How to Get Contacts with the Pipeliner CRM API in Javascript
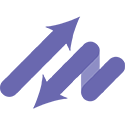
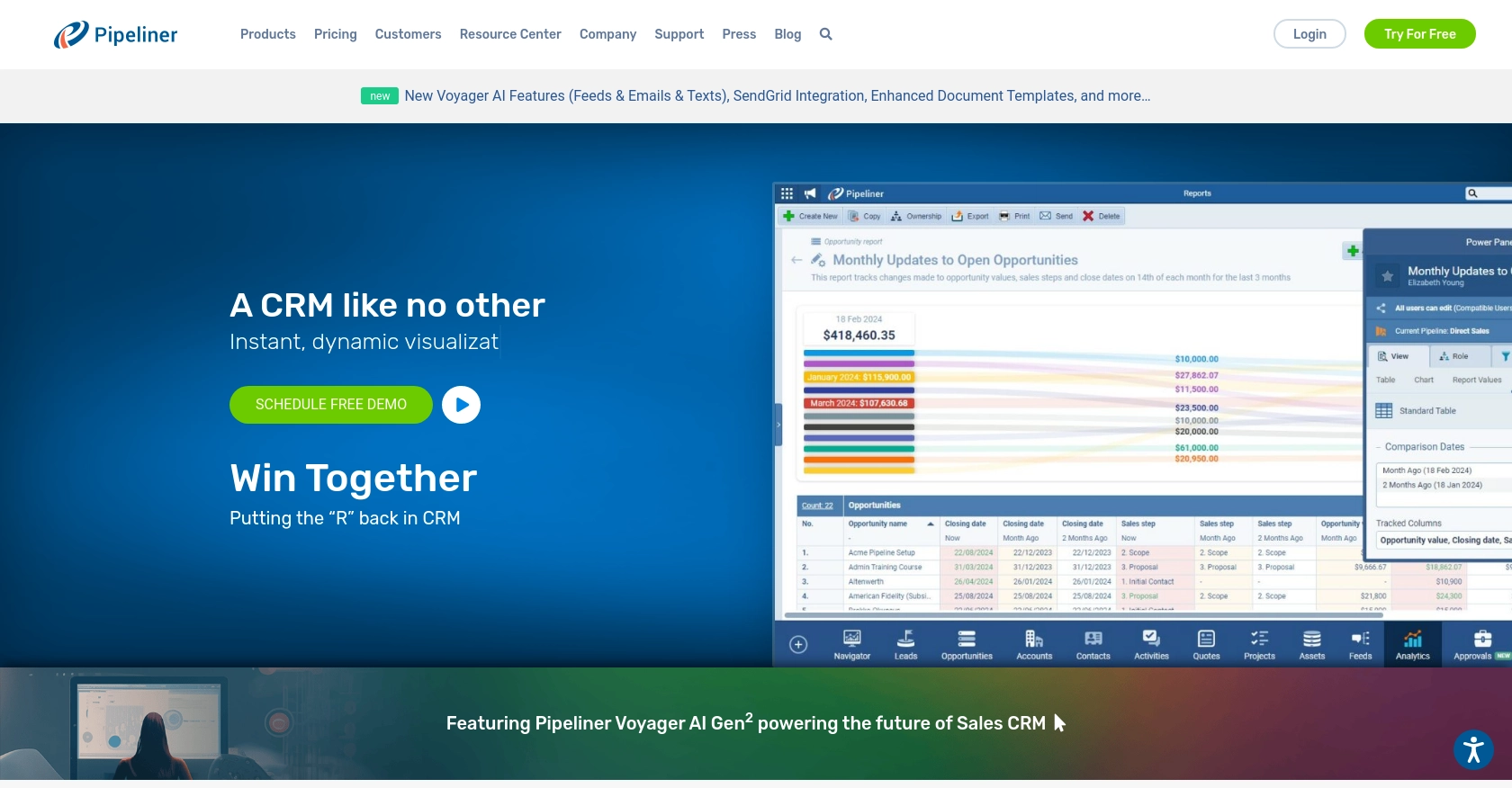
Introduction to Pipeliner CRM
Pipeliner CRM is a powerful customer relationship management platform designed to enhance sales processes and improve team collaboration. With its intuitive interface and robust features, Pipeliner CRM helps businesses manage contacts, track opportunities, and streamline workflows.
