Using the FreshDesk API to Create or Update Contacts (with Javascript examples)
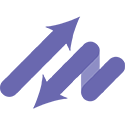
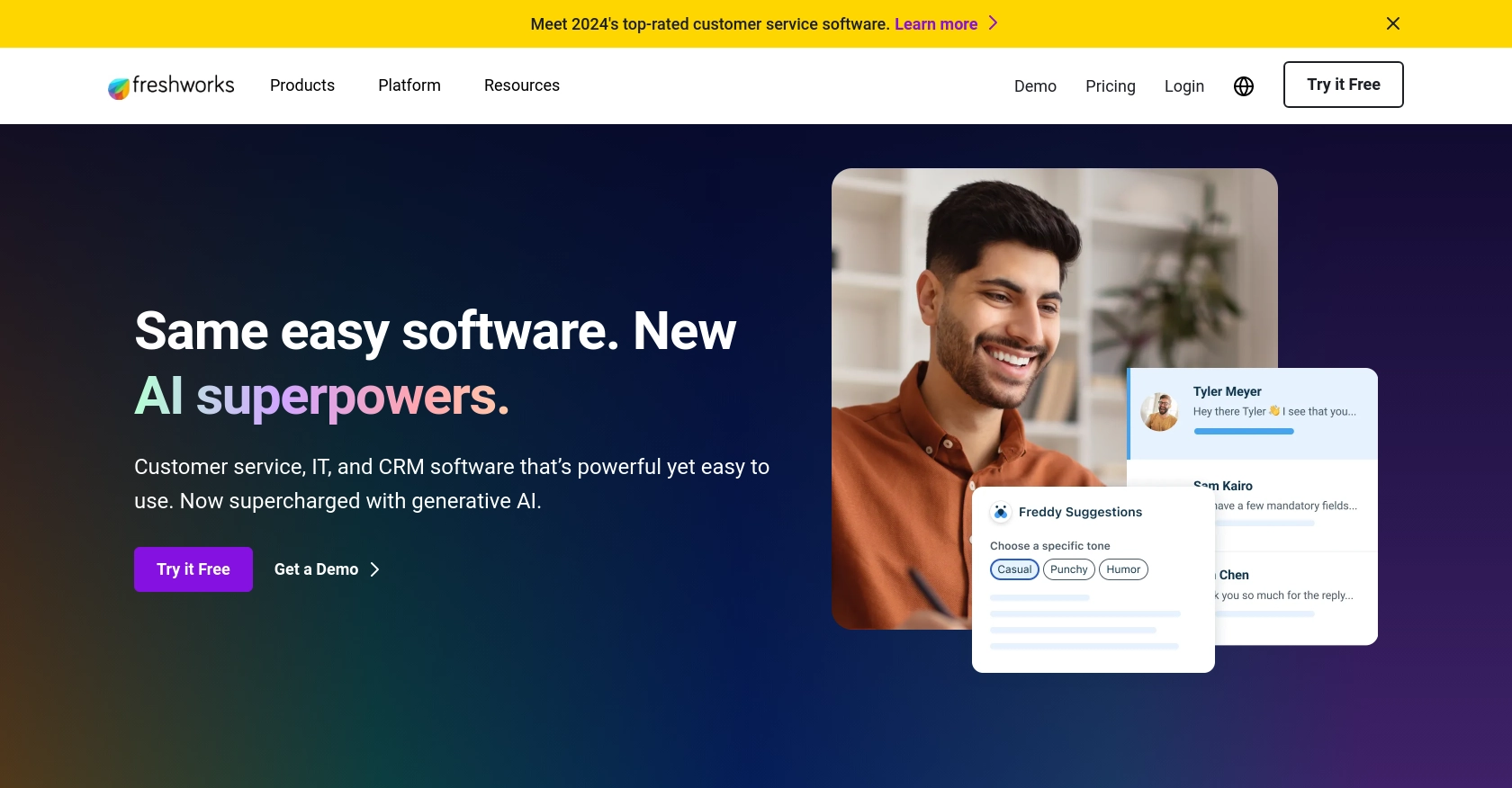
Introduction to FreshDesk API Integration
FreshDesk is a powerful customer support software that enables businesses to manage customer interactions efficiently. It offers a comprehensive suite of tools for ticketing, collaboration, and automation, making it a popular choice for companies looking to enhance their customer service operations.
