Using the BigCommerce API to Create Or Update Products in PHP
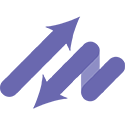
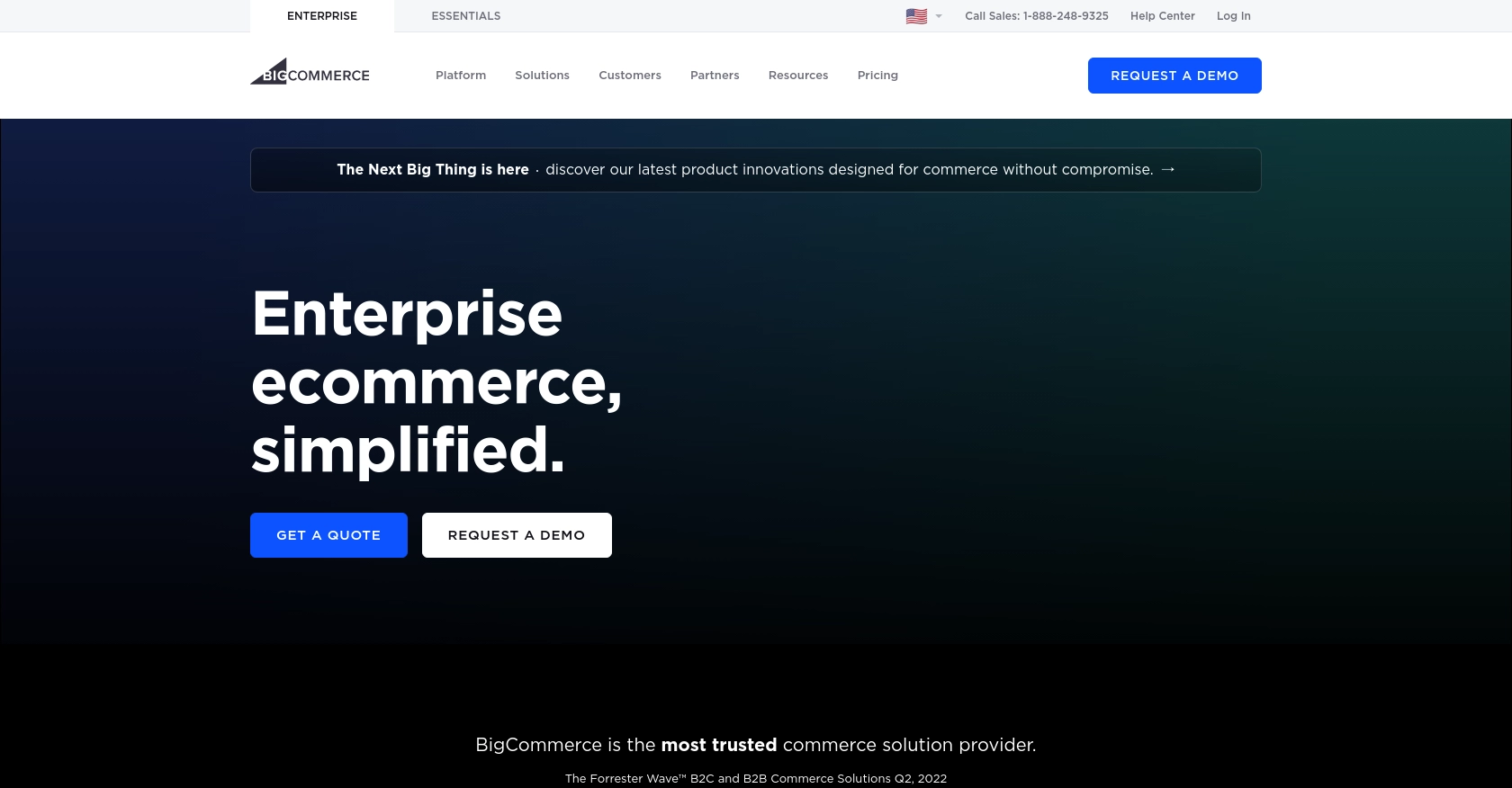
Introduction to BigCommerce API Integration
BigCommerce is a powerful e-commerce platform that provides businesses with the tools they need to create and manage online stores. With its robust set of features, BigCommerce supports everything from product management to order processing, making it a popular choice for businesses looking to scale their online presence.
