Using the Copper API to Get Opportunities (with Python examples)
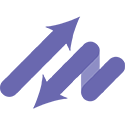
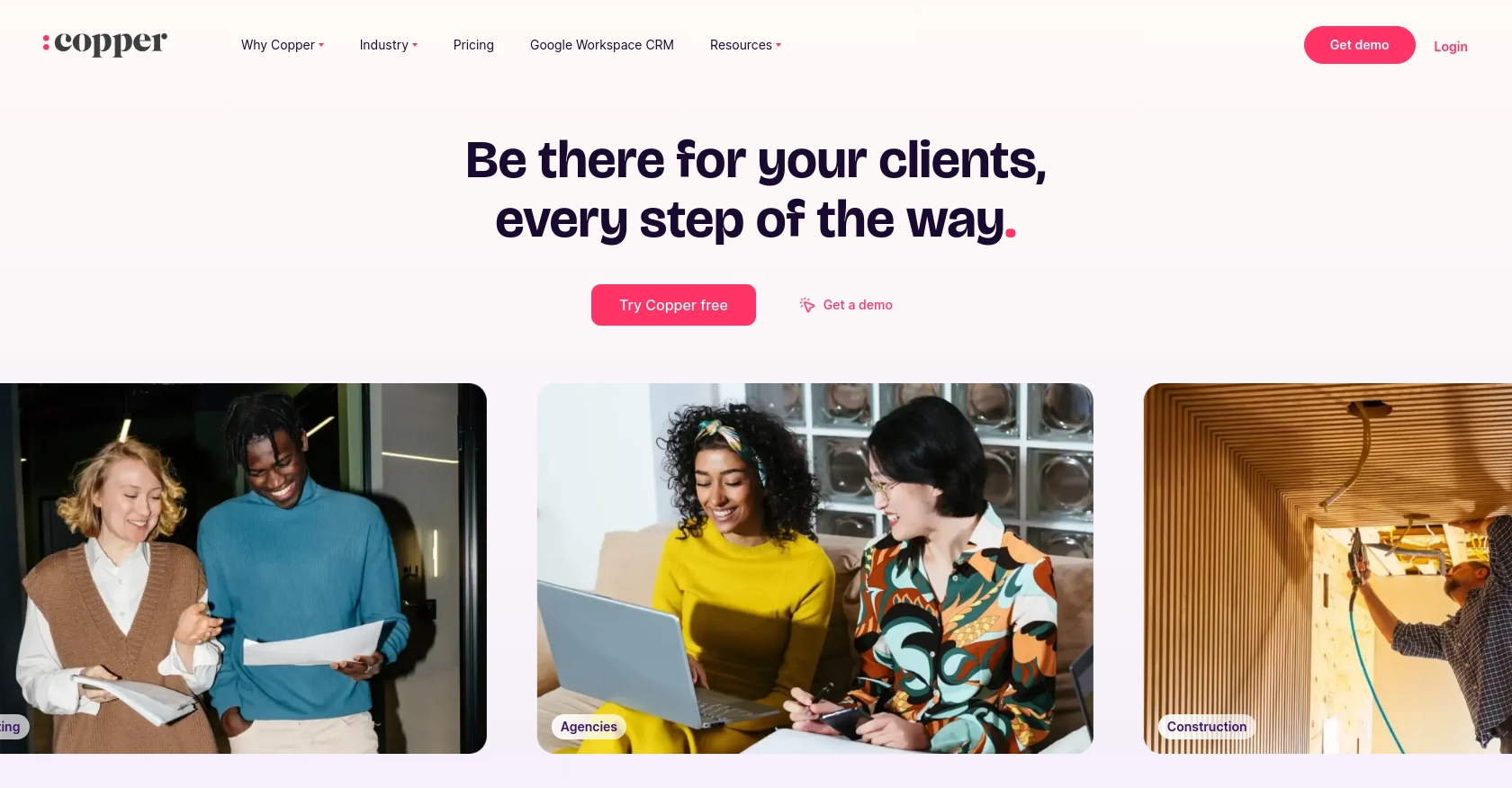
Introduction to Copper API
Copper is a robust platform designed to streamline financial operations, offering a suite of tools for managing digital assets. It provides a secure environment for trading, transferring, and managing cryptocurrencies, making it a preferred choice for businesses seeking efficient financial solutions.
Integrating with Copper's API allows developers to automate and enhance their financial operations. For example, using the Copper API, developers can retrieve opportunities such as trading orders or portfolio details, enabling more informed decision-making and streamlined asset management.
This article will guide you through using Python to interact with the Copper API, focusing on retrieving opportunities. By the end of this tutorial, you'll be equipped to efficiently access and manage financial data on the Copper platform.