Developers may want to integrate with FreshDesk's API to streamline customer data management, such as creating or updating contact information. For example, a developer could use the FreshDesk API to automatically update contact details when a customer submits a new support ticket, ensuring that the support team always has the most current information.
Setting Up Your FreshDesk Sandbox Account for API Integration
Before diving into the FreshDesk API, it's essential to set up a sandbox account. This allows developers to test API interactions without affecting live data. FreshDesk offers a free trial that can serve as a sandbox environment for development and testing purposes.
Creating a FreshDesk Free Trial Account
To begin, visit the FreshDesk signup page and register for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is created, you'll have access to the FreshDesk dashboard, where you can manage your sandbox environment.
Generating API Key for FreshDesk Authentication
FreshDesk uses a custom authentication method that involves an API key. To generate your API key, follow these steps:
- Log in to your FreshDesk account.
- Navigate to your profile icon in the top right corner and select Profile Settings.
- In the Profile Settings page, you'll find your API key under the API Key section.
- Copy the API key and store it securely, as you'll need it to authenticate your API requests.
Configuring FreshDesk App for API Access
To interact with the FreshDesk API, you may need to configure an app within your FreshDesk account:
- Go to the Admin section from the sidebar.
- Select Apps and click on Create New App.
- Fill in the necessary details and configure the app permissions to allow access to contacts.
- Save the app configuration to finalize the setup.
With your sandbox account and API key ready, you can now proceed to make API calls to create or update contacts using JavaScript. This setup ensures a secure and isolated environment for testing your integration with FreshDesk.
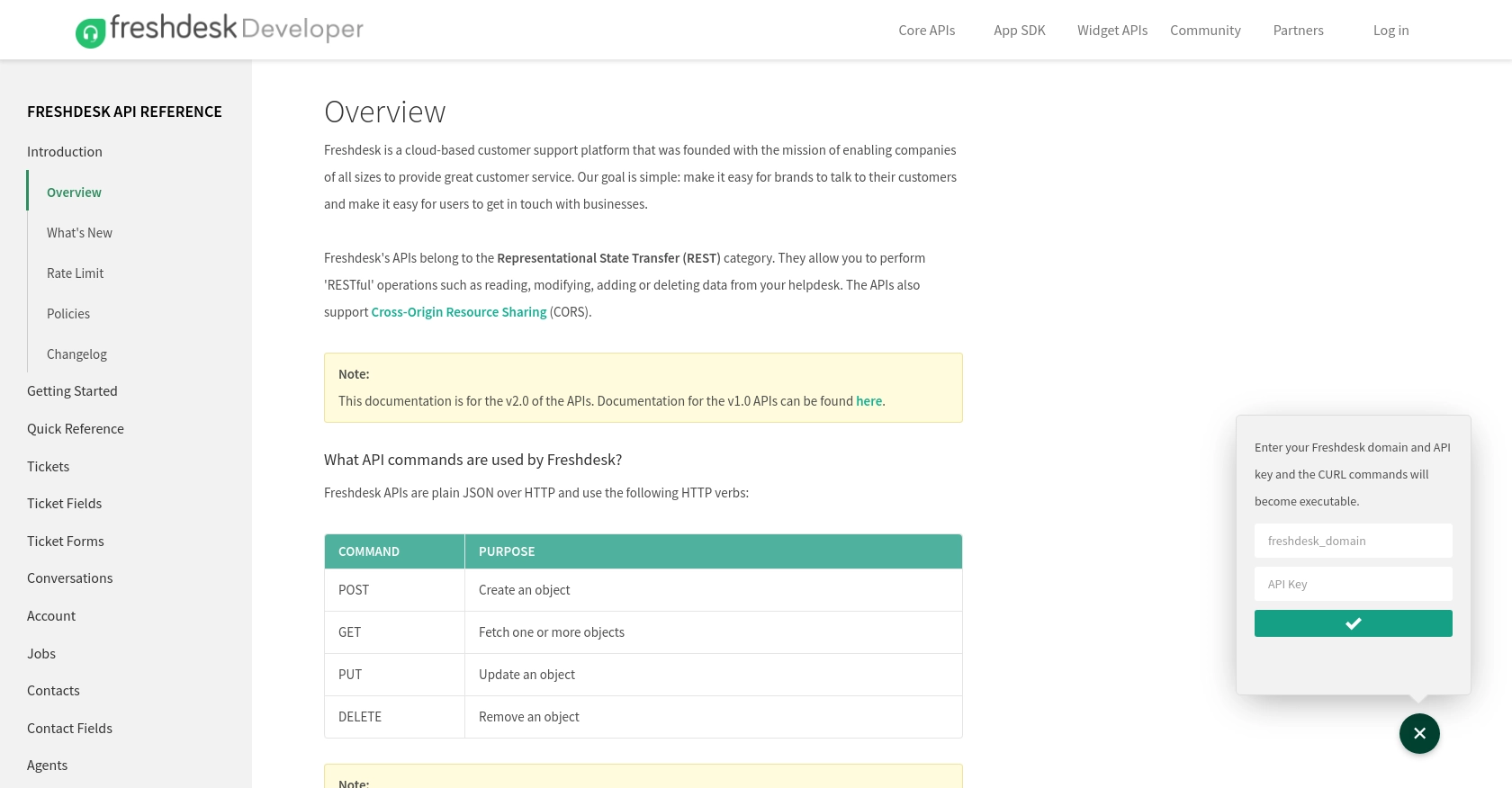
sbb-itb-96038d7
Making API Calls to FreshDesk for Creating or Updating Contacts Using JavaScript
To interact with the FreshDesk API for creating or updating contacts, you'll need to use JavaScript to send HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for FreshDesk API Integration
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js or a browser-based environment. For this tutorial, we'll use Node.js. Make sure you have Node.js installed on your machine.
You'll also need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Create or Update Contacts in FreshDesk
Now, let's write the JavaScript code to interact with the FreshDesk API. We'll create a function to either create a new contact or update an existing one.
