How to Get Users with the Airtable API in Python
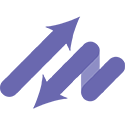
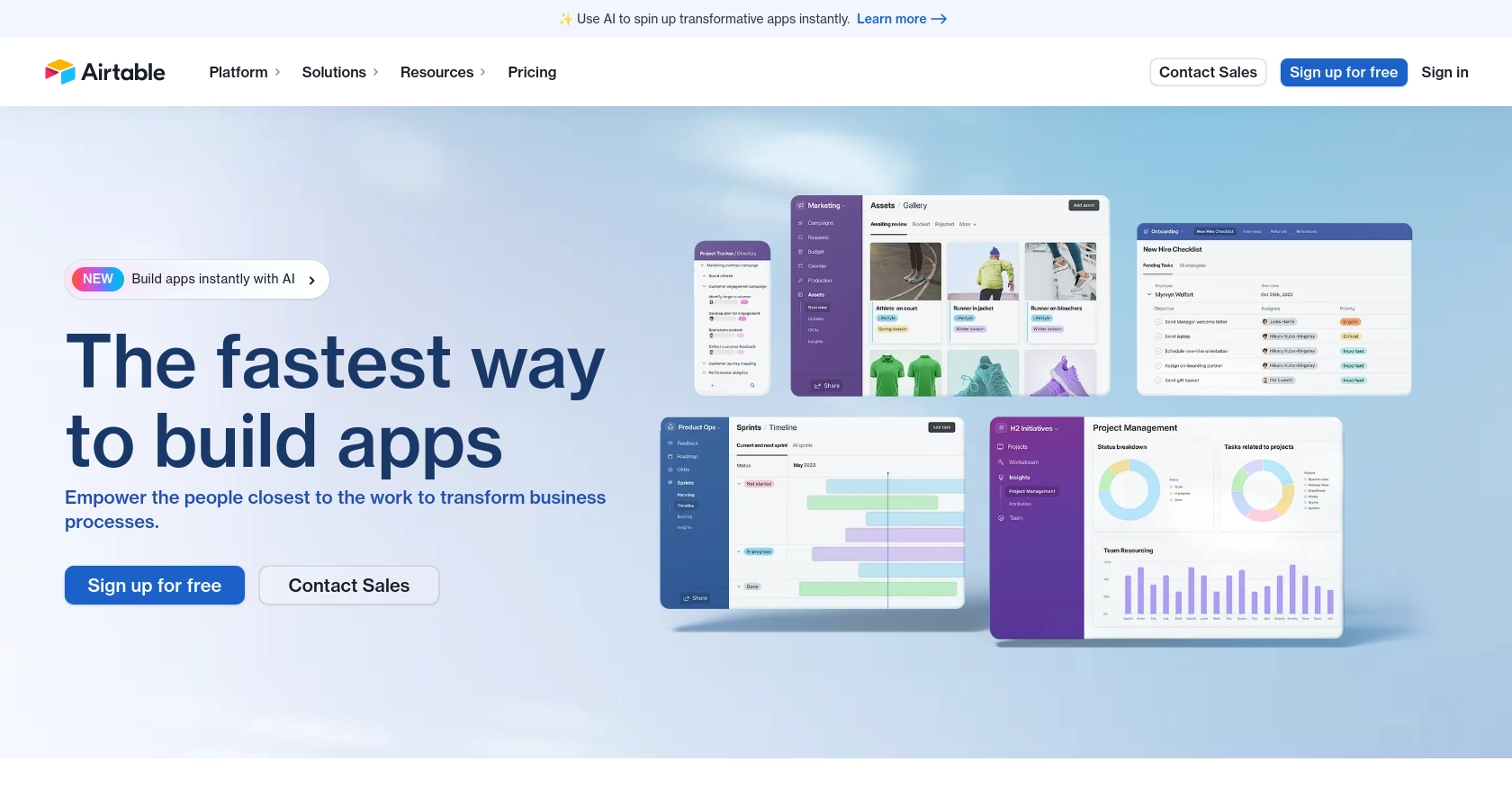
Introduction to Airtable API Integration
Airtable is a versatile platform that combines the simplicity of a spreadsheet with the power of a database, allowing businesses to organize and manage their data efficiently. With its intuitive interface and robust API, Airtable is a popular choice for developers looking to build custom applications and integrations.
