How to Create Or Update Purchase Orders with the Quickbooks API in Python
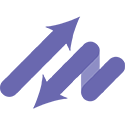
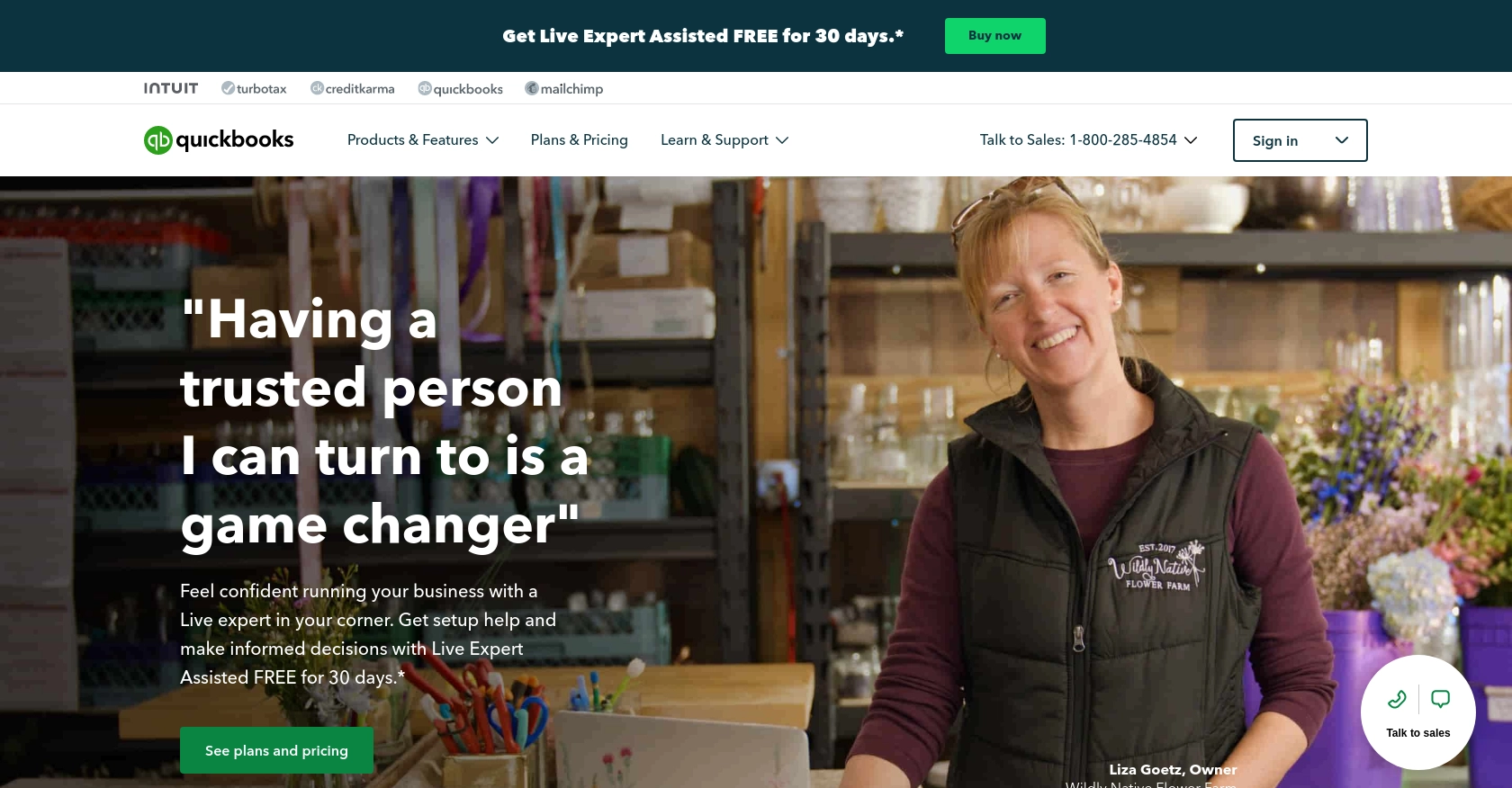
Introduction to QuickBooks API for Purchase Orders
QuickBooks is a widely-used accounting software that helps businesses manage their finances efficiently. It offers a comprehensive suite of tools for invoicing, expense tracking, payroll, and more. QuickBooks is particularly popular among small to medium-sized businesses due to its user-friendly interface and robust feature set.
