How to Send Messages (Channel) with the Slack API in Python
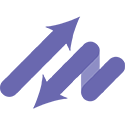
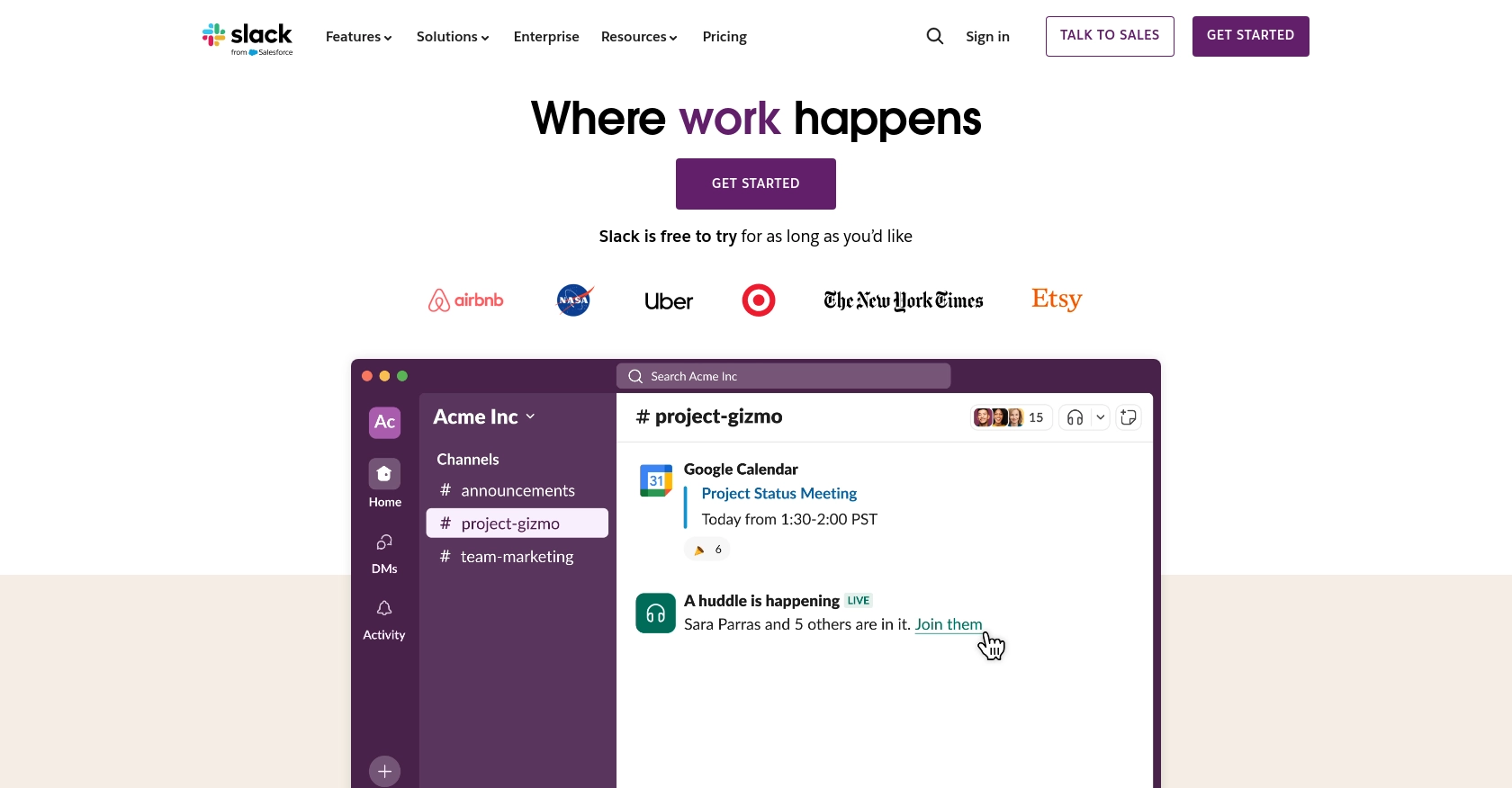
Introduction to Slack API Integration
Slack is a powerful collaboration platform that facilitates communication and teamwork across organizations. With features like channels, direct messaging, and integrations, Slack has become an essential tool for businesses aiming to enhance productivity and streamline communication.
Integrating with Slack's API allows developers to automate and enhance interactions within Slack workspaces. For example, a developer might want to send automated messages to a channel to notify team members of important updates or events. This can be achieved using the Slack API in Python, enabling seamless communication and efficient workflow management.