Setting Up Your Copper API Test/Sandbox Account
Before you can start interacting with the Copper API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data, making it an essential step for developers looking to integrate Copper's capabilities into their applications.
Creating a Copper Sandbox Account
To begin, you'll need to create a Copper sandbox account. Follow these steps:
- Visit the Copper website and navigate to the developer section.
- Sign up for a sandbox account using your email address. This account will provide you with access to Copper's API in a test environment.
- Once registered, log in to your sandbox account to access the developer dashboard.
Generating Copper API Keys
With your sandbox account set up, the next step is to generate API keys. These keys are crucial for authenticating your API requests:
- In the developer dashboard, locate the API keys section.
- Generate a new API key. You will receive two strings: the API key and the API key secret.
- Store these keys securely, as they will be used to authenticate your API requests.
Understanding Copper API Authentication
Copper uses a custom authentication method that requires the following headers in each API request:
- Authorization: Use the format
ApiKey {Your_API_Key}
. - X-Timestamp: Include the current UNIX timestamp in milliseconds.
- X-Signature: Generate a signature using HMAC SHA256 with your API secret.
Example Code for Copper API Authentication in Python
Below is an example of how to set up authentication for Copper API requests using Python:
import hashlib
import hmac
import requests
import time
ApiKey = 'Your_API_Key'
Secret = 'Your_API_Secret'
timestamp = str(round(time.time() * 1000))
method = "GET"
path = "/platform/accounts"
body = ""
signature = hmac.new(
key=bytes(Secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
url = 'https://api.copper.co' + path
response = requests.get(url, headers={
'Authorization': 'ApiKey ' + ApiKey,
'X-Signature': signature,
'X-Timestamp': timestamp
})
print(response.json())
Replace Your_API_Key
and Your_API_Secret
with the keys you generated earlier. This code sets up the necessary headers and makes a GET request to the Copper API.
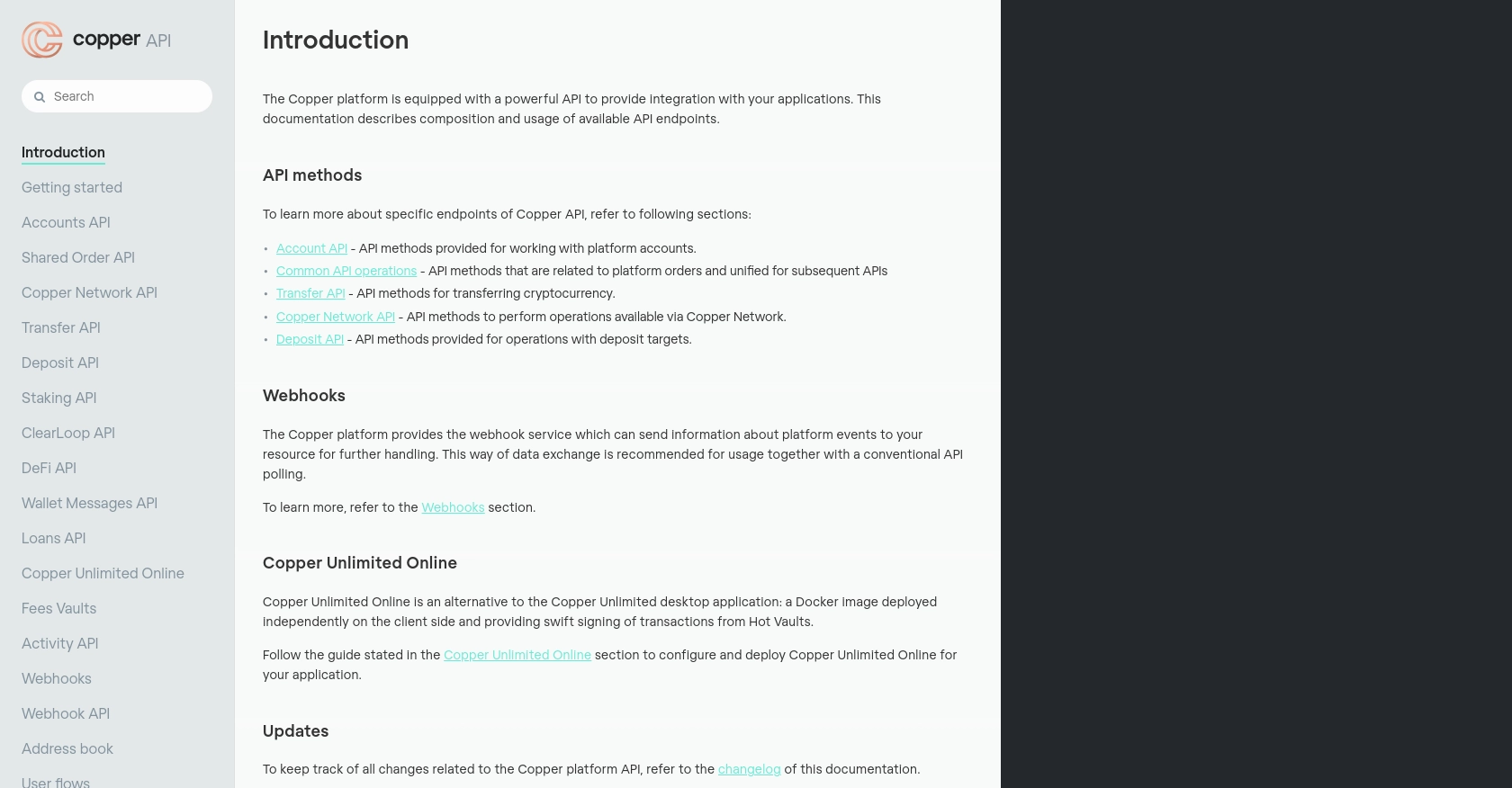
sbb-itb-96038d7
Executing Copper API Calls with Python
To effectively interact with the Copper API, you'll need to understand how to make API calls using Python. This section will guide you through the process of retrieving opportunities from Copper, ensuring you have the tools to manage your financial data efficiently.
Python Setup and Dependencies for Copper API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Making a GET Request to Retrieve Opportunities from Copper
To retrieve opportunities, such as trading orders or portfolio details, you'll need to make a GET request to the Copper API. Here's a step-by-step guide:
- Set the API endpoint for retrieving opportunities. For example, use
/platform/opportunities
. - Prepare the headers with the necessary authentication details, including the API key, timestamp, and signature.
- Execute the GET request and handle the response.
Python Code Example for Copper API GET Request
Below is a Python code example demonstrating how to make a GET request to the Copper API to retrieve opportunities:
import hashlib
import hmac
import requests
import time
ApiKey = 'Your_API_Key'
Secret = 'Your_API_Secret'
timestamp = str(round(time.time() * 1000))
method = "GET"
path = "/platform/opportunities"
body = ""
signature = hmac.new(
key=bytes(Secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
url = 'https://api.copper.co' + path
response = requests.get(url, headers={
'Authorization': 'ApiKey ' + ApiKey,
'X-Signature': signature,
'X-Timestamp': timestamp
})
if response.status_code == 200:
print("Opportunities retrieved successfully:")
print(response.json())
else:
print("Failed to retrieve opportunities:", response.status_code, response.text)
Replace Your_API_Key
and Your_API_Secret
with your actual API credentials. This code sets up the request headers, makes the API call, and prints the retrieved opportunities if successful.
Handling Copper API Response and Errors
After making the API call, it's crucial to handle the response correctly. Check the status code to verify success (HTTP 200) or identify errors. Common error codes include:
- 400 Bad Request: Validation errors, such as missing required fields.
- 401 Unauthorized: Invalid API key or signature.
- 429 Request Limit Exceeded: Too many requests in a short period.
For more detailed error handling, refer to the Copper API documentation at Copper API Documentation.
Best Practices for Using Copper API in Python
When working with the Copper API, it's essential to follow best practices to ensure secure and efficient integration. Here are some key recommendations:
- Securely Store API Credentials: Always store your API key and secret securely, using environment variables or a secure vault, to prevent unauthorized access.
- Implement Rate Limiting: Copper API has a rate limit of 30,000 requests per 5 minutes per IP address. Implement logic to handle rate limits gracefully and avoid exceeding them.
- Handle Errors Appropriately: Use error handling to manage API response codes effectively. Log errors for debugging and provide user-friendly messages for known issues.
- Data Transformation and Standardization: Ensure that data retrieved from the Copper API is transformed and standardized according to your application's requirements for consistency and accuracy.
Leveraging Endgrate for Efficient Copper API Integrations
Integrating multiple APIs can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Copper. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop integration logic once and apply it across multiple platforms, reducing redundancy.
- Enhance User Experience: Offer your users a seamless and intuitive integration experience with minimal effort.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Conclusion on Copper API Integration with Python
Integrating with the Copper API using Python provides a powerful way to automate and enhance financial operations. By following the steps outlined in this guide, you can efficiently retrieve and manage opportunities on the Copper platform. Remember to adhere to best practices for security and efficiency, and consider leveraging tools like Endgrate to simplify your integration processes.
For more information and detailed documentation, visit the Copper API Documentation.
Read More
Ready to get started?