Using the Brevo API to Get Contacts in Python
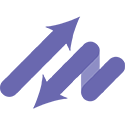
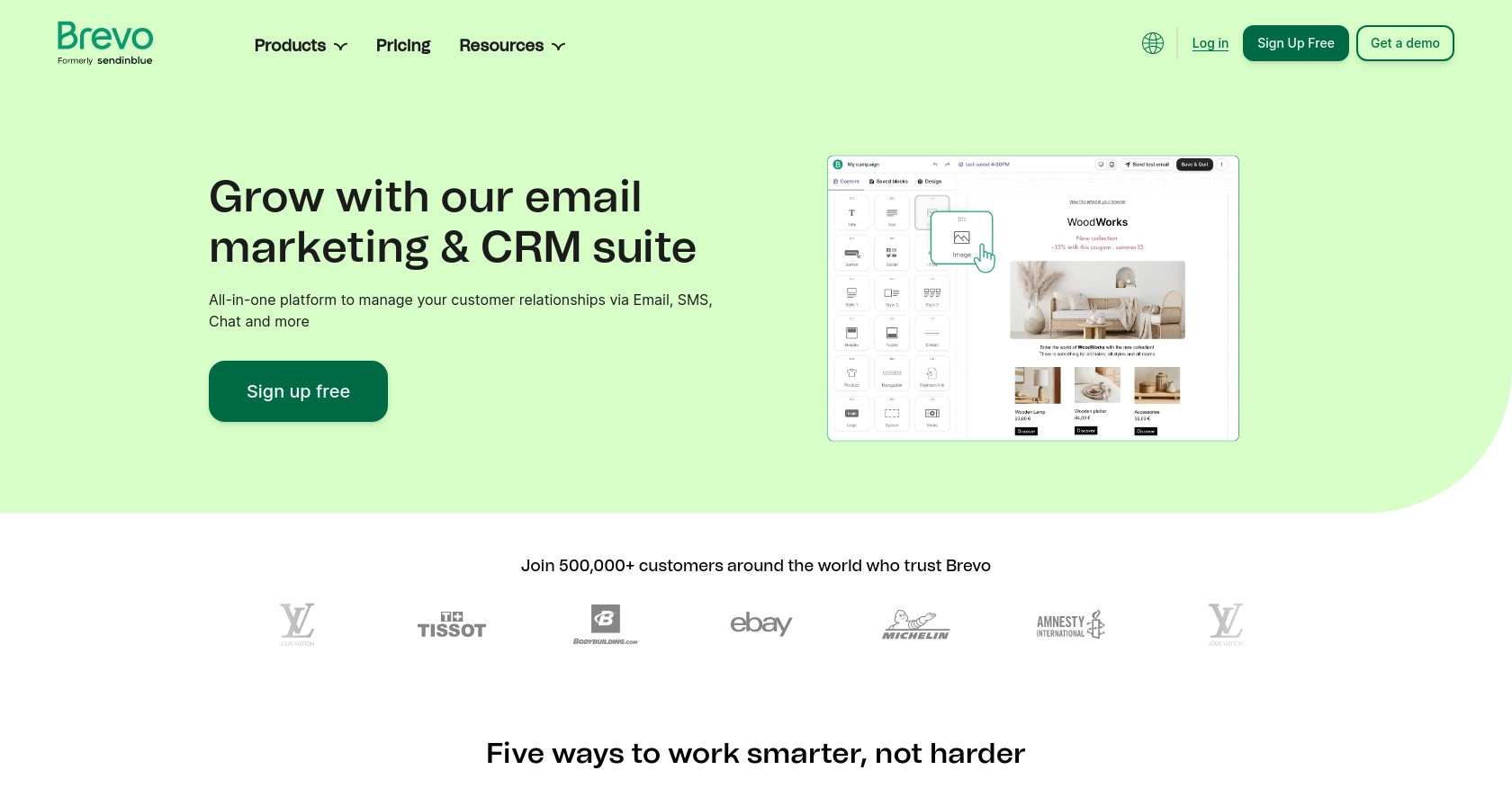
Introduction to Brevo API Integration
Brevo is a versatile platform offering a range of communication tools, including email marketing, SMS campaigns, and more. It empowers businesses to effectively manage their customer interactions and marketing efforts through a unified interface.