Integrating with the BigCommerce API allows developers to automate and streamline various aspects of store management. For example, using the API to create or update products can significantly reduce the time spent on manual data entry, ensuring that product information is always up-to-date and accurate.
This article will guide you through the process of using PHP to interact with the BigCommerce API, specifically focusing on creating and updating products. By the end of this tutorial, you'll be able to efficiently manage product data within your BigCommerce store programmatically.
Setting Up Your BigCommerce API Test Account
Before you can start integrating with the BigCommerce API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting a live store. BigCommerce provides sandbox stores for developers to test their integrations.
Creating a BigCommerce Sandbox Store
To begin, you'll need to create a sandbox store. Follow these steps:
- Visit the BigCommerce Developer Portal and sign up for a developer account if you haven't already.
- Once logged in, navigate to the "Sandbox Stores" section and click on "Create a Sandbox Store."
- Fill out the necessary details and submit the form to create your sandbox store.
Generating API Credentials for BigCommerce
After setting up your sandbox store, you'll need to generate API credentials to authenticate your requests:
- Go to the "API Accounts" section in your BigCommerce control panel.
- Click on "Create API Account" and select "V2/V3 API Token" as the type.
- Provide a name for your API account and set the necessary OAuth scopes for product management.
- Once created, you'll receive an Access Token. Make sure to store this securely as it will be used to authenticate your API requests.
Understanding BigCommerce API Authentication
BigCommerce uses the X-Auth-Token
header for authenticating API requests. You'll need to include this token in the header of each request you make to the BigCommerce API. For more details on authentication, refer to the BigCommerce Authentication Documentation.
With your sandbox store and API credentials ready, you're all set to start integrating with the BigCommerce API using PHP.
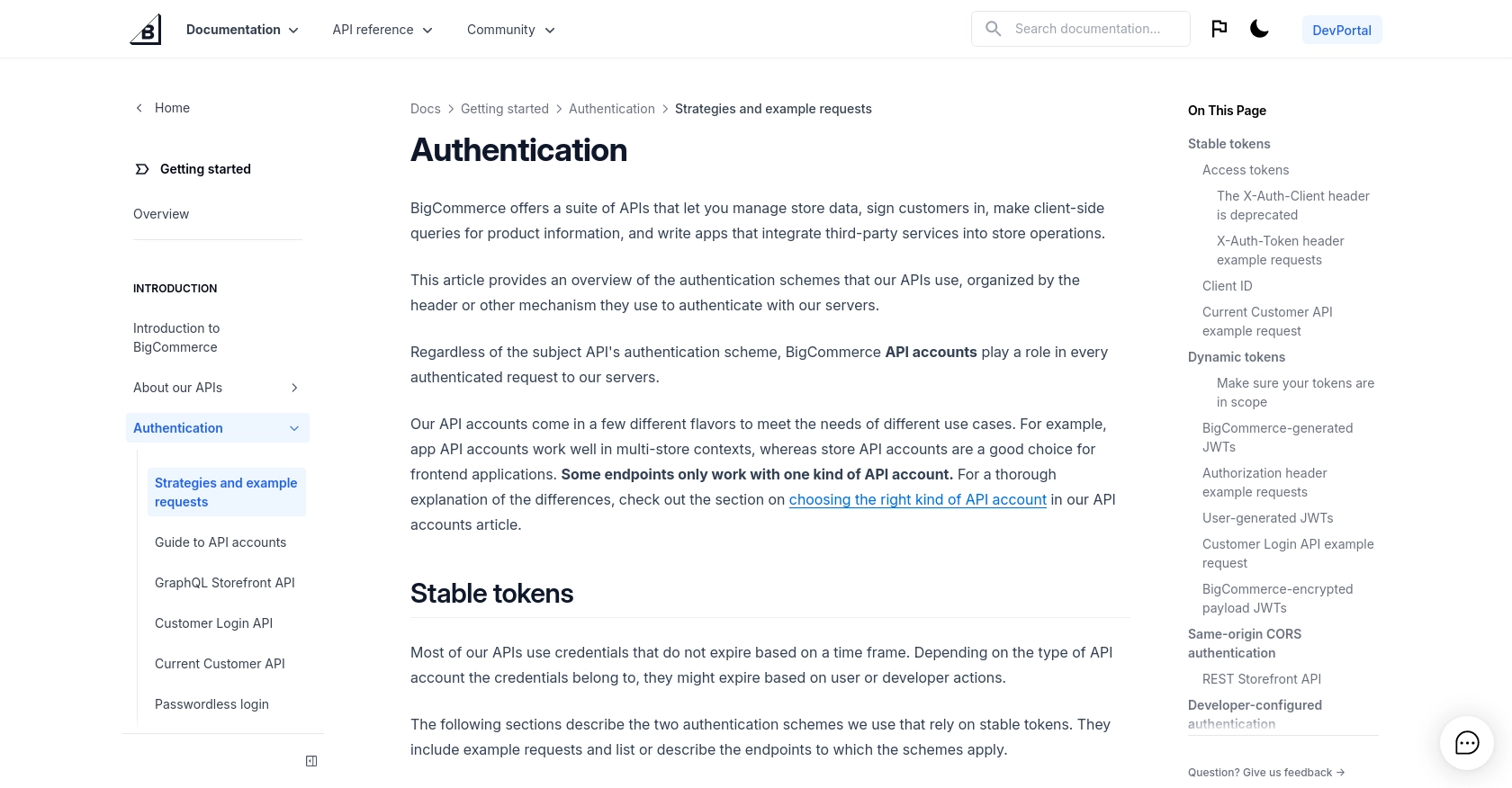
sbb-itb-96038d7
Making API Calls to BigCommerce Using PHP
