How to Create or Update Contacts with the Hubspot API in Javascript
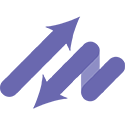
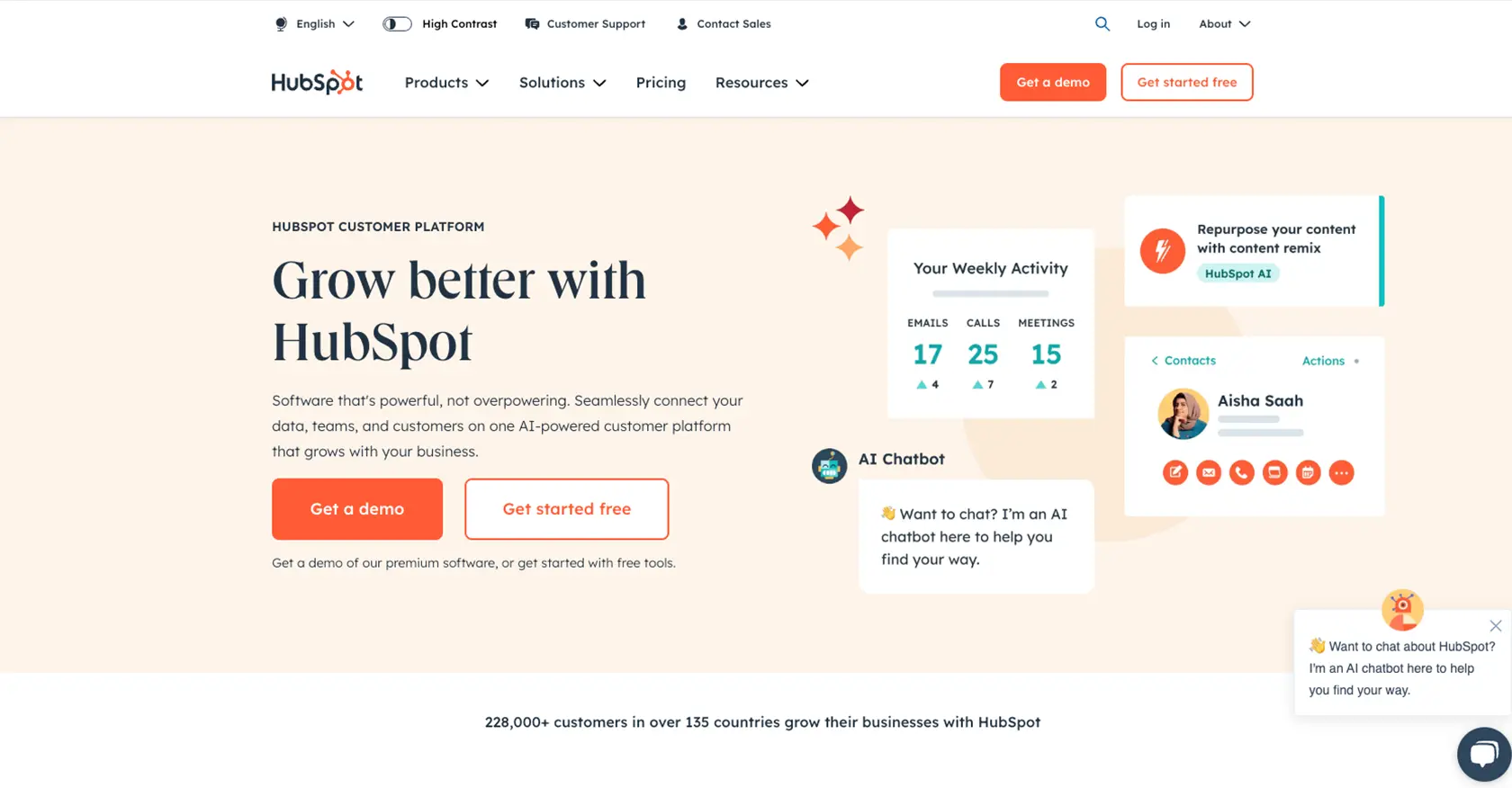
Introduction to HubSpot API Integration
HubSpot is a comprehensive CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for businesses looking to streamline their operations and enhance customer engagement.
Developers often seek to integrate with HubSpot's API to automate and manage customer data efficiently. By connecting with HubSpot's API, developers can create or update contact information seamlessly, enabling personalized marketing strategies and improved customer relationship management. For example, a developer might use the HubSpot API to automatically update contact details from a web form submission, ensuring that the CRM always contains the most current information.