Integrating with the QuickBooks API allows developers to automate and streamline financial operations, such as managing purchase orders. By connecting to the QuickBooks API, developers can create or update purchase orders programmatically, ensuring that financial data is accurate and up-to-date. For example, a developer might use the QuickBooks API to automatically generate purchase orders from an inventory management system, reducing manual data entry and minimizing errors.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start creating or updating purchase orders with the QuickBooks API, you'll need to set up a sandbox account. This environment allows you to test your integration without affecting live data, ensuring that your application functions correctly before deployment.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. If you don't have one, follow these steps:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" and fill in the required information to create your account.
- Once registered, log in to your QuickBooks Developer account.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, you'll need to set up a sandbox company:
- Navigate to the "Dashboard" in your QuickBooks Developer account.
- Select "Sandbox" from the menu and click on "Add Sandbox Company."
- Follow the prompts to create a new sandbox company, which will be used for testing your API integration.
Creating a QuickBooks App for OAuth Authentication
QuickBooks uses OAuth 2.0 for authentication. To interact with the API, you'll need to create an app to obtain your client ID and client secret:
- Go to the App Management section in your QuickBooks Developer account.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the necessary details, such as app name and scope of access.
- Once created, navigate to the "Keys & OAuth" section to find your client ID and client secret.
Configuring OAuth 2.0 for API Access
With your app created, configure OAuth 2.0 to authenticate API requests:
- In the "Keys & OAuth" section, set the redirect URI to a valid endpoint in your application.
- Use the client ID and client secret to generate access tokens for API calls.
- Refer to the QuickBooks OAuth 2.0 documentation for detailed instructions on obtaining and refreshing tokens.
With your sandbox account and app set up, you're ready to start integrating with the QuickBooks API to manage purchase orders programmatically.
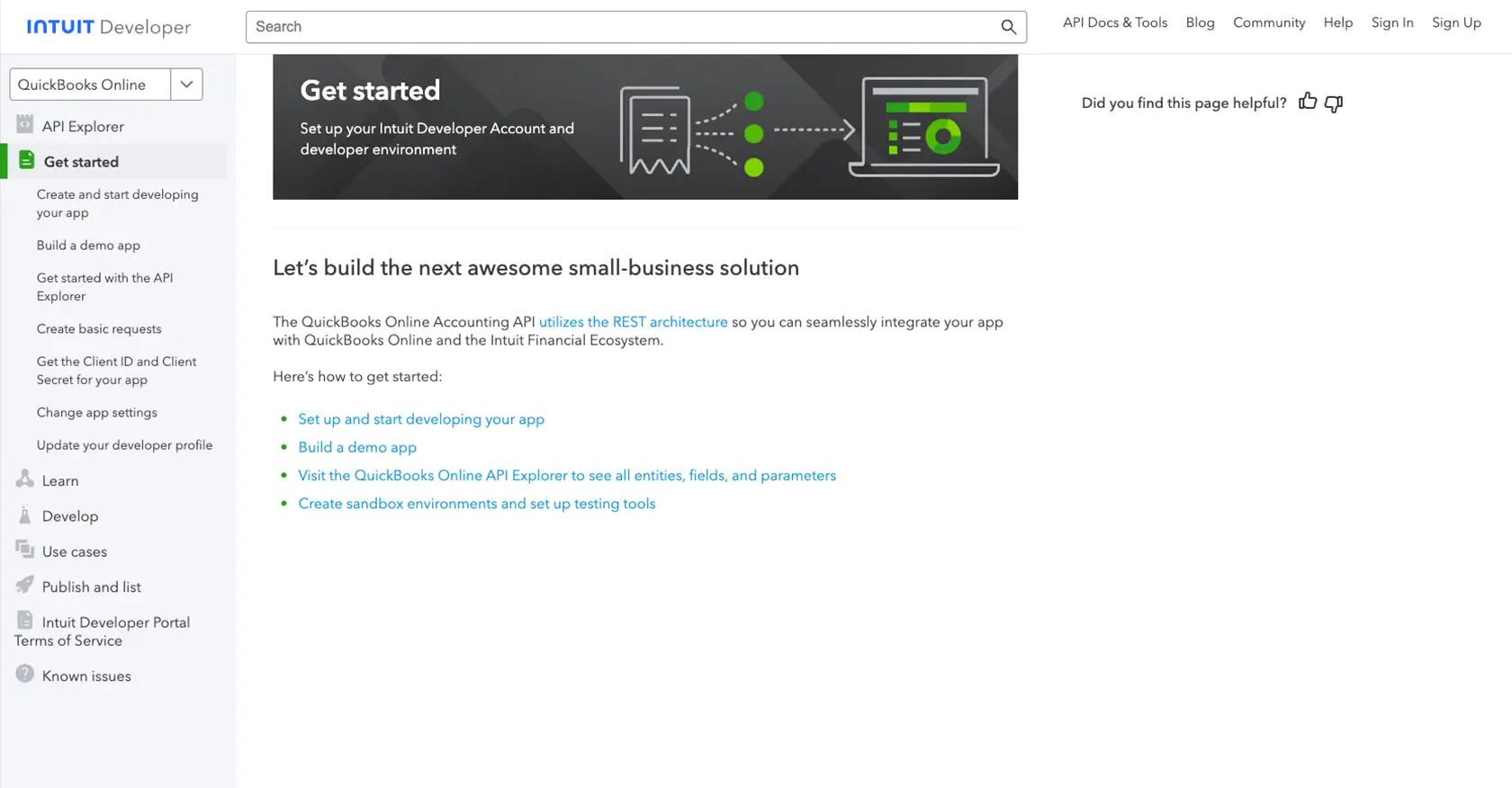
sbb-itb-96038d7
Making API Calls to Create or Update Purchase Orders with QuickBooks API in Python
To interact with the QuickBooks API for creating or updating purchase orders, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your Python environment, making API calls, and handling responses effectively.
