Using the Mixpanel API to Get Profiles in Javascript
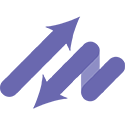
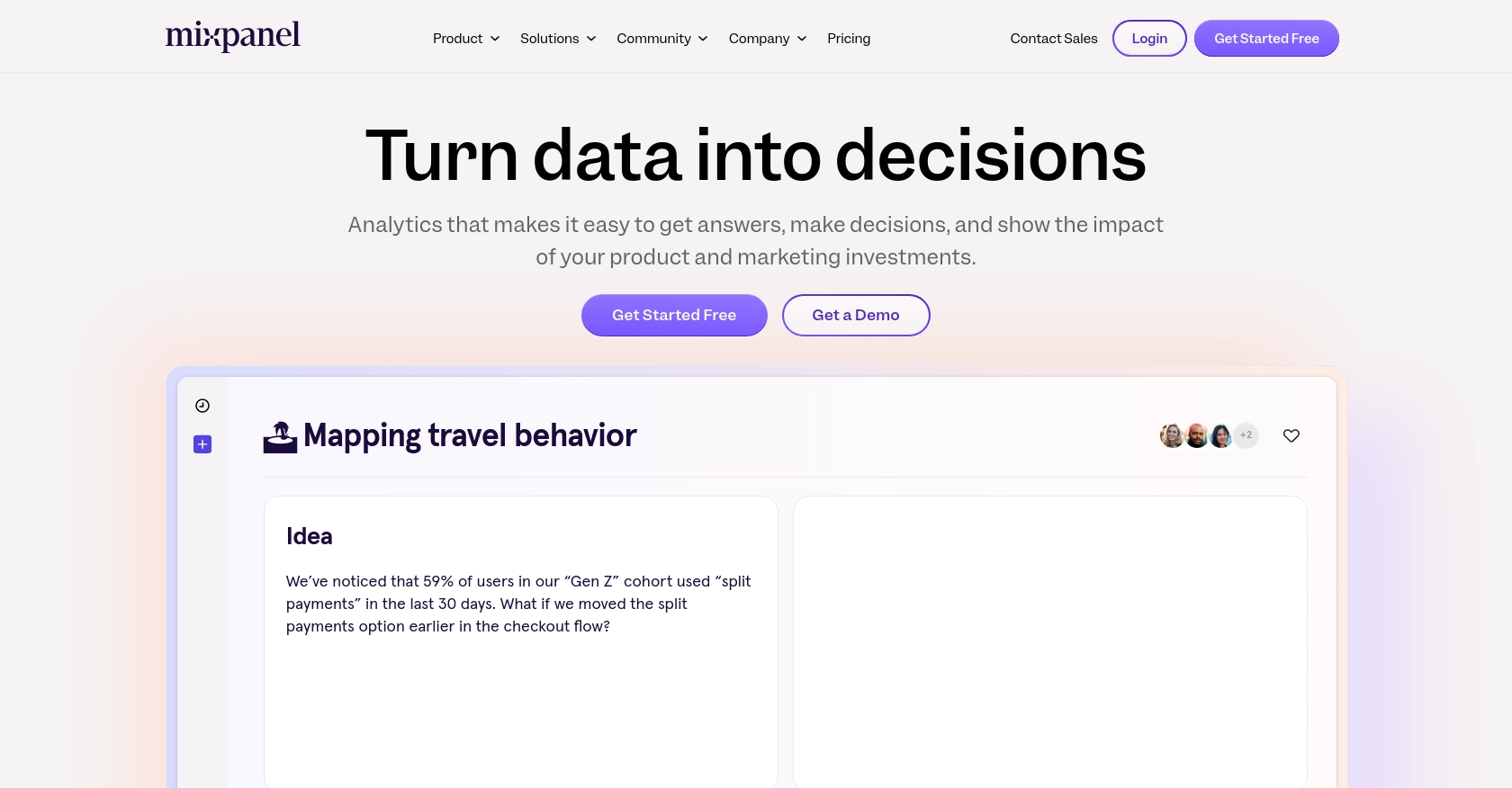
Introduction to Mixpanel API
Mixpanel is a powerful analytics platform that enables businesses to track user interactions and gain insights into customer behavior. It offers a comprehensive suite of tools for analyzing user data, creating reports, and driving data-driven decisions.