Setting Up Your HubSpot Test/Sandbox Account for API Integration
Before you can start creating or updating contacts using the HubSpot API in JavaScript, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, you'll need to create one. Follow these steps to get started:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill out the necessary information.
- Once your account is set up, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Environment
HubSpot provides a sandbox environment for testing integrations. Here's how to set it up:
- In your HubSpot developer account, navigate to the "Test Accounts" section.
- Create a new test account by following the on-screen instructions.
- Once the test account is created, you can use it to test API calls without affecting your live data.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for authentication, which allows secure access to the API. Follow these steps to configure OAuth:
- In your developer account, go to the "Apps" section and create a new app.
- Provide the necessary details, such as the app name and description.
- Under the "Auth" tab, configure the OAuth settings. You'll need to specify the redirect URL and select the necessary scopes for accessing contact data.
- Save the app settings to generate your client ID and client secret.
For more details on OAuth setup, refer to the HubSpot OAuth Documentation.
Generating an OAuth Access Token
With your app configured, you can now generate an OAuth access token:
- Direct users to the authorization URL to obtain their consent for your app to access their HubSpot data.
- Once consent is granted, you'll receive an authorization code.
- Exchange this authorization code for an access token by making a POST request to the HubSpot token endpoint.
// Example code to exchange authorization code for access token
const axios = require('axios');
async function getAccessToken(authCode) {
const response = await axios.post('https://api.hubapi.com/oauth/v1/token', {
grant_type: 'authorization_code',
client_id: 'YOUR_CLIENT_ID',
client_secret: 'YOUR_CLIENT_SECRET',
redirect_uri: 'YOUR_REDIRECT_URI',
code: authCode
});
return response.data.access_token;
}
Ensure you securely store the access token, as it will be used to authenticate your API requests.
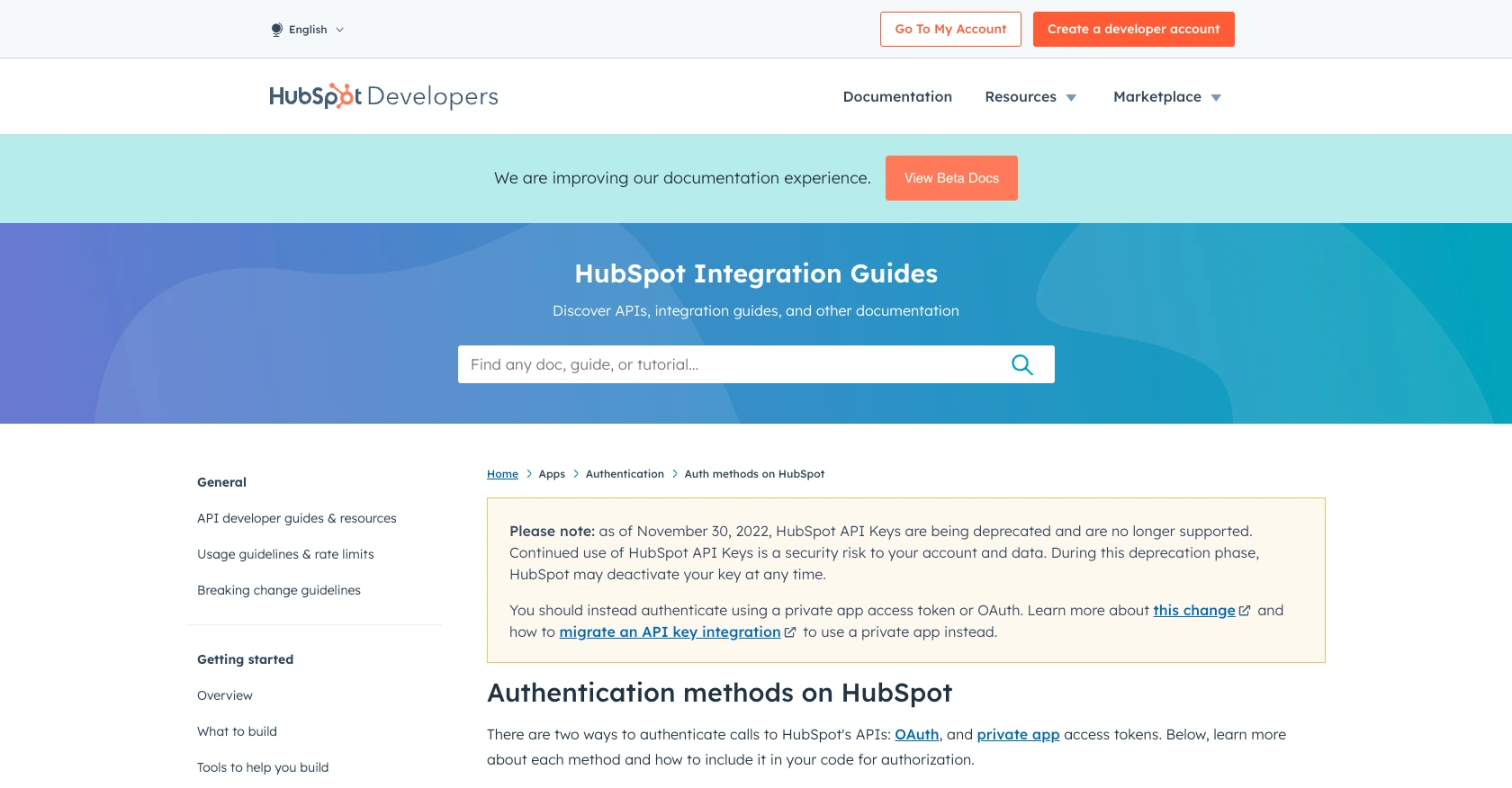
sbb-itb-96038d7
Making API Calls to Create or Update Contacts with HubSpot API in JavaScript
To interact with HubSpot's API using JavaScript, you'll need to ensure you have the right setup and dependencies. This section will guide you through making API calls to create or update contacts in HubSpot using JavaScript.