Developers might want to integrate with Brevo's API to streamline contact management and enhance marketing automation. For example, using the Brevo API, a developer can retrieve contact information to personalize marketing campaigns or synchronize contact data with other systems, ensuring seamless communication across platforms.
Setting Up Your Brevo API Test Account
Before you can start integrating with the Brevo API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Create a Brevo Account
If you don't already have a Brevo account, you can sign up for a free account on the Brevo signup page. Follow the instructions to complete your registration.
Generate Your Brevo API Key
Once your account is set up, you'll need to generate an API key to authenticate your requests. Follow these steps:
- Log in to your Brevo account.
- Click on your name at the top-right corner of the screen and select SMTP & API.
- Navigate to the API keys tab and click Generate a new API key.
- Name your API key for easy identification and click Generate.
- Copy the generated API key and store it securely, as you will need it for API requests.
For more detailed instructions, refer to the Brevo API documentation.
Understanding Brevo API Key Authentication
Brevo uses API key authentication to verify the identity of the user making the request. This key must be included in the header of each API call to ensure secure communication. Make sure to keep your API key confidential to prevent unauthorized access.
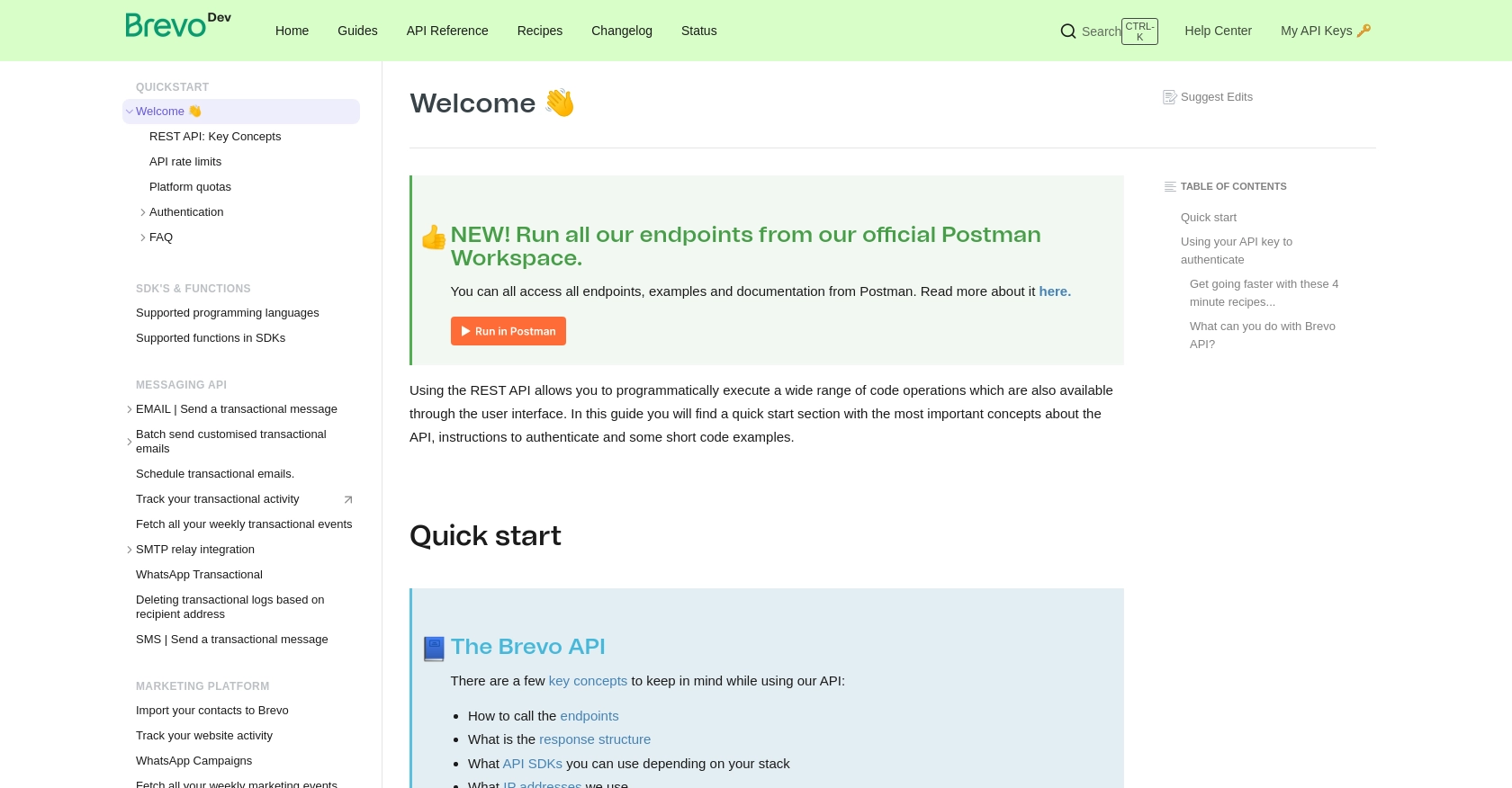
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Brevo Using Python
To interact with the Brevo API and retrieve contact information, you'll need to use Python. This section will guide you through setting up your environment, making the API call, and handling the response.
Setting Up Your Python Environment for Brevo API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the sib_api_v3_sdk
