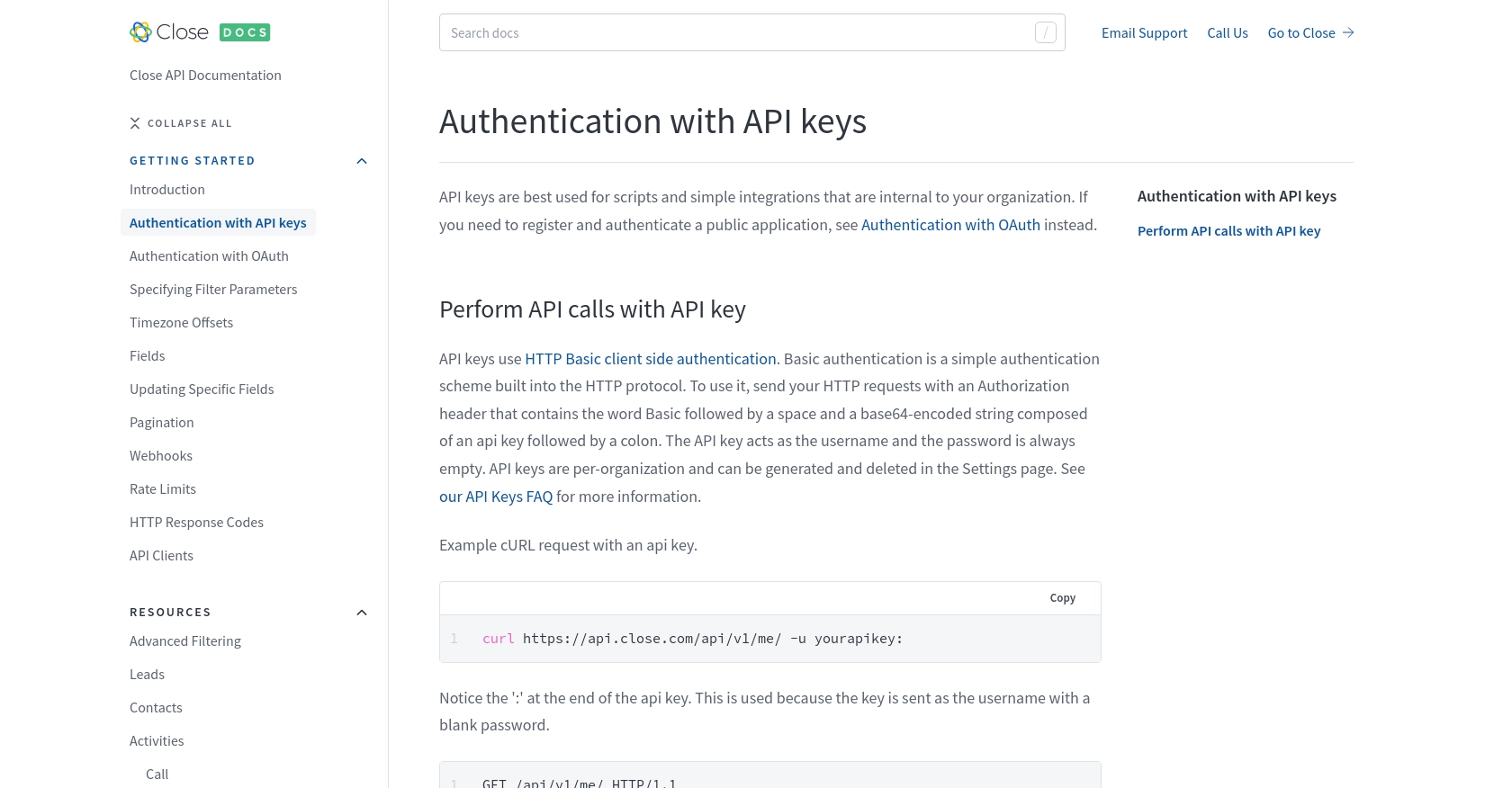
sbb-itb-96038d7
How to Make API Calls to Retrieve Leads from Close Using JavaScript
To interact with the Close API and retrieve leads, you'll need to set up your JavaScript environment and make HTTP requests to the API endpoints. This section will guide you through the necessary steps, including setting up your environment, writing the code to make API calls, and handling responses and errors.
Setting Up Your JavaScript Environment for Close API Integration
Ensure you have Node.js installed on your machine, as it provides a runtime environment for executing JavaScript code outside the browser. You can download and install Node.js from the official website.
Once Node.js is installed, you can use npm (Node Package Manager) to install the required dependencies. For this tutorial, we'll use the axios
library to make HTTP requests. Run the following command in your terminal:
npm install axios
Writing JavaScript Code to Retrieve Leads from Close API
Create a new JavaScript file named getCloseLeads.js
and add the following code:
const axios = require('axios');
// Your Close API key
const apiKey = 'your_api_key_here';
// Set the API endpoint
const endpoint = 'https://api.close.com/api/v1/lead/';
// Configure the request headers
const headers = {
'Authorization': `Basic ${Buffer.from(apiKey + ':').toString('base64')}`
};
// Function to retrieve leads
async function getLeads() {
try {
const response = await axios.get(endpoint, { headers });
const leads = response.data.data;
console.log('Leads retrieved successfully:', leads);
} catch (error) {
console.error('Error retrieving leads:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getLeads();
Replace your_api_key_here
with the API key you generated earlier. This script uses the axios
library to send a GET request to the Close API's lead endpoint. The response data is logged to the console, displaying the retrieved leads.
Understanding the Response and Handling Errors
Upon executing the script, you should see the list of leads printed in the console. The Close API returns data in a structured format, which includes lead details such as name, status, and custom fields.