Setting Up Your JavaScript Environment for HubSpot API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside a browser. You'll also need the Axios library to handle HTTP requests.
- Install Node.js from the official website if you haven't already.
- Initialize a new Node.js project and install Axios by running the following commands in your terminal:
npm init -y
npm install axios
Creating Contacts with HubSpot API Using JavaScript
To create a new contact in HubSpot, you'll make a POST request to the HubSpot API endpoint for contacts. Here's how you can do it using JavaScript:
const axios = require('axios');
async function createContact() {
const url = 'https://api.hubapi.com/crm/v3/objects/contacts';
const token = 'YOUR_ACCESS_TOKEN';
const contactData = {
properties: {
email: 'example@hubspot.com',
firstname: 'John',
lastname: 'Doe'
}
};
try {
const response = await axios.post(url, contactData, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Contact Created:', response.data);
} catch (error) {
console.error('Error Creating Contact:', error.response.data);
}
}
createContact();
Replace YOUR_ACCESS_TOKEN
with the OAuth access token you generated earlier. This script sends a POST request to create a contact with the specified email, first name, and last name.
Updating Contacts with HubSpot API Using JavaScript
To update an existing contact, you'll make a PATCH request to the HubSpot API. Here's an example:
async function updateContact(contactId) {
const url = `https://api.hubapi.com/crm/v3/objects/contacts/${contactId}`;
const token = 'YOUR_ACCESS_TOKEN';
const updatedData = {
properties: {
firstname: 'Jane',
lastname: 'Smith'
}
};
try {
const response = await axios.patch(url, updatedData, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Contact Updated:', response.data);
} catch (error) {
console.error('Error Updating Contact:', error.response.data);
}
}
updateContact('CONTACT_ID');
Replace CONTACT_ID
with the ID of the contact you wish to update. This script updates the contact's first and last name.
Handling API Response and Errors
After making an API call, it's crucial to handle the response and any potential errors. Successful responses will contain the contact data, while errors might include status codes like 400 for bad requests or 401 for unauthorized access. Always check the response status and error messages to debug issues effectively.
Verifying API Requests in HubSpot Sandbox
Once you've made the API calls, verify the changes in your HubSpot sandbox account. Check the contacts section to ensure the new contact is created or the existing contact is updated as expected.
For more details on handling errors and rate limits, refer to the HubSpot API documentation.