library to interact with the Brevo API. Install it using pip:
pip install sib-api-v3-sdk
Writing Python Code to Fetch Contacts from Brevo
Create a new Python file named get_brevo_contacts.py
and add the following code:
from __future__ import print_function
import sib_api_v3_sdk
from sib_api_v3_sdk.rest import ApiException
from pprint import pprint
# Configure API key authorization
configuration = sib_api_v3_sdk.Configuration()
configuration.api_key['api-key'] = 'YOUR_API_KEY'
# Create an instance of the API class
api_instance = sib_api_v3_sdk.ContactsApi(sib_api_v3_sdk.ApiClient(configuration))
limit = 50 # Number of contacts to retrieve
offset = 0 # Starting point for the list of contacts
try:
# Fetch contacts
api_response = api_instance.get_contacts(limit=limit, offset=offset)
pprint(api_response)
except ApiException as e:
print("Exception when calling ContactsApi->get_contacts: %s\n" % e)
Replace 'YOUR_API_KEY'
with the API key you generated earlier. This script configures the API client, sets the parameters for the number of contacts to retrieve, and makes the API call to fetch contacts from Brevo.
Running Your Python Script and Verifying the Output
Execute the script from your terminal or command line:
python get_brevo_contacts.py
If successful, the script will print the list of contacts retrieved from your Brevo account. You can verify the output by checking the contact details in your Brevo dashboard.
Handling Errors and Understanding Brevo API Response Codes
When making API calls, it's crucial to handle potential errors. The Brevo API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 429 Too Many Requests: Rate limit exceeded. Brevo allows a specific number of requests per minute.
Ensure your application gracefully handles these errors by implementing appropriate error handling mechanisms in your code.
For more details on error codes, refer to the Brevo API documentation.