Developers often integrate with Mixpanel's API to access detailed user profiles and behavioral data. For example, a developer might use the Mixpanel API to retrieve user profiles in order to personalize user experiences or to analyze user engagement patterns across different segments.
This article will guide you through the process of using JavaScript to interact with the Mixpanel API, specifically focusing on retrieving user profiles. By following this tutorial, you will learn how to efficiently access and manage user data on the Mixpanel platform using JavaScript.
Setting Up Your Mixpanel Test Account
Before diving into the Mixpanel API, it's essential to set up a test account to safely experiment with API calls and data retrieval. Mixpanel provides developers with the ability to create service accounts, which are ideal for backend services and scripts.
Creating a Mixpanel Service Account
To interact with the Mixpanel API, you'll need a service account. This account allows you to authenticate API requests securely. Follow these steps to create a service account:
- Log in to your Mixpanel account and navigate to the Organization Settings.
- In the settings menu, find the Service Accounts tab.
- Click on Create Service Account and fill in the necessary details.
- Select the appropriate role and projects for the service account. Ensure it has the permissions needed for your API interactions.
- Once created, securely store the service account's username and secret. You will need these credentials for authentication, and they cannot be retrieved again.
For more details, refer to the Mixpanel Service Accounts documentation.
Authenticating with Mixpanel API Using Service Account
Mixpanel's API uses HTTP Basic Auth for service account authentication. Here's how you can set it up in JavaScript:
const username = 'your_service_account_username';
const secret = 'your_service_account_secret';
const auth = btoa(`${username}:${secret}`);
fetch('https://mixpanel.com/api/query/engage', {
method: 'GET',
headers: {
'Authorization': `Basic ${auth}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace your_service_account_username
and your_service_account_secret
with your actual credentials. This code snippet demonstrates how to authenticate and make a basic API call to Mixpanel.
Testing Your Mixpanel API Setup
After setting up your service account and authentication, it's crucial to test your configuration:
- Run the JavaScript code snippet above to ensure it successfully retrieves data from Mixpanel.
- If you encounter any errors, double-check your credentials and ensure they are correctly encoded.