const axios = require('axios');
// Set your FreshDesk domain and API key
const freshdeskDomain = 'yourdomain.freshdesk.com';
const apiKey = 'Your_API_Key';
// Function to create or update a contact
async function createOrUpdateContact(contactData) {
const url = `https://${freshdeskDomain}/api/v2/contacts`;
try {
const response = await axios({
method: 'post',
url: url,
auth: {
username: apiKey,
password: 'X' // FreshDesk requires 'X' as the password
},
headers: {
'Content-Type': 'application/json'
},
data: contactData
});
console.log('Contact created or updated successfully:', response.data);
} catch (error) {
console.error('Error creating or updating contact:', error.response.data);
}
}
// Example contact data
const contactData = {
name: 'John Doe',
email: 'john.doe@example.com',
phone: '1234567890'
};
// Call the function
createOrUpdateContact(contactData);
Replace yourdomain.freshdesk.com
and Your_API_Key
with your actual FreshDesk domain and API key.
Verifying API Call Success and Handling Errors
After running the script, verify the success of the API call by checking the response data. If the contact is created or updated successfully, you'll see the contact details in the response.
In case of errors, the script will log the error details. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed. Check your API key.
- 404 Not Found: The requested resource could not be found.
Refer to the FreshDesk API documentation for more details on error codes and handling.
Conclusion and Best Practices for FreshDesk API Integration
Integrating with the FreshDesk API using JavaScript can significantly enhance your customer support operations by automating contact management. By following the steps outlined in this guide, you can efficiently create or update contacts, ensuring your support team always has access to the latest customer information.
Best Practices for Secure and Efficient FreshDesk API Usage
- Secure API Key Storage: Always store your FreshDesk API key securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of FreshDesk's rate limits to avoid request throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure consistent data formats when creating or updating contacts to maintain data integrity across your systems.
- Error Handling: Implement robust error handling to manage API call failures and log errors for troubleshooting.
Streamline Your Integrations with Endgrate
While integrating with FreshDesk is a powerful way to enhance your customer support, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including FreshDesk.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API endpoint, you can manage multiple integrations efficiently, providing a seamless experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?