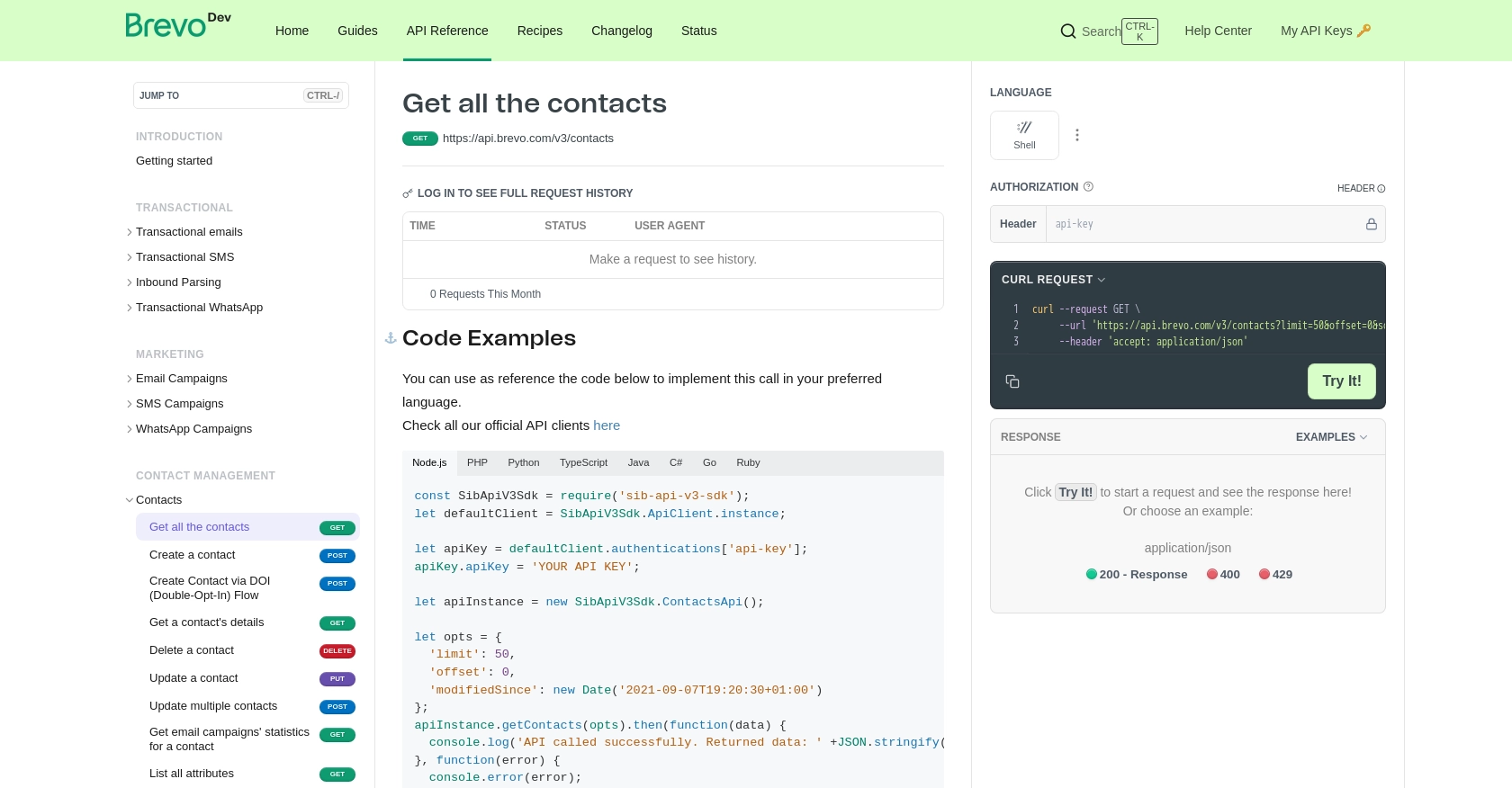
Conclusion and Best Practices for Using Brevo API in Python
Integrating with the Brevo API using Python can significantly enhance your ability to manage contacts and automate marketing efforts. By following the steps outlined in this guide, you can efficiently retrieve contact information and ensure seamless communication across platforms.
Best Practices for Secure and Efficient Brevo API Integration
- Secure API Key Storage: Always store your API keys securely. Consider using environment variables or a secure vault to keep them safe from unauthorized access.
- Handle Rate Limiting: Be mindful of Brevo's rate limits to avoid exceeding the allowed number of requests. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that contact data retrieved from Brevo is transformed and standardized to fit your application's requirements, facilitating smooth data integration.
- Error Handling: Implement robust error handling to manage API response codes effectively. This includes logging errors and providing meaningful feedback to users.
Enhance Your Integration Experience with Endgrate
While integrating with Brevo API is straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Brevo. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration processes and enhance your product's capabilities.
Read More
Ready to get started?