Integrating with the Airtable API enables developers to access and manipulate data programmatically, making it easier to automate workflows and enhance productivity. For example, a developer might want to retrieve user information from Airtable to synchronize it with another system, ensuring data consistency across platforms.
This article will guide you through the process of using Python to interact with the Airtable API, specifically focusing on how to get user information. By following this tutorial, you'll learn how to set up authentication, make API calls, and handle responses effectively.
Setting Up Your Airtable Test Account for API Integration
Before you can start interacting with the Airtable API using Python, you'll need to set up a test account and configure the necessary authentication credentials. This process involves creating an Airtable account, setting up an OAuth app, and obtaining the required access tokens.
Creating an Airtable Account
If you don't already have an Airtable account, you can sign up for a free account on the Airtable website. Follow the instructions to complete the registration process. Once your account is created, you'll be able to log in and access the Airtable dashboard.
Registering an OAuth App in Airtable
To interact with the Airtable API, you'll need to create an OAuth app. This app will allow you to request access tokens that are necessary for authenticating API requests.
- Navigate to the Developer Hub on Airtable's website.
- Click on Create an OAuth App.
- Fill in the required details, including the app name, description, and redirect URI. Ensure that the redirect URI is HTTPS compliant.
- Submit the form to create your app. You will receive a client ID and client secret.
Generating OAuth Access Tokens
With your OAuth app set up, you can now generate access tokens to authenticate your API requests. Follow these steps:
- Use the client ID and client secret to initiate the OAuth flow. This involves directing users to Airtable's authorization URL.
- Once users authorize your app, they will be redirected to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to Airtable's token endpoint.
For detailed instructions on the OAuth flow, refer to the Airtable OAuth documentation.
Configuring API Scopes and Permissions
Ensure that your OAuth app has the necessary scopes to access user information. You can configure these scopes during the app registration process or by editing your app settings later. For retrieving user data, you will need the enterprise.user:read
scope.