It's essential to handle potential errors that may occur during API requests. Common HTTP response codes include:
- 200: Request was successful.
- 400: Bad request, check your query parameters.
- 401: Unauthorized, verify your API key.
- 429: Too many requests, respect rate limits.
For more details on HTTP response codes, refer to the Close API HTTP Response Codes Documentation.
Verifying Successful API Requests in Close CRM
After running your script, you can verify the successful retrieval of leads by checking the Close CRM dashboard. The leads displayed in your console should match those in your Close account.
For further information on making API calls and handling responses, visit the Close API Leads Documentation.
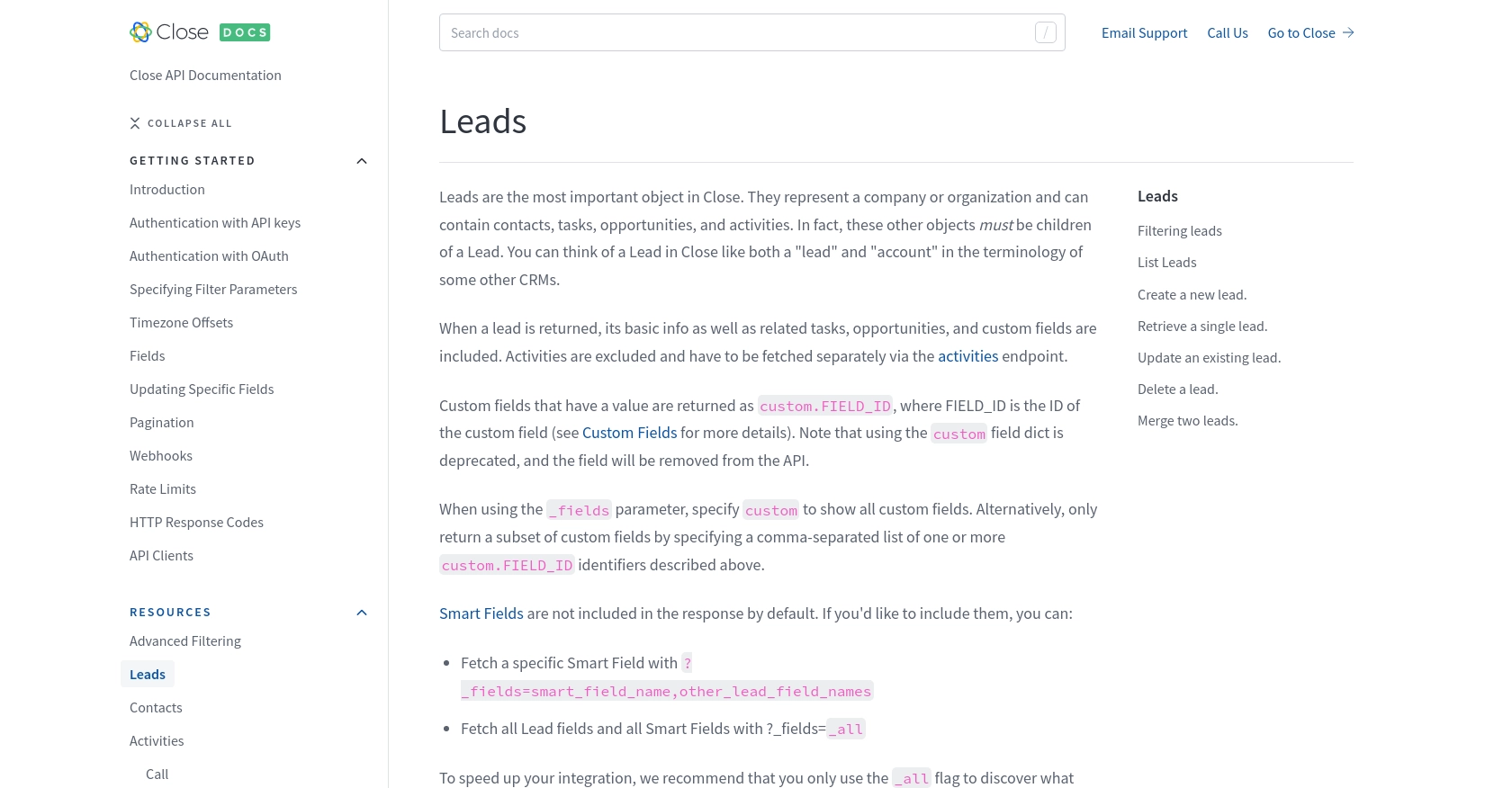
Conclusion and Best Practices for Using Close API with JavaScript
Integrating with the Close API using JavaScript can significantly enhance your sales operations by automating lead retrieval and management. By following the steps outlined in this tutorial, you can efficiently access lead data and integrate it with other business systems to drive data-driven decision-making.
Best Practices for Secure and Efficient Close API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Close API's rate limits. If you encounter a 429 status code, pause your requests as specified by the
rate_reset
value. For more details, refer to the Close API Rate Limits Documentation. - Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your existing systems.
- Error Handling: Implement robust error handling to manage different HTTP response codes effectively. This ensures your application can gracefully handle issues like unauthorized access or bad requests.
Enhance Your Integration Experience with Endgrate
While integrating with Close API directly is powerful, using a tool like Endgrate can further streamline your integration process. Endgrate offers a unified API endpoint that connects to multiple platforms, including Close, allowing you to build once and deploy across various integrations. This saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/close
- https://developer.close.com/topics/authentication/
- https://developer.close.com/topics/authentication-oauth2/
- https://developer.close.com/topics/pagination/
- https://developer.close.com/topics/rate-limits/
- https://developer.close.com/topics/http-response-codes/
- https://developer.close.com/resources/leads/
Ready to get started?