Setting Up Your Python Environment for QuickBooks API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Creating a Purchase Order with QuickBooks API Using Python
To create a purchase order, you'll need to send a POST request to the QuickBooks API endpoint. Here's a step-by-step guide:
import requests
import json
# Set the API endpoint
url = "https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/purchaseorder"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
# Define the purchase order data
purchase_order = {
"VendorRef": {
"value": "123"
},
"Line": [
{
"DetailType": "ItemBasedExpenseLineDetail",
"Amount": 100.00,
"ItemBasedExpenseLineDetail": {
"ItemRef": {
"value": "1"
}
}
}
]
}
# Send the POST request
response = requests.post(url, headers=headers, data=json.dumps(purchase_order))
# Check the response status
if response.status_code == 200:
print("Purchase Order Created Successfully")
else:
print("Failed to Create Purchase Order:", response.json())
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token. The purchase_order
dictionary contains the data for the purchase order you wish to create.
Updating a Purchase Order with QuickBooks API Using Python
To update an existing purchase order, you'll need to send a POST request with the updated data:
# Set the API endpoint for updating
url_update = "https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/purchaseorder/ORDER_ID"
# Define the updated purchase order data
updated_purchase_order = {
"Id": "ORDER_ID",
"SyncToken": "0",
"VendorRef": {
"value": "123"
},
"Line": [
{
"DetailType": "ItemBasedExpenseLineDetail",
"Amount": 150.00,
"ItemBasedExpenseLineDetail": {
"ItemRef": {
"value": "1"
}
}
}
]
}
# Send the POST request for update
response_update = requests.post(url_update, headers=headers, data=json.dumps(updated_purchase_order))
# Check the response status
if response_update.status_code == 200:
print("Purchase Order Updated Successfully")
else:
print("Failed to Update Purchase Order:", response_update.json())
Replace ORDER_ID
with the ID of the purchase order you wish to update. Ensure the SyncToken
is correct to avoid conflicts.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors gracefully. QuickBooks API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. Always check the response status and handle errors accordingly:
if response.status_code != 200:
error_info = response.json()
print("Error:", error_info.get("Fault", {}).get("Error", [{}])[0].get("Message"))
To verify the success of your API requests, you can log into your QuickBooks sandbox account and check the purchase orders section to ensure the changes reflect accurately.
For more detailed information on error codes, refer to the QuickBooks API documentation.