To interact with the BigCommerce API for creating or updating products, you'll need to use PHP to send HTTP requests. This section will guide you through setting up your PHP environment and making API calls to BigCommerce.
Setting Up Your PHP Environment for BigCommerce API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or later
- The
cURL
extension enabled - Composer for dependency management
Install the necessary dependencies using Composer:
composer require guzzlehttp/guzzle
Creating a Product in BigCommerce Using PHP
To create a product in BigCommerce, you'll need to send a POST request to the appropriate endpoint. Here's a step-by-step guide:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://api.bigcommerce.com/stores/{store_hash}/v3/catalog/products', [
'headers' => [
'X-Auth-Token' => 'your_access_token',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'name' => 'New Product',
'type' => 'physical',
'price' => 19.99,
'weight' => 1.0,
],
]);
$data = json_decode($response->getBody(), true);
echo 'Product Created: ' . $data['data']['name'];
Replace your_access_token
with your actual access token and {store_hash}
with your store's hash. This code creates a new product with the specified details.
Updating a Product in BigCommerce Using PHP
To update an existing product, use a PUT request. Here's how you can update a product's details:
$response = $client->put('https://api.bigcommerce.com/stores/{store_hash}/v3/catalog/products/{product_id}', [
'headers' => [
'X-Auth-Token' => 'your_access_token',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'price' => 24.99,
'name' => 'Updated Product Name',
],
]);
$data = json_decode($response->getBody(), true);
echo 'Product Updated: ' . $data['data']['name'];
Replace {product_id}
with the ID of the product you want to update. This code updates the product's price and name.
Handling API Responses and Errors in BigCommerce
After making an API call, it's crucial to handle the response and any potential errors. Check the status code to ensure the request was successful:
if ($response->getStatusCode() == 200) {
echo 'Request successful!';
} else {
echo 'Error: ' . $response->getStatusCode();
}
Refer to the BigCommerce API documentation for more details on error codes and handling.