Integrating with Pipeliner CRM's API allows developers to automate and enhance CRM functionalities, such as retrieving and managing contact data. For example, a developer might use the Pipeliner CRM API to fetch contact details and synchronize them with other business applications, ensuring data consistency across platforms.
Setting Up Your Pipeliner CRM Test Account
Before you can start interacting with the Pipeliner CRM API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Pipeliner CRM Account
- Visit the Pipeliner CRM website and sign in using your Pipeliner CRM account credentials.
- Select your team space and navigate to the administration section.
Generating Pipeliner CRM API Keys
Pipeliner CRM uses Basic authentication, which requires a username and password. These are generated as API keys for your team space. Follow these steps to obtain them:
- Under the "Unit, Users & Roles" tab, open the "Applications" section.
- Create a new application and click on "Show API Access" to reveal the API keys.
- Store the generated username and password securely, as they are shown only once.
These credentials will be used for authenticating your API requests.
Understanding Pipeliner CRM API Authentication
Each API request requires the Space ID and Service URL, which are part of every request URL. Ensure you have these details ready for making API calls.
For more details on authentication, refer to the Pipeliner CRM Authentication Documentation.
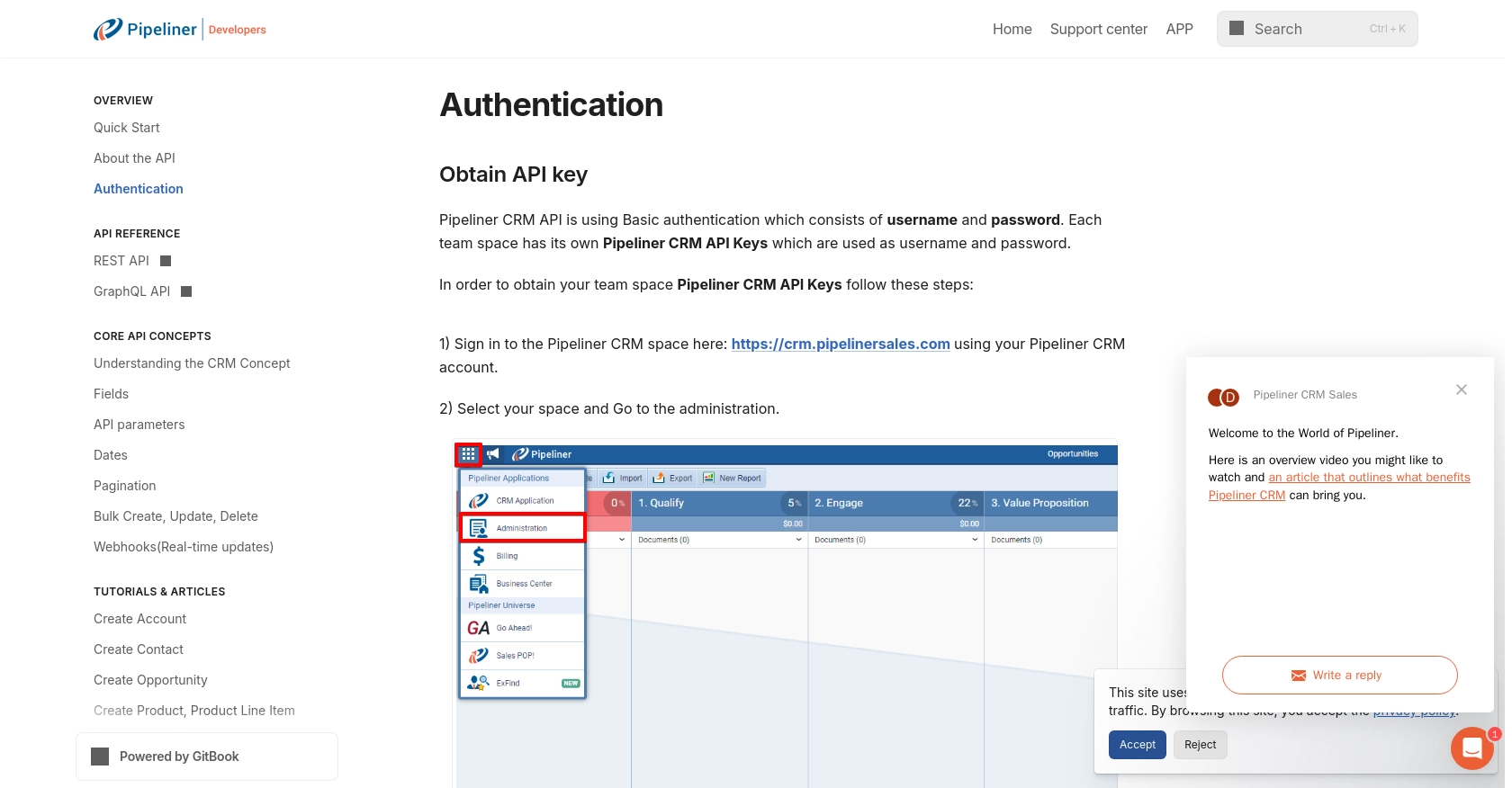
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Pipeliner CRM Using JavaScript
To interact with the Pipeliner CRM API and retrieve contact data, you'll need to set up your development environment with JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code to make API calls, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Pipeliner CRM API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment needed to execute JavaScript code outside a browser.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal. - Create a new project directory and navigate into it using your terminal.