Once you have your access token, you are ready to make authenticated requests to the Airtable API. Keep your access token secure and avoid sharing it with unauthorized parties.
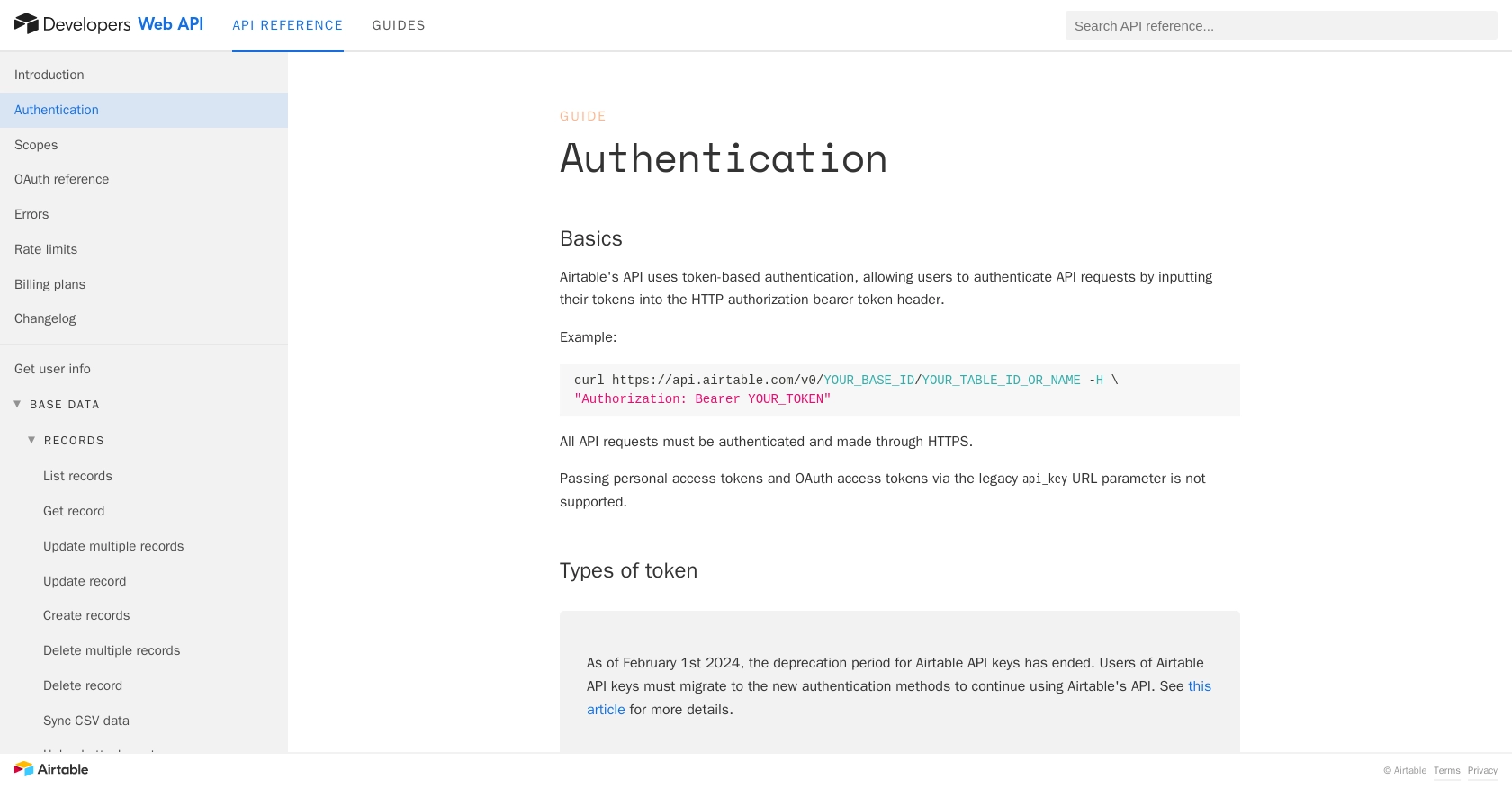
sbb-itb-96038d7
Making API Calls to Retrieve Users with Airtable API in Python
To interact with the Airtable API using Python, you'll need to ensure you have the right environment set up. This section will guide you through the necessary steps to make API calls to retrieve user information from Airtable.
Setting Up Your Python Environment for Airtable API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Verify your Python installation by running
python --version
in your terminal. - Install the
requests
library using pip:
pip install requests
Writing Python Code to Get Users from Airtable API
With your environment ready, you can now write the Python code to interact with the Airtable API. Create a new Python file named get_airtable_users.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.airtable.com/v0/meta/enterpriseAccounts/{enterpriseAccountId}/users"
headers = {"Authorization": "Bearer YOUR_ACCESS_TOKEN"}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
users = response.json()["users"]
for user in users:
print(f"User ID: {user['id']}, Email: {user['email']}, Name: {user['name']}")
else:
print(f"Failed to retrieve users: {response.status_code} - {response.json()['error']['message']}")
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup. Also, ensure you replace {enterpriseAccountId}
with your actual enterprise account ID.
Understanding the API Response and Handling Errors
The above code sends a GET request to the Airtable API to retrieve user information. If successful, it prints out the user ID, email, and name. If the request fails, it will print an error message.
Common error codes you might encounter include:
- 401 Unauthorized: Invalid or missing access token.
- 403 Forbidden: Insufficient permissions to access the resource.
- 404 Not Found: Incorrect endpoint or resource does not exist.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
For more details on error handling, refer to the Airtable API error documentation.
Verifying Successful API Requests in Airtable
To verify that your API requests are successful, you can cross-check the returned data with the user information available in your Airtable account. Ensure that the users listed in the response match those in your Airtable dashboard.
By following these steps, you can effectively retrieve user information from Airtable using Python, allowing you to integrate and synchronize data across platforms seamlessly.