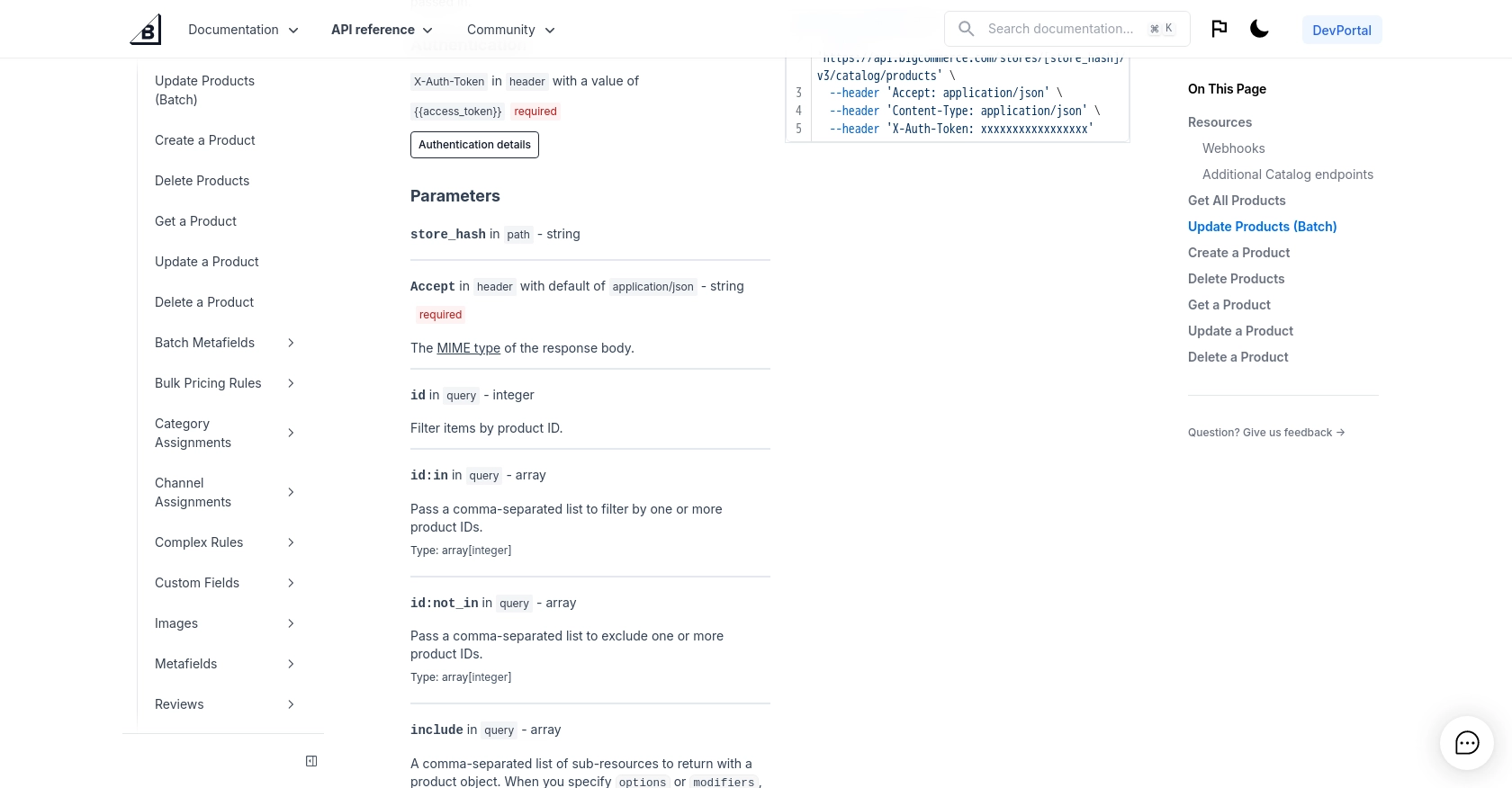
Conclusion and Best Practices for BigCommerce API Integration
Integrating with the BigCommerce API using PHP provides a powerful way to automate and manage your online store's product data. By following the steps outlined in this guide, you can efficiently create and update products, ensuring your store remains accurate and up-to-date.
Best Practices for Secure and Efficient BigCommerce API Usage
- Securely Store API Credentials: Always store your API credentials, such as the access token, in a secure environment. Avoid hardcoding them directly in your codebase.
- Handle Rate Limiting: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic and exponential backoff strategies to manage requests efficiently.
- Validate API Responses: Always check the response status codes and handle errors gracefully. Refer to the BigCommerce API documentation for detailed information on error codes.
- Optimize Data Handling: When dealing with large datasets, consider implementing pagination and filtering to optimize performance and reduce load times.
Streamlining Integrations with Endgrate
While integrating with the BigCommerce API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate's website.
Read More
Ready to get started?