- Initialize a new Node.js project by running
npm init -y
. - Install the Axios library for making HTTP requests by running
npm install axios
.
Writing JavaScript Code to Fetch Contacts from Pipeliner CRM
With your environment set up, you can now write the JavaScript code to interact with the Pipeliner CRM API. Use Axios to handle HTTP requests and responses.
const axios = require('axios');
// Define your API credentials and endpoint
const username = 'Your_Username';
const password = 'Your_Password';
const spaceId = 'Your_Space_ID';
const serviceUrl = 'Your_Service_URL';
// Create a function to fetch contacts
async function getContacts() {
try {
const response = await axios.get(`${serviceUrl}/entities/Contacts`, {
auth: {
username: username,
password: password
},
params: {
spaceId: spaceId
}
});
// Check if the request was successful
if (response.data.success) {
console.log('Contacts retrieved successfully:', response.data.data);
} else {
console.error('Failed to retrieve contacts:', response.data);
}
} catch (error) {
console.error('Error fetching contacts:', error.message);
}
}
// Call the function to fetch contacts
getContacts();
Replace Your_Username
, Your_Password
, Your_Space_ID
, and Your_Service_URL
with your actual Pipeliner CRM API credentials and details.
Understanding the API Response and Handling Errors
After executing the code, you should see the list of contacts printed in your console if the request is successful. The response includes a success
flag and a data
array containing the contact details.
In case of errors, the catch block will log the error message. Common issues might include incorrect credentials or network problems. Ensure your credentials are correct and your network connection is stable.
Verifying API Call Success in Pipeliner CRM
To confirm that the API call was successful, you can log into your Pipeliner CRM account and check the contacts section. The data retrieved by your API call should match the contacts listed in your CRM space.
For more information on handling API responses and errors, refer to the Pipeliner CRM API Documentation.