By following these steps, you can confidently set up and test your Mixpanel API integration, paving the way for more advanced data interactions.
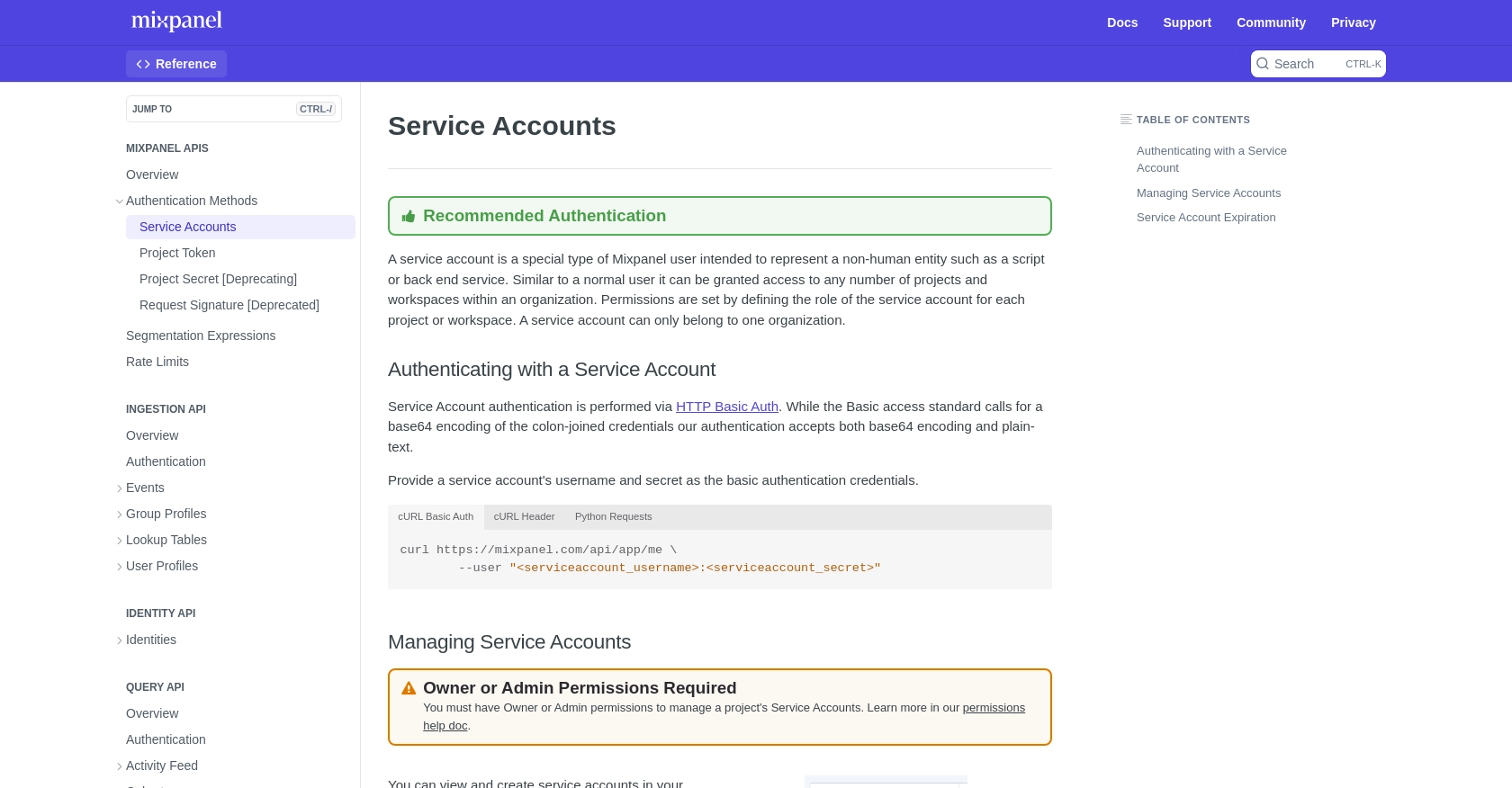
sbb-itb-96038d7
Making API Calls to Retrieve User Profiles from Mixpanel Using JavaScript
Once you have set up your Mixpanel service account and authenticated successfully, you can proceed to make API calls to retrieve user profiles. This section will guide you through the process of making these calls using JavaScript, ensuring you can efficiently access and manage user data on the Mixpanel platform.
Setting Up JavaScript Environment for Mixpanel API
Before making API calls, ensure your JavaScript environment is ready. You should have a basic understanding of JavaScript and be familiar with using the Fetch API for making HTTP requests. Here's what you need:
- A modern web browser or Node.js environment to run JavaScript code.
- Basic knowledge of JavaScript promises and asynchronous operations.
Executing Mixpanel API Calls to Fetch User Profiles
To retrieve user profiles from Mixpanel, you will use the Engage API endpoint. The following JavaScript code demonstrates how to make a GET request to this endpoint:
const username = 'your_service_account_username';
const secret = 'your_service_account_secret';
const auth = btoa(`${username}:${secret}`);
fetch('https://mixpanel.com/api/query/engage', {
method: 'GET',
headers: {
'Authorization': `Basic ${auth}`
}
})
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => {
console.log('User Profiles:', data);
})
.catch(error => console.error('Error fetching user profiles:', error));
Replace your_service_account_username
and your_service_account_secret
with your actual credentials. This code snippet demonstrates how to authenticate and retrieve user profiles from Mixpanel.
Handling API Response and Errors
When making API calls, it's crucial to handle responses and potential errors effectively:
- Check the response status to ensure the request was successful. A status code of 200 indicates success.
- Use the
catch
block to handle any errors that occur during the fetch operation. - Log the retrieved user profiles to the console for verification.
Verifying API Call Success in Mixpanel
After executing the API call, verify the success of your request by checking the console output for user profiles. You can also cross-reference the retrieved data with the profiles available in your Mixpanel dashboard.
By following these steps, you can efficiently interact with the Mixpanel API to retrieve user profiles, enabling you to leverage user data for enhanced analytics and personalized user experiences.