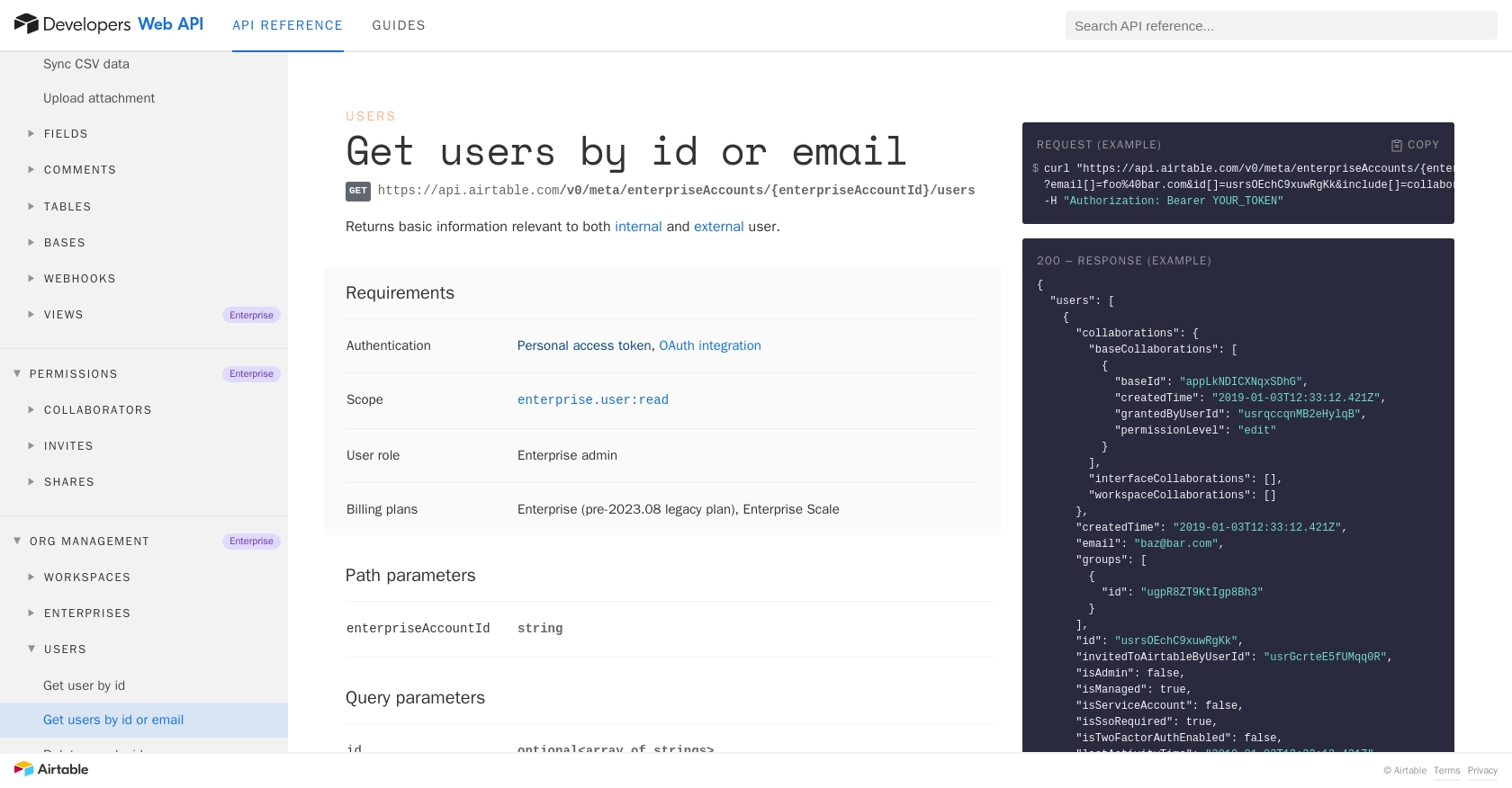
Conclusion and Best Practices for Using Airtable API in Python
Integrating with the Airtable API using Python provides a powerful way to automate data management and enhance productivity across platforms. By following the steps outlined in this guide, you can efficiently retrieve user information and ensure seamless data synchronization.
Best Practices for Secure and Efficient Airtable API Integration
- Secure Token Storage: Always store your OAuth access tokens securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limits: Airtable imposes a rate limit of 5 requests per second per base. Implement back-off strategies and retry logic to handle 429 status codes gracefully. For more details, refer to the Airtable API rate limits documentation.
- Data Transformation: Consider transforming and standardizing data fields to match the requirements of your application or other integrated systems.
Enhancing Integration Capabilities with Endgrate
For developers looking to streamline their integration processes, Endgrate offers a unified API solution that simplifies connecting with multiple platforms, including Airtable. By leveraging Endgrate, you can focus on your core product while outsourcing complex integrations, saving time and resources.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website and discover how companies like yours have scaled their integrations effortlessly.
Read More
- https://endgrate.com/provider/airtable
- https://airtable.com/developers/web/api/authentication
- https://airtable.com/developers/web/api/scopes
- https://airtable.com/developers/web/api/oauth-reference
- https://airtable.com/developers/web/api/errors
- https://airtable.com/developers/web/api/rate-limits
- https://airtable.com/developers/web/api/get-users-by-id-or-email
Ready to get started?