Setting Up Your Slack Test/Sandbox Account
Before you can start sending messages with the Slack API, you need to set up a test or sandbox account. This will allow you to safely develop and test your integration without affecting your live workspace.
Create a Slack App for API Integration
To interact with Slack's API, you'll need to create a Slack app. Follow these steps to set up your app:
- Visit the Slack Apps page and click on Create New App.
- Select From scratch and enter your app's name, such as "Message Bot".
- Choose the workspace where you want to develop your app and click Create App.
Request Necessary OAuth Scopes for Slack API
To send messages, your app needs specific permissions. Configure these scopes as follows:
- Navigate to OAuth & Permissions in your app settings.
- Scroll to the Scopes section and add the following scopes under Bot Token Scopes:
chat:write
- Allows your app to send messages.channels:read
- Enables your app to read channel information.
Install and Authorize Your Slack App
Once you've set up the necessary scopes, install your app to your workspace:
- Go back to the Basic Information section and click Install to Workspace.
- Follow the authorization flow and click Allow to grant the app permissions.
- After installation, you'll receive an OAuth access token. Store this token securely as it will be used to authenticate API requests.
Invite Your Slack App to a Channel
To send messages, your app must be a member of the channel. Use the following steps to invite your app:
- Open Slack and navigate to the channel where you want to test your app.
- Use the slash command
/invite @YourAppName
to add your app to the channel.
With your Slack app set up and authorized, you're ready to start sending messages using the Slack API in Python.
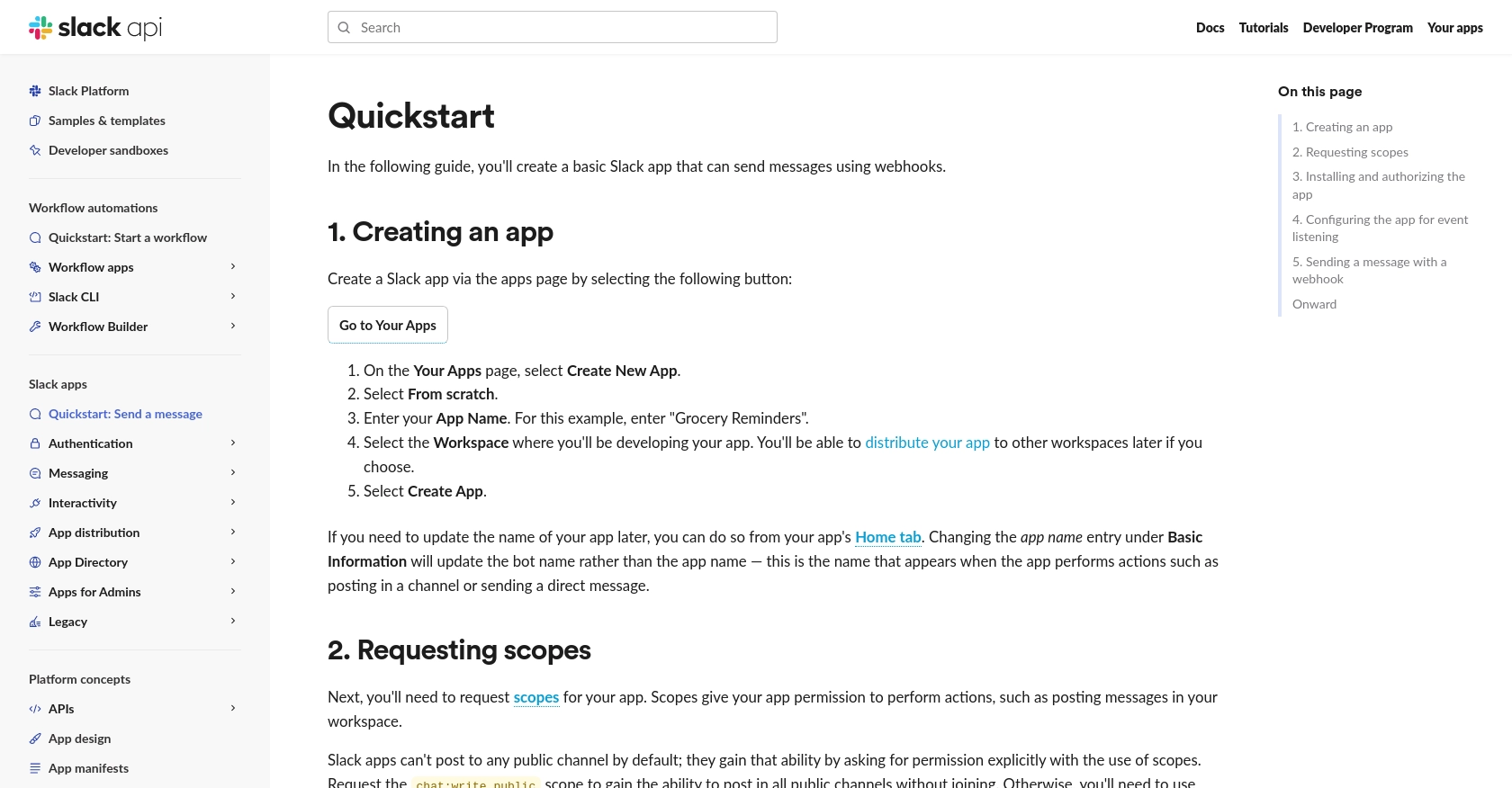
sbb-itb-96038d7
How to Make the Actual API Call to Send Messages with Slack API in Python
Now that your Slack app is set up and authorized, it's time to dive into the actual process of sending messages using the Slack API in Python. This section will guide you through the necessary steps, including setting up your Python environment, writing the code, and handling potential errors.
Setting Up Python Environment for Slack API Integration
Before making API calls, ensure your Python environment is ready. You'll need Python 3.x and the Slack SDK for Python. Follow these steps to set up your environment:
- Ensure Python 3.x is installed on your machine. You can download it from the official Python website.
- Install the Slack SDK for Python using pip:
pip install slack-sdk
Writing Python Code to Send Messages Using Slack API
With your environment set up, you can now write the Python code to send messages to a Slack channel. Here's a step-by-step guide:
- Create a new Python file named
send_slack_message.py
. - Import the necessary modules and set up the Slack client:
import os
from slack_sdk import WebClient
from slack_sdk.errors import SlackApiError