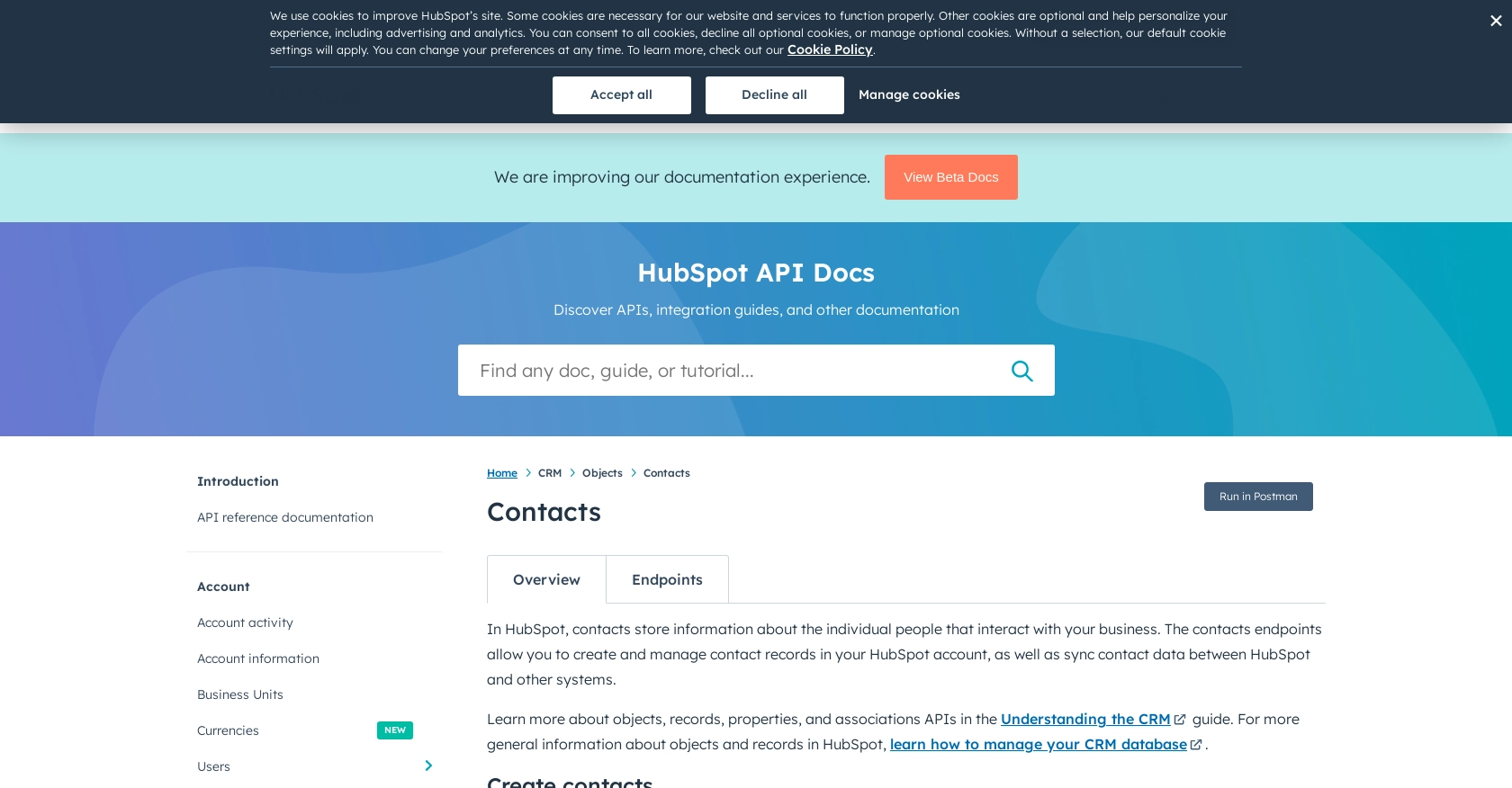
Conclusion and Best Practices for HubSpot API Integration in JavaScript
Integrating with the HubSpot API using JavaScript allows developers to efficiently manage contact data, enhancing customer relationship management and enabling personalized marketing strategies. By following the steps outlined in this guide, you can create and update contacts seamlessly within your HubSpot CRM.
Best Practices for Secure and Efficient HubSpot API Usage
- Securely Store Credentials: Always store your OAuth access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: HubSpot enforces rate limits to ensure fair usage. For OAuth apps, the limit is 100 requests every 10 seconds per account. Implement retry logic and exponential backoff to handle 429 rate limit errors gracefully. For more details, refer to the HubSpot API usage guidelines.
- Data Standardization: Ensure that data fields are standardized before sending them to HubSpot. This helps maintain data consistency and integrity across your CRM.
- Error Handling: Implement robust error handling to capture and log API errors. This will aid in debugging and maintaining a smooth integration experience.
Enhance Your Integration Experience with Endgrate
For developers looking to streamline their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that simplifies interactions with multiple platforms, including HubSpot, offering an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/contacts
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?