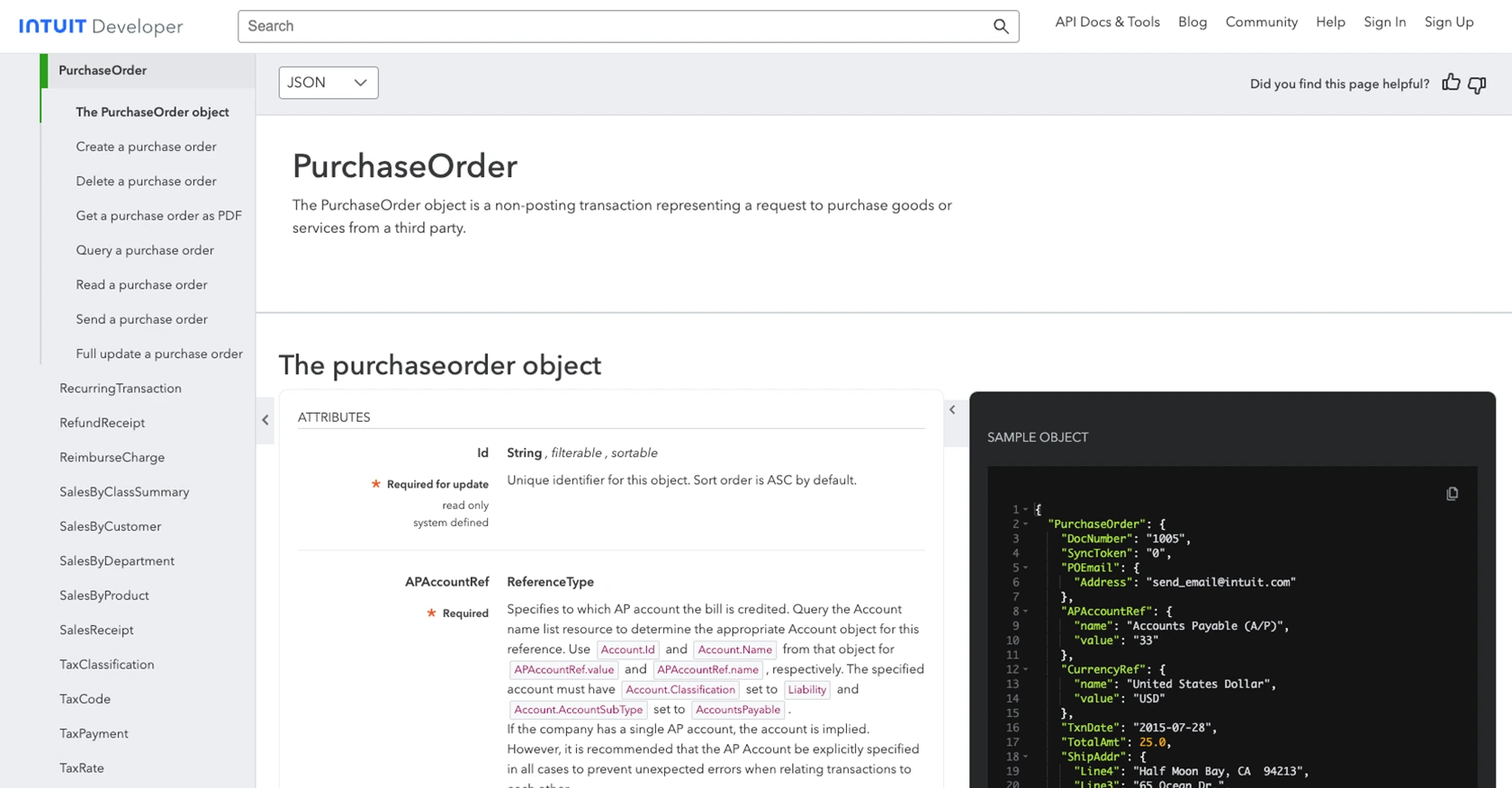
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API to create or update purchase orders can significantly enhance your financial management processes by automating routine tasks and ensuring data accuracy. By following the steps outlined in this guide, you can efficiently set up your development environment, authenticate using OAuth 2.0, and interact with the QuickBooks API using Python.
Best Practices for Secure and Efficient QuickBooks API Usage
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Ensure your application handles these limits gracefully by implementing retry logic or backoff strategies. Refer to the QuickBooks API documentation for specific rate limit details.
- Data Standardization: When integrating with multiple systems, standardize data fields to maintain consistency across platforms. This will help in seamless data exchange and reduce errors.
Streamlining Integration with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including QuickBooks, allowing you to focus on your core product while outsourcing integration complexities. Save time and resources by building once for each use case and providing an intuitive integration experience for your customers.
For more information on how Endgrate can enhance your integration strategy, visit Endgrate.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/purchaseorder
Ready to get started?