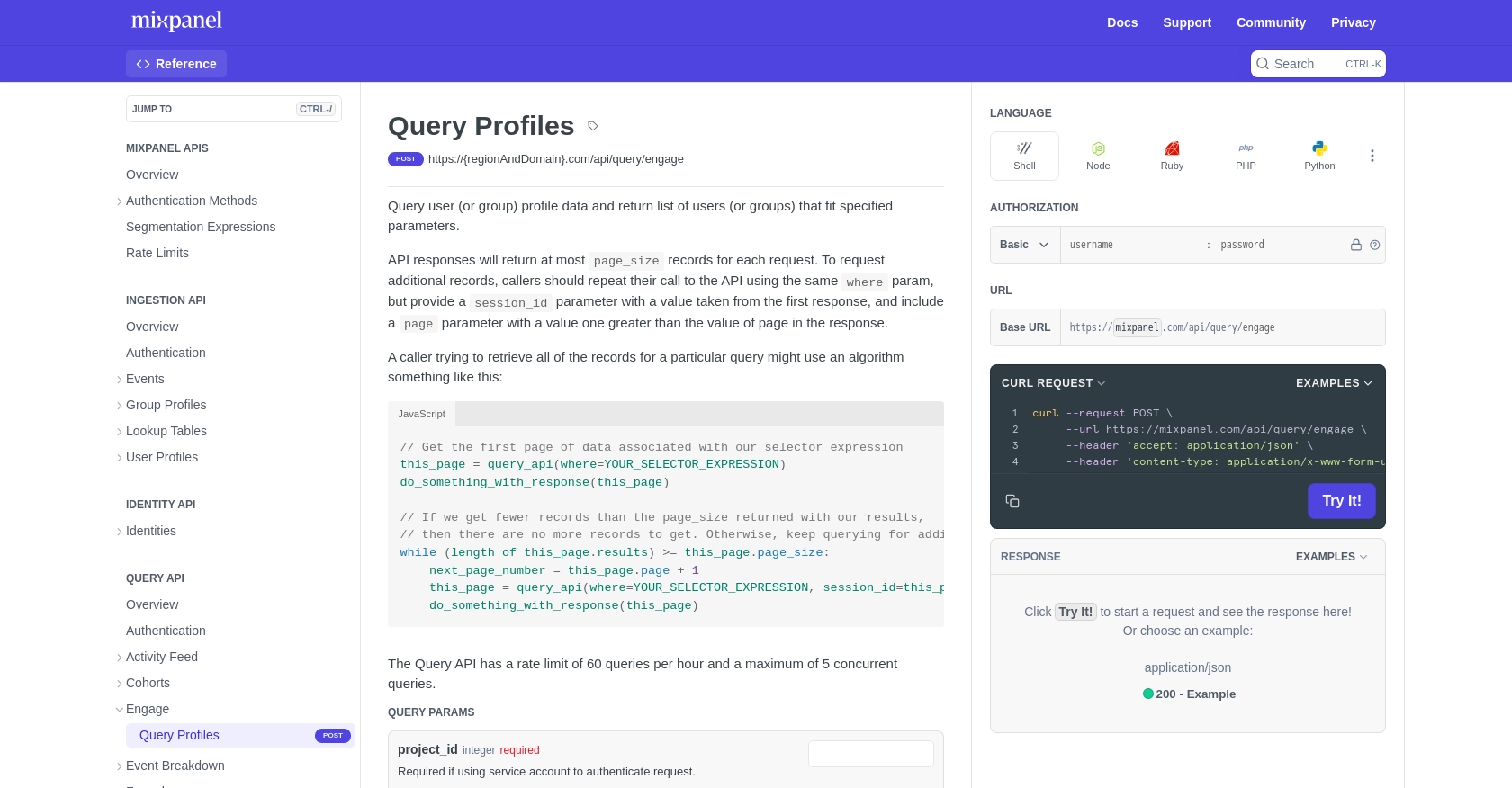
Conclusion and Best Practices for Using Mixpanel API with JavaScript
Integrating with the Mixpanel API using JavaScript provides developers with powerful tools to access and analyze user data, enabling data-driven decisions and personalized user experiences. By following the steps outlined in this guide, you can efficiently retrieve user profiles and leverage Mixpanel's analytics capabilities.
Best Practices for Secure and Efficient Mixpanel API Integration
- Securely Store Credentials: Always store your service account credentials securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limits: Mixpanel imposes rate limits on API calls. Ensure your application handles these limits gracefully by spreading out requests or consolidating queries. For more details, refer to the Mixpanel Rate Limits documentation.
- Error Handling: Implement robust error handling to manage API response errors and network issues. This ensures your application can recover gracefully from unexpected failures.
- Data Transformation: Consider transforming and standardizing data fields to maintain consistency across different systems and applications.
Enhancing Your Integration Strategy with Endgrate
While integrating with Mixpanel is a powerful way to harness user data, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Mixpanel. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover the benefits of a unified integration strategy.
Read More
Ready to get started?