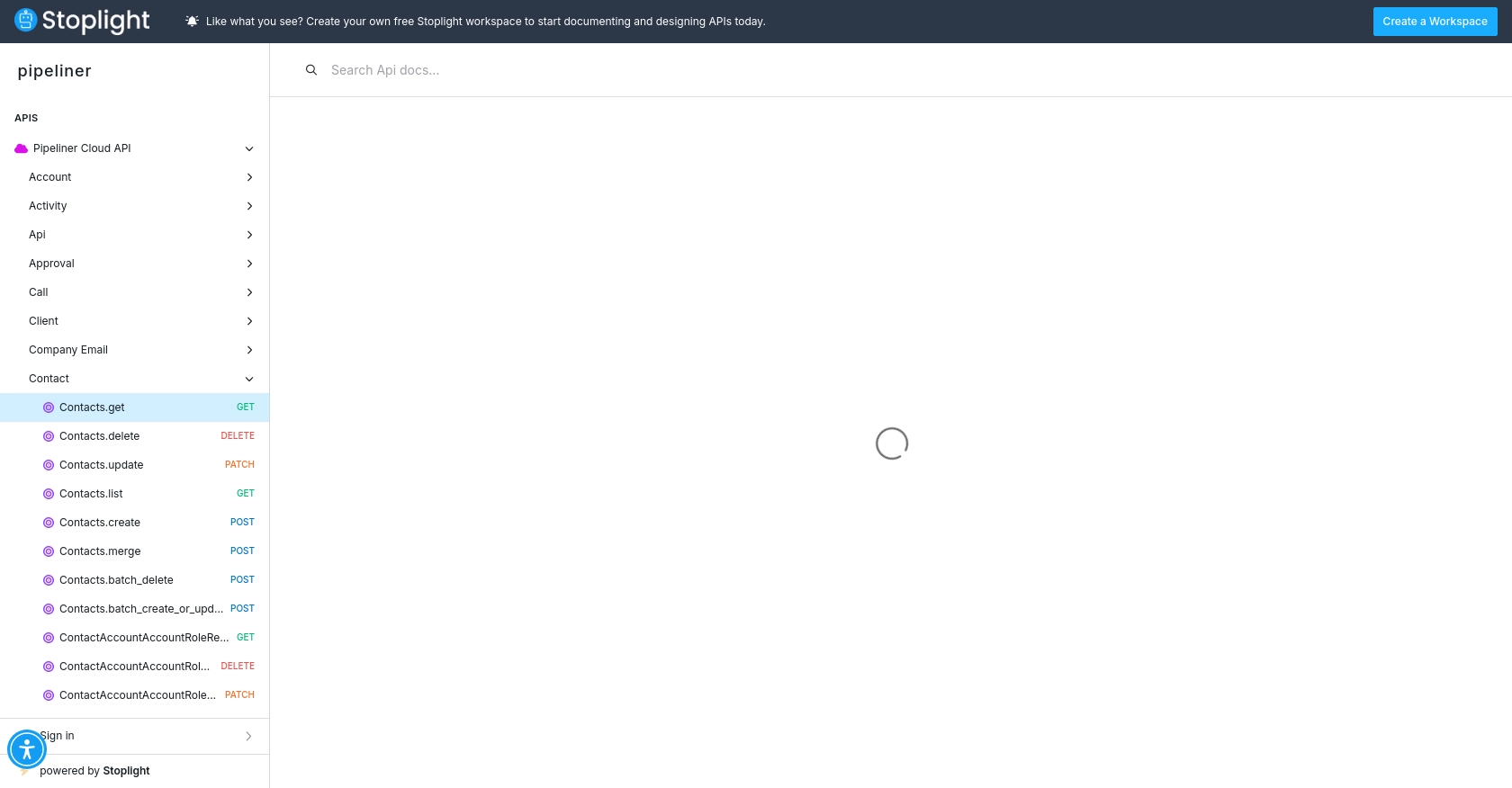
Conclusion and Best Practices for Pipeliner CRM API Integration
Integrating with the Pipeliner CRM API using JavaScript can significantly enhance your application's ability to manage and synchronize contact data efficiently. By following the steps outlined in this guide, you can seamlessly retrieve and handle contact information, ensuring your business processes remain streamlined and effective.
Best Practices for Secure and Efficient Pipeliner CRM API Usage
- Secure Storage of Credentials: Always store your Pipeliner CRM API credentials securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid service interruptions. Implement retry logic with exponential backoff to manage requests efficiently.
- Data Standardization: Ensure that the data retrieved from Pipeliner CRM is standardized and transformed as needed to maintain consistency across different platforms.
- Error Handling: Implement robust error handling to manage potential issues such as network failures or incorrect API usage. Log errors for monitoring and debugging purposes.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider leveraging Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Pipeliner CRM, allowing you to focus on your core product while ensuring a seamless integration experience for your customers.
By using Endgrate, you can save time and resources, build once for each use case, and enjoy an intuitive integration experience. Visit Endgrate to learn more about how it can support your integration needs.
Read More
Ready to get started?