# Initialize the Slack client with your OAuth token
client = WebClient(token=os.environ['SLACK_BOT_TOKEN'])
- Define a function to send a message to a specific channel:
def send_message(channel_id, text):
try:
# Use the chat.postMessage method to send a message
response = client.chat_postMessage(
channel=channel_id,
text=text
)
print(f"Message sent: {response['message']['text']}")
except SlackApiError as e:
print(f"Error sending message: {e.response['error']}")
- Call the function with the channel ID and message text:
if __name__ == "__main__":
channel_id = "YOUR_CHANNEL_ID"
message_text = "Hello, Slack!"
send_message(channel_id, message_text)
Verifying Successful Message Delivery in Slack
After running your script, verify that the message was successfully sent by checking the specified Slack channel. You should see the message appear in the channel, confirming that the API call was successful.
Handling Errors and Error Codes in Slack API
When interacting with the Slack API, it's crucial to handle potential errors gracefully. The Slack SDK for Python provides detailed error messages that can help you troubleshoot issues. Common error codes include:
invalid_auth
: The provided token is invalid.channel_not_found
: The specified channel ID does not exist.rate_limited
: The app has exceeded the rate limits for API calls.
Refer to the Slack API documentation for more information on error codes and handling strategies.
.webp)
Conclusion and Best Practices for Sending Messages with Slack API in Python
Integrating with the Slack API to send messages using Python is a powerful way to enhance communication within your workspace. By following the steps outlined in this guide, you can automate notifications, streamline workflows, and improve team collaboration.
Best Practices for Secure and Efficient Slack API Integration
- Securely Store OAuth Tokens: Always store your OAuth tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Slack's rate limits, which can vary based on the API method. Implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Slack API documentation.
- Validate API Responses: Always check the response from Slack API calls to ensure successful execution and handle any errors appropriately.
- Use Descriptive Logging: Implement logging to track API requests and responses, which can aid in debugging and monitoring your integration.
Enhance Your Integration Experience with Endgrate
While building integrations with the Slack API can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution to manage integrations efficiently. By using Endgrate, you can:
- Save time and resources by outsourcing integration development.
- Focus on your core product while Endgrate handles the complexities of multiple integrations.
- Provide an intuitive integration experience for your customers with a unified API endpoint.
Explore how Endgrate can simplify your integration process by visiting Endgrate's website.
Read